这个示例程序估计很多人都用过。没有用过的话可以从
http://www.microsoft.com/downloads/details.aspx?FamilyID=966C3279-2EE9-4E14-A4F7-D4807239A396&displaylang=en 下载
一个简单的股票买卖程序,数据库访问和部分业务逻辑提供了 COM+ 企业服务和 DotNet Remoting 两种方式。
具体的架构参考一下图:
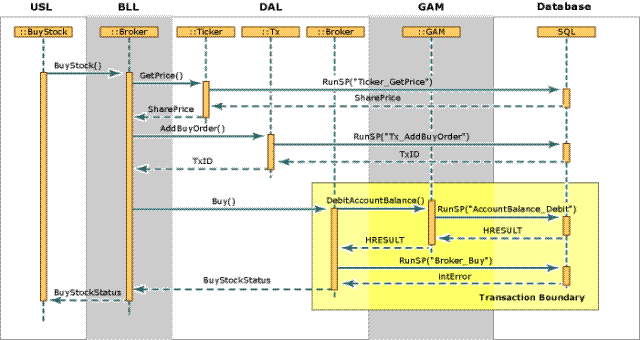
两种分布式应用,有一下特征,呵呵。 msdn 说得
- ASP.NET 应用程序(通过 IIS)承载远程组件以利用进程回收等功能和应用程序配置。
- 通过 HTTP 信道使用二进制格式化程序进行远程调用。使用 HTTP 信道将能够使用 IIS 承载组件,而二进制格式化程序的性能优于 SOAP 格式化程序。
- GAM 和 BLL 对象都是无状态的,这使得可以在 Application Center 群集中承载它们。
- 虽然 GAM 和 BLL 对象是无状态的,但 Fitch and Mather 7.0 使用实例方法(与静态方法相对)以便能够远程处理方法调用。静态方法总是在本地执行,即与调用方在同一个 AppDomain 类中执行。有关更多信息,请参见。
当然对于第一点,你也可以host Remoting 对象在一个 console 应用程序或者 windows 服务中。从而可以使用 TCP/Binary. 缺点TCP 需要自己去考虑安全监听。
我觉得有几点写的不错:
1。清晰的应用程序架构,由于问题比较简单。很容易整理清楚
2。 很好的coding 风格。最近在整理公司的代码规范,发现 FMStocks7 的代码注释确实不错。
比如一下是 买一个新股票的 DAO 代码

/**//// <summary>
/// Purchase shares of a specific stock.
/// <param name='accountID'>The accountID number</param>
/// <param name='txID'>The transaction ID</param>
/// <param name='ticker'>The ticker symbol to be purchased</param>
/// <param name='shares'>Amount of shares</param>
/// <param name='sharePrice'>The share price</param>
/// <param name='commission'>The buy commission</param>
/// <returns>BrokerStatus enum value</returns>
/// </summary>
public BrokerStatus Buy( int accountID, int txID, string ticker, int shares, decimal sharePrice, decimal commission )

{
Debug.Assert( sproc == null );


try
{
BrokerStatus status;
// Initialize order info

Order order;

order.AccountID = accountID;
order.TxID = txID;
order.Ticker = ticker;
order.Shares = shares;
order.SharePrice = sharePrice;
order.Commission = commission;

// Debit account balance

decimal debitAmt = ( decimal )order.Shares * order.SharePrice + order.Commission;
GAM gam = new GAM();

if ( gam.DebitAccountBalance( order.AccountID, debitAmt ) == 0 )

{
status = BrokerStatus.InsufficientFunds;
ContextUtil.SetAbort();
}
else

{
// Create parameter array

SqlParameter[] parameters =

{
new SqlParameter( "@TxID", SqlDbType.Int, 4 ), // 0
new SqlParameter( "@AccountID", SqlDbType.Int, 4 ), // 1
new SqlParameter( "@Ticker", SqlDbType.NChar, 6 ), // 2
new SqlParameter( "@Shares", SqlDbType.Int, 4 ), // 3
new SqlParameter( "@SharePrice", SqlDbType.Money, 8 ), // 4
new SqlParameter( "@Commission", SqlDbType.Money, 8 ), // 5
};

// Set parameter values and directions

parameters[ 0 ].Value = order.TxID;
parameters[ 1 ].Value = order.AccountID;
parameters[ 2 ].Value = order.Ticker;
parameters[ 3 ].Value = order.Shares;
parameters[ 4 ].Value = order.SharePrice;
parameters[ 5 ].Value = order.Commission;

// Run the stored procedure

sproc = new StoredProcedure( "Broker_Buy", parameters );
int error = sproc.Run();
Debug.Assert( error == 0 );

status = BrokerStatus.Success;
ContextUtil.SetComplete();
}
return status;
}
catch

{
ContextUtil.SetAbort();
throw;
}
}
有几点很符合代码规范
1。合理的使用断言
2。// 注释风格
3。异常处理
4。。。。