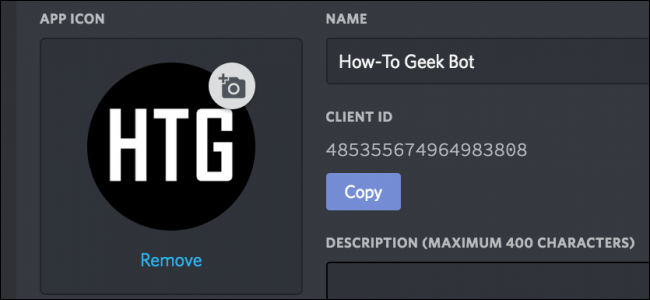
Discord has an excellent API for writing custom bots, and a very active bot community. Today we’ll take a look at how to get started making your own.
Discord具有出色的用于编写自定义机器人的API,以及非常活跃的机器人社区。 今天,我们将探讨如何开始制作自己的作品。
You will need a bit of programming knowledge to code a bot, so it isn’t for everyone, but luckily there are some modules for popular languages that make it very easy to do. We’ll be using the most popular one, discord.js.
您将需要一点编程知识来编写机器人程序,因此并非所有人都可以,但是幸运的是,有一些流行语言模块可以很容易地实现。 我们将使用最受欢迎的discord.js 。
入门 (Getting Started)
Head over to Discord’s bot portal, and create a new application.
转到Discord的机器人门户,并创建一个新应用程序。
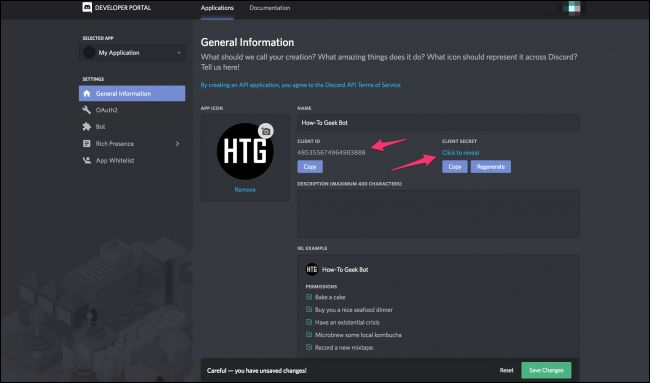
You’ll want to make a note of the Client ID and secret (which you should keep a secret, of course). However, this isn’t the bot, just the “Application.” You’ll have to add the bot under the “Bot” tab.
您需要记下Client ID和机密(当然,您应该保守机密)。 但是,这不是机器人,而是“应用程序”。 您必须在“启动”标签下添加机器人。
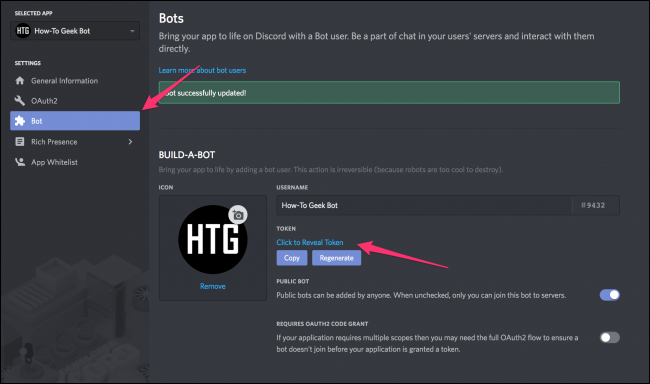
Make a note of this token as well, and keep it a secret. Do not, under any circumstances, commit this key to Github. Your bot will be hacked almost immediately.
还要记下此令牌,并将其保密。 在任何情况下,请勿将此密钥提交给Github。 您的漫游器几乎会立即被黑客入侵。
安装Node.js并获取编码 (Install Node.js and Get Coding)
To run Javascript code outside of a webpage, you need Node. Download it, install it, and make sure it works in a terminal (or Command Prompt, as all of this should work on Windows systems). The default command is “node.”
