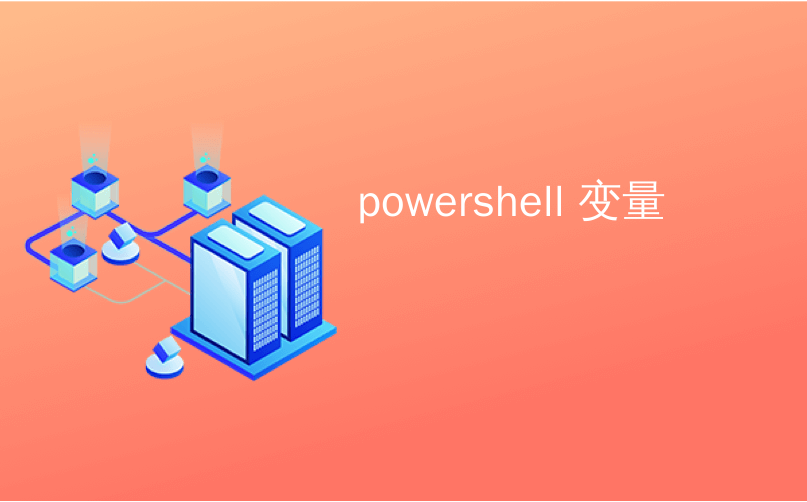
powershell 变量
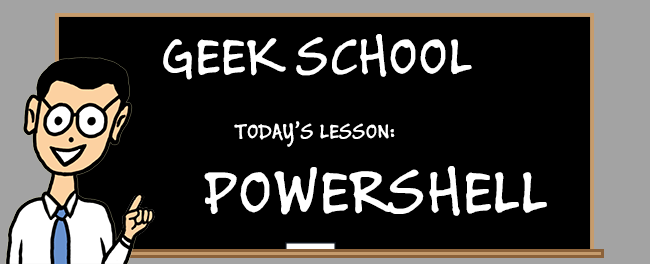
As we move away from simply running commands and move into writing full blown scripts, you will need a temporary place to store data. This is where variables come in.
随着我们不再只是运行命令而转而编写完整的脚本,您将需要一个临时位置来存储数据。 这是变量进入的地方。
Be sure to read the previous articles in the series:
确保阅读本系列中的先前文章:
Learn How to Automate Windows with PowerShell
了解如何使用PowerShell自动执行Windows
Learning to Use Cmdlets in PowerShell
学习在PowerShell中使用Cmdlet
Learning How to Use Objects in PowerShell
学习如何在PowerShell中使用对象
Learning Formatting, Filtering and Comparing in PowerShell
学习在PowerShell中进行格式化,过滤和比较
Learn to Use Remoting in PowerShell
了解在PowerShell中使用远程处理
Using PowerShell to Get Computer Information
使用PowerShell获取计算机信息
Working with Collections in PowerShell
在PowerShell中使用集合
And stay tuned for the rest of the series all week.
并继续关注本系列的其余部分。
变数 (Variables)
Most programming languages allow the use of variables, which are simply containers which hold values. In PowerShell, we too have variables and they are really easy to use. Here is how to create a variable called “FirstName” and give it the value “Taylor”.
大多数编程语言都允许使用变量,这些变量只是保存值的容器。 在PowerShell中,我们也有变量,它们确实非常易于使用。 这是创建变量“ FirstName”并为其赋予值“ Taylor”的方法。
$FirstName = “Taylor”
$ FirstName =“泰勒”
The first thing most people seem to ask is why we put a dollar sign in front of the variables name, and that is actually a very good question. Really, the dollar sign is just a little hint to the shell that we want to access the contents of the variable (think what’s inside the container) and not the container itself. In PowerShell, variable names do not include the dollar sign, meaning that in the above example the variables name is actually “FirstName”.
多数人似乎要问的第一件事是为什么我们在变量名前加一个美元符号,这实际上是一个很好的问题。 确实,美元符号只是向shell提示我们要访问变量的内容(请考虑容器内部的内容),而不是容器本身。 在PowerShell中,变量名称不包含美元符号,这意味着在上面的示例中,变量名称实际上是“ FirstName”。
In PowerShell, you can see all the variables you have created in the variable PSDrive.
