文章目录
- 一、Promise的三种状态
- 1. 初始态pending
- 2. 成功态fulfilled,调用resolve方法
- 3. 失败态rejected,调用reject方法
- 二、Promise的方法
-
- 三、async和await
-
- 四、代码举例帮助理解
- 1、Promise的值通过then方法获取
- 2、reject的值通过catch方法获取
- 3、Promise得到状态之后,状态不可改变
- 4、then和catch方法执行之后会返回一个相同状态值为fulfilled(成功)的Promise对象
- 5、then方法的返回值会进入到Promise对象中的PromiseResult
- 6、then方法的连续调用
- 7、Promise对象可以反复使用,调用then方法并不会改变对象本身
- 8、让then和catch方法返回一个resolved(错误)状态的Promise对象
- 封装ajax请求
- 参考文档
一、Promise的三种状态
1. 初始态pending
- pending。它的意思是 “待定的,将发生的”,相当于是一个初始状态。创建Promise对象时,且没有调用resolve或者是reject方法,相当于是初始状态。这个初始状态会随着你调用resolve,或者是reject函数而切换到另一种状态。
2. 成功态fulfilled,调用resolve方法
- resolved。表示解决了,就是说这个承诺实现了。 要实现从pending到resolved的转变,需要在 创建Promise对象时,在函数体中调用了resolve方法(即第一个参数)。
3. 失败态rejected,调用reject方法
- rejected。拒绝,失败。表示这个承诺没有做到,失败了。要实现从pending到rejected的转换,只需要在创建Promise对象时,调用reject函数。
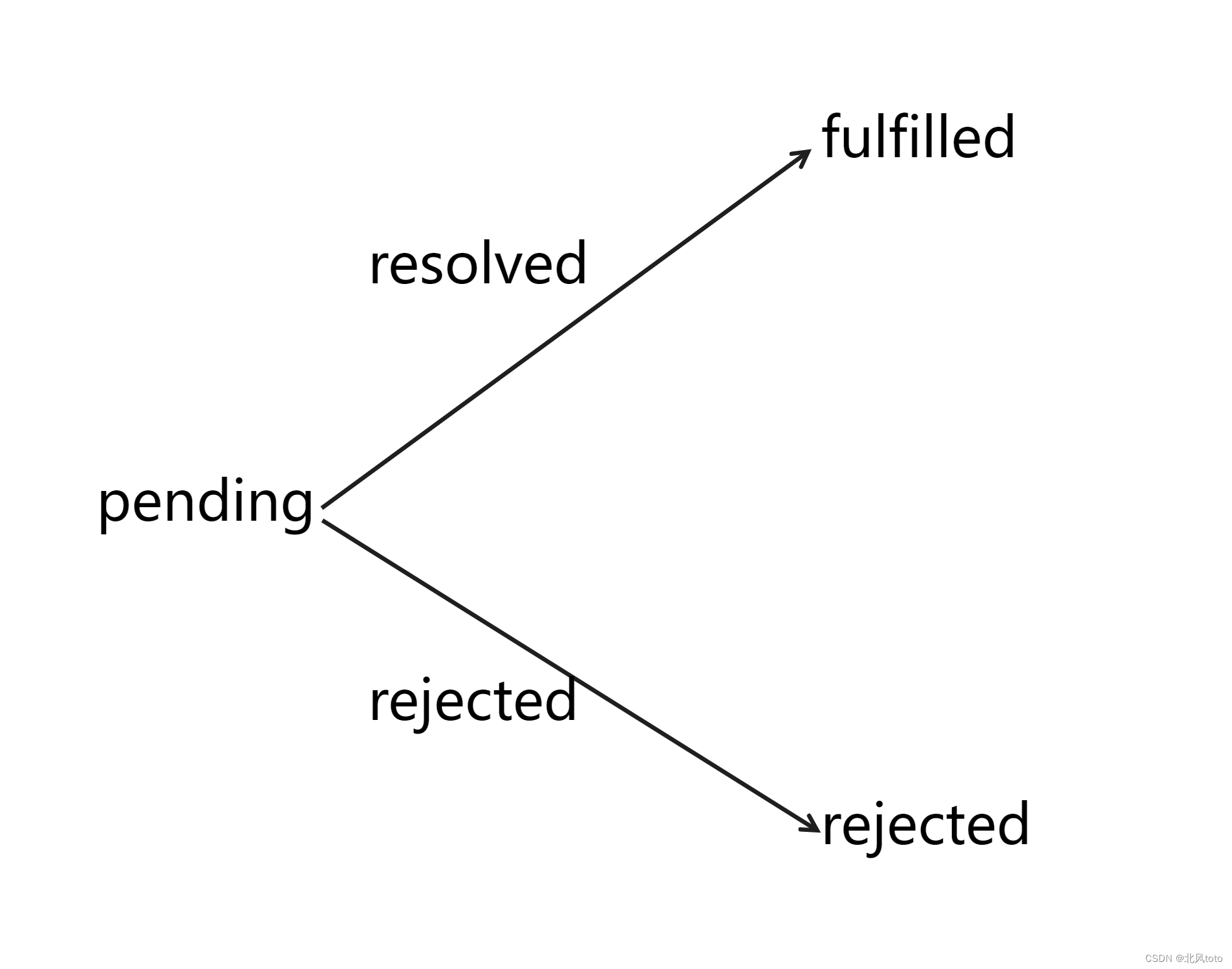
二、Promise的方法
then方法
- 在then方法的参数函数中,通过形参使用Promise对象的结果
- then方法返回一个新的Promise实例,状态是pending
- 在then方法中,通过return将返回的Promise实例改为fulfilled状态
- 在then方法中,出现代码错误,将返回的Promise实例改为rejected状态
catch方法
- 当Promise的状态改为rejcted.被执行
- 当Promise执行过程出现代码错误时,被执行
三、async和await
async 函数
