实验3:工厂方法模式
本次实验属于模仿型实验,通过本次实验学生将掌握以下内容:
1、理解工厂方法模式的动机,掌握该模式的结构;
2、能够利用工厂方法模式解决实际问题。
[实验任务]:加密算法
目前常用的加密算法有DES(Data Encryption Standard)和IDEA(International Data Encryption Algorithm)国际数据加密算法等,请用工厂方法实现加密算法系统。
类图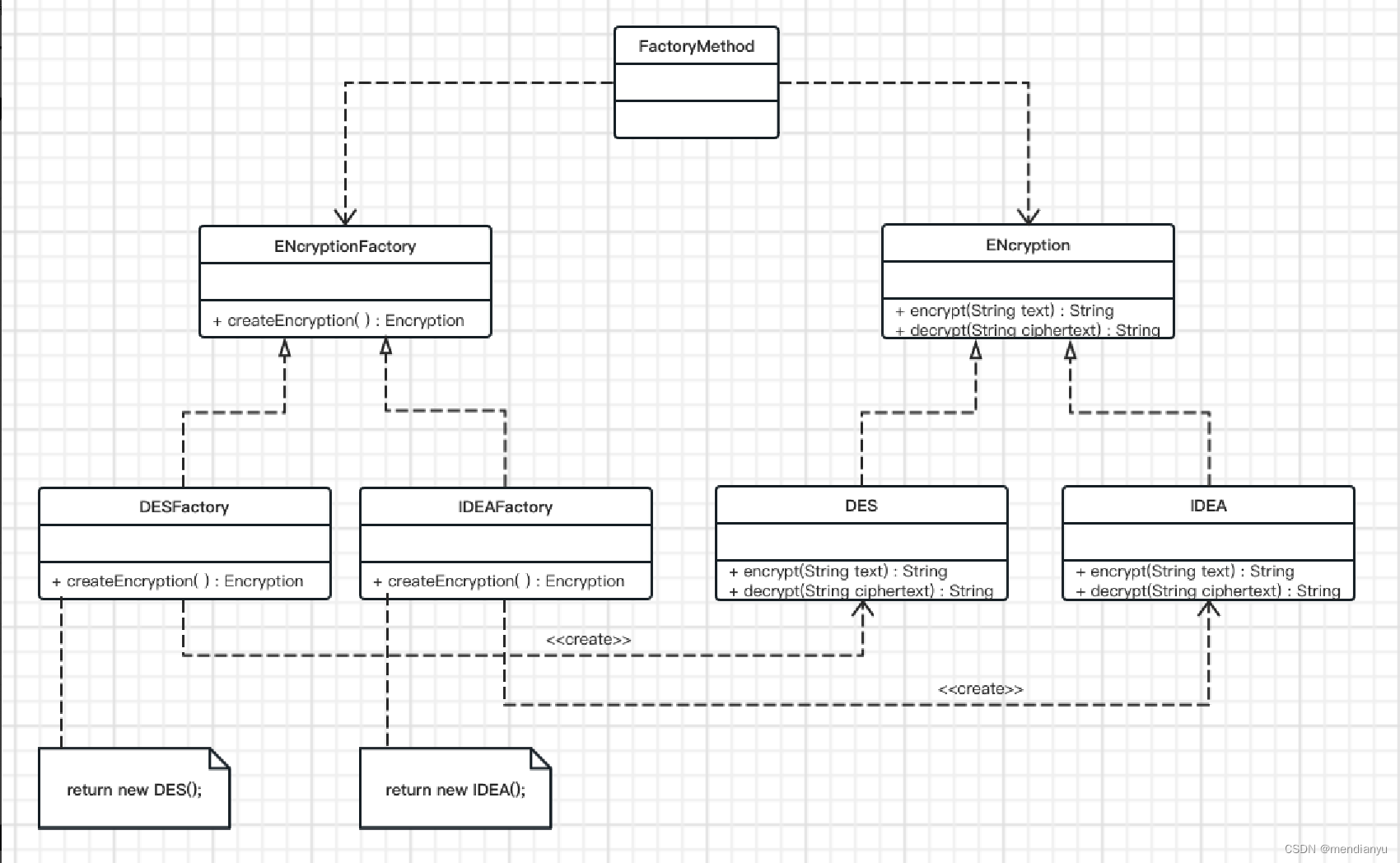
运行效果
源代码
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.nio.charset.StandardCharsets;
import java.util.Base64;public class DES implements Encryption
{private SecretKey secretKey;public DES(){try{KeyGenerator keyGenerator = KeyGenerator.getInstance("DES");secretKey = keyGenerator.generateKey();}catch (Exception e){e.printStackTrace();}}//DES加密@Overridepublic String encrypt(String text){try{Cipher cipher = Cipher.getInstance("DES");cipher.init(Cipher.ENCRYPT_MODE, secretKey);byte[] encryptedBytes = cipher.doFinal(text.getBytes(StandardCharsets.UTF_8));return Base64.getEncoder().encodeToString(encryptedBytes);}catch (Exception e){e.printStackTrace();return null;}}//DES解密@Overridepublic String decrypt(String ciphertext){try{Cipher cipher = Cipher.getInstance("DES");cipher.init(Cipher.DECRYPT_MODE, secretKey);byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(ciphertext));return new String(decryptedBytes, StandardCharsets.UTF_8);}catch (Exception e){e.printStackTrace();return null;}}
}public class DESFactory implements EncryptionFactory
{@Overridepublic Encryption createEncryption(){return new DES();}
}public interface Encryption
{String encrypt(String text);String decrypt(String ciphertext);
}public interface EncryptionFactory
{Encryption createEncryption();
}import java.util.Scanner;public class FactoryMethod
