重新实现 UIPickerView
参考资料:
http://www.cocoachina.com/bbs/read.php?tid=85374
http://www.cocoachina.com/iphonedev/toolthain/2011/1205/3663.html
设置循环滚动
设置 UIPickerView 的数据源数量为很大的规模, 取数据时对行数进行取模, 从而实现循环滚动的效果.
在每次滚动结束后, 把当前选这个行无动画滚动到数据的中间, 减少用户滚动到数据边界的可能.
参考资料:
http://www.cocoachina.com/bbs/read.php?tid=27023
http://code4app.com/codesample/4f67fbb96803fa833f000002
http://blog.csdn.net/ipromiseu/article/details/7436521
修改滚动速度
比较困难. 需要继承 UIPickerView, 并实现未公开函数
参考资料:
http://iphonedevelopment.blogspot.com/2009/01/longer-spinning-blurring.html
需要FQ, 把原文章复制过来.
Longer Spinning & Blurring
I can't tell you how many times I've been asked lately how to do the "UrbanSpoon Effect".
Now, I have no idea exactly how the folks at UrbanSpoon did their application, but they did it well. I don't have time for a full tutorial right now, or to do a full implementation of the effect, but in the process of helping somebody in a forum, I did write a little sample application that shows the basic theory of how to make your UIPickerView go round and round for longer.
As I said, this project nowhere near as polished as what UrbanSpoon does - I cheated on the blurring because I hit some bugs while porting my NSImage convolution kernel code to work with UIImage and I don't have the time to debug something that gnarly right now. My "blur" is just a double resize - down then back up. It's really just interpolation, but it sorta makes it blurry. I probably wouldn't use that method of blurring in a real application but, then again, I'd be getting paid for a real application.
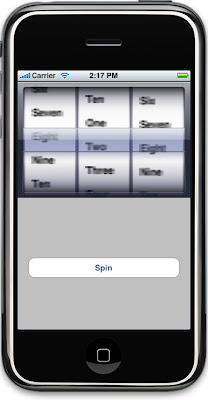
This application does support using the accelerometer to start the spin. In order to make the picker view spin longer, I subclassedUIPickerView. My subclass had exactly one method. This is an undocumented method that returns the duration of the spin, so you just return the number of seconds you want your spin to go for:
The other key thing to note in this application is that my UIPickerViewDataSource lies about how many rows each component has. I have a constant called kRowMultiplier. I take the count of the array that corresponds to a particular component, and I multiply it by kRowMultiplier. This value is currently set to 100, so if the array that feeds a component has ten items, my datasource lies and says there are a thousand, and then it just keeps feeding the same ten items over and over in the same order by calculating the actual row in the array to use:
This creates a component with the same values repeated over and over.
When the view first appears, I load arrays with views containing both the blurred and unblurred data. You don't want to be programmatically blurring the values over and over when the spin happens, so we do it once and store the values in arrays. I then set the component's row to a value in the middle. This means that the user will never see the blank rows above and below the real values, which helps create the illusion of endless spinning.
Now, when a value is selected in the picker, I do a bait-and-switch back to the middle. I move the component to the same value they're currently on, but back in the middle of the wheel. This way, no matter what happens, they'll never see the blank rows at the top or bottom. Since I'm moving them to the same value and the surrounding values are the same, and I tell it not to animate the change, the user has no idea it has happened.
Finally, when the spin button is pressed, or the user shakes the phone, I calculate a random number for each component. This number is a value between 0 and the size of the array minus 1. This tells me what the new, randomly selected value will be. Then I move the components (again, without animating) to the corresponding row near the top or bottom of the dial, and then animate to the selected value at the other end of the wheel.
I set a variable called isSpinning so that I know whether to provide blurred or unblurred data to the spinner. I have the spin set for 5 seconds, so 4.7 seconds in the future, I set the value back to NO. This cause it to stop blurring a moment before the spinning stops. This is done so that the final words displayed will not be blurred.
You can download the sample project here.
One note, though: I have used a couple of undocumented, private methods in this project. I ordinarily shy away from doing that, but in the sake of getting this code out the door quickly, I did. I use one undocumented method for resizing the UIImage in order to create the fake blur effect, and another one to keep the picker from making sounds when I initially set the values because I don't want the user to realize I'm moving the picker - that would shatter the illusion. These are pretty subtle uses of undocumented methods so I doubt they'd get your application rejected, but they could. They're also not methods that are likely to change. But, again, they could - that's always a risk with undocumented methods, so caveat emptor - use at your own risk. If you want to play it safe, to a real motion blur and take out the calls to UIPickerView's setSoundsEnabled: method.