- 函数的返回值为 promise 对象
- promise 对象的结果由 async 函数执行的返回值决定
await 表达式
- await 右侧的表达式一般为 promise 对象, 但也可以是其它的值
- 如果表达式是 promise 对象, await 返回的是 promise 成功的值
- 如果表达式是其它值, 直接将此值作为 await 的返回值
四、代码举例帮助理解
1、Promise的值通过then方法获取
const firstPromise001 = new Promise((resolve,reject)=>{resolve("这是我的成功状态")
})
firstPromise001.then((res)=>{console.log('resolve中的值为='+res)
})
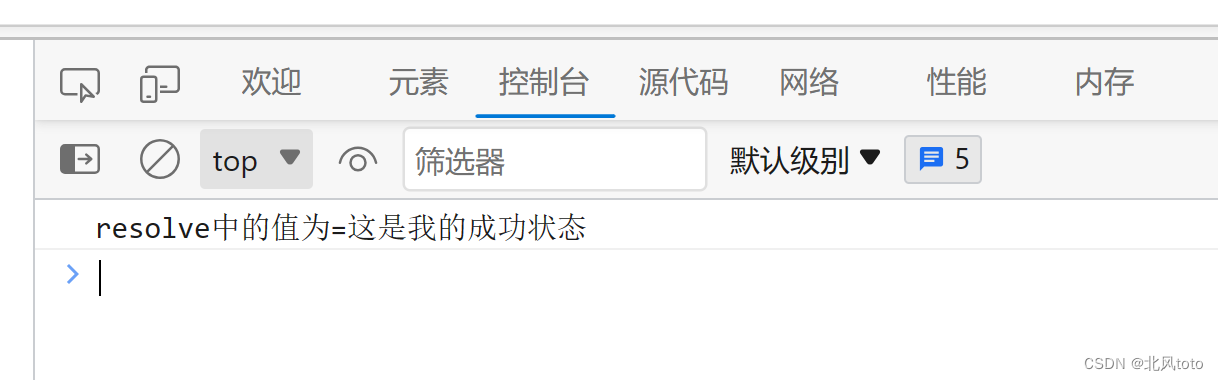
const firstPromise002 = new Promise((resolve,reject)=>{reject("这是我的失败状态")
})
firstPromise002.then((res)=>{console.log('resolve(正确)中的值为='+res)
