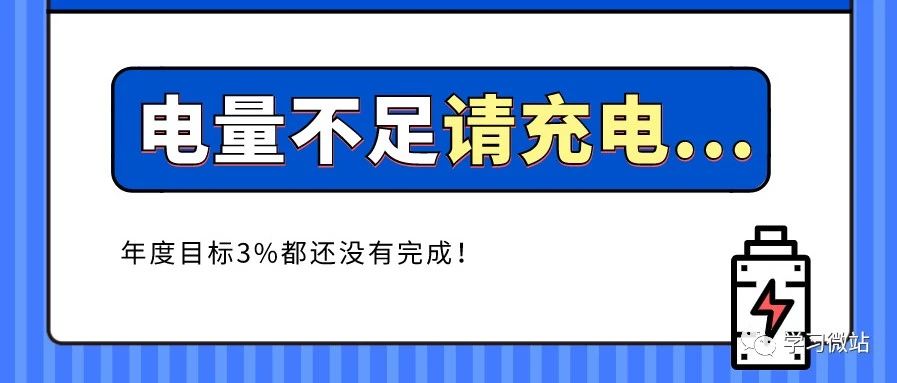
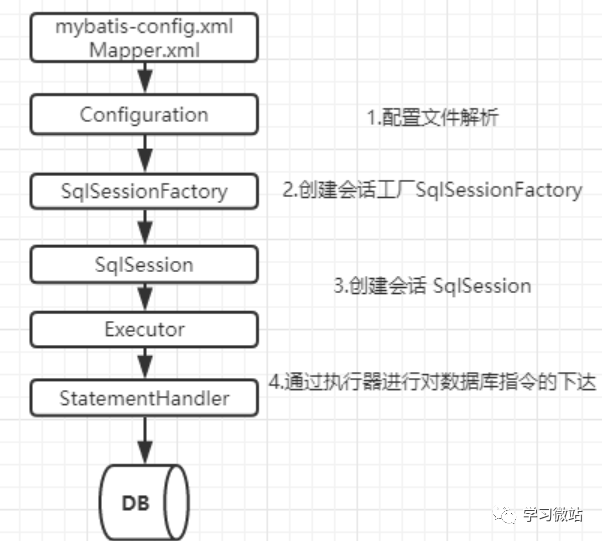
2.有了这些信息就能创建SqlSessionFactory,SqlSessionFactory的生命周期是程序级,程序运行的时候建立起来,程序结束的时候消亡
3.SqlSessionFactory建立SqlSession,目的执行sql语句,SqlSession是过程级,一个方法中建立,方法结束应该关闭
4.当用户使用mapper.xml文件中配置的的方法时,mybatis首先会解析sql动态标签为对应数据库sql语句的形式,并将其封装进MapperStatement对象,然后通过executor将sql注入数据库执行,并返回结果。
5.将返回的结果通过映射,包装成java对象。
1、jdbc.properties,下面会调用
jdbc.username=rootjdbc.password=rootjdbc.driverClass=com.mysql.jdbc.Driverjdbc.url=jdbc:mysql://localhost:3306/数据库
2、mapper配置
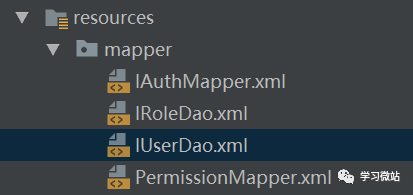
<?xml version="1.0" encoding="UTF-8" ?><!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"><mapper namespace="com.....IUserDao">
1 在mybatis中,映射文件中的namespace是用于绑定Dao接口的,即面向接口编程。当你的namespace绑定接口后,你可以不用写接口实现类,mybatis会通过该绑定自动帮你找到对应要执行的SQL语句
public interface IUserDao extends IBaseDao<User>{ public void modifyPassword(User user);}
对应xml有
<!--修改密码--> <update id="modifyPassword" parameterType="com.qf.entity.User"> UPDATE t_user set password=#{password} where id=#{id}; </update>
2 resultMap的使用
在mybatis中有一个resultMap标签,它是为了映射select查询出来结果的集合,其主要作用是将实体类中的字段与数据库表中的字段进行关联映射。2.1数据库与实体类之间名称相同前提要实体类和数据库字段名称一毛一样,实际一般不这样,在mapper中的查询方式:

2.2 数据库与实体类之间不相同第一种:开启驼峰规则mybtis中开启
<setting name="mapUnderscoreToCamelCase" value="true" />
<?xml version="1.0" encoding="UTF-8" ?><!DOCTYPE mapperPUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd"><mapper namespace="menu.mysql"> <select id="selectMenu" resultType="org.me.menu.Menu"> select MENU_ID, MENU_NAME, PARENT_ID, URL, TITLE, LEAF, ORDER_SEQ from mysql.MENU order by ORDER_SEQ </select></mapper>
第二种:使用resultMap标签来映射
然后在mapper.xml中书写resultMap标签,使得数据库字段和实体类
名称映射。(将实体类字段与数据库字段在标签中进行一一映射)
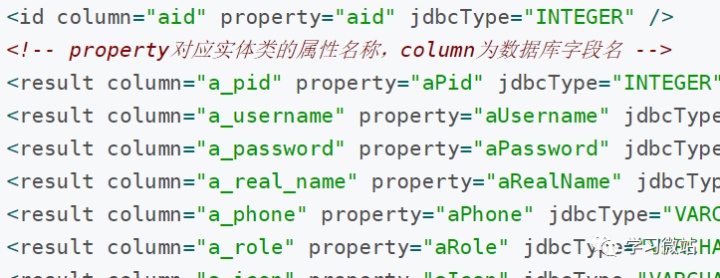
<resultMap id="BaseResultMap" type="com...entity.User" > <id column="id" property="id" jdbcType="INTEGER"/> <result column="username" property="username" jdbcType="VARCHAR" /> <result column="password" property="password" jdbcType="VARCHAR" /> <result column="age" property="age" jdbcType="INTEGER" /> <result column="sex" property="sex" jdbcType="INTEGER" /> <result column="birthday" property="birthday" jdbcType="DATE" /> <result