react-hooks
Sometimes 5 minutes is all you've got. So in this article, we're just going to touch on two of the most used hooks in React: useState
and useEffect
.
有时只有5分钟。 因此,在本文中,我们仅涉及React中两个最常用的钩子: useState
和useEffect
。
If you're not famliar with hooks, here's the TL;DR: because of hooks, there's almost no more need for class-based components. Hooks let you "hook into" the underlying lifecycle and state changes of a component within a functional component. More than that, they often also improve readability and organization of your components.
如果您不熟悉钩子,请参阅TL; DR:由于有了钩子,几乎不再需要基于类的组件。 挂钩使您可以“挂钩”功能组件内组件的基础生命周期和状态更改。 不仅如此,它们还经常提高组件的可读性和组织性。
If you want a proper introduction to this subject, you can join the waitlist for my upcoming advanced React course, or if you're still a beginner, check out my introductory course on React.
如果您想对该主题进行适当的介绍,可以加入我即将举行的高级React课程的候补名单,或者如果您仍然是初学者,请查看我的React入门课程。
useState
(useState
)
Let's begin with a functional component.
让我们从功能组件开始。
import React from 'react';function App() {return (<div><h1>0</h1><button>Change!</button></div>);
}
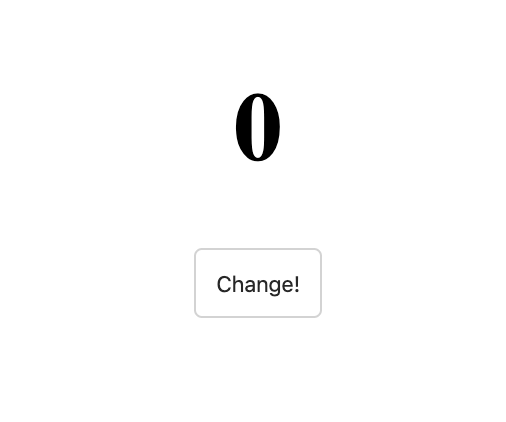
As you can see, nothing fancy at the moment. We're just rendering some text and a (useless) button.
如您所见,目前没有任何幻想。 我们只是渲染一些文本和一个(无用的)按钮。
Now let's import our very first hook, useState
to learn how to handle state in our functional component.
现在,让我们导入我们的第一个钩子useState
以学习如何处理功能组件中的状态。
As this hook is a function, let's console.log
what we get returned from it.
由于此钩子是一个函数,因此,请console.log
从钩子返回的内容。
import React, { useState } from 'react';function App() {const value = useState();console.log(value);return (<div><h1>0</h1><button>Change!</button></div>);
}
In the console, we get an array
在控制台中,我们得到一个数组
> [null, ƒ()]
And when we pass an argument to useState
当我们将参数传递给useState
const value = useState(true);
In the console, we get an array with our value as the first member.
在控制台中,我们得到一个以我们的值作为第一个成员的数组。
> [true, ƒ()]
Now, in our component, we can access our state at value[0]
and render it in <h1>
instead of a hardcoded value.
现在,在组件中,我们可以访问value[0]
处的状态,并在<h1>
呈现它,而不是硬编码值。
import React, { useState } from 'react';function App() {const value = useState(0);console.log(value); // [0, ƒ()]return (<div><h1>{value[0]}</h1><button>Change!</button></div>);
}
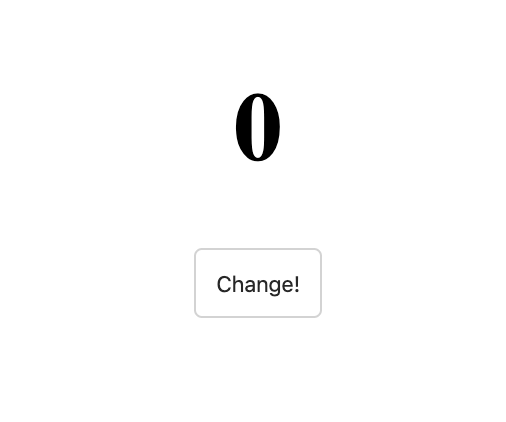
We can improve our code by using array destructuring to store the value from useState
hook. It's similar to object destructuring, which tends to be a bit more commonly seen. In case you're not super familiar with object destructuring, here's a quick recap:
我们可以通过使用数组解构来存储useState
挂钩中的值来改进代码。 这类似于对象解构,后者往往更常见。 如果您对对象分解不太熟悉,请快速回顾一下:
const person = {name: 'Joe',age: 42
};// creates 2 const values from person object
const { name, age } = person;
console.log(name); // 'Joe'
console.log(age); // 42
Array destructing is almost the same, but uses square brackets []
instead of curly braces {}
.