},(err)=>{console.log('reject(错误)中的值为='+err)
})
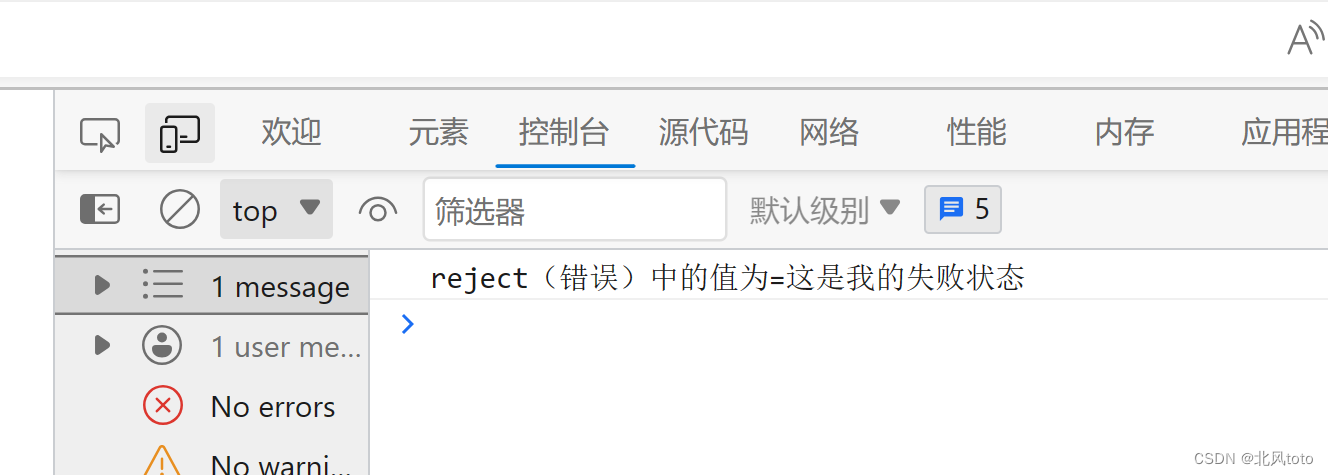
2、reject的值通过catch方法获取
const firstPromise001 = new Promise((resolve,reject)=>{reject("这是我的失败状态")
})
firstPromise001.catch((err)=>{console.log('reject中的值为='+err)
})
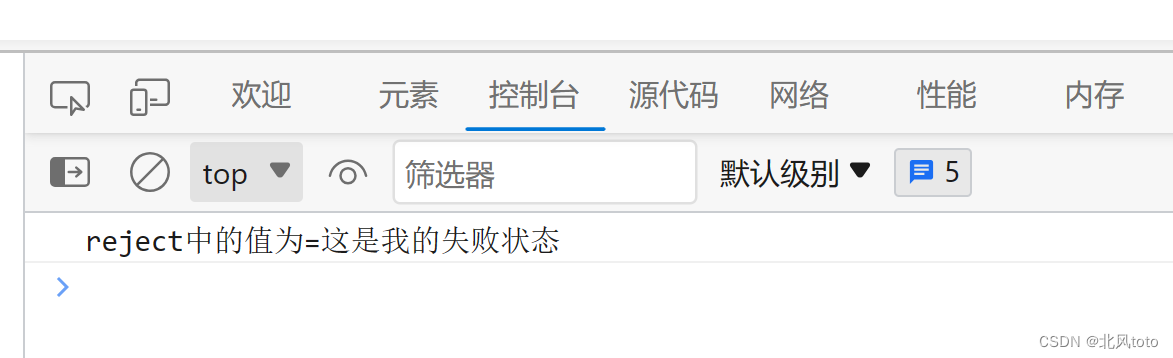
3、Promise得到状态之后,状态不可改变
a=0
const firstPromise = new Promise((resolve,reject)=>{a++resolve("这是我的成功状态")a++reject("这是我的失败状态")a++
})
console.log(firstPromise)
console.log(a)
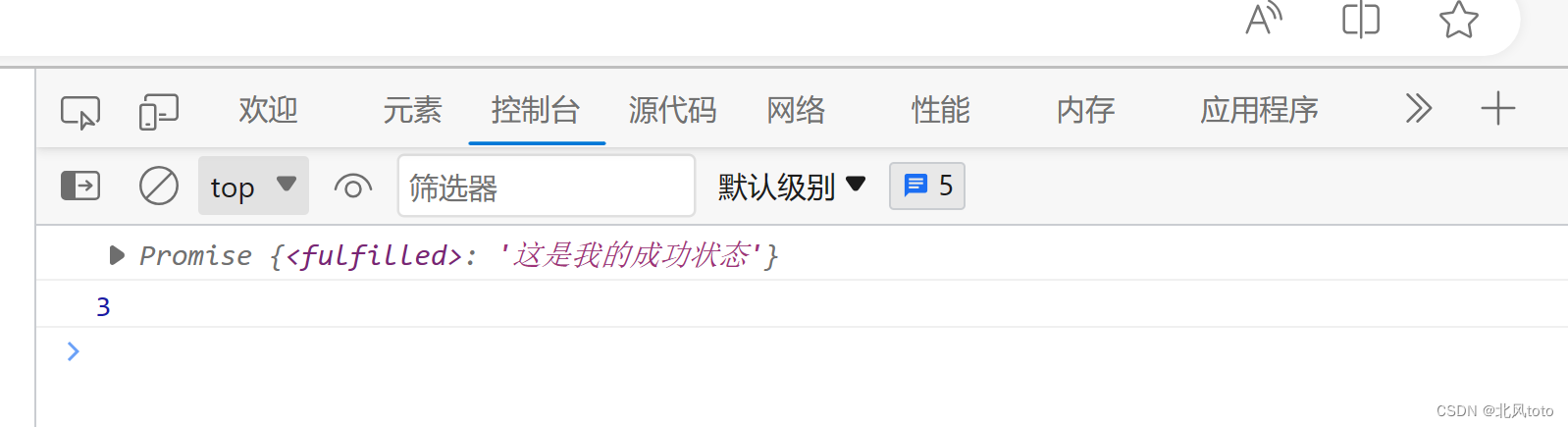
4、then和catch方法执行之后会返回一个相同状态值为fulfilled(成功)的Promise对象
