Vuex 的状态存储是响应式的。当 Vue 组件从 store 中读取状态的时候,若 store 中的状态发生变化,那么相应的组件也会相应地得到高效更新。
你不能直接改变 store 中的状态。改变 store 中的状态的唯一途径就是显式地提交 (commit) mutation。
vuex有哪几种属性
有五种,分别是State、 Getter、Mutation 、Action、Module
state => 基本数据(数据源存放地)
getters => 从基本数据派生出来的数据
mutations => 提交更改数据的方法,同步!
actions => 像一个装饰器,包裹mutations,使之可以异步。
modules => 模块化Vuex
a) 在vue组件里面,通过dispatch来触发actions提交修改数据的操作。
b) 然后再通过actions的commit来触发mutations来修改数据。
c) mutations接收到commit的请求,就会自动通过Mutate来修改state(数据中心里面的数据状态)里面的数据。
d) 最后由store触发每一个调用它的组件的更新
Vuex的作用:项目数据状态的集中管理,复杂组件(如兄弟组件、远房亲戚组件)的数据通信问题。
Vue组件(action里面的dispatch)---》actions(方法commit)---》mutations(Mutate)---》state(getter)---》store更新所有调用vuex的组件(Vue Component组件)
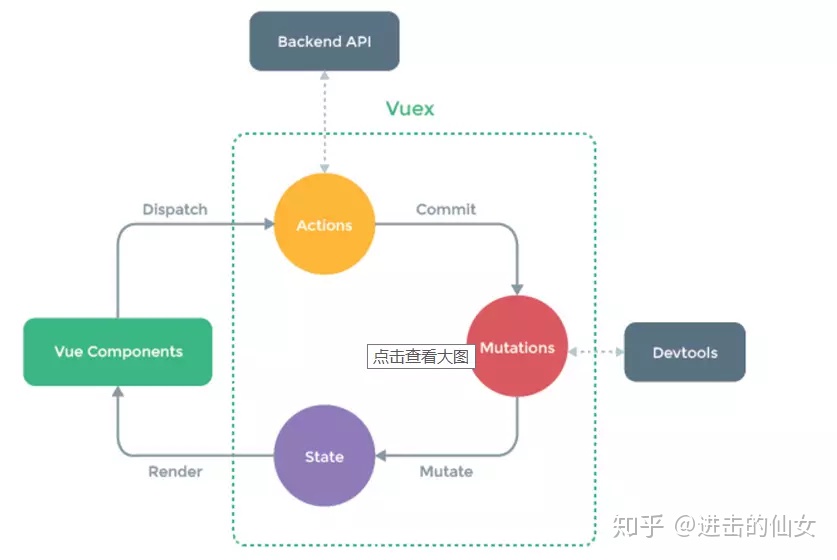
mapState辅助函数
computed: mapState([// 映射 this.count 为 store.state.count'count'
])相当于
computed:{count(){ return this.$store.state.count }
}computed: mapState({// 箭头函数可使代码更简练count: state => state.count,// 传字符串参数 'count' 等同于 `state => state.count`// countAlias : state => state.count,countAlias: 'count',// 为了能够使用 `this` 获取局部状态,必须使用常规函数countPlusLocalState (state) {return state.count + this.localCount}
})
通过属性访问的:
const store = new Vuex.Store({state: {todos: [{ id: 1, text: '...', done: true },{ id: 2, text: '...', done: false }]},getters: {doneTodos: state => {return state.todos.filter(todo => todo.done)},doneTodosCount: (state, getters) => {return getters.doneTodos.length}}
})//使用方法
computed: {doneTodosCount () {return this.$store.doneTodos // [{ id: 1, text: '...', done: true }]}
}还有一种情况,自带getter参数的
//使用方法
computed: {doneTodosCount () {return this.$store.getters.doneTodosCount //2}
}
通过方法访问的:(注意,getter 在通过方法访问时,每次都会去进行调用,而不会缓存结果)
const store = new Vuex.Store({state: {todos: [{ id: 1, text: '...', done: true },{ id: 2, text: '...', done: false }]},getters: {getTodoById: (state) => (id) => {return state.todos.find(todo => todo.id === id)}}
})//使用方法
computed: {doneTodosCount () {return this.$store.getters.getTodoById(2) // -> { id: 2, text: '...', done: false }}
}
mapGetters函数
import { mapGetters } from 'vuex'export default {// ...computed: {// 使用对象展开运算符将 getter 混入 computed 对象中...mapGetters(['doneTodosCount','anotherGetter',// ...])}
}
//相当于
export default {// ...computed: {doneTodosCount:this.$store.getters.doneTodosCount,anotherGetter :this.$store.getters.anotherGetter }
}
mutation特点
每个 mutation 都有一个字符串的事件类型 (type)和 一个回调函数 (handler)。这个回调函数就是我们实际进行状态更改的地方,并且它会接受 state 作为第一个参数:
const store = new Vuex.Store({state: {count: 1},mutations: {increment (state) {// 变更状态state.count++}}
})// ...
mutations: {increment (state, n) {state.count += n}
}store.commit('increment', 10)