python中格式化字符串
Strings are one of the most essential and used datatypes in programming. It allows the computer to interact and communicate with the world, such as printing instructions or reading input from the user. The ability to manipulate and formate strings give the programmer the power to process and handle text, for example, extract user input from websites forms, data from processed text, and use this data to perform activities such as sentimental analysis. Sentimental analysis is a subfield of natural language processing that allows the computer to classify emotions — negative, positive, or neutral — based on a piece of text.
小号 trings在编程中最重要和使用的数据类型之一。 它允许计算机与世界互动和交流,例如打印指令或从用户那里读取输入。 操纵和组合字符串的能力使程序员能够处理和处理文本,例如,从网站表单中提取用户输入,从处理后的文本中提取数据,并使用该数据执行诸如情感分析之类的活动。 情感分析是自然语言处理的一个子领域,它使计算机可以根据一段文本对情绪(消极,积极或中立)进行分类。
“All sentiment is right; because sentiment has a reference to nothing beyond itself, and is always real, wherever a man is conscious of it.” — David Hume
“所有观点都是正确的; 因为情感无处不在,而无论男人意识到什么,情感总是真实的。” —大卫·休ume
Python is one of the main programming languages used to analyze and manipulate data next to R. Python is used in data analytics to help access databases and communicate with them. It is also useful in importing and exporting data using different web scraping techniques. Once this data is obtained, Python is used to clean and prepare data sets before they are fed to a machine-learning algorithm.
Python是用于分析和处理R之后的数据的主要编程语言之一。Python用于数据分析以帮助访问数据库并与它们进行通信。 在使用不同的Web抓取技术导入和导出数据时,它也很有用。 一旦获得了这些数据,就可以使用Python清理并准备数据集,然后再将其馈送到机器学习算法中。
There are five ways you can use Python to format strings: formatting strings using the modulo operator, formatting using Python’s built-in format function, formatting using Python 3.7 f-strings, formatting manually, and finally using template strings. We will cover each one of these methods as well as when to use each of them.
使用Python格式化字符串的方法有五种:使用模运算符格式化字符串,使用Python的内置格式化函数格式化,使用Python 3.7 f字符串格式化, 手动格式化以及最后使用模板字符串。 我们将介绍这些方法中的每一种以及何时使用它们。
Let’s get right to it…
让我们开始吧...
使用模运算符 (Using the modulo operator)
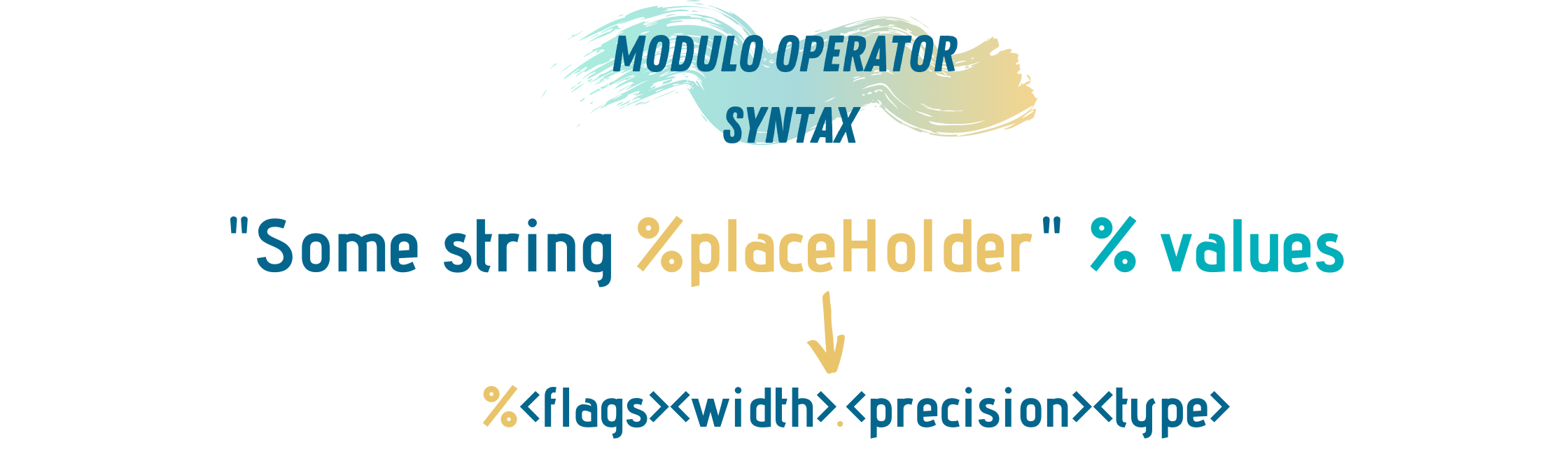
If you wrote code using C or C++ before, this would look very familiar to you. In Python, we can use the modulo operator (%) to perform simple positional formatting. What I call placeHolder here, represents the control specs for formatting and converting the string. The % tells the interpreter that the following characters are the rules for converting the string. The conversion rules include information about the desired width of the result formatted string, the type of the result, and the precision of it — in case of floats.