- 无论是什么状态的Promise对象都能调用then方法,但是只有rejected(失败)状态的才能调用catch方法
- 作者为什么要探究这个呢?因为 then方法返回的对象是一个状态为fulfilled(成功)的Promise,所以then方法调用结束之后可以继续调用then方法。但是catch不行,catch方法结束之后只能调用then方法,不能调用catch方法,因为调用catch方法的条件是状态为rejected(失败)。
const thenPromise = new Promise((resolve,reject)=>{resolve("这是我的成功状态")
}).then((res)=>{console.log('resolve中的值为='+res)
})
const catchPromise = new Promise((resolve,reject)=>{reject("这是我的失败状态")
}).catch((err)=>{console.log('reject中的值为='+err)
})
console.log(thenPromise)
console.log(catchPromise)
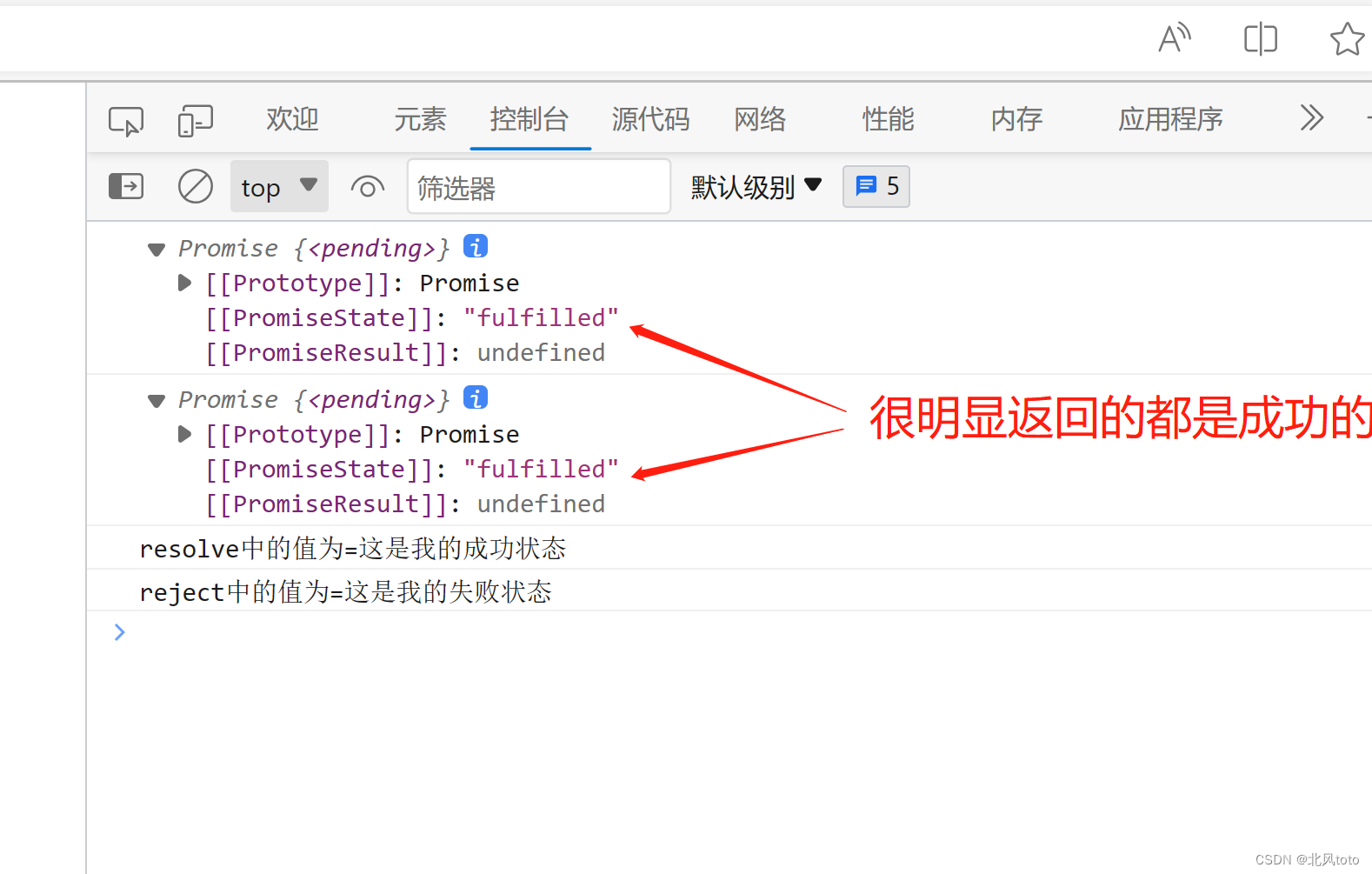
5、then方法的返回值会进入到Promise对象中的PromiseResult
