appweb ejs
HI! Welcome to NODE AND EJS TEMPLATE ENGINE SERIES. Today, we will see how we can work with EJS and routes?
嗨! 欢迎使用NODE和EJS模板引擎系列 。 今天,我们将看到如何使用EJS和路由?
A route is like a sub domain with it's own function or web page/document.
路由就像具有其自身功能或网页/文档的子域。
For Example: On most websites, we see: .../home, .../about, .../admin
例如:在大多数网站上,我们看到: ... / home,... / about,... / admin
Read basic about EJS, read this article: Node and EJS Template Engine Series | Introduction to EJS
阅读有关EJS的基础知识,阅读本文: 节点和EJS模板引擎系列| EJS简介
In this article, we'll set up an EJS file that will render an html file with a portion of JavaScript code which is variable gotten from the express server code.
在本文中,我们将设置一个EJS文件,该文件将呈现一部分JavaScript代码的html文件,该JavaScript代码是从express服务器代码获取的 。
In our app.js file, we will setup 2 routes. The first will be our root route (or home route) and the second will be a /user/:x route.
在我们的app.js文件中,我们将设置2条路由。 第一个是我们的根路由(或本地路由),第二个是/ user /:x路由。
The x represents user request under the route user.
x表示路由用户下的用户请求。
We are going to write some code that will copy the user request and save it in a variable. To that, we will use req.params.x; where "x" represents the user request and will be saved in a variable called data.
我们将编写一些代码来复制用户请求并将其保存在变量中。 为此,我们将使用req.params.x;。 其中“ x”代表用户请求,将保存在名为data的变量中。
Finally, we then render the ejs file which will capture the content of the data variable and pass it to x in the EJS template.
最后,然后渲染ejs文件,该文件将捕获数据变量的内容并将其传递给EJS模板中的x。
More will be understood as you code.
您编写代码时会了解更多。
Open your text editor and type the following code, Save as app.js.
打开文本编辑器,然后输入以下代码, 另存为app.js。
var express = require('express');
var ejs = require('ejs');
var app = express();
app.set('view engine', 'ejs');
app.get("/", function(req, res) { // root route or home route
res.send('welcome to home page');
});
app.get("/user/:x", function(req, res) { // user route
var data = req.params.x;
res.render("user.ejs", {
x: data
});
});
app.listen(3000, function() {
console.log("server is listening!!!");
});
Now, let's create our ejs file.
现在,让我们创建我们的ejs文件。
Open a text editor and type the following code, Save as user.ejs
打开文本编辑器,然后输入以下代码, 另存为user.ejs
<html>
<h1><%= message%><h1>
</html>
Create a folder in your app.js directory called views as usual.
和往常一样,在app.js目录中创建一个名为views的文件夹。
Cut and paste the ejs file in the views folder.
将ejs文件剪切并粘贴到views文件夹中 。
Take Note: The folder name views is not a random word I selected but it's the reserved folder name where express checks for template engine by default.
请注意:文件夹名称视图不是我选择的随机词,而是默认情况下Express检查模板引擎的保留文件夹名称。
In our express server we used app.set () and passed in our template engine unlike our other examples.
在我们的快递服务器中,我们使用app.set()并将其传递到模板引擎中,这与其他示例不同。
Finally, initiate the app.js file with node app.js in a terminal and view the port in a browser. localhost:3000
最后,在终端中使用节点app.js初始化app.js文件,并在浏览器中查看端口。 本地主机:3000
Or for those using nodemon, use
或对于那些使用nodemon的人,使用
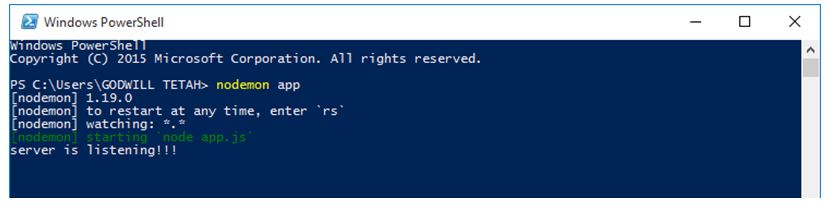
In our browser, we realize that whatever we type after that /user route is used by the ejs template.
在我们的浏览器中,我们意识到ejs模板使用在/ user路由之后键入的任何内容。
We can also add, some basic JavaScript functionalities such as the toUpperCase() method.
我们还可以添加一些基本JavaScript功能,例如toUpperCase()方法。
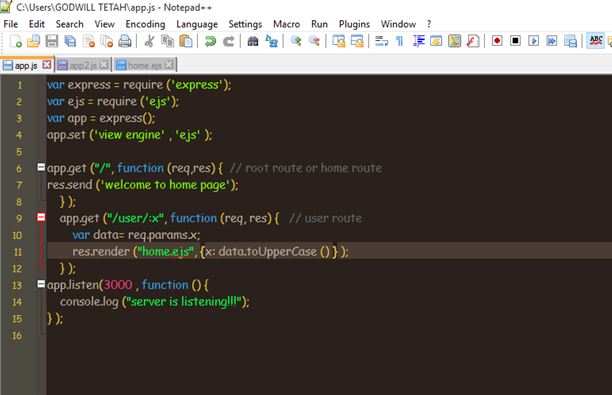
Thanks for coding with me! Feel free to drop a comment or question.
感谢您与我编码! 随意发表评论或问题。
翻译自: https://www.includehelp.com/node-js/ejs-with-express-routes.aspx
appweb ejs