如果您以前使用C或C ++编写代码,那么您会觉得很熟悉。 在Python中,我们可以使用模运算符(%)进行简单的位置格式设置。 我在这里所谓的placeHolder代表用于格式化和转换字符串的控件规范。 %告诉解释器以下字符是转换字符串的规则。 转换规则包括有关结果格式字符串的所需宽度,结果类型及其精度(如果是浮点数)的信息。
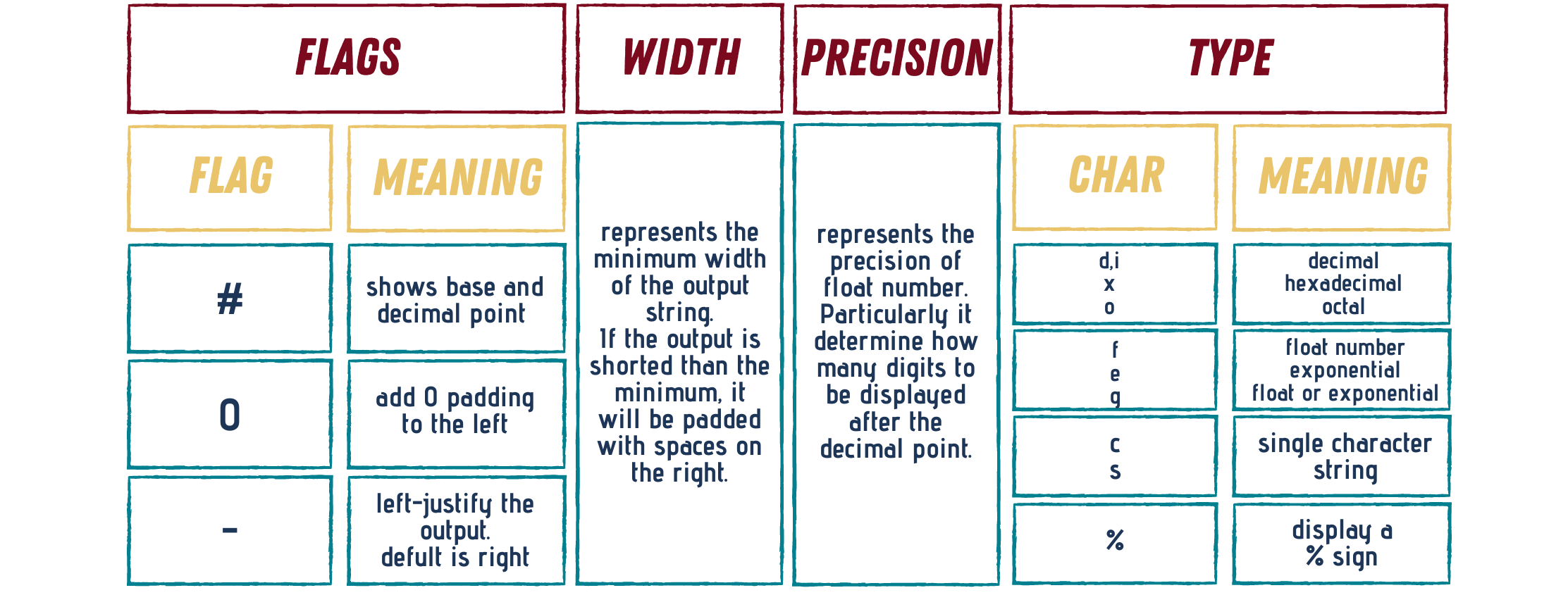
This type of string formatting is sometimes referred to as the “old style” formatting. The placeHolder can refer to values directly or can be specified using keywords arguments.