const firstPromise = new Promise((resolve,reject)=>{resolve("这是我的成功状态")
}).then((res)=>{console.log('第一次then输出的状态='+res)return "第一次调用then的返回";
})
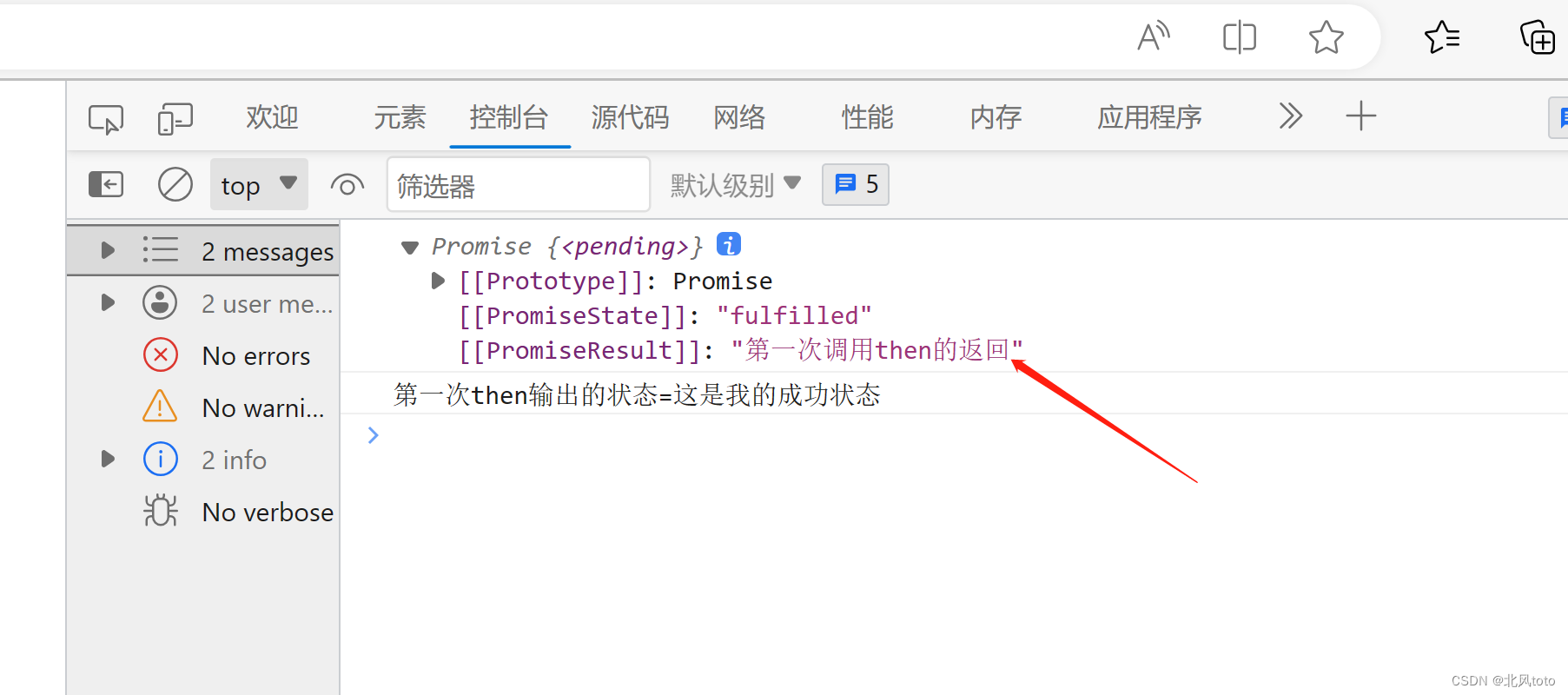
6、then方法的连续调用
const firstPromise = new Promise((resolve,reject)=>{resolve("这是我的成功状态")
}).then((res)=>{console.log('第一次then输出的状态='+res)return "第一次调用then的返回";
}).then((res)=>{console.log('第二次then输出的状态='+res)return "北京"
}).then((res)=>{console.log('第三次then输出的状态='+res)
})
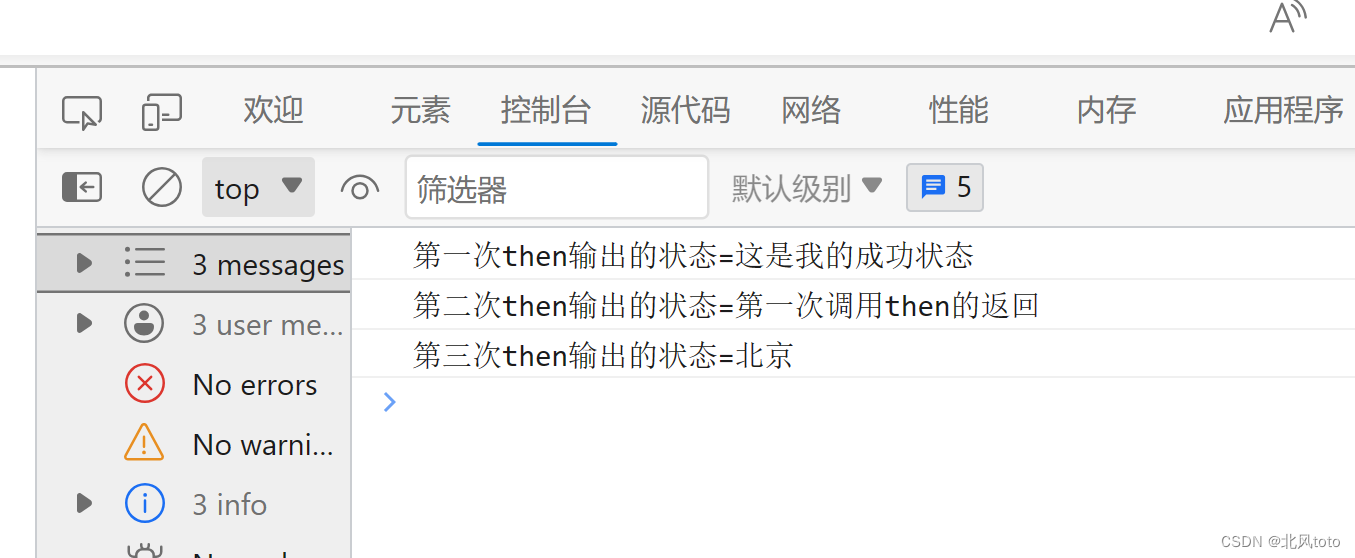
7、Promise对象可以反复使用,调用then方法并不会改变对象本身