在PowerShell中,您可以看到在变量PSDrive中创建的所有变量。
gci variable:
gci变量:
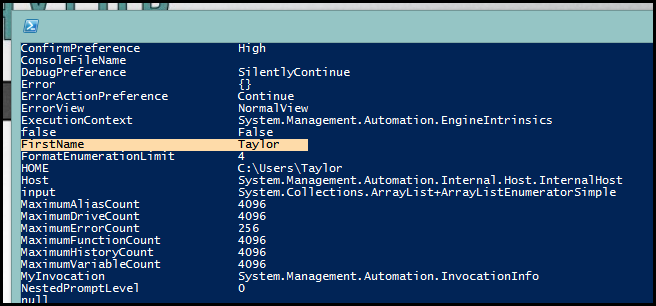
Which means you can delete a variable from the shell at anytime too:
这意味着您也可以随时从外壳中删除变量:
Remove-Item Variable:\FirstName
删除项变量:\ FirstName
Variables don’t have to contain a single object either; you can just as easily store multiple objects in a variable. For example, if you wanted to store a list of running processes in a variable, you can just assign it the output of Get-Process.
变量也不必包含单个对象。 您可以轻松地将多个对象存储在一个变量中。 例如,如果要在变量中存储正在运行的进程的列表,则只需为其分配Get-Process的输出即可。
$Proc = Get-Process
$ Proc =获取进程
The trick to understanding this is to remember that the right hand side of the equals sign is always evaluated first. This means that you can have an entire pipeline on the right hand side if you want to.
理解这一点的技巧是要记住,始终首先评估等号的右侧。 这意味着您可以根据需要在右侧拥有整个管道。
$CPUHogs = Get-Process | Sort CPU -Descending | select -First 3
$ CPUHogs =获取进程| 排序CPU-降序| 选择-前3个
The CPUHogs variable will now contain the three running processes using the most CPU.
现在,CPUHogs变量将包含使用最多CPU的三个正在运行的进程。
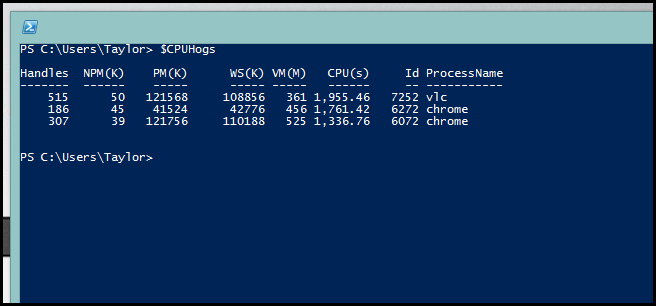
When you do have a variable holding a collection of objects, there are some things to be aware of. For example, calling a method on the variable will cause it to be called on each object in the collection.
当确实有一个保存对象集合的变量时,有一些事情要注意。 例如,在变量上调用方法将导致在集合中的每个对象上调用该方法。
$CPUHogs.Kill()
$ CPUHogs.Kill()
Which would kill all three process in the collection. If you want to access a single object in the variable, you need to treat it like an array.
这将杀死集合中的所有三个过程。 如果要访问变量中的单个对象,则需要将其视为数组。
$CPUHogs[0]
$ CPUHogs [0]
Doing that will give you the first object in the collection.
这样做将为您提供集合中的第一个对象。
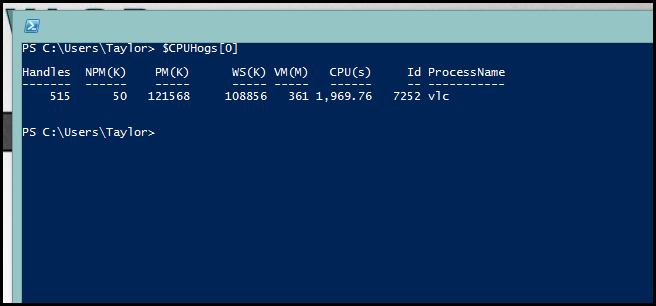
不要被抓住! (Don’t Get Caught!)
Variables in PowerShell are weakly typed by default meaning they can contain any kind of data, this seems to catch new comers to PowerShell all the time!
默认情况下,PowerShell中的变量类型较弱,这意味着它们可以包含任何类型的数据,这似乎一直吸引着PowerShell的新手!
$a = 10
$ a = 10
$b = ‘20’
$ b ='20'
So we have two variables, one contains a string and the other an integer. So what happens if you add them? It actually depends on which order you add them in.
