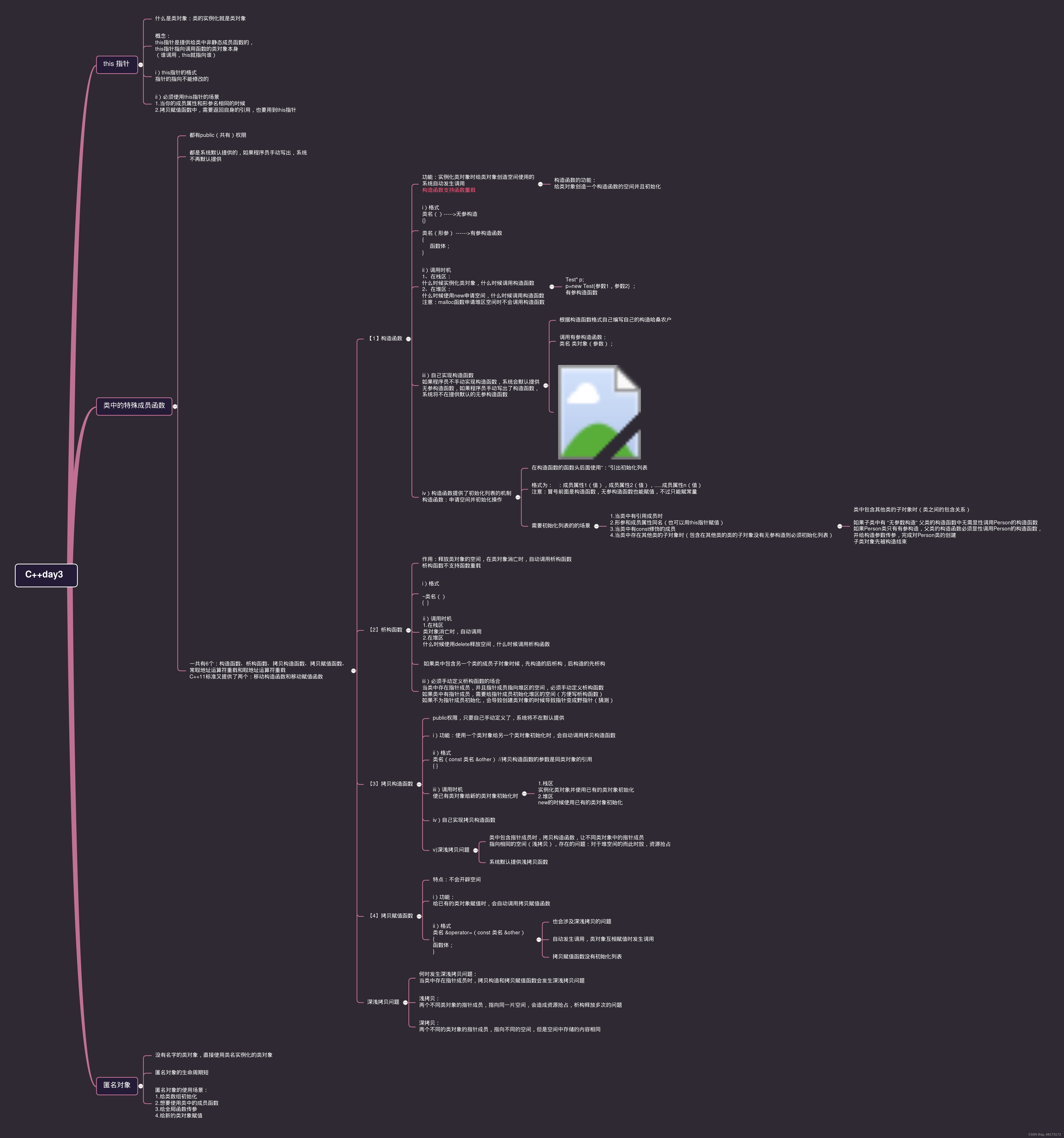
#include <iostream>
#include <iomanip>
using namespace std;
class Person{const string name;int age;char sex;
public:Person(const string name):name(name){cout << "第一个Person构造函数" << endl;}Person():name("zhangsan"){cout << "第二个Person构造函数" << endl;}~Person(){cout << "Person的析构函数" << endl;}void show(){cout << "name:" << name << endl << "age:" << age << endl << "sex:" << sex << endl;}void set(int age,char sex){this->age=age;this->sex=sex;}Person(const Person &other):name(other.name){this->age = other.age;this->sex = other.sex;cout << "Person的拷贝构造函数" << endl;}Person &operator=(const Person &other){//this->name=other.name;this->age=other.age;this->sex=other.sex;cout << "Person的拷贝赋值函数" << endl;return* this;}
};
class Stu{Person p1;double* score;
public:Stu():score(new double){cout << "这是第一个Stu构造函数" << endl;}Stu(const string name):p1(name),score(new double){cout << "这是第二个Stu构造函数" << endl;}~Stu(){delete score;cout << "Stu的析构函数" << endl;}void show(){p1.show();cout << "score:" << score << endl;}void set(int age,char sex,double score){p1.set(age,sex);*(this->score)=score;}Stu(const Stu &other):p1(other.p1),score(new double(*(other.score))){ //初始化的顺序很重要,否则会报一个警告cout << "Stu的拷贝构造函数" << endl;}Stu &operator=(const Stu &other){*(this->score)=*(other.score);cout << "Stu的拷贝赋值函数" <<endl;return* this;}
