分页查询实体:PageQuery
package com.example.demo.demos.model.query;import com.baomidou.mybatisplus.core.metadata.OrderItem;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import lombok.Data;
import org.springframework.util.StringUtils;/*** 分页查询实体*/
@Data
public class PageQuery {//页码private Integer pageNo = 1;//分页大小private Integer pageSize = 10;//排序字段private String sortBy;//是否升序private Boolean isIncrease = true;/*** PageQuery转Page** @param orderItem 排序条件* @return Page对象*/public <T> Page<T> toMyBatisPlusPage(OrderItem... orderItem) {//TODO:构建分页条件//分页条件Page<T> page = new Page<>(pageNo, pageSize);//排序条件if (StringUtils.hasText(sortBy)) {page.addOrder(new OrderItem(sortBy, isIncrease));} else if (orderItem != null) {//默认按照更新时间排序-[此处改为默认按照id排序]page.addOrder(orderItem);}//返回return page;}/*** PageQuery转Page:根据Id排序** @param orderBy 排序字段* @param isIncrease 是否升序* @return Page对象*/public <T> Page<T> toMyBatisPlusPage(String orderBy, Boolean isIncrease) {return toMyBatisPlusPage(new OrderItem(orderBy, isIncrease));}/*** PageQuery转Page:根据Id排序** @return Page对象*/public <T> Page<T> toMyBatisPlusPageSortById() {return toMyBatisPlusPage(new OrderItem("id", true));}/*** PageQuery转Page:根据create_time排序** @return Page对象*/public <T> Page<T> toMyBatisPlusPageSortByCreateTime() {return toMyBatisPlusPage(new OrderItem("create_time", true));}/*** PageQuery转Page:根据update_time排序** @return Page对象*/public <T> Page<T> toMyBatisPlusPageSortByUpdateTime() {return toMyBatisPlusPage(new OrderItem("update_time", true));}
}
分页响应实体:PageDTO
package com.example.demo.demos.model.dto;import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import lombok.Data;
import org.springframework.beans.BeanUtils;
import org.springframework.util.CollectionUtils;import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.function.Function;
import java.util.stream.Collectors;/*** 分页查询结果*/
@Data
public class PageDTO<T> {//总条数private Long total;//总页数private Long pages;//集合private List<T> list;/*** Page转换为PageDTO** @param page MyBatis Plus分页对象* @param clazz 类型参数* @return PageDTO*/public static <PO, VO> PageDTO<VO> of(Page<PO> page, Class<VO> clazz) {//TODO:构建Vo结果PageDTO<VO> pageDTO = new PageDTO<>();//总条数pageDTO.setTotal(page.getTotal());//总页数pageDTO.setPages(page.getPages());//当前页数据List<PO> records = page.getRecords();//转换为voif (CollectionUtils.isEmpty(records)) {pageDTO.setList(Collections.emptyList());return pageDTO;}//forList<VO> voList = new ArrayList<>(records.size());records.forEach(po -> {try {VO vo = clazz.newInstance();BeanUtils.copyProperties(po, vo, clazz);voList.add(vo);} catch (InstantiationException | IllegalAccessException e) {e.printStackTrace();}});pageDTO.setList(voList);//返回结果return pageDTO;}/*** Page转换为PageDTO-【自定义PO->VO的转换方法】** @param page MyBatis Plus分页对象* @param convertor PO->VO的转换逻辑* @return PageDTO*/public static <PO, VO> PageDTO<VO> of(Page<PO> page, Function<PO,VO> convertor) {//TODO:构建Vo结果PageDTO<VO> pageDTO = new PageDTO<>();//总条数pageDTO.setTotal(page.getTotal());//总页数pageDTO.setPages(page.getPages());//当前页数据List<PO> records = page.getRecords();//转换为voif (CollectionUtils.isEmpty(records)) {pageDTO.setList(Collections.emptyList());return pageDTO;}List<VO> voList = records.stream().map(convertor).collect(Collectors.toList());pageDTO.setList(voList);//返回结果return pageDTO;}}