数组销毁几乎相同,但是使用方括号[]
代替大括号{}
。
A quick tip: in object destructuring, the names of created variables must match the names of properties in the object. For array destructuring, that's not the case. It's all about the order. The benefit here is we can name the items whatever we want.
快速提示:在对象分解中,创建的变量的名称必须与对象中的属性的名称匹配。 对于数组解构,情况并非如此。 都是为了订单。 这样做的好处是我们可以随意命名项目。
Using array destructuring, we can get the initial value of state from the useState()
hook.
使用数组解构,我们可以从useState()
挂钩中获取state的初始值。
import React, { useState } from 'react';function App() {// remember, there's a second item from the array that's missing here, but we'll come right back to use it soonconst [count] = useState(0); return (<div><h1>{count}</h1><button>Change!</button></div>);
}
OK, we've got the initial state value. How do we change the value in the state with hooks?
好的,我们已经有了初始状态值。 我们如何使用钩子更改状态值?
Remember that useState()
hook returns an array with 2 members. The second member is a function that updates the state!
请记住, useState()
挂钩返回一个包含2个成员的数组。 第二个成员是一个更新状态的函数!
const [count, setCount] = useState(0);
You can, of course, call it what you wish, but by convention, it's normally called with prefix "set-", and then whatever state variable we wish to update was called, so setCount
it is.
您当然可以将其命名为所需的名称,但是按照惯例,通常使用前缀“ set-”来调用它,然后调用我们希望更新的任何状态变量,因此使用setCount
。
It's simple to use this function. Just call it and pass the new value you want that state to have! Or, just like this.setState
in a class component, you can pass a function that receives the old state and returns the new state. Rule of thumb: do this anytime you need to rely on the past state to determine the new state.
使用此功能很简单。 只需调用它并传递您希望该状态具有的新值即可! 或者,就像类组件中的this.setState
一样,您可以传递一个接收旧状态并返回新状态的函数。 经验法则:只要您需要依靠过去的状态来确定新的状态,就可以执行此操作。
To call it, we'll pass it to the onClick
event listener. And just like with a regular setState
in a class-based component, we can pass our state update to setCount
.
要调用它,我们将其传递给onClick
事件侦听器。 就像在基于类的组件中使用常规setState
一样,我们可以将状态更新传递给setCount
。
function App() {const [count, setCount] = useState(0);return (<div><h1>{count}</h1><button onClick={() => setCount(prevCount => prevCount + 1)}>Change!</button></div>);
}
We can clean this up a bit, by extracting our state update to a separate function.
通过将状态更新提取到单独的函数中,我们可以进行一些清理。
function App() {const [count, setCount] = useState(0);function change() {setCount(prevCount => prevCount + 1);}return (<div><h1>{count}</h1><button onClick={change}>Change!</button></div>);
}
Great! And now when we can see the counter going up when we click the button.
大! 现在,当我们单击按钮时可以看到计数器在上升。
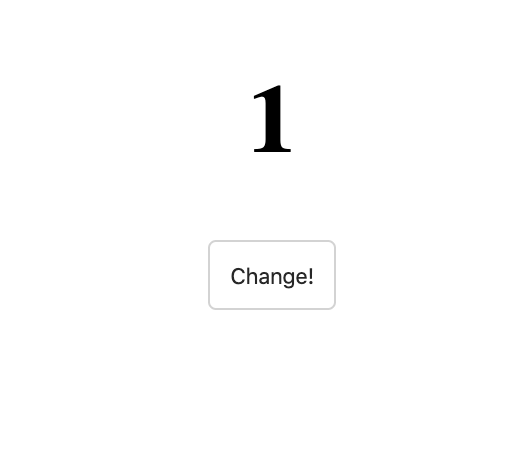
Of course, useState
can get a lot more complicated than this, but we've only got 5 minutes here, so let's move on to the next hook for now.
当然, useState
可能比这复杂得多,但是这里只有5分钟,所以现在让我们继续下一个钩子。
useEffect
(useEffect
)
Hooks have simplified quite a few things, compared to the way things were in class-based components. Previously we needed to know a bit about lifecycle methods and which one is best suited for which situation. useEffect
hook simplified this situation. If you wish to perform side effects, network request, manual DOM manipulation, event listeners or timeouts and intervals.
与基于类的组件相比,钩子简化了很多事情。 以前,我们需要对生命周期方法有所了解,并且哪种方法最适合哪种情况。 useEffect
钩子简化了这种情况。 如果您希望执行副作用,网络请求,手动DOM操作,事件侦听器或超时和间隔。
useEffect
hook can be imported just like useState
.
useEffect
钩子可以像useState
一样useState
。
import React, { useState, useEffect } from 'react';
To make useEffect
do something, we pass it an anonymous function as an argument. Whenever React re-renders this component, it will run the function we pass to useEffect
.
