python多项式回归
Let’s start with an example. We want to predict the Price of a home based on the Area and Age. The function below was used to generate Home Prices and we can pretend this is “real-world data” and our “job” is to create a model which will predict the Price based on Area and Age:
让我们从一个例子开始。 我们想根据面积和年龄来预测房屋价格。 下面的函数用于生成房屋价格,我们可以假装这是“真实数据”,而我们的“工作”是创建一个模型,该模型将根据面积和年龄预测价格:
价格= -3 *面积-10 *年龄+ 0.033 *面积²-0.0000571 *面积³+ 500 (Price = -3*Area -10*Age + 0.033*Area² -0.0000571*Area³ + 500)
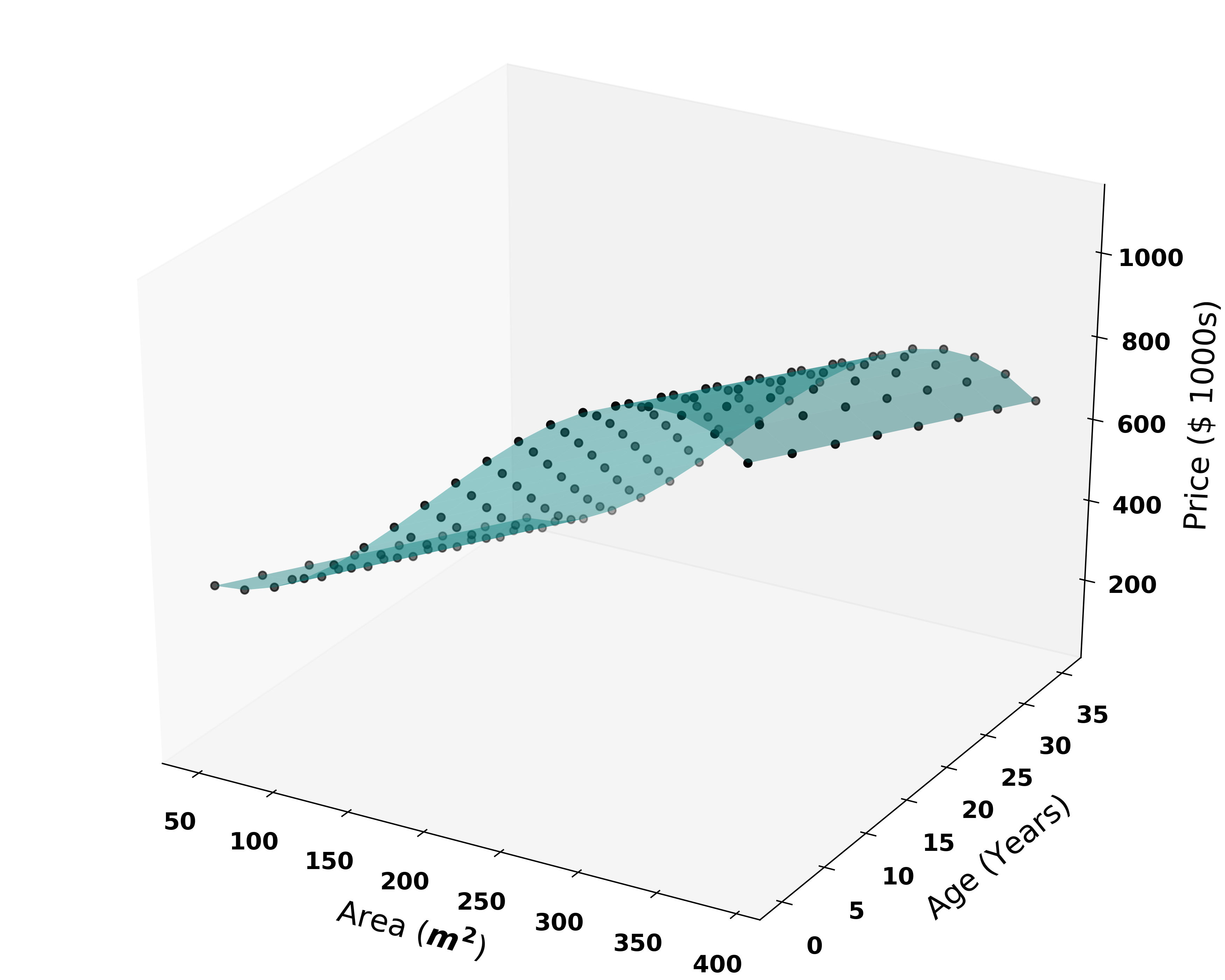
线性模型 (Linear Model)
Let’s suppose we just want to create a very simple Linear Regression model that predicts the Price using slope coefficients c1 and c2 and the y-intercept c0:
假设我们只想创建一个非常简单的线性回归模型,该模型使用斜率系数c1和c2以及y轴截距 c0来预测价格:
Price = c1*Area+c2*Age + c0
价格= c1 *面积+ c2 *年龄+ c0
We’ll load the data and implement Scikit-Learn’s Linear Regression. Behind the scenes, model coefficients (c0, c1, c2) are computed by minimizing the sum of squares of individual errors between target variable y and the model prediction:
我们将加载数据并实现Scikit-Learn的线性回归 。 在幕后,通过最小化目标变量y与模型预测之间的各个误差的平方和来计算模型系数(c0,c1,c2):
But you see we don’t do a very good job with this model.