使用示例
封装之前
@Overridepublic PageDTO<ProductVo> queryProductsPage(ProductQuery productQuery) {//获取参数String name = productQuery.getName();Boolean status = productQuery.getStatus();Double minPrice = productQuery.getMinPrice();Double maxPrice = productQuery.getMaxPrice();//TODO:构建分页条件//分页条件Page<Product> page = new Page<>(productQuery.getPageNo(), productQuery.getPageSize());//排序条件if (StringUtils.hasText(productQuery.getSortBy())){page.addOrder(new OrderItem(productQuery.getSortBy(),productQuery.getIsIncrease()));}else{//默认按照更新时间排序-[此处改为默认按照id排序]page.addOrder(new OrderItem("id",true));}//TODO:分页查询Page<Product> pageResult = lambdaQuery().like(name != null && name != "", Product::getName, name).eq(status != null, Product::getStatus, status).ge(minPrice != null && minPrice != 0, Product::getPrice, minPrice).le(maxPrice != null && maxPrice != 0, Product::getPrice, maxPrice).page(page);//TODO:构建Vo结果PageDTO<ProductVo> productPageDTO = new PageDTO<>();//总条数productPageDTO.setTotal(pageResult.getTotal());//总页数productPageDTO.setPages(pageResult.getPages());//当前页数据List<Product> records = pageResult.getRecords();//转换为voif (CollectionUtils.isEmpty(records)){productPageDTO.setList(Collections.emptyList());return productPageDTO;}//返回结果List<ProductVo> productVoList = records.stream().map(product -> {ProductVo productVo = new ProductVo();BeanUtils.copyProperties(product, productVo);return productVo;}).collect(Collectors.toList());productPageDTO.setList(productVoList);return productPageDTO;}
封装之后
@Overridepublic PageDTO<ProductVo> queryProductsPage(ProductQuery productQuery) {//获取参数String name = productQuery.getName();Boolean status = productQuery.getStatus();Double minPrice = productQuery.getMinPrice();Double maxPrice = productQuery.getMaxPrice();//TODO:构建分页条件//调用封装好的方法Page<Product> page = productQuery.toMyBatisPlusPageSortById();//TODO:分页查询Page<Product> pageResult = lambdaQuery().like(name != null && name != "", Product::getName, name).eq(status != null, Product::getStatus, status).ge(minPrice != null && minPrice != 0, Product::getPrice, minPrice).le(maxPrice != null && maxPrice != 0, Product::getPrice, maxPrice).page(page);//TODO:构建Vo结果//方式1:默认转换PO->VO
// return PageDTO.of(pageResult, ProductVo.class);//方式2:自定义转换PO->VOreturn PageDTO.of(pageResult,(product -> {//自定义转换逻辑ProductVo productVo = new ProductVo();BeanUtils.copyProperties(product,productVo);return productVo;}));}
接口测试
这里省略Controller、Mapper、Model、Service等其它代码。
查询参数:
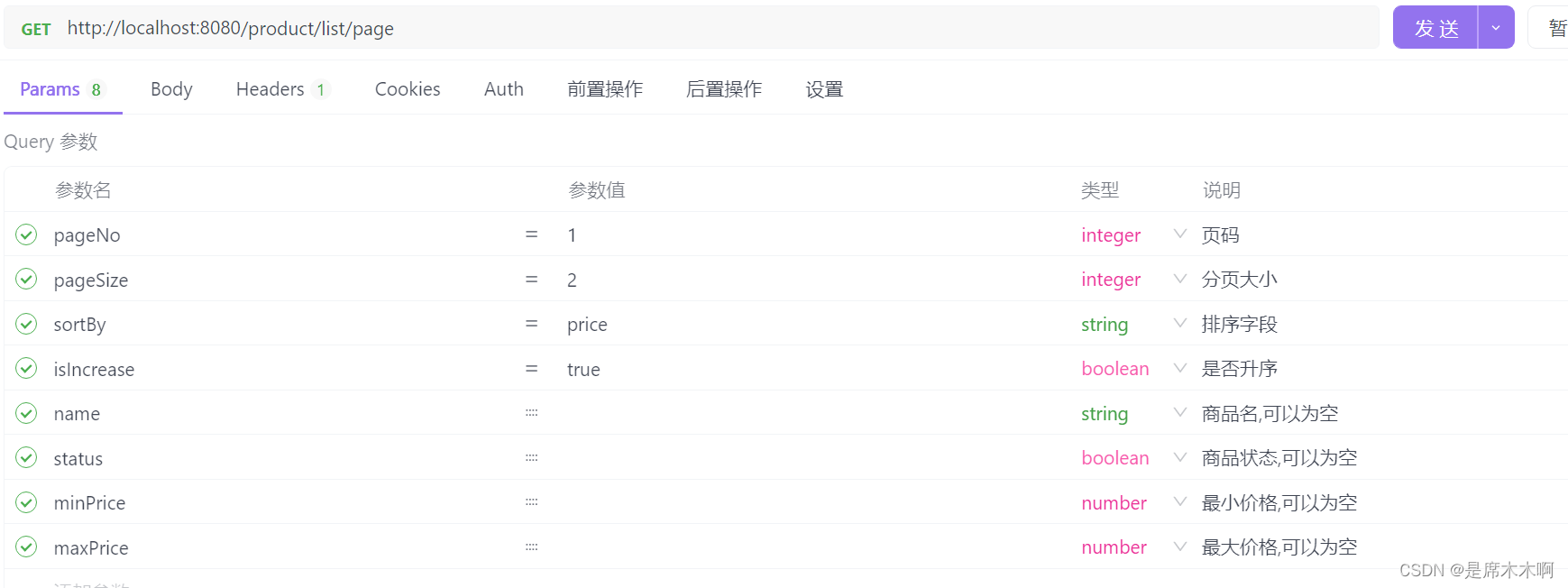
查询结果:
{"code": 200,"data": {"total": 3,"pages": 2,"list": [{"id": 2,"name": "冰可乐","price": 12.12,"address": "淮阳","status": false,"createTime": "2024-02-18 00:35:07","updateTime": null,"enumState": 2,"info": "{\"bar\": \"baz\", \"balance\": 7.77, \"active\": false}"},{"id": 4,"name": "雪糕","price": 38.47,"address": "阜阳","status": true,"createTime": "2024-02-18 01:23:12","updateTime": null,"enumState": 1,"info": "{\"bar\": \"baz\", \"balance\": 7.77, \"active\": false}"}]},"message": "ok"
}