因此,我们有两个变量,一个包含一个字符串,另一个包含一个整数。 那么,如果添加它们会怎样? 实际上,这取决于您添加它们的顺序。
$a + $b = 30
$ a + $ b = 30
While
而
$b + $a = 2010
$ b + $ a = 2010
In the first example, the first operand is an integer, $a, so PowerShell thinks thinks that you are trying to do math and therefore tries to convert any other operands into integers as well. However, in the second example the first operand is a string, so PowerShell just converts the rest of the operands to strings and concatenates them. More advanced scripters prevent this kind of gotcha by casting the variable to the type they are expecting.
在第一个示例中,第一个操作数是整数$ a,因此PowerShell认为您正在尝试进行数学运算,因此也尝试将任何其他操作数也转换为整数。 但是,在第二个示例中,第一个操作数是一个字符串,因此PowerShell只会将其余操作数转换为字符串并将它们连接起来。 更高级的脚本编写者通过将变量强制转换为期望的类型来防止这种情况。
[int]$Number = 5 [int]$Number = ‘5’
[int] $ Number = 5 [int] $ Number ='5'
The above will both result in the Number variable containing an integer object with a value of 5.
上面两者都会导致Number变量包含一个值为5的整数对象。
输入输出 (Input and Output)
Because PowerShell is meant to automate things, you’re going to want to avoid prompting users for information wherever possible. With that said, there are going to be times where you can’t avoid it, and for those times we have the Read-Host cmdlet. Using it is really simple:
由于PowerShell旨在使事情自动化,因此您将避免在任何可能的情况下提示用户输入信息。 话虽如此,在某些时候您将无法避免它,并且在那些时候,我们拥有Read-Host cmdlet。 使用它真的很简单:
$FirstName = Read-Host –Prompt ‘Enter your first name’
$ FirstName =读主机–提示'输入您的名字'
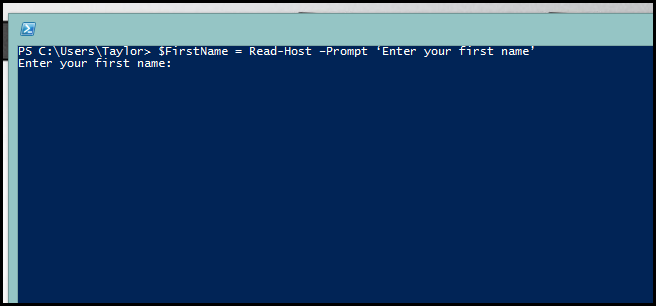
Whatever you enter will then be saved in the variable.
输入的内容将保存在变量中。
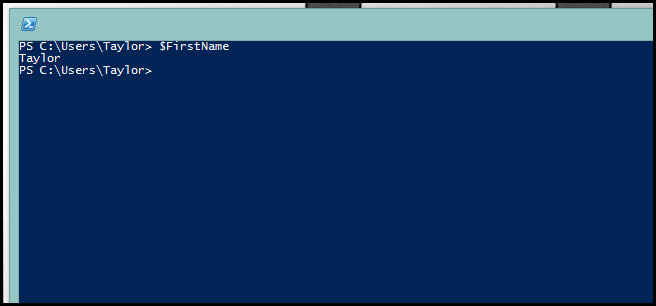
Writing output is just as easy with the Write-Output cmdlet.
使用Write-Output cmdlet可以轻松写入输出。
Write-Output “How-To Geek Rocks!”
写输出“ How-To Geek Rocks!”
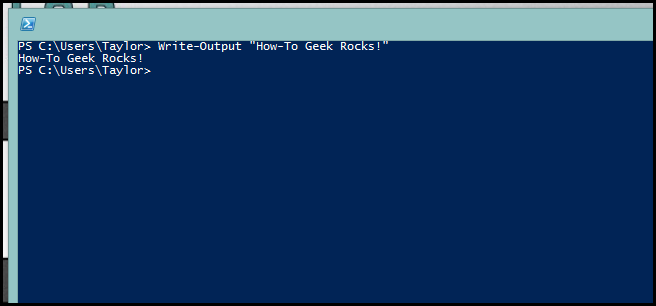
Join us tomorrow where we tie everything we have learned together!
明天加入我们,在这里我们会将我们学到的一切联系在一起!
翻译自: https://www.howtogeek.com/141099/geek-school-learning-powershell-variables-input-and-output/
powershell 变量