//wiget.h#ifndef WIDGET_H
#define WIDGET_H#include <QWidget>
#include <QTime> //时间类
#include <QTimer> //定时器类
#include <QTextToSpeech>
#include <QDebug>
QT_BEGIN_NAMESPACE
namespace Ui { class Widget; }
QT_END_NAMESPACEclass Widget : public QWidget
{Q_OBJECTpublic:Widget(QWidget *parent = nullptr);~Widget();void time_slot1(); //自定义处理timeout信号的槽函数void time_slot2();private slots:void on_startbtn_clicked();void on_stopbtn_clicked();private:Ui::Widget *ui;//定义一个定时器指针,基于类对象版本的定时器QTimer *timer;QTimer *timer_timeEdit;//定义一个播报员QTextToSpeech *speecher;int flag = 0;
};
#endif // WIDGET_H//main.cpp
#include "widget.h"#include <QApplication>int main(int argc, char *argv[])
{QApplication a(argc, argv);Widget w;w.show();return a.exec();
}//widget.cpp#include "widget.h"
#include "ui_widget.h"Widget::Widget(QWidget *parent): QWidget(parent), ui(new Ui::Widget)
{ui->setupUi(this);//实例化定时器timer = new QTimer(this);//启动定时器timer->start(100); //从此时起,该定时器会每隔1秒钟的时间发射一个timeout的信号//将该定时器的timeout信号连接到自定义的槽函数中connect(timer,&QTimer::timeout,this,&Widget::time_slot1);speecher = new QTextToSpeech(this);//设置定时行编辑器的格式ui->timeEdit->setAlignment(Qt::AlignCenter);//标签文本对齐方式 居中ui->timeEdit->setFont(QFont("微软雅黑",15));}Widget::~Widget()
{delete ui;
}
//自定义处理timeout信号的槽函数
void Widget::time_slot1()
{//调用QTime的静态成员函数获取当前系统时间QTime sys_time = QTime::currentTime();//获取时、分、秒
// int hour = sys_time.hour();
// int minute = sys_time.minute();
// int sec = sys_time.second();//将时间类对象调用函数转化为字符串QString t = sys_time.toString("hh:mm:ss");//时间字符串t展示到ui界面的lab中ui->time_label->setText(t);ui->time_label->setAlignment(Qt::AlignCenter);//标签文本对齐方式 居中ui->time_label->setFont(QFont("微软雅黑",20));if(flag == 0){ui->timeEdit->setText(ui->time_label->text());flag = 1;}
}
//处理时间行编辑器的定时器的timeout信号
void Widget::time_slot2()
{if(ui->timeEdit->text() == ui->time_label->text()){speecher->say(ui->textEdit->toPlainText());}
}void Widget::on_startbtn_clicked()
{//启动后时间文本编辑器、文本编辑器和启动按钮将不可用ui->startbtn->setEnabled(false);ui->timeEdit->setEnabled(false);ui->textEdit->setEnabled(false);timer_timeEdit = new QTimer(this);timer->start(100); //从此时起,该定时器会每隔1秒钟的时间发射一个timeout的信号connect(timer,&QTimer::timeout,this,&Widget::time_slot2);}void Widget::on_stopbtn_clicked()
{//停止后时间文本编辑器、文本编辑器和启动按钮可用ui->startbtn->setEnabled(true);ui->timeEdit->setEnabled(true);ui->textEdit->setEnabled(true);}
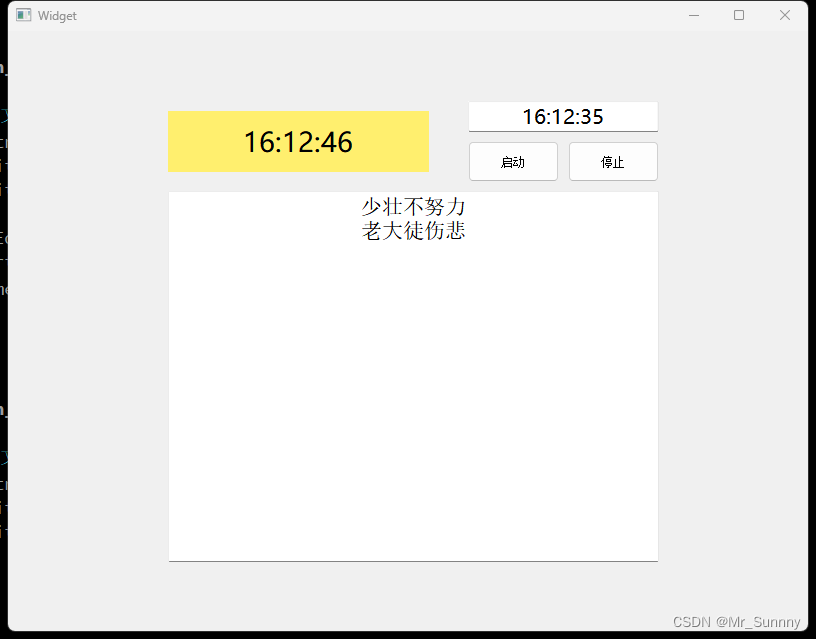