但是您会看到我们在此模型上做得不好。
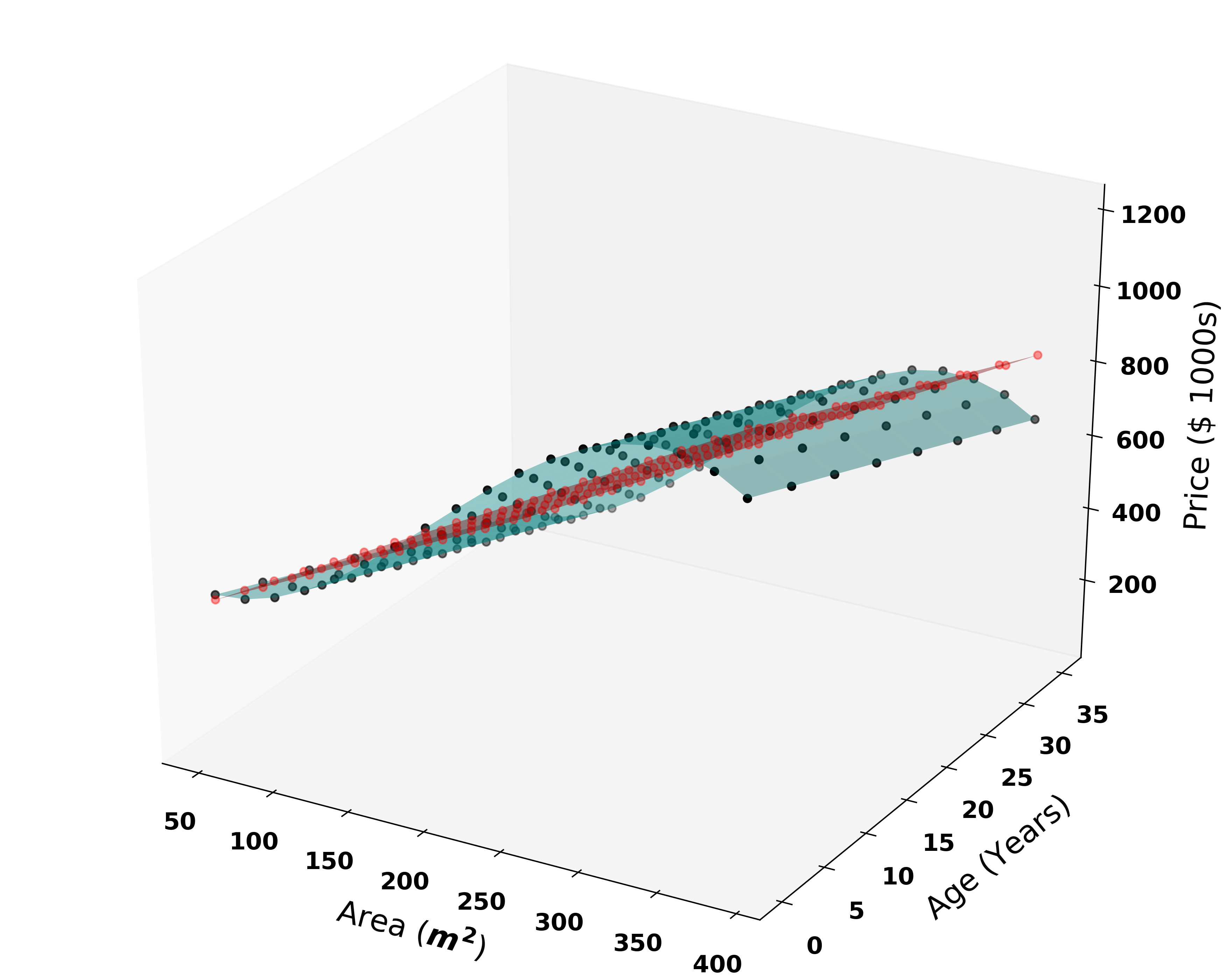
多项式回归模型 (Polynomial Regression Model)
Next, let’s implement the Polynomial Regression model because it’s the right tool for the job. Rewriting the initial function used to generate the home Prices, where x1 = Area, and x2 = Age, we get the following:
接下来,让我们实现多项式回归模型,因为它是这项工作的正确工具。 重写用于生成房屋价格的初始函数,其中x1 =面积,x2 =年龄,我们得到以下信息:
价格= -3 * x1 -10 * x2 + 0.033 *x1²-0.0000571 *x1³+ 500 (Price = -3*x1 -10*x2 + 0.033*x1² -0.0000571*x1³ + 500)
So now instead of the Linear model (Price = c1*x1 +c2*x2 + c0), Polynomial Regression requires we transform the variables x1 and x2. For example, if we want to fit a 2nd-degree polynomial, the input variables are transformed as follows:
因此,现在多项式回归代替线性模型(价格= c1 * x1 + c2 * x2 + c0),需要转换变量x1和x2。 例如,如果要拟合二阶多项式,则输入变量的转换如下:
1, x1, x2, x1², x1x2, x2²
1,x1,x2,x1²,x1x2,x2²
But our 3rd-degree polynomial version will be:
但是我们的三阶多项式将是:
1, x1, x2, x1², x1x2, x2², x1³, x1²x2, x1x2², x2³