要在网页外部运行Javascript代码,您需要Node 。 下载它,安装它,并确保它可以在终端上运行(或命令提示符,因为所有这些都应在Windows系统上运行)。 默认命令是“节点”。
We also recommend installing the nodemon tool. It’s a command line app that monitors your bot’s code and restarts automatically on changes. You can install it by running the following command:
我们还建议安装nodemon工具。 这是一个命令行应用程序,可监视您的机器人代码并在更改后自动重新启动。 您可以通过运行以下命令来安装它:
npm i -g nodemon
You’ll need a text editor. You could just use notepad, but we recommend either Atom or VSC.
您需要一个文本编辑器。 您可以只使用记事本,但是我们建议使用Atom或VSC 。
Here’s our “Hello World”:
这是我们的“ Hello World”:
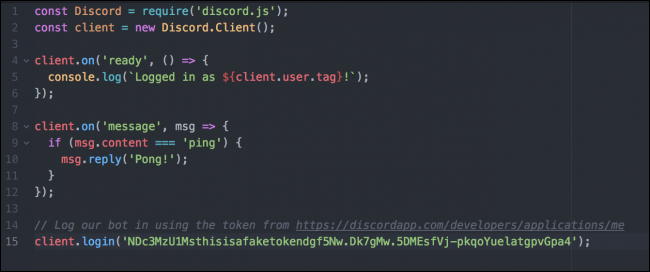
const Discord = require('discord.js');
const client = new Discord.Client();
client.on('ready', () => {
console.log(`Logged in as ${client.user.tag}!`);
});
client.on('message', msg => {
if (msg.content === 'ping') {
msg.reply('pong');
}
});
client.login('token');
This code is taken from the discord.js example. Let’s break it down.
此代码取自discord.js示例。 让我们分解一下。
- The first two lines are to configure the client. Line one imports the module into an object called “Discord,” and line two initializes the client object. 前两行用于配置客户端。 第一行将模块导入名为“ Discord”的对象,第二行初始化客户端对象。
The
client.on('ready')
block will fire when the bot starts up. Here, it’s just configured to log its name to the terminal.机器人启动时,
client.on('ready')
块将触发。 在这里,它只是配置为将其名称记录到终端。The
client.on('message')
block will fire everytime a new message is posted to any channel. Of course, you’ll need to check the message content, and that’s what theif
block does. If the message just says “ping,” then it will reply with “Pong!”每当有新消息发布到任何频道时,
client.on('message')
块都会触发。 当然,您需要检查消息的内容,这就是if
块的作用。 如果该消息仅显示“ ping”,则将回复“ Pong!”。- The last line logs in with the token from the bot portal. Obviously, the token in the screenshot here is fake. Don’t ever post your token on the internet. 最后一行使用Bot门户中的令牌登录。 显然,此屏幕截图中的令牌是伪造的。 永远不要将令牌发布到互联网上。
Copy this code, paste in your token at the bottom, and save it as index.js
in a dedicated folder.
复制此代码,将其粘贴在底部的令牌中,并将其另存为index.js
在专用文件夹中。
如何运行机器人 (How to Run the Bot)
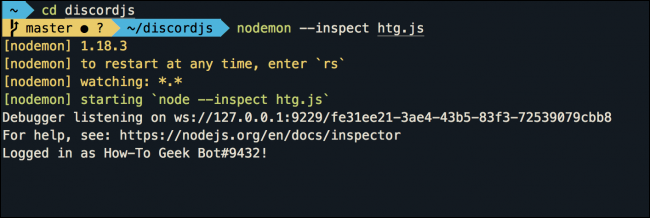
Head over to your terminal, and run the following command:
转到您的终端,然后运行以下命令:
nodemon --inspect index.js
This starts up the script, and also fires up the Chrome debugger, which you can access by typing chrome://inspect/
into Chrome’s Omnibar and then opening “dedicated devtools for Node.”