{public static void main(String[] args){Scanner sc = new Scanner(System.in);System.out.println("请输入要加密的文本:");String text = sc.next();EncryptionFactory factory = null;System.out.println("请输入加密算法编号:\n" +"1、DES加密 2、IDEA加密");int choose = sc.nextInt();if (choose == 1){//DES加密factory = new DESFactory();System.out.println("DES加密");} else if (choose == 2){//IDEA加密factory = new IDEAFactory();System.out.println("IDEA加密");}Encryption encryptionAlgorithm = factory.createEncryption();String encryptedText = encryptionAlgorithm.encrypt(text);String decryptedText = encryptionAlgorithm.decrypt(encryptedText);System.out.println("原文本:" + text);System.out.println("加密后:" + encryptedText);System.out.println("解密后:" + decryptedText);}
}import org.bouncycastle.jce.provider.BouncyCastleProvider;import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.nio.charset.StandardCharsets;
import java.security.Security;
import java.util.Base64;public class IDEA implements Encryption
{private SecretKey secretKey;static{// 添加Bouncy Castle提供程序Security.addProvider(new BouncyCastleProvider());}public IDEA(){try{KeyGenerator keyGenerator = KeyGenerator.getInstance("IDEA", "BC");secretKey = keyGenerator.generateKey();}catch (Exception e){e.printStackTrace();}}@Overridepublic String encrypt(String text){try{// 创建一个IDEA加密器Cipher cipher = Cipher.getInstance("IDEA/ECB/PKCS5Padding", "BC");cipher.init(Cipher.ENCRYPT_MODE, secretKey);byte[] encryptedBytes = cipher.doFinal(text.getBytes());return Base64.getEncoder().encodeToString(encryptedBytes);}catch (Exception e){e.printStackTrace();return null;}}@Overridepublic String decrypt(String ciphertext){try{Cipher cipher = Cipher.getInstance("IDEA/ECB/PKCS5Padding", "BC");cipher.init(Cipher.DECRYPT_MODE, secretKey);byte[] encryptedBytes = Base64.getDecoder().decode(ciphertext);return new String(cipher.doFinal(encryptedBytes), StandardCharsets.UTF_8);}catch (Exception e){e.printStackTrace();return null;}}
}public class IDEAFactory implements EncryptionFactory
{@Overridepublic Encryption createEncryption(){return new IDEA();}
}