Note: There is a newer version of this code available here.
Now, I have no idea exactly how the folks at UrbanSpoon did their application, but they did it well. I don't have time for a full tutorial right now, or to do a full implementation of the effect, but in the process of helping somebody in a forum, I did write a little sample application that shows the basic theory of how to make your UIPickerView go round and round for longer.
As I said, this project nowhere near as polished as what UrbanSpoon does - I cheated on the blurring because I hit some bugs while porting my NSImage convolution kernel code to work with UIImage and I don't have the time to debug something that gnarly right now. My "blur" is just a double resize - down then back up. It's really just interpolation, but it sorta makes it blurry. I probably wouldn't use that method of blurring in a real application but, then again, I'd be getting paid for a real application.
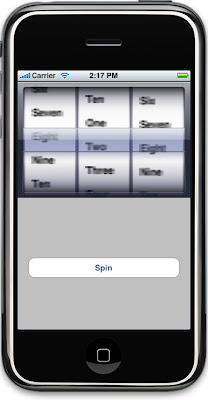
This application does support using the accelerometer to start the spin. In order to make the picker view spin longer, I subclassedUIPickerView. My subclass had exactly one method. This is an undocumented method that returns the duration of the spin, so you just return the number of seconds you want your spin to go for:
The other key thing to note in this application is that my UIPickerViewDataSource lies about how many rows each component has. I have a constant called kRowMultiplier. I take the count of the array that corresponds to a particular component, and I multiply it by kRowMultiplier. This value is currently set to 100, so if the array that feeds a component has ten items, my datasource lies and says there are a thousand, and then it just keeps feeding the same ten items over and over in the same order by calculating the actual row in the array to use:
int actualRow = row% ;
This creates a component with the same values repeated over and over.
When the view first appears, I load arrays with views containing both the blurred and unblurred data. You don't want to be programmatically blurring the values over and over when the spin happens, so we do it once and store the values in arrays. I then set the component's row to a value in the middle. This means that the user will never see the blank rows above and below the real values, which helps create the illusion of endless spinning.
;
Now, when a value is selected in the picker, I do a bait-and-switch back to the middle. I move the component to the same value they're currently on, but back in the middle of the wheel. This way, no matter what happens, they'll never see the blank rows at the top or bottom. Since I'm moving them to the same value and the surrounding values are the same, and I tell it not to animate the change, the user has no idea it has happened.
- (void)pickerView:(UIPickerView *)pickerView didSelectRow:(NSInteger)row inComponent:(NSInteger)component
{
NSArray *componentArray = ;
int actualRow = row% ;
int newRow = ( * (kRowMultiplier / 2)) + actualRow;
;
}
Finally, when the spin button is pressed, or the user shakes the phone, I calculate a random number for each component. This number is a value between 0 and the size of the array minus 1. This tells me what the new, randomly selected value will be. Then I move the components (again, without animating) to the corresponding row near the top or bottom of the dial, and then animate to the selected value at the other end of the wheel.
- (IBAction)spin
{
if
{
// Calculate a random index in the array
spin1 = arc4random()% ;
spin2 = arc4random()% ;
spin3 = arc4random()% ;
// Put first and third component near top, second near bottom
;
;
;
// Spin to the selected value
;
;
;
isSpinning = YES;
// Need to have it stop blurring a fraction of a second before it stops spinning so that the final appearance is not blurred.
;
}
}
I set a variable called isSpinning so that I know whether to provide blurred or unblurred data to the spinner. I have the spin set for 5 seconds, so 4.7 seconds in the future, I set the value back to NO. This cause it to stop blurring a moment before the spinning stops. This is done so that the final words displayed will not be blurred.
You can download the sample project here.
One note, though: I have used a couple of undocumented, private methods in this project. I ordinarily shy away from doing that, but in the sake of getting this code out the door quickly, I did. I use one undocumented method for resizing the UIImage in order to create the fake blur effect, and another one to keep the picker from making sounds when I initially set the values because I don't want the user to realize I'm moving the picker - that would shatter the illusion. These are pretty subtle uses of undocumented methods so I doubt they'd get your application rejected, but they could. They're also not methods that are likely to change. But, again, they could - that's always a risk with undocumented methods, so caveat emptor - use at your own risk. If you want to play it safe, to a real motion blur and take out the calls to UIPickerView's setSoundsEnabled: method.