这种类型的字符串格式有时称为“旧样式”格式。 placeHolder可以直接引用值,也可以使用关键字参数指定。
formatted_str = 'Yesterday I bought %d %s for only %.2f$!' % (10, 2'bananas',2.6743)
If we try to print this string, we will get Yesterday I bought 10 bananas for only 2.67$!
.
如果我们尝试打印此字符串,我们将得到Yesterday I bought 10 bananas for only 2.67$!
。
As you can see here, I had three placeHolders for three values, the first integer, the string — bananas — and the price of the bananas, which is a float. When referring to the float, I decided that a precision of two is all I needed; that’s why only 67 is displayed after the decimal point.
如您在这里看到的,我为三个值设置了三个placeHolders ,它们是第一个整数 , 字符串 -bananas和香蕉的价格,即float 。 当提到浮点数时,我决定只需要2的精度即可。 这就是为什么小数点后仅显示67。
In the previous example, we replaced the placeHolders with the values directly. There is another way we can achieve this mapping, which is through using keyword arguments or by passing a dictionary.
在前面的示例中,我们直接用值替换了placeHolders 。 我们可以通过使用关键字参数或通过传递字典来实现此映射的另一种方法。
name = 'John'
balance = 235765
formatted_str = 'Hello %(name)s, your current balance is %(balance)6.0f dollars.' % {"name":name, "balance":balance}
Using this method, we need to use the {}
instead of the ()
. Because of that, we can also use a dictionary directly to fill in the placeHolders.
使用此方法,我们需要使用{}
而不是()
。 因此,我们还可以直接使用字典来填充placeHolders 。
dictionary = {'quantity': 10, 'name': 'bananas', 'price': 2.6743}
formatted_str = 'I got %(quantity)d %(name)s for $%(price).2f$' % dictionary
The complete code for this section:
本节的完整代码:
使用格式化方法 (Using the format method)
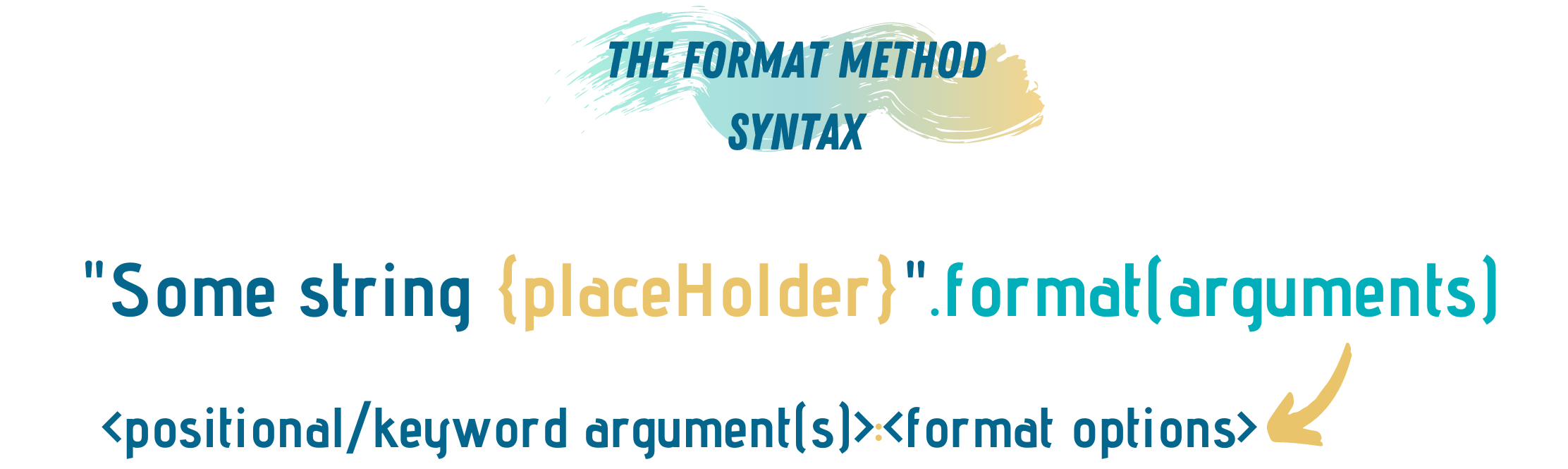
In Python 3, a new method to regulate the % operator syntax was introduced to the language. This is done using the built-in format method, also known as the “new style” formatting. Using the format method has many commonalities with using the % operator. Let’s consider rewriting some of the examples from before:
在Python 3中,该语言引入了一种用于调节%运算符语法的新方法。 这是使用内置的格式化方法(也称为“新样式”格式化)完成的。 使用格式方法与使用%运算符有许多共性。 让我们考虑重写之前的一些示例:
name = 'John'
balance = 235765
