历时3周,记账器项目终于可以运行了,这次项目是基于Vue开发,用到了typeScript和Scss,下面基于项目做一个阶段性的总结,回顾一下项目中用到的知识点。
一.组件
一开始用的是JS对象的写法:
构造选项:
{
data(){return {}
},
methods: {}
}
这次项目用的是类组件和装饰器(可以用js写也可以用ts写)
import Vue from 'vue';import {Component, Prop} from 'vue-property-decorator';@Componentexport default class Tags extends Vue {}
二、computed
computed的用处非常多:比如获取Vuex的数据、获取计算出来的列表
优点:自动根据所需依赖缓存,减少计算
深入了解computed和watch的区别:
good V:Vue-computed和watch的区别zhuanlan.zhihu.comts中computed用法:
@Component({components: {Types, Tags, NumberPad, FormItem},computed: {recordList() {return this.$store.state.recordList;},tagList() {return this.$store.state.tagList;}}})
三、watch
装饰器写法:
@Watch('tags', {immediate: true})onTagChange(tags) {this.outputTags = tags.filter((item) => item.type === '-');this.inputTags = tags.filter((item) => item.type === '+');}
四、Prop、.sync、v-model
Prop传值:
//父组件likes="10"//子组件
props: {likes: Number,
}
子组件收到后,改变值:
//子组件
<button v-on:click="$emit('changeLikes', 5)">按钮
</button>//父组件
v-on:changeLikes="likes += $event"
.sync、v-model都是语法糖
good V:深入了解Vue的修饰符.sync【 vue sync修饰符示例】zhuanlan.zhihu.com五、插槽slot
项目中的Layout组件就是用的插槽
//Layout.vue 组件
<template><div ><slot></slot></div>
</template>//其他组件引用Layout组件
<template><Layout>我是插槽里面的内容,会替换Layout中的slot</Layout>
</template>
具名插槽:
插槽 — Vue.jscn.vuejs.org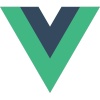
六、svg symbols
svg图标引入:
1、从Iconfont-阿里巴巴矢量图标库中找到需要的图标,下载为svg模式,存到assets>icon里面
2、在vue.config.js中能够配置loader:
const path = require('path')module.exports = {publicPath: process.env.NODE_ENV === 'production'? '/mango-bill': '/',lintOnSave: false,chainWebpack: config => {const dir = path.resolve(__dirname, 'src/assets/icons') // 当前目录config.module.rule('svg-sprite').test(/.svg$/).include.add(dir).end() // 只包含icons目录.use('svg-sprite-loader').loader('svg-sprite-loader').options({extract: false}).end() //不需要解析成文件// .use('svgo-loader').loader('svgo-loader')// .tap(options => ({...options, plugins: [{removeAttrs: {attrs: 'fill'}}]})).end()config.plugin('svg-sprite').use(require('svg-sprite-loader/plugin'), [{plainSprite: true}])config.module.rule('svg').exclude.add(dir) // 其他svg loader排除icons目录}
}
3、创建Icon组件
<template><svg class="icon" @click="$emit('click', $event)"><use :xlink:href="'#' + name"></use></svg></template><script lang="ts">const importAll = (requireContext: __WebpackModuleApi.RequireContext) => requireContext.keys().forEach(requireContext);try {importAll(require.context('../assets/icons', true, /.svg$/));} catch (error) {console.log(error);}export default {name: 'Icon',props: ['name']};
</script><style scoped lang="scss">.icon {width: 1em; height: 1em;vertical-align: -0.15em;fill: currentColor;overflow: hidden;}
</style>
4、在main.ts中挂载为全局组件
import Icon from '@/components/Icon.vue';Vue.component('Icon', Icon);
5、组件中使用
只要写上svg的名称就可以使用了
<Icon name="money"></Icon>
七、Window.localStorage(一般只能存5~10M)
//存
localStorage.setItem('myCat', 'Tom');//取
let cat = localStorage.getItem('myCat');
序列化:即js中的Object转化为字符串
var str=JSON.stringify(obj); //将JSON对象转化为JSON字符
反序列化:即js中JSON字符串转化为Object
var obj = JSON.parse(data); //由JSON字符串转换为JSON对象
八、表驱动编程
例如项目中用到的:":class={key:value,key:value}"
<div :class="{selected: IsSeletedTag(item) >= 0,red:toggle === '-'}"
</div>
九、模块化思想
项目中用到的store就是典型的模块化,Vuex也是模块化