#include <iostream> //运算符重载
using namespace std;class Person
{
// //全局函数实现+运算符重载
// friend const Person operator+(const Person &L,const Person &R);
// //全局函数实现-运算符重载
// friend const Person operator-(const Person &L,const Person &R);
// //全局函数实现*运算符重载
// friend const Person operator*(const Person &L,const Person &R);
// //全局函数实现/运算符重载
// friend const Person operator/(const Person &L,const Person &R);
// //全局函数实现%运算符重载
// friend const Person operator%(const Person &L,const Person &R);// //全局函数实现>运算符重载
// friend bool operator>(const Person &L, const Person &R);
// //全局函数实现>=运算符重载
// friend bool operator>=(const Person &L, const Person &R);
// //全局函数实现<运算符重载
// friend bool operator<(const Person &L, const Person &R);
// //全局函数实现<=运算符重载
// friend bool operator<=(const Person &L, const Person &R);
// //全局函数实现==运算符重载
// friend bool operator==(const Person &L, const Person &R);
// //全局函数实现!=运算符重载
// friend bool operator!=(const Person &L, const Person &R);// //全局函数实现=运算符重载
// friend Person &operator=(Person &L, const Person &R);
// //全局函数实现+=运算符重载
// friend Person &operator+=(Person &L, const Person &R);
// //全局函数实现-=运算符重载
// friend Person &operator-=(Person &L, const Person &R);
// //全局函数实现*=运算符重载
// friend Person &operator*=(Person &L, const Person &R);
// //全局函数实现/=运算符重载
// friend Person &operator/=(Person &L, const Person &R);
// //全局函数实现%=运算符重载
// friend Person &operator%=(Person &L, const Person &R);private:int a;int b;
public:Person(){}Person(int a, int b):a(a),b(b){}
/****************成员函数实现+运算符重载*******************/const Person operator+(const Person &p)const{Person temp;temp.a = a + p.a;temp.b = b + p.b;return temp;}/****************成员函数实现-运算符重载*******************/const Person operator-(const Person &p)const{Person temp;temp.a = a - p.a;temp.b = b - p.b;return temp;}/****************成员函数实现*运算符重载*******************/const Person operator*(const Person &p)const{Person temp;temp.a = a * p.a;temp.b = b * p.b;return temp;}/****************成员函数实现/运算符重载*******************/const Person operator/(const Person &p)const{Person temp;if(0==p.a && 0==p.b)cout << "除数为0" << endl;else if(0==p.a && 0!=p.b){cout << "a除数为0" << " b = " << b << endl;temp.a = a / p.b;}else if(0!=p.a && 0==p.b){temp.b = b / p.a;cout << "a = " << a << " b除数为0" << endl;}else{temp.b = b / p.a;temp.a = a / p.b;cout << "a = " << a << " b = " << b << endl;}return temp;}/****************成员函数实现%运算符重载*******************/const Person operator%(const Person &p)const{Person temp;temp.a = a % p.a;temp.b = b % p.b;return temp;}