formatted_str = 'Hello %(name)s, your current balance is %(balance)6.0f dollars.' % {"name":name, "balance":balance}
Will become:
会变成:
name = 'John'
balance = 235765
formatted_str = 'Hello {name:s}, your current balance is {balance:6.0f} dollars.'.format("name":name, "balance":balance)
We can summarize the differences between the % operator syntax and the format method as:
我们可以将%运算符语法和format方法的区别总结为:
Using the % operator, we use the
()
inside the string, while with format method we use{}
.使用%运算符,我们在字符串内部使用
()
,而使用format方法,则使用{}
。To define the type of the conversion in the % operator, we set it right after the %, while in the format function we set the conversion after
:
inside the{}
.要在%运算符中定义转换的类型,我们将其设置在%之后,而在format函数中,将转换设置在以下位置
:
{}
。
However, if we want to pass a dictionary to the format string, we will need to modify the reforestation slightly. Instead of passing the name of the dictionary, we need to pass a pointer to the dictionary, for example:
但是,如果我们想将字典传递给格式字符串,则需要稍作修改。 除了传递字典名称之外,我们还需要传递一个指向字典的指针,例如:
dictionary = {'quantity': 10, 'name': 'bananas', 'price': 2.6743}
formatted_str = 'I got {quantity:d} {name:s} for {price:.2f}$'.format(**dictionary)
Moreover, we can use the same conversion rules of the % operator that can be used in the format method as well. In Python 3 and up to 3.6, the format method is preferred to the % operator approach. The format method presented a powerful approach to format strings; it didn’t complicate the simple case — such as the examples above — but, at the same time providing new levels to how we format strings.
此外,我们可以使用%运算符的转换规则,该转换规则也可以在format方法中使用。 在Python 3和3.6以下版本中,format方法优于%运算符方法。 格式化方法提供了一种强大的格式化字符串的方法。 它并没有使简单的情况(例如上面的示例)复杂化,但是同时为我们格式化字符串的方式提供了新的层次 。
The complete code for this section:
本节的完整代码:
使用f弦 (Using f-strings)
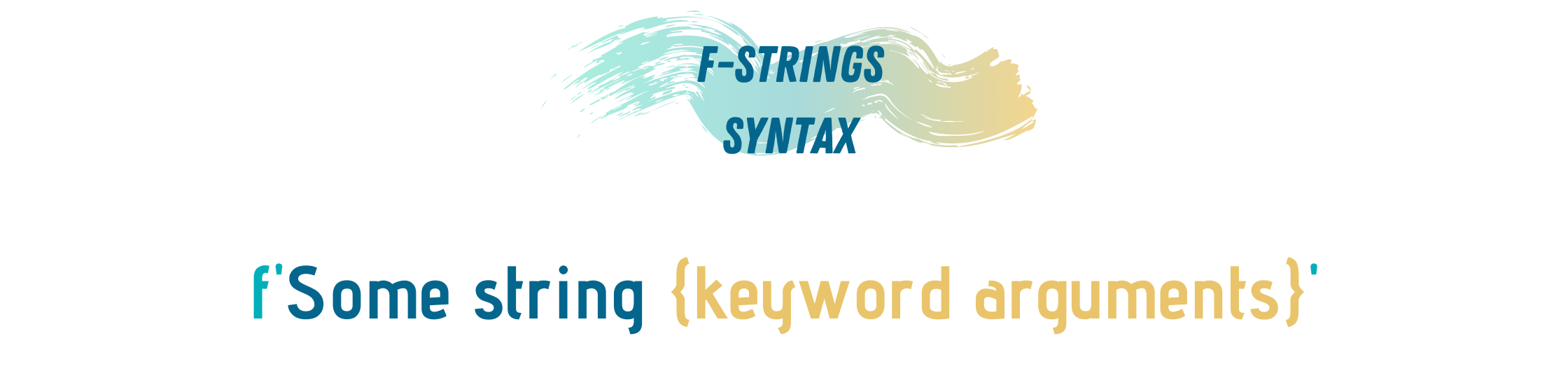
This, in my opinion, the fanciest and most elegant method to format strings. The f-string was presented in Python 3.8 as a more straightforward approach to string formating. Before we get into f-strings, let’s quickly cover the three types of strings in Python 3.8 and up.