column="create_time" property="createTime" jdbcType="TIMESTAMP" /> <result column="create_user" property="createUser" jdbcType="INTEGER" /> <result column="update_time" property="updateTime" jdbcType="TIMESTAMP" /> <result column="update_user" property="updateUser" jdbcType="INTEGER" /> <result column="email" property="email" jdbcType="VARCHAR" /> <result column="flag" property="flag" jdbcType="INTEGER" /> <result column="png" property="png" jdbcType="LONGVARCHAR" /> </resultMap>
3、配置数据库
什么是数据源JDBC2.0 提供了javax.sql.DataSource接口,它负责建立与数据库的连接,当在应用程序中访问数据库时不必编写连接数据库的代码,直接引用DataSource获取数据库的连接对象即可。用于获取操作数据Connection对象。
<!-- 1.加载配置文件--> <context:property-placeholder location="classpath:jdbc.properties"/> <!-- 2.dataSource --> <bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource" init-method="init" destroy-method="close"> <property name="driverClassName" value="${jdbc.driverClass}"/> <property name="url" value="${jdbc.url}"/> <property name="username" value="${jdbc.username}"/> <property name="password" value="${jdbc.password}"/> </bean>
4、spring-mybatis.xml核心文件的配置
<?xml version="1.0" encoding="UTF-8"?><beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:lang="http://www.springframework.org/schema/lang" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd http://www.springframework.org/schema/lang http://www.springframework.org/schema/lang/spring-lang.xsd"> <!-- 包含配置文件进来--> <import resource="classpath:spring-datasource.xml"/> <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <property name="dataSource" ref="dataSource"/> <property name="typeAliasesPackage" value="com....entity"/> <property name="mapperLocations" value="classpath:mapper/*.xml"/> <property name="plugins"> <array> <bean class="com.github.pagehelper.PageInterceptor"></bean><!--这里用了pagehelper插件在mybtis的配置,就是分页的插件--> </array> </property> </bean> <bean id="tx" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name="dataSource" ref="dataSource"/> </bean> <tx:annotation-driven transaction-manager="tx"/> <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <property name="basePackage" value="com.qf.dao"/> <property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"/> </bean></beans>