目的:
- Mybatis整合Ehcache实现二级缓存
- Mybatis整合Redis实现二级缓存
Mybatis整合ehcache实现二级缓存
- ssm中整合ehcache
在POM中导入相关依赖

org.springframework spring-context-support ${spring.version}org.mybatis.caches mybatis-ehcache 1.1.0net.sf.ehcache ehcache 2.10.0

- 修改日志配置,因为ehcache使用了Slf4j作为日志输出
日志我们使用slf4j,并用log4j来实现。SLF4J不同于其他日志类库,与其它有很大的不同。
SLF4J(Simple logging Facade for Java)不是一个真正的日志实现,而是一个抽象层( abstraction layer),
它允许你在后台使用任意一个日志类库。
Pom.xml

2.9.13.2.01.7.13org.slf4j slf4j-api ${slf4j.version}org.slf4j jcl-over-slf4j ${slf4j.version}runtimeorg.apache.logging.log4j log4j-api ${log4j2.version}org.apache.logging.log4j log4j-core ${log4j2.version}org.apache.logging.log4j log4j-slf4j-impl ${log4j2.version}org.apache.logging.log4j log4j-web ${log4j2.version}runtimecom.lmax disruptor ${log4j2.disruptor.version}

在Resource中添加一个ehcache.xml的配置文件
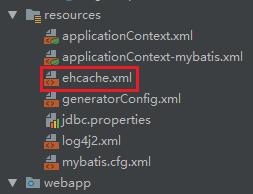
ehcache.xml

<?xml version="1.0" encoding="UTF-8"?>

在applicationContext-mybatis.xml中给mybatis设置支持
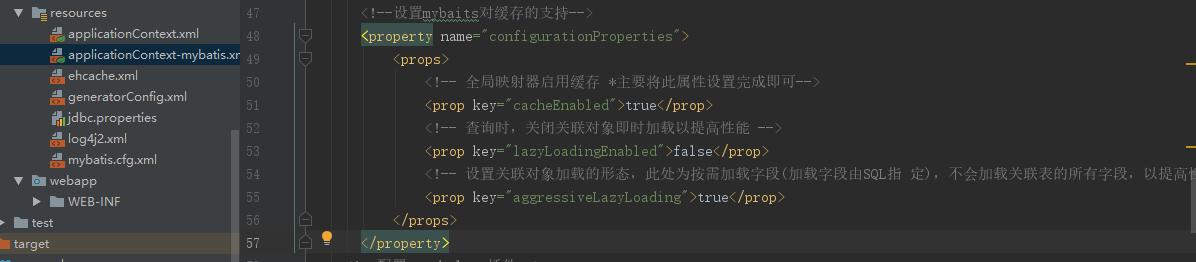

truefalsetrue

现在我们来测试一下它访问是否使用了二级缓存

@Test public void cacheSingle() { Book book= this.bookService.selectByPrimaryKey(5); System.out.println(book); Book book2= this.bookService.selectByPrimaryKey(5); System.out.println(book2); }

效果:
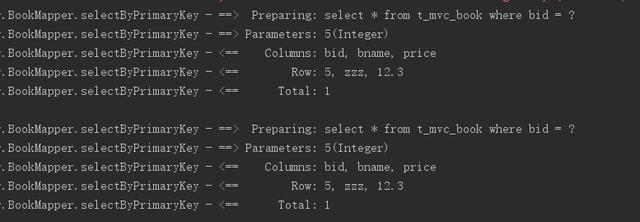
很明显查询了两次数据库,没有成功,继续往下看如何解决这个问题
我们需要在BookMapper.xml中加入chache配置

我们在运行之前的测试方法测试一下
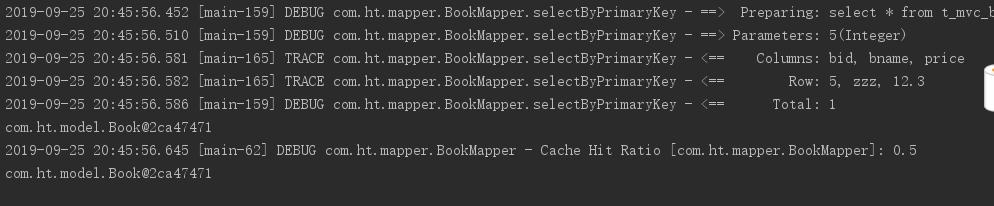
很明显,第一次从数据库中查询数据第二次就从缓存中拿数据了
现在我们来测试查询多条数据的效果