我认为,这是格式化字符串的最简单,最优雅的方法。 f字符串在Python 3.8中提出,是一种更简单的字符串格式化方法。 在介绍f字符串之前,让我们快速介绍一下Python 3.8及更高版本中的三种字符串。
Normal/ standard strings: contained between “” or ‘’, example
“Hello, world!”
. These are the most commonly used string type.普通/标准字符串:包含在“”或“”之间,例如
“Hello, world!”
。 这些是最常用的字符串类型。Raw strings: lead by r”, for example
r’hello\nworld’
. In this type of strings, no processing is done on the string. Which means, escape characters don't work in these strings. Instead, they will be printed without any processing. This type of strings is mostly used in regular expressions.原始字符串 :以r“
r'hello\nworld'
,例如r'hello\nworld'
。 在这种类型的字符串中,不对字符串进行任何处理。 这意味着转义字符在这些字符串中不起作用。 取而代之的是,将不进行任何处理就打印它们。 这种类型的字符串通常用于正则表达式中。f-strings: lead by f”, for example,
f’hello John!’
. F-strings as used as an alternative way to format strings.f弦:以f
f'hello John!'
,例如f'hello John!'
。 F字符串用作格式化字符串的替代方法。
f-strings present a simple way to format strings by directly embedding expression into the string. Let’s revisit the examples from before, one more time.
f字符串提供了一种通过直接将表达式直接嵌入到字符串中来格式化字符串的简单方法。 让我们再回顾一次以前的示例。
name = 'John'
balance = 235765
formatted_str = 'Hello {name:s}, your current balance is {balance:6.0f} dollars.'.format("name":name, "balance":balance)
If we want to rewrite this formatting using f-strings, it will look like:
如果我们想使用f字符串重写此格式,则它将类似于:
name = 'John'
balance = 235765
formatted_str = f'Hello {name}, your current balance is {balance} dollars.'
One way f-string simplifies string formatting is, we can directly pass iterables — dictionaries, lists, etc. — to the string, as follows:
f字符串简化字符串格式的一种方法是,我们可以将可迭代对象(字典,列表等)直接传递给字符串,如下所示:
dictionary = {'quantity': 10, 'name': 'bananas', 'price': 2.6743}
formatted_str = f'I got dictionary[quantity] dictionary[name] for dictionary[price]$'#f-string with lists
myList = [1,2,3,4]
formatted_str = f'The last item of the list is myList[-1]'
You can also perform simple arithmetic or logic operations within an f-string directly.