这将启动脚本,并启动Chrome调试器,您可以通过在Chrome的Omnibar中键入chrome://inspect/
,然后打开“ Node专用devtools”来访问该调试器。
Now, it should just say “Logged in as <bot-name>,” but here I’ve added a line that will log all message objects received to the console:
现在,它应该只说“以<bot-name>登录”,但是在这里我添加了一行,它将记录接收到控制台的所有消息对象:
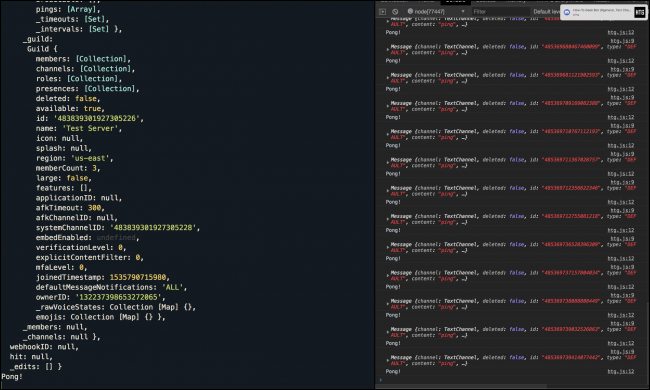
So what makes up this message object? A lot of stuff, actually:
那么,什么构成了这个消息对象呢? 实际上有很多东西:
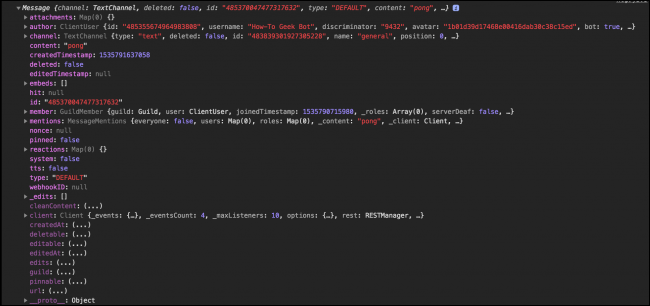
Most notably, you have the author info and the channel info, which you can access with msg.author and msg.channel. I recommend this method of logging objects to the Chrome Node devtools, and just looking around to see what makes it work. You may find something interesting. Here, for example, the bot logs its replies to the console, so the bot’s replies trigger client.on('message')
. So, I made a spambot:
最值得注意的是,您具有作者信息和频道信息,可以使用msg.author和msg.channel访问这些信息。 我建议您使用这种方法将对象记录到Chrome Node devtools,然后四处看看是否能使它起作用。 您可能会发现一些有趣的东西。 例如,在这里,僵尸程序将其答复记录到控制台,因此,僵尸程序的答复会触发client.on('message')
。 所以,我做了一个垃圾邮件机器人:
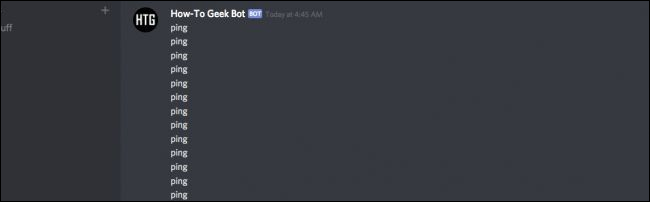
Note: Be careful with this, as you don’t really want to deal with recursion.