};int main()
{Stu s1("王华");s1.set(18,'m',70);s1.show();Stu s2=s1;s2.show();return 0;
}
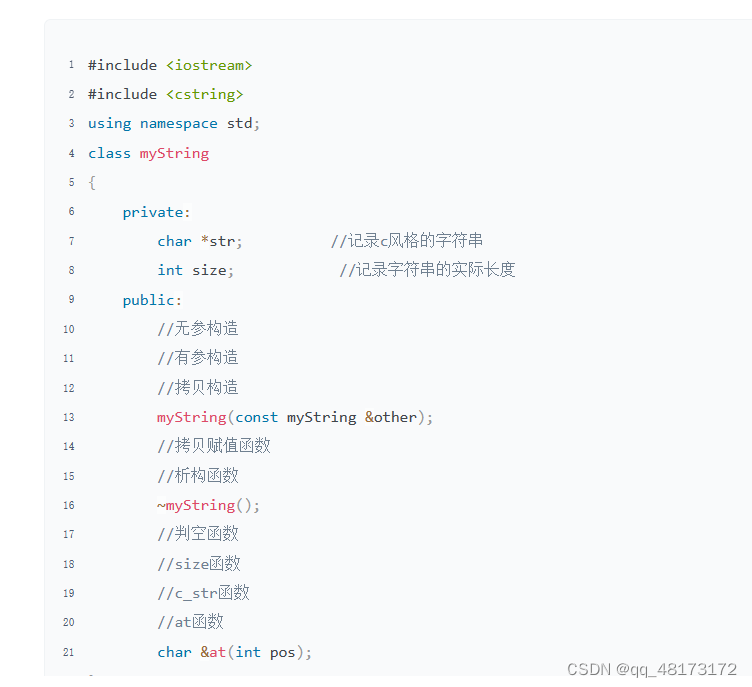
#include <iostream>
#include <iomanip>
#include <cstring>
using namespace std;
class myString{
private:char* str;int size;
public:myString():str(new char[120]){cout << "无参构造函数" << endl;}myString(char* str):str(new char[120]){this->str=str;this->size=strlen(str);cout << "有参构造函数" << endl;}myString(const myString &other):str(new char[120]){this->str=other.str;cout << "拷贝构造函数" << endl;}myString &operator=(const myString &other){this->str=other.str;this->size=other.size;cout << "拷贝赋值函数" << endl;return* this;}~myString(){delete[] str;cout << "析构函数" << endl;}int empty(){cout << "判空函数" << endl;if(strlen(this->str)==0){return 1;}else return 0;}int mysize(){return strlen(this->str);}const char* c_str(){return this->str;}char& at(int pos){if(size>=pos){return str[pos];}else{cout << "error ,out " << endl;}}
};int main()
{char s[20]="hello world";myString s1(s);myString s2;s2=s1;cout << s1.at(10) << endl;cout << s2.c_str() << endl;return 0;
}