您还可以直接在f字符串中执行简单的算术或逻辑运算。
#Arithmetic
myList = [1,2,3,4]
formatted_str = f'The sum of the last and first items of the list is myList[0] + myList[-1]'
#Logic
formatted_str = f'myList[0] == myList[-1]'
f-strings provide much more freedom to the options we can use inside the string we want to format, such as list indexing, calling list or dictionary methods, or general iterables functions — e.g., len
, sum
, min
, and max
. We can also use conditional statements in an f-string:
f字符串为我们可以在要格式化的字符串中使用的选项提供了更大的自由度,例如列表索引,调用列表或字典方法或常规的可迭代函数(例如len
, sum
, min
和max
。 我们还可以在f字符串中使用条件语句:
age = 21
formatted_str = f'You
The complete code for this section:
本节的完整代码:
手动格式化 (Manual Formatting)
Manual formatting refers to using standard string methods to format strings. Mostly, we use the rjust
, ljust
, and center
to justify the strings. The rjust
method right-justifies a string given a specific width by padding it with spaces on the left. The ljust
adds padding to the right and the center
method adds padding on both sides of the string. These methods do not modify the original string; they instead return a new modified string. There is another string method that can be used to format strings, which is the zfill
method. This method pads a string with a numeric value with zeros on the left.
手动格式化是指使用标准字符串方法来格式化字符串。 通常,我们使用rjust
, ljust
和center
来对齐字符串。 rjust
方法通过在字符串左侧填充空格来对指定宽度的字符串进行右对齐。 ljust
会在右边添加填充,而center
方法会在字符串的两侧添加填充。 这些方法不修改原始字符串。 相反,它们返回一个新的修改后的字符串。 还有另一种可用于格式化字符串的字符串方法,即zfill
方法。 此方法用左边的零填充数字值的字符串。
使用模板字符串 (Using template strings)
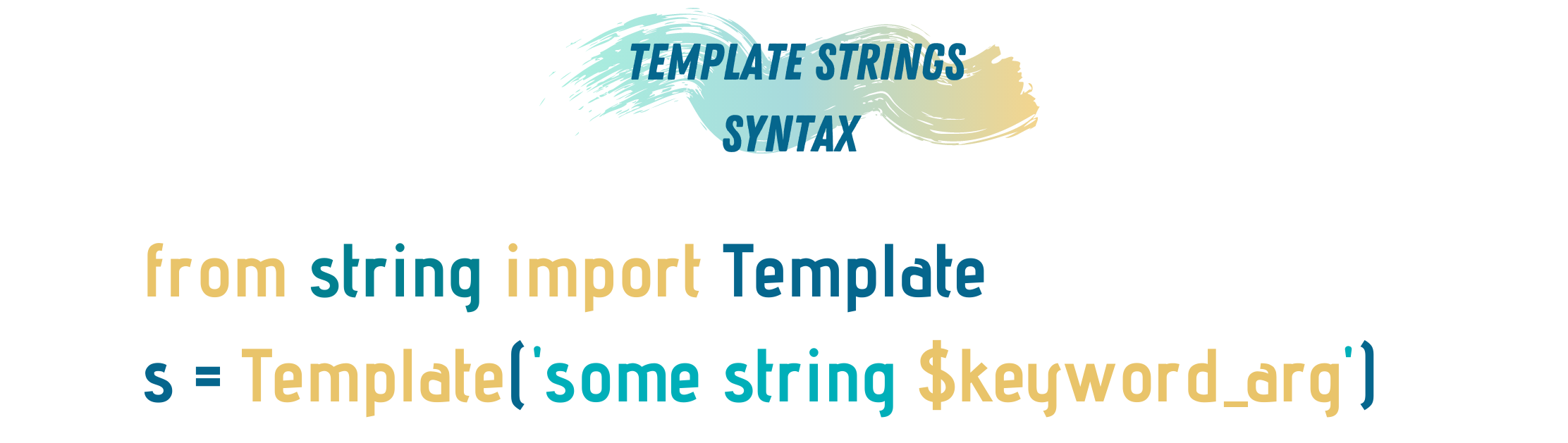
Template strings are an alternative to the % operator, but instead of the %, template strings use the $ sign. Both methods provide what we refer to as substitution formatting. Template strings are not built-in in Python, hence to use them, we need to first import the string
module. Template strings have three rules:
模板字符串是%运算符的替代方法,但是模板字符串使用$符号代替%。 两种方法都提供了我们称为替代格式的内容。 模板字符串不是Python内置的,因此要使用它们,我们需要首先导入string
模块。 模板字符串具有三个规则:
$$
is an escape character.$$
是转义字符。Whatever follows the
$
sign directly is the placeHolder for the template. By default, these placeHolders are restricted to any case-insensitive alphanumeric string. The first non-placeHolder character after the $ sign terminates this placeHolder specs, for example, a whitespace character.直接在
$
符号后面的是模板的placeHolder 。 默认情况下,这些placeHolders限于任何不区分大小写的字母数字字符串。 $符号后的第一个非placeHolder字符终止了该placeHolder规范,例如,空白字符。
These rules ad more are already defined in the string module, so once imported, you can go ahead and use them without the need to define them.