const firstPromise = new Promise((resolve,reject)=>{resolve("这是我的成功状态")
})console.log(firstPromise)firstPromise.then((res)=>{console.log('resolve中的值为='+res)
})
firstPromise.then((res)=>{console.log('resolve中的值为='+res)
})
firstPromise.then((res)=>{console.log('resolve中的值为='+res)
})console.log(firstPromise)
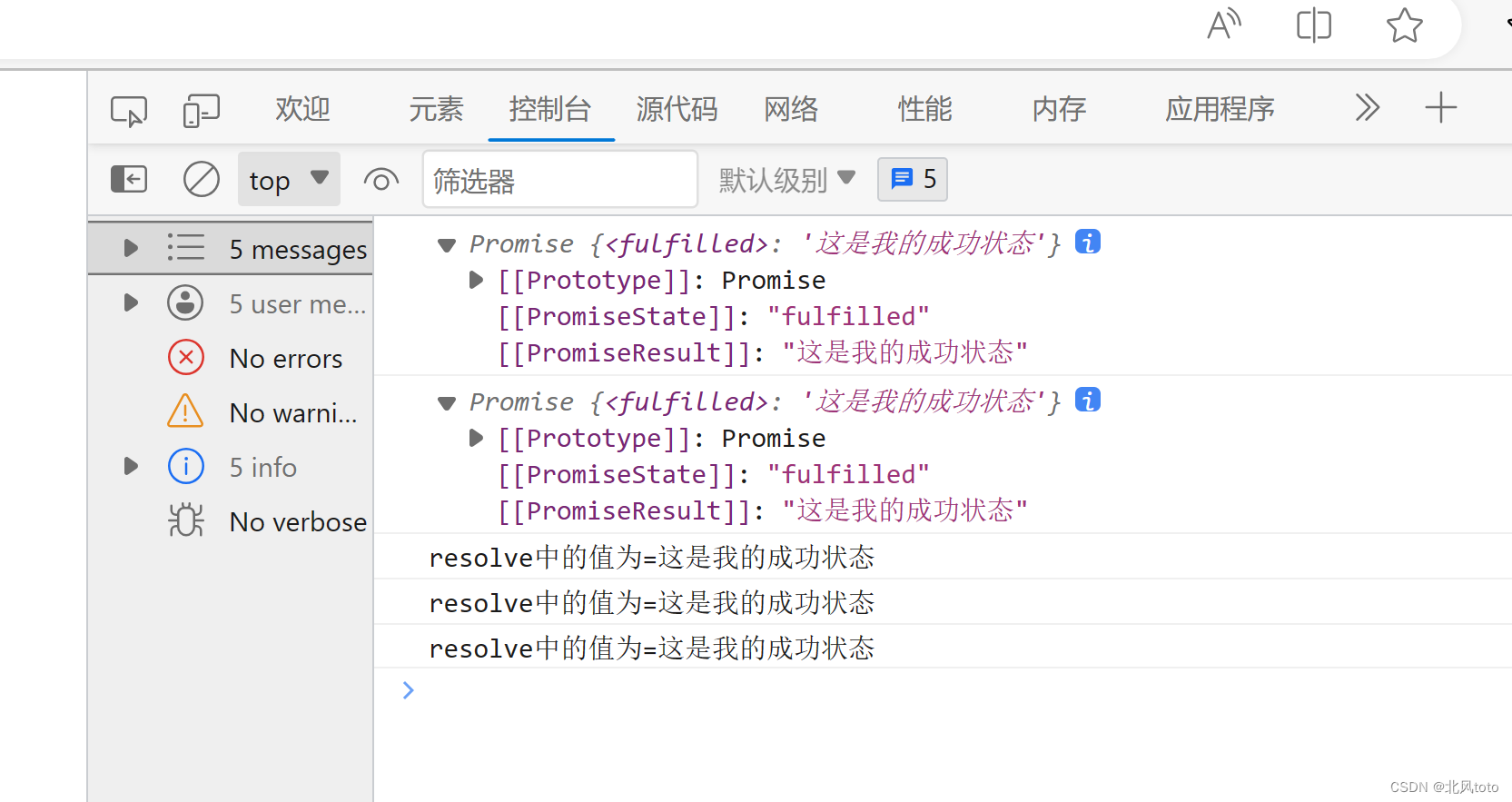
8、让then和catch方法返回一个resolved(错误)状态的Promise对象
- 错误信息会封装到Promise对象中的PromiseResult
const firstPromise = new Promise((resolve,reject)=>{resolve("这是我的成功状态")
}).then((res)=>{throw new Error('作者用于测试的程序错误')console.log('第一次then输出的状态='+res)return "第一次调用then的返回";