1,x1,x2,x1²,x1x2,x2²,x1³,x1²x2,x1x2²,x2³
Then we can use the Linear model with the polynomially transformed input features and create a Polynomial Regression model in the form of:
然后,我们可以将线性模型与多项式转换后的输入特征一起使用,并创建以下形式的多项式回归模型:
Price = 0*1 + c1*x1 + c2*x2 +c3*x1² + c4*x1x2 + … + cn*x2³ + c0
价格= 0 * 1 + c1 * x1 + c2 * x2 + c3 *x1²+ c4 * x1x2 +…+ cn *x2³+ c0
(0*1 relates to the bias (1s) column)
(0 * 1与偏置(1s)列有关)
After training the model on the data we can check the coefficients and see if they match our original function used to generate home prices:
在对数据进行模型训练之后,我们可以检查系数,看看它们是否与用于生成房屋价格的原始函数匹配:
Original Function:
原始功能:
价格= -3 * x1 -10 * x2 + 0.033 *x1²-0.0000571 *x1³+ 500 (Price = -3*x1 -10*x2 + 0.033*x1² -0.0000571*x1³ + 500)
Polynomial Regression model coefficients:
多项式回归模型系数:
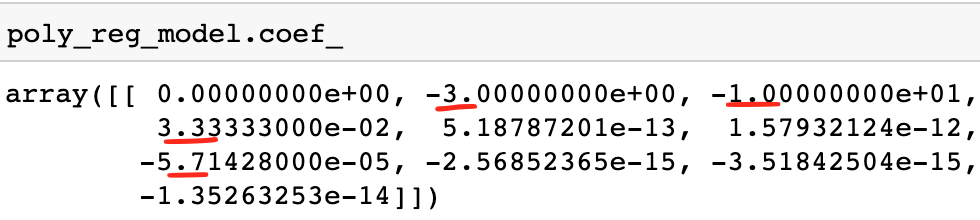
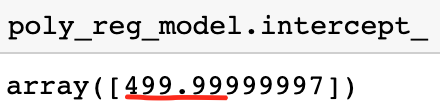
and indeed they match!
确实匹配!
Now you can see we do a much better job.