字符串模块中已经定义了这些规则,因此,一旦导入,您就可以继续使用它们而无需定义它们。
The template string has two main methods: the substitute
method and the safe_substitute
method. Both methods take the same argument, which is the substitute mapping. Moreover, both methods return a new formatted string, you can think of the template object as the blueprint for how future strings will be formatted. For example:
模板字符串有两个主要方法: substitute
方法和safe_substitute
方法。 两种方法都采用相同的参数,即替代映射。 而且,这两种方法都返回一个新的格式化字符串,您可以将模板对象视为将来如何格式化字符串的蓝图。 例如:
from string import Template
formatted_str = Template('Hello $who, your current balance is $how dollars.')
formatted_str.substitute(who='John', how=235765)
To see the difference between the substitute and the safe_substitute methods, let’s try using a dictionary as our substitution mapping.
要查看替代方法和safe_substititute方法之间的区别,让我们尝试使用字典作为替代映射。
from string import Template
mapping = dict(who='John', how=235765)
formatted_str = Template('Hello $who, your current balance is $how dollars.')
Returned_str = formatted_str.substitute(mapping)
Assume our dictionary doesn’t have all the keys we need for the mapping, using the substitute
method will give a keyError. Here's where safe_substitute
comes to the help, if this method didn't find the correct key, it will return a string with the placeHolder for the wrong key. For example, this code:
假设我们的字典没有我们映射所需的所有键,那么使用substitute
方法将给出一个keyError 。 这是safe_substitute
提供帮助的地方,如果此方法找不到正确的密钥,它将为错误的密钥返回一个带有placeHolder的字符串。 例如,此代码:
from string import Template
mapping = dict(who='John')
formatted_str = Template('Hello $who, your current balance is $how dollars.')
Returned_str = formatted_str.safe_substitute(mapping)
Will return Hello John, your current balance is $how dollars.
where if I had used the substitute
method, I would've gotten an error.
将返回Hello John, your current balance is $how dollars.
如果我使用了substitute
方法,那我会得到一个错误。
The complete code for this section:
本节的完整代码:
结论 (Conclusion)
In this article, we covered five different ways you can use to format a string in Python:
在本文中,我们介绍了可用于在Python中格式化字符串的五种不同方式:
- The modulo operator. 模运算符。
- The format function. 格式功能。
- f-strings. F弦。
- Manual formatting. 手动格式化。
- Template strings. 模板字符串。
Each method has its usage advantages and disadvantages. A general rule of thumb, as put by Dan Badar, if the string you’re formatting is user-obtained, template strings are the way to go. If not, use f-strings if you’re on Python 3.7 or the format function elsewhere.
每种方法都有其使用的优缺点。 根据Dan Badar的一般经验法则,如果要格式化的字符串是用户获得的,则使用模板字符串是可行的方法。 否则,如果您使用的是Python 3.7或其他地方的format函数,请使用f字符串。
翻译自: https://towardsdatascience.com/a-guide-to-everything-string-formatting-in-python-e724f101eac5
python中格式化字符串
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如若转载,请注明出处:http://www.mzph.cn/news/389453.shtml
如若内容造成侵权/违法违规/事实不符,请联系多彩编程网进行投诉反馈email:809451989@qq.com,一经查实,立即删除!