/***************成员函数实现>关系运算符重载***************/bool operator>(const Person &R)const{if(a>R.a && b>R.b){return true;}else{return false;}}/***************成员函数实现>=关系运算符重载***************/bool operator>=(const Person &R)const{if(a>=R.a && b>=R.b){return true;}else{return false;}}/***************成员函数实现<关系运算符重载***************/bool operator<(const Person &R)const{if(a<R.a && b<R.b){return true;}else{return false;}}/***************成员函数实现<=关系运算符重载***************/bool operator<=(const Person &R)const{if(a<=R.a && b<=R.b){return true;}else{return false;}}/***************成员函数实现==关系运算符重载***************/bool operator==(const Person &R)const{if(a==R.a && b==R.b){return true;}else{return false;}}/***************成员函数实现!=关系运算符重载***************/bool operator!=(const Person &R)const{if(a!=R.a && b!=R.b){return true;}else{return false;}}
/***************成员函数实现=赋值运算符重载***************/Person &operator=(const Person &R){a = R.a;b = R.b;return *this;}/***************成员函数实现+=赋值运算符重载***************/Person &operator+=(const Person &R){a += R.a;b += R.b;return *this;}/***************成员函数实现-=赋值运算符重载***************/Person &operator-=(const Person &R){a -= R.a;b -= R.b;return *this;}/***************成员函数实现*=赋值运算符重载***************/Person &operator*=(const Person &R){a *= R.a;b *= R.b;return *this;}/***************成员函数实现/=赋值运算符重载***************/Person &operator/=(const Person &R){if(0==R.a && 0==R.b)cout << "除数为0" << endl;else if(0==R.a && 0!=R.b){cout << "a除数为0" << " b = " << b << endl;a = a / R.b;}else if(0!=R.a && 0==R.b){b = b / R.a;cout << "a = " << a << " b除数为0" << endl;}else if(0!=R.a && 0!=R.b){a = a / R.a;b = b / R.b;cout << "a = " << a << " b = " << b << endl;}return *this;}/***************成员函数实现%=赋值运算符重载***************/Person &operator%=(const Person &R){a %= R.a;b %= R.b;return *this;}void show(){cout << "a = " << a << " b = " << b << endl;}};///****************全局函数实现+运算符重载*******************/
//const Person operator+(const Person &L,const Person &R)
//{
// Person temp;
// temp.a = L.a + R.a;
// temp.b = L.b + R.b;
// return temp;
//}
///****************全局函数实现-运算符重载*******************/
//const Person operator-(const Person &L,const Person &R)
//{
// Person temp;
// temp.a = L.a - R.a;
// temp.b = L.b - R.b;
// return temp;
//}
///****************全局函数实现*运算符重载*******************/
//const Person operator*(const Person &L,const Person &R)
//{
// Person temp;
// temp.a = L.a * R.a;
// temp.b = L.b * R.b;
// return temp;
//}
///****************全局函数实现/运算符重载*******************/
//const Person operator/(const Person &L,const Person &R)
//{
// Person temp;
// if(0==R.a && 0==R.b)
// cout << "除数为0" << endl;
// else if(0==R.a && 0!=R.b)
// {
// cout << "a除数为0" << " b = " << L.b << endl;
// temp.a = L.a / R.b;
// }
// else if(0!=R.a && 0==R.b)
// {
// temp.b = L.b / R.a;
// cout << "a = " << L.a << " b除数为0" << endl;
// }
// else if(0!=R.a && 0!=R.b)
// {
// temp.b = L.b / R.a;
// temp.a = L.a / R.b;
// cout << "a = " << L.a << " b = " << L.b << endl;
// }
// return temp;
//}
///****************全局函数实现%运算符重载*******************/
//const Person operator%(const Person &L,const Person &R)
//{
// Person temp;
// temp.a = L.a % R.a;
// temp.b = L.b % R.b;
// return temp;
//}///***************全局函数实现>关系运算符重载***************/
//bool operator>(const Person &L, const Person &R)
//{
// if(L.a>R.a && L.b>R.b)
// {