现在您可以看到我们做得更好。
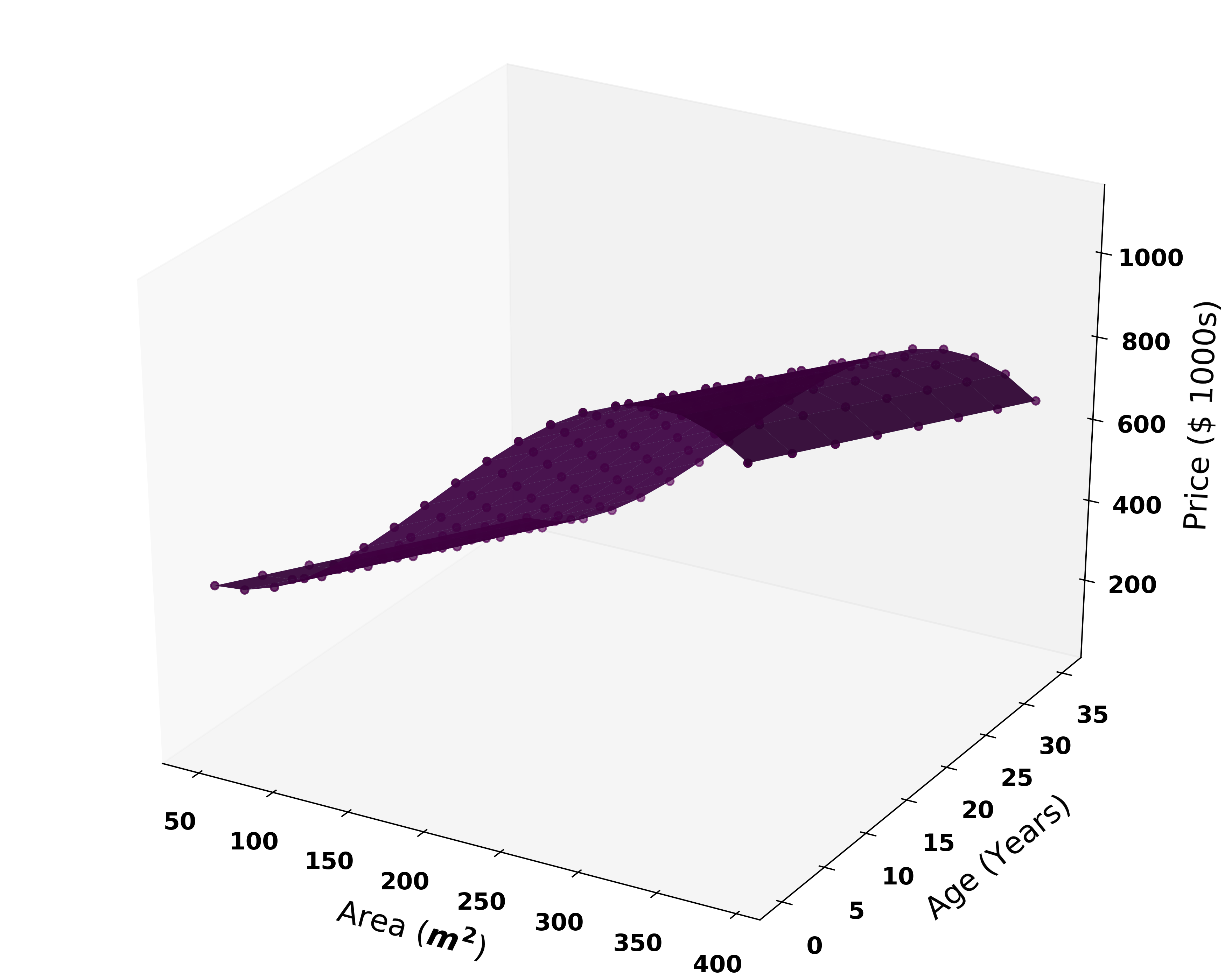
And there you have it, now you know how to implement a Polynomial Regression model in Python. Entire code can be found here.
有了它,现在您知道如何在Python中实现多项式回归模型。 完整的代码可以在这里找到。
结束语 (Closing remarks)
- If this were a real-world ML task, we should have split data into training and testing sets, and evaluated the model on the testing set. 如果这是现实世界中的ML任务,我们应该将数据分为训练和测试集,并在测试集上评估模型。
- It’s better to use other accuracy metrics such as RMSE because MRE will be undefined if there’s a 0 in the y values. 最好使用其他精度度量标准,例如RMSE,因为如果y值中为0,则MRE将不确定。
翻译自: https://medium.com/@nikola.kuzmic945/how-to-implement-a-polynomial-regression-model-in-python-6250ce96ba61
python多项式回归
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如若转载,请注明出处:http://www.mzph.cn/news/389367.shtml
如若内容造成侵权/违法违规/事实不符,请联系多彩编程网进行投诉反馈email:809451989@qq.com,一经查实,立即删除!