为了使useEffect
有所作为,我们将其传递给匿名函数作为参数。 每当React重新渲染此组件时,它将运行我们传递给useEffect
的函数。
useEffect(() => {/* any update can happen here */
});
This is what the whole code might look like.
这就是整个代码的样子。
import React, { useState, useEffect } from 'react';function App() {const [count, setCount] = useState(0);function change() {setCount(prevCount => prevCount + 1);}useEffect(() => {/* any update can happen here */});return (<div><h1>{count}</h1><button onClick={change}>Change!</button></div>);
}export default App;
As an example, we will use a nice npm
package that generates a random color. Feel free to write your own if you wish of course, but for this tutorial, we will just install it, npm i randomcolor
, and import.
例如,我们将使用一个不错的npm
包,该包会生成随机颜色。 当然,如果愿意,可以自己编写,但是对于本教程,我们将直接安装它, npm i randomcolor
并导入。
import randomcolor from 'randomcolor';
Let's now use our knowledge about useState
hook to store some random color in the state.
现在,让我们使用关于useState
挂钩的知识在状态中存储一些随机颜色。
const [color, setColor] = useState(''); // initial value can be an empty string
We then can then assign the color of the counter we already have.
然后,我们可以分配已有的计数器的颜色。
<h1 style={{ color: color }}>{count}</h1>
Now, just for the sake of it, let's change the color of the counter on every click of the Change!
button. useEffect
will run every time the component re-renders, and the component will re-render every time the state is changed.
现在,仅出于此目的,让我们在每次单击Change!
计数器的颜色Change!
按钮。 useEffect
将在每次重新渲染组件时运行,并且每次在状态更改时都将重新渲染组件。
So if we write the following code, it would get us stuck in an infinite loop! This is a very common gotcha with useEffect
因此,如果我们编写以下代码,它将使我们陷入无限循环! 这是useEffect
的非常常见的useEffect
useEffect(() => {setColor(randomcolor());
});
setColor
updates state, which re-renders the component, which calls useEffect
, which runs setColor
to update the state, which re-renders the component... Yikes!
setColor
更新状态,此状态将重新渲染该组件,并调用useEffect
,后者将运行setColor
来更新状态,该状态将重新渲染该组件……kes!
We probably only want to run this useEffect
when the count
variable changes.
我们可能只需要运行这个useEffect
当count
变量的变化。
To tell useEffect
which variable(s) to keep track of, we give an array of such variables as a second argument.
为了告诉useEffect
哪个变量,我们将此类变量的数组作为第二个参数。
useEffect(() => {setColor(randomcolor());
}, [count]);
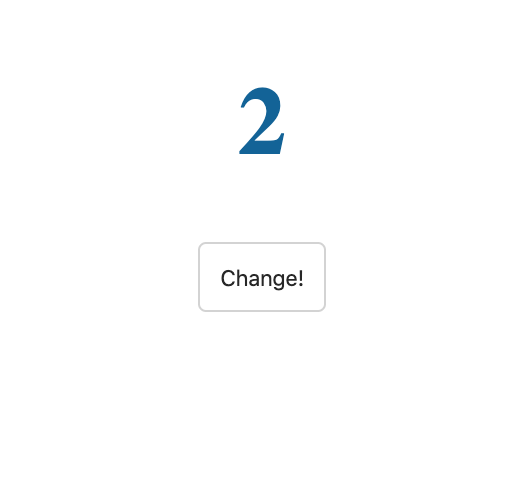
This basically says "only run this effect if the count
state changes. This way we can change the color and not cause our effect to run infinitely.
这基本上说“只运行,如果这种影响count
状态的变化。这样我们就可以改变颜色,并没有引起我们的影响无限运行。
结论 (Conclusion)
There's a lot more to learn about hooks, but I hope you've enjoyed this quick 5-minute peek into hooks.
关于钩子,还有很多要学习的东西,但我希望您喜欢这种快速的5分钟速览钩子。
To learn more about React Hooks and other great features of React, you can join the waitlist for my upcoming advanced React course. Or if you're looking for a more beginner friendly you can check out my introductory course on React.
要了解有关React Hooks和React其他重要功能的更多信息,可以加入我即将举行的高级React课程的候补名单。 或者,如果您正在寻找对初学者更友好的内容,则可以查看我关于React的入门课程。
Happy coding 🤠
快乐编码🤠
翻译自: https://www.freecodecamp.org/news/react-hooks-in-5-minutes/
react-hooks