})console.log(firstPromise)firstPromise.catch((err)=>{console.log('把错误信息打印到控制台='+err)
})
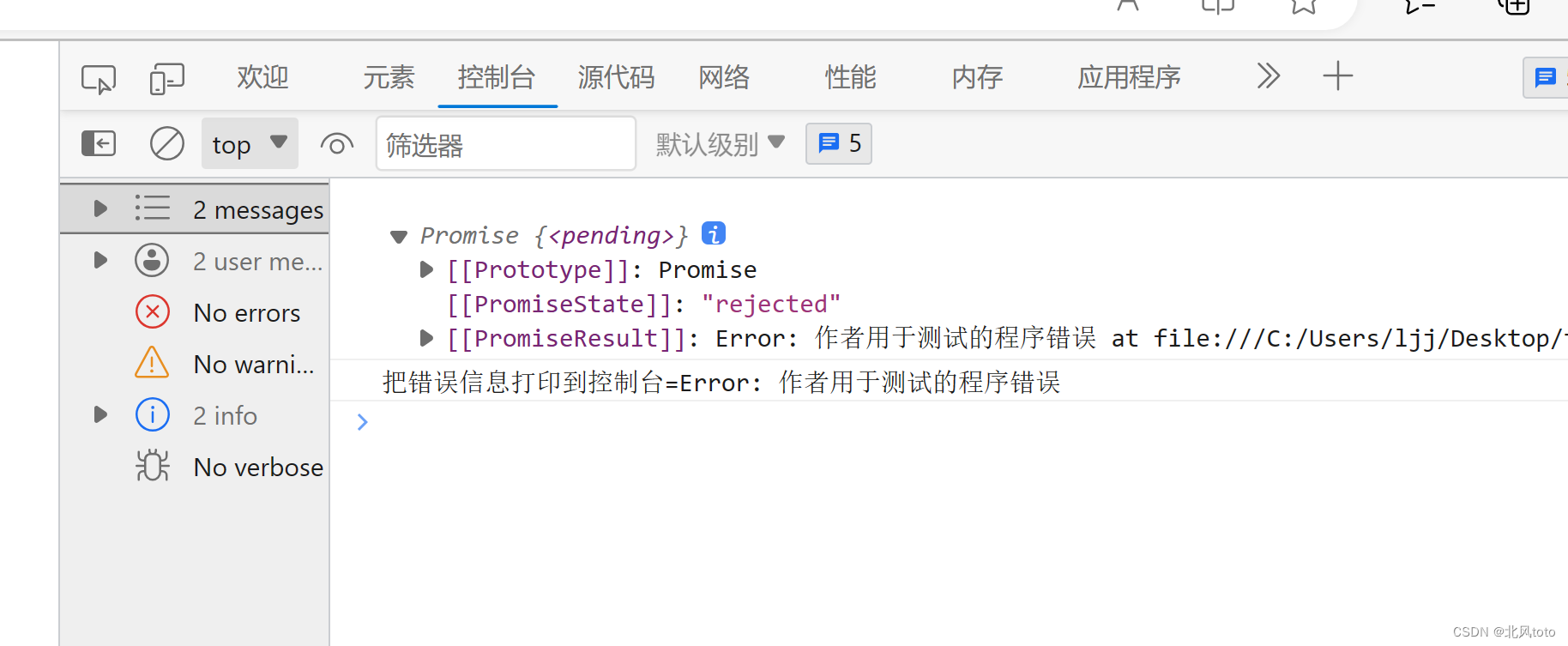
封装ajax请求
function getData(url, data = {}){return new Promise((resolve, reject) => {$.ajax({type: "GET",url: url,data: data,success: function (res) {resolve(res)},error:function (res) {reject(res)}})}
}
getData("data1.json").then((data) => {const { id } = datareturn getData("data2.json", {id})}).then((data) => {const { usename } = datareturn getData("data3.json", {usename})}).then((data) => {console.log(data)})
参考文档