注意:请谨慎操作,因为您确实不想处理递归。
如何将Bot添加到服务器 (How to Add the Bot to Your Server)
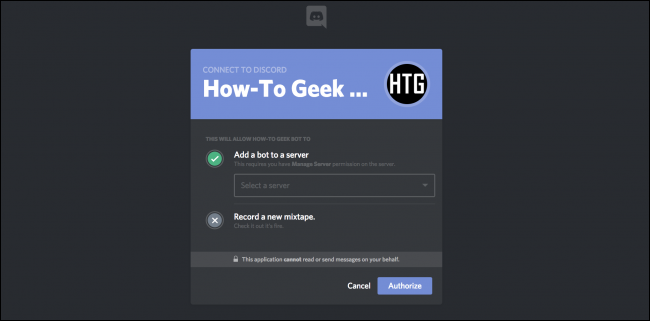
This part is harder than it should be. You have to take this URL:
这部分比应做的要难。 您必须使用以下网址:
https://discordapp.com/oauth2/authorize?client_id=CLIENTID&scope=bot
And replace CLIENTID with your bot’s client ID, found on the general information tab of the application page. Once this is done though, you can give the link to your friends to have them add the bot to their servers as well.
并将CLIENTID替换为bot的客户端ID,该ID可在应用程序页面的常规信息标签上找到。 一旦完成,您就可以将链接提供给您的朋友,让他们也将机器人添加到他们的服务器中。
好吧,那我还能做什么? (Alright, So What Else Can I Do?)
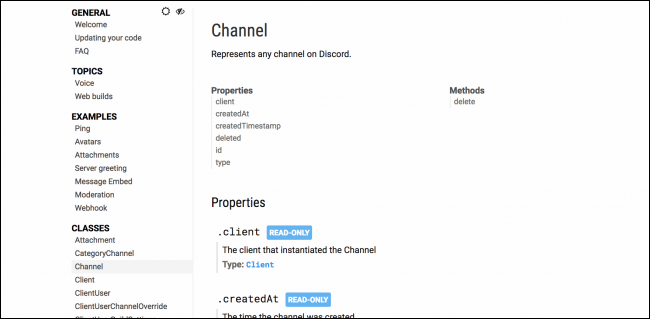
Beyond basic setup, anything else is entirely up to you. But, this wouldn’t be much of a tutorial if we stopped at hello world, so let’s go over some of the documentation, so you have a better idea of what’s possible. I suggest you read through as much as you can, as it’s very well documented.
除了基本设置外,其他任何事情都完全由您决定。 但是,如果我们停滞不前,那么本教程就不会多了,所以让我们看一下一些文档,这样您就更好地了解了可能。 我建议您尽可能多地通读,因为它有充分的记录。
I would recommend adding console.log(client)
to the start of your code, and taking a look at the client object in the console:
我建议将console.log(client)
添加到代码的开头,并在控制台中查看client对象:
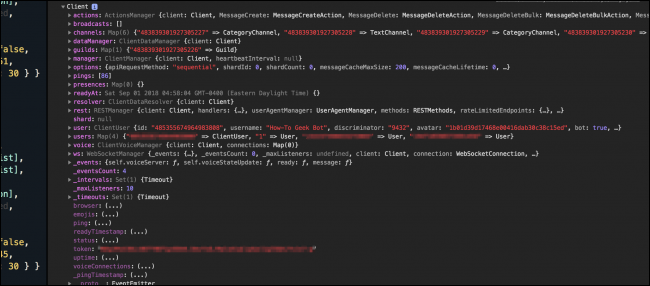
From here, you can learn a lot. Since you can add a bot to multiple servers at once, servers are part of the Guilds
map object. In that object are the individual Guilds (which is the API’s name for “server”) and those guild objects have channel lists that contain all the info and lists of messages. The API is very deep, and may take a while to learn, but at least it’s easy to set up and get started learning.
从这里,您可以学到很多东西。 由于您可以一次将漫游器添加到多个服务器,因此服务器是Guilds
映射对象的一部分。 在该对象中是各个公会(这是“服务器”的API名称),并且那些公会对象具有包含所有信息和消息列表的通道列表。 该API非常深入,可能需要花费一些时间来学习,但至少它很容易设置并开始学习。
翻译自: https://www.howtogeek.com/364225/how-to-make-your-own-discord-bot/