#include<iostream>
#include<math.h>
using namespace std;
class Circle
{public:float Center(int t);void Compute(void );void BackTrack(int t);float min; float *x; float *r; float *result; int n;
};
float Circle::Center(int t)
{float temp = 0;for(int j=1;j<t;j++){float valuex = x[j]+2.0*sqrt(r[t]*r[j]);if(valuex > temp)temp = valuex; } return temp;} void Circle::Compute(void){float low = 0,high = 0;for(int i=1;i<=n;i++){if(x[i]-r[i]<low)low = x[i] - r[i];if(x[i]+r[i]>high)high = x[i] + r[i];}if(high-low<min)min = high - low;}
void Circle::BackTrack(int t)
{if(t>n){Compute();}else{for(int j=t;j<=n;j++){swap(r[t],r[j]);float centerx = Center(t);if(centerx+r[t]+r[1]<min){result[j]=r[t];x[t] = centerx;BackTrack(t+1);}swap(r[t],r[j]);} }
}
float CirclePerm(int n,float *a)
{Circle X;X.n =n;X.r =a;X.min = 100000;float *x =new float [n+1];X.x= x;float *result = new float [n+1]; X.result= result;X.BackTrack(1);cout<<"输出圆排列后圆半径的序列:";for(int i=1;i<=n;i++){cout<<X.result[i]<<" ";}cout<<endl;delete[] x;return X.min;
}
int main()
{int n;cout<<"输入圆的个数:";cin>>n; cout<<"输入圆半径序列:";float a[n+1];for(int i=1;i<=n;i++){cin>>a[i];}cout<<CirclePerm(n,a);
}
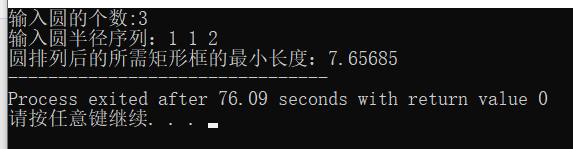