CS 0449 – Project 1: Mastermind and EXIF Viewer
Mastermind Implementation (30 points)
In this game, the computer chooses 4 pegs each with one of 6 colors. This is the color-based predecessor to Wordle. The player’s job is then to guess the colors that the computer has chosen in the proper order. After each guess by the player, the computer will then give two numbers as feedback. The first number is how many pegs are the proper color and in the proper position. The second number is how many pegs are the proper color, but not in the
correct position.
What you need to do:
Generate a random computer guess of four colors out of: o Red, Orange, Yellow, Green, Blue, Purple
Read a guess from the user as a string of colors (see example below)
Score the guess, and report the two values back to the user
Allow the user to continue to guess until they get it correct, or reach 10 turns and they lose.
Allow the user to play the game multiple times
Example:
Welcome to Mastermind!
Would you like to play? yes
Enter guess number 1: rrrr
Colors in the correct place: 1
Colors correct but in wrong position: 0
Enter guess number 2: rrgg
Hints
Generating random numbers in C is a two-step part. First, we need to seed the random number generator once per program. The idiom to do this is:
srand((unsigned int)time(NULL));
When we need random numbers, we can use the rand() function. It returns an unsigned integer between 0 and RAND_MAX. We can use modulus to reduce it to the range we need:
int value = rand() % (high - low + 1) + low;
EXIF Viewer (70 points)
An EXIF tag is embedded in many image files taken on a digital camera to add metadata about the camera and exposure. It is a complicated format, but we can simplify it to where we can write a simple viewer that will work with many JPEG files.
A JPEG file begins with the 2 byte sequence 0xFF, 0xD8. After that, a special JPEG marker (0xFF 0xE1) indicates an application specific segment, called APP1. We will assume (but you must verify) that there is no APP0 (0xFF 0xE0) section prior to APP1. This means the first 20 bytes of a JPEG
with an EXIF tag will be:
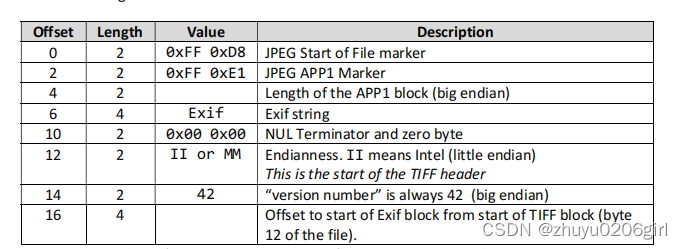
Next, an array of TIFF (Tagged Image File Format) Tags will store the information we are looking for. At offset 20, we find a 2-byte (unsigned short) count that tells us how many tags there will
be in this section.