@Test public void cacheMany() { Map map = new HashMap(); map.put("bname", StringUtils.toLikeStr("圣墟")); List hhhh = this.bookService.listPager(map,pageBean); for (Map m : hhhh) { System.out.println(m); } List hhhh2 = this.bookService.listPager(map,pageBean); for (Map m : hhhh2) { System.out.println(m); } }

效果:
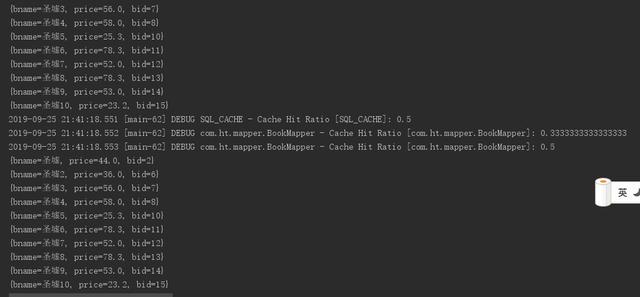
- 关闭缓存
既然开启了那就会有关闭缓存的时候,对吧
我们可以通过select标签的useCache属性打开或关闭二级缓存即可
注意:
1、mybatis默认使用的二级缓存框架就是ehcache(org.mybatis.caches.ehcache.EhcacheCache),无缝结合
2、Mybatis缓存开关一旦开启,可缓存单条记录,也可缓存多条,hibernate不能缓存多条。
3、Mapper接口上的所有方法上另外提供关闭缓存的属性
Mybatis整合redis实现二级缓存
1. redis常用类
1.1 Jedis
jedis就是集成了redis的一些命令操作,封装了redis的java客户端
1.2 JedisPoolConfig
Redis连接池
1.3 ShardedJedis
基于一致性哈希算法实现的分布式Redis集群客户端
实现 mybatis 的二级缓存,一般来说有如下两种方式:
1) 采用 mybatis 内置的 cache 机制。
2) 采用三方 cache 框架, 比如ehcache, oscache 等等.
2. 添加jar依赖
添加redis相关依赖

2.9.01.7.1.RELEASEredis.clients jedis ${redis.version}org.springframework.data spring-data-redis ${redis.spring.version}

log4j2配置
jackson依赖

2.9.3com.fasterxml.jackson.core jackson-databind ${jackson.version}com.fasterxml.jackson.core jackson-core ${jackson.version}com.fasterxml.jackson.core jackson-annotations ${jackson.version}

- spring + redis 集成实现缓存功能(与mybatis无关)
添加两个redis的配置文件,并将redis.properties和applicationContext-redis.xml配置到applicationContext.xml文件中
redis.properties

redis.hostName=192.168.80.128redis.password=613613redis.timeout=10000redis.maxIdle=300redis.maxTotal=1000redis.maxWaitMillis=1000redis.minEvictableIdleTimeMillis=300000redis.numTestsPerEvictionRun=1024redis.timeBetweenEvictionRunsMillis=30000redis.testOnBorrow=trueredis.testWhileIdle=true

applicationContext-redis.xml

<?xml version="1.0" encoding="UTF-8"?>

将redis.properties导入到applicationContext.xml文件中
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>

xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd">

将redis缓存引入到mybatis中

package com.ht.Util;import org.apache.ibatis.cache.Cache;import org.slf4j.Logger;import org.slf4j.LoggerFactory;import org.springframework.dao.DataAccessException;import org.springframework.data.redis.connection.RedisConnection;import org.springframework.data.redis.core.RedisCallback;import org.springframework.data.redis.core.RedisTemplate;import java.util.concurrent.TimeUnit;import java.util.concurrent.locks.ReadWriteLock;import java.util.concurrent.locks.ReentrantReadWriteLock;public class RedisCache implements Cache //实现类{ private static final Logger logger = LoggerFactory.getLogger(RedisCache.class); private static RedisTemplate redisTemplate; private final String id; /** * The {@code ReadWriteLock}. */ private final ReadWriteLock readWriteLock = new ReentrantReadWriteLock(); @Override public ReadWriteLock getReadWriteLock() { return this.readWriteLock; } public static void setRedisTemplate(RedisTemplate redisTemplate) { RedisCache.redisTemplate = redisTemplate; } public RedisCache(final String id) { if (id == null) { throw new IllegalArgumentException("Cache instances require an ID"); } logger.debug("MybatisRedisCache:id=" + id); this.id = id; } @Override public String getId() { return this.id; } @Override public void putObject(Object key, Object value) { try{ logger.info(">>>>>>>>>>>>>>>>>>>>>>>>putObject: key="+key+",value="+value); if(null!=value) redisTemplate.opsForValue().set(key.toString(),value,60, TimeUnit.SECONDS); }catch (Exception e){ e.printStackTrace(); logger.error("redis保存数据异常!"); } } @Override public Object getObject(Object key) { try{ logger.info(">>>>>>>>>>>>>>>>>>>>>>>>getObject: key="+key); if(null!=key) return redisTemplate.opsForValue().get(key.toString()); }catch (Exception e){ e.printStackTrace(); logger.error("redis获取数据异常!"); } return null; } @Override public Object removeObject(Object key) { try{ if(null!=key) return redisTemplate.expire(key.toString(),1,TimeUnit.DAYS); }catch (Exception e){ e.printStackTrace(); logger.error("redis获取数据异常!"); } return null; } @Override public void clear() { Long size=redisTemplate.execute(new RedisCallback() { @Override public Long doInRedis(RedisConnection redisConnection) throws DataAccessException { Long size = redisConnection.dbSize(); //连接清除数据 redisConnection.flushDb(); redisConnection.flushAll(); return size; } }); logger.info(">>>>>>>>>>>>>>>>>>>>>>>>clear: 清除了" + size + "个对象"); } @Override public int getSize() { Long size = redisTemplate.execute(new RedisCallback() { @Override public Long doInRedis(RedisConnection connection) throws DataAccessException { return connection.dbSize(); } }); return size.intValue(); }}

RedisCacheTransfer

package com.ht.Util;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.data.redis.core.RedisTemplate;public class RedisCacheTransfer { @Autowired public void setRedisTemplate(RedisTemplate redisTemplate) { RedisCache.setRedisTemplate(redisTemplate); }}

接下来,我们在BookMapper.xml中配置RedisCache缓存

测试:

@Test public void cacheSingle() { Book book= this.bookService.selectByPrimaryKey(5); System.out.println(book); Book book2= this.bookService.selectByPrimaryKey(5); System.out.println(book2); }

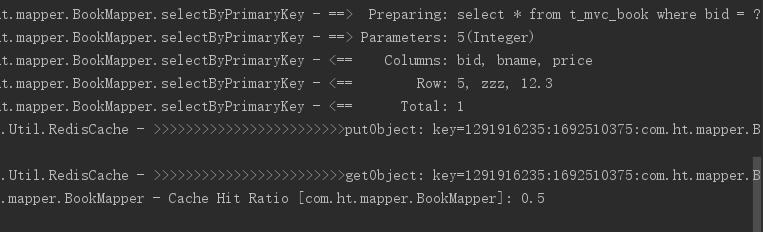
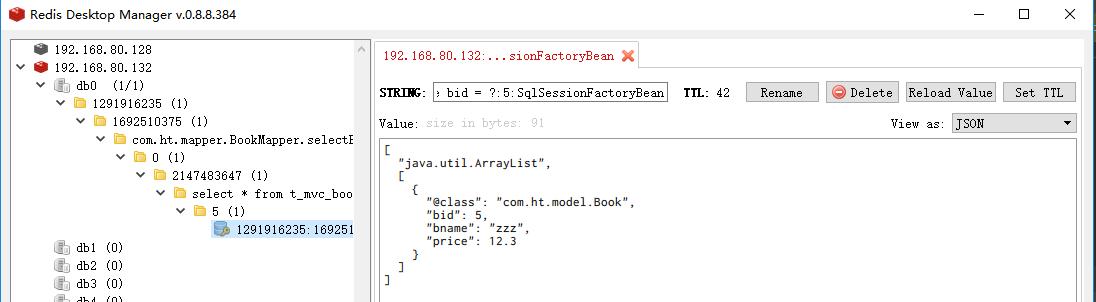