// return true;
// }
// else
// {
// return false;
// }
//}
///***************全局函数实现>=关系运算符重载***************/
//bool operator>=(const Person &L, const Person &R)
//{
// if(L.a>=R.a && L.b>=R.b)
// {
// return true;
// }
// else
// {
// return false;
// }
//}
///***************全局函数实现<关系运算符重载***************/
//bool operator<(const Person &L, const Person &R)
//{
// if(L.a<R.a && L.b<R.b)
// {
// return true;
// }
// else
// {
// return false;
// }
//}
///***************全局函数实现>关系运算符重载***************/
//bool operator<=(const Person &L, const Person &R)
//{
// if(L.a<=R.a && L.b<=R.b)
// {
// return true;
// }
// else
// {
// return false;
// }
//}
///***************全局函数实现==关系运算符重载***************/
//bool operator==(const Person &L, const Person &R)
//{
// if(L.a==R.a && L.b==R.b)
// {
// return true;
// }
// else
// {
// return false;
// }
//}
///***************全局函数实现!=关系运算符重载***************/
//bool operator!=(const Person &L, const Person &R)
//{
// if(L.a!=R.a && L.b!=R.b)
// {
// return true;
// }
// else
// {
// return false;
// }
//}///***************全局函数实现=赋值运算符重载***************/
//Person &operator=(Person &L, const Person &R)
//{
// L.a = R.a;
// L.b = R.b;
// return L;
//}
///***************全局函数实现+=赋值运算符重载***************/
//Person &operator+=(Person &L, const Person &R)
//{
// L.a += R.a;
// L.b += R.b;
// return L;
//}
///***************全局函数实现-=赋值运算符重载***************/
//Person &operator-=(Person &L, const Person &R)
//{
// L.a -= R.a;
// L.b -= R.b;
// return L;
//}
///***************全局函数实现*=赋值运算符重载***************/
//Person &operator*=(Person &L, const Person &R)
//{
// L.a *= R.a;
// L.b *= R.b;
// return L;
//}
///***************全局函数实现/=赋值运算符重载***************/
//Person &operator/=(Person &L, const Person &R)
//{
// if(0==R.a && 0==R.b)
// cout << "除数为0" << endl;
// else if(0==R.a && 0!=R.b)
// {
// cout << "a除数为0" << " b = " << L.b << endl;
// L.b /= R.b;
// }
// else if(0!=R.a && 0==R.b)
// {
// L.a /= R.a;
// cout << "a = " << L.a << " b除数为0" << endl;
// }
// else if(0!=R.a && 0!=R.b)
// {
// L.a /= R.a;
// L.b /= R.b;
// cout << "a = " << L.a << " b = " << L.b << endl;
// }
// return L;
//}
///***************全局函数实现%=赋值运算符重载***************/
//Person &operator%=(Person &L, const Person &R)
//{
// L.a %= R.a;
// L.b %= R.b;
// return L;
//}/****************************************************/
int main()
{Person p1(3,4);Person p2(1,2);Person p3 = p1 + p2; p3.show();Person p4 = p1 - p2; p4.show();Person p5 = p1 * p2; p5.show();Person p6 = p1 / p2;Person p7 = p1 % p2; p7.show();if(p3>p2)cout << "p3>p2" << endl;else if(p3>=p2)cout << "p3>=p2" << endl;else if(p3<p2)cout << "p3<p2" << endl;else if(p3<=p2)cout << "p3<=p2" << endl;else if(p3==p2)cout << "p3==p2" << endl;else if(p3!=p2)cout << "p3!=p2" << endl;p3+=p2;p3.show();p3-=p2;p3.show();p3*=p2;p3.show();p3/=p2;p3%=p2;p3.show();return 0;
}
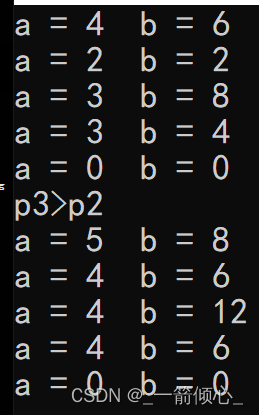
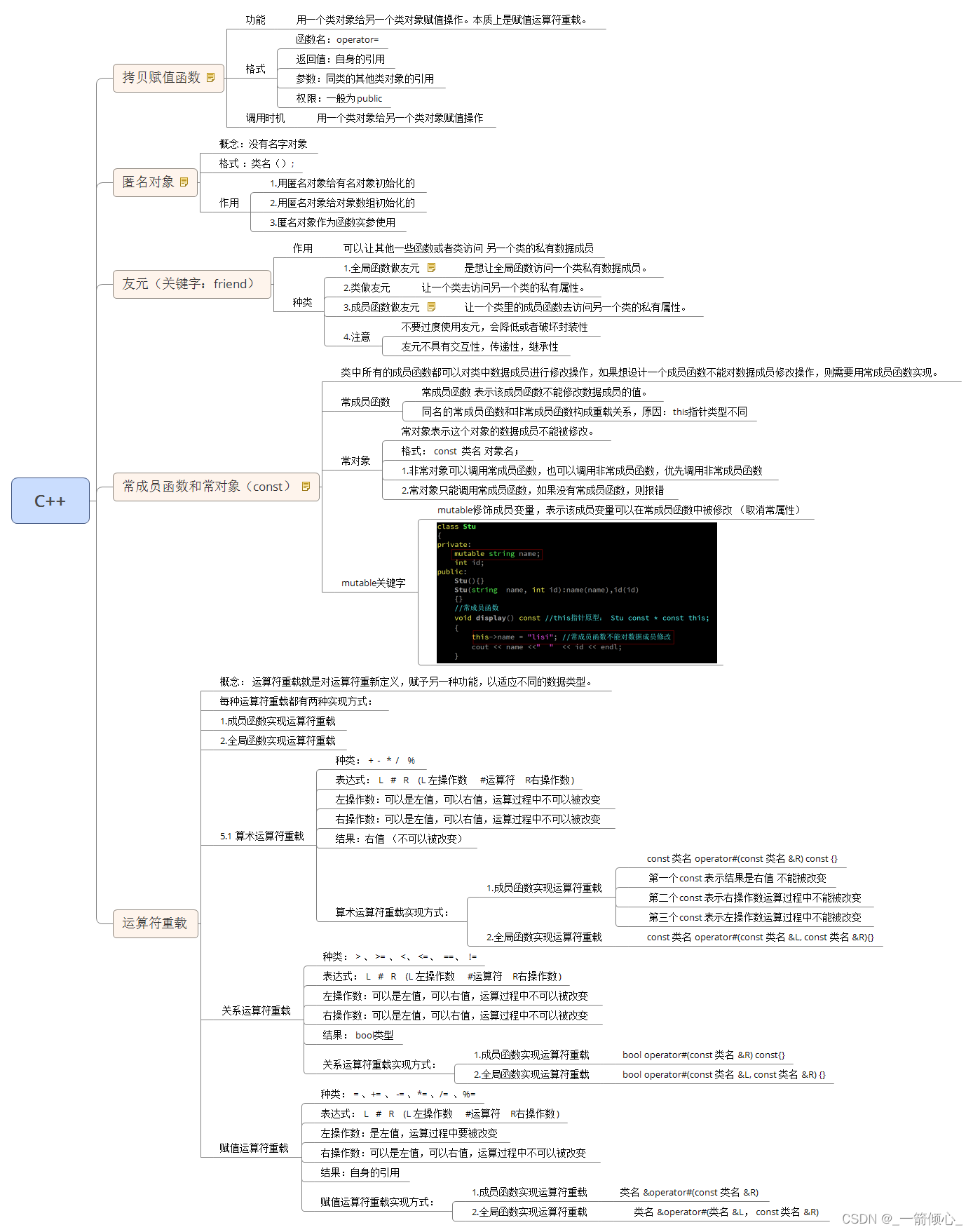