python 开发api
If you have read some of my previous Python articles, you know I’m a Flask fan. It is my go-to for building APIs in Python. However, recently I started to hear a lot about a new API framework for Python called FastAPI. After building some APIs with it, I can say it is amazing!
如果您阅读过我以前的一些Python文章,您就会知道我是Flask的粉丝。 这是我用Python构建API的必修课。 但是,最近我开始听到很多关于Python的新API框架FastAPI的信息 。 用它构建了一些API之后,我可以说它很棒!
This project was created by Sebastian Ramírez, and at the time of writing, it has accumulated almost 20K stars. Big names like Microsoft, Uber, Netflix, and others have been building APIs with it.
这个项目是由塞巴斯蒂安·拉米雷斯 ( SebastianRamírez)创建的,在撰写本文时,它已经积累了近2万颗星。 像Microsoft,Uber,Netflix等大公司都在使用它来构建API。
But why is this new library so popular and how does it compare to Flask or Django?
但是,为什么这个新库如此受欢迎?与Flask或Django相比,它又如何呢?
特征 (Features)
FastAPI is a rather minimalistic framework. However, that doesn’t make it less powerful. FastAPI is built using modern Python concepts and is based on Python 3.6 type declarations. Let’s see some of the features this library is packed with.
FastAPI是一个相当简单的框架。 但是,这并不会使它的功能降低。 FastAPI是使用现代Python概念构建的,并且基于Python 3.6类型声明。 让我们看一下该库附带的一些功能。
自动文档 (Automatic docs)
A must-have for any API is documentation about the endpoints and types. A common approach to solve this problem is the use of OpenAPI and tools like Swagger UI or ReDoc to present the information. These come packed automatically with FastAPI, allowing you to focus more on your code than setting up tools.
任何API的必备组件都是有关端点和类型的文档。 解决此问题的常用方法是使用OpenAPI和诸如Swagger UI或ReDoc之类的工具来显示信息。 这些都与FastAPI自动打包在一起,使您可以比设置工具更专注于代码。
键入Python (Typed Python)
This is a big one: FastAPI makes use of Python 3.6 type declarations (thanks to Pydantic). This means that it uses a Python feature that allows you to specify the type of a variable. And this framework makes extensive use out of it, providing you with great editor support. Autocompletion works amazingly well.
这是一个很大的问题:FastAPI使用Python 3.6类型声明(感谢Pydantic)。 这意味着它使用Python功能,可让您指定变量的类型。 并且该框架对其进行了广泛使用,为您提供了强大的编辑器支持。 自动补全效果非常好。
Here is some sample code using typed declarations:
这是一些使用类型声明的示例代码:
We just went from:
我们刚从:
name
to:
至:
name: str
That’s it! And as a result:
而已! 结果是:
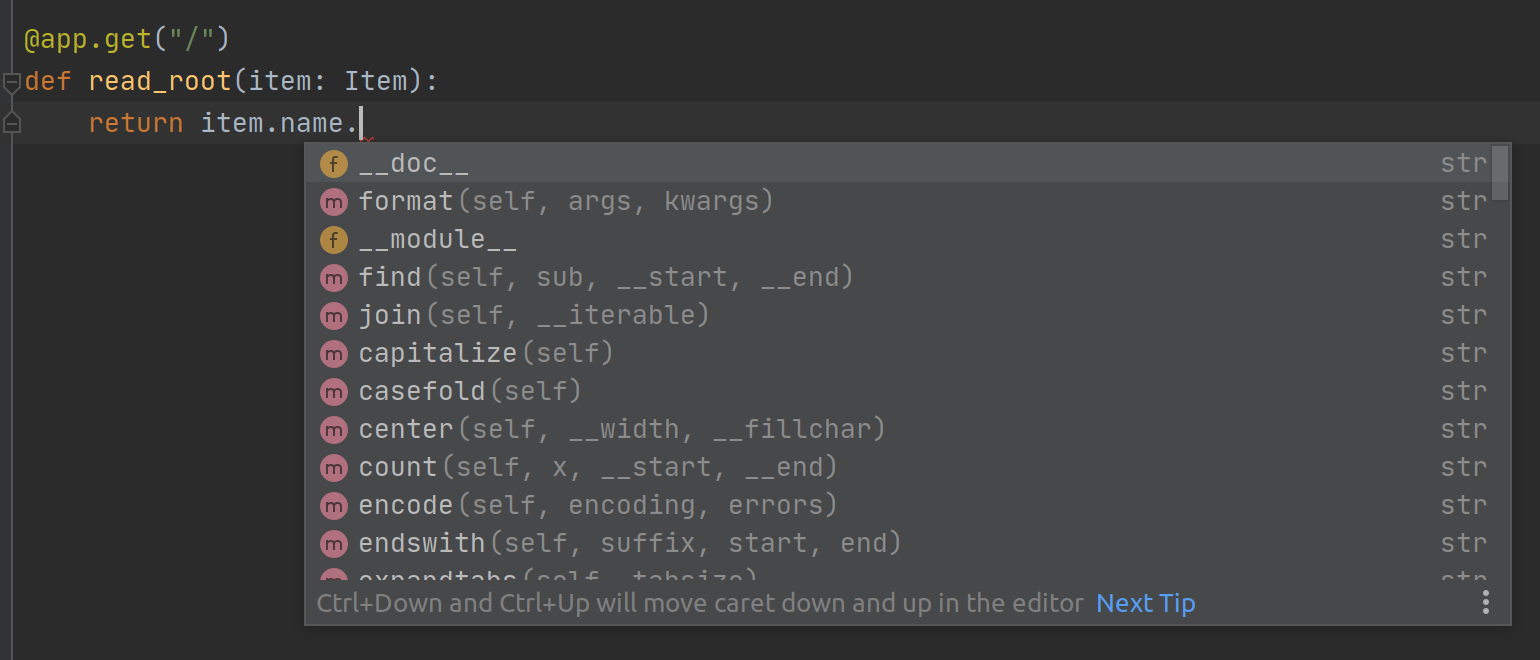
Beautiful!
美丽!
验证方式 (Validation)
Validation is already integrated into this framework thanks to Pydantic. You can validate standard Python types as well as some custom field validations. Here are a few examples:
由于Pydantic,验证已集成到此框架中。 您可以验证标准Python类型以及一些自定义字段验证。 这里有一些例子:
JSON objects (
dict
)JSON对象(
dict
)JSON array (
list
)JSON数组(
list
)String (
str
) with min and max lengths最小长度和最大长度的字符串(
str
)Numbers (
int
,float
) with min and max values带有最小值和最大值的数字(
int
,float
)- URL 网址
- Email 电子邮件
- UUID UUID
- And many more… 还有很多…
安全与认证 (Security and authentication)
This is a crucial part of any API and it’s code that we usually just repeat, so why not integrate much of it into the framework? FastAPI does exactly that.
这是任何API的关键部分,我们通常只重复其中的代码,那么为什么不将其大量集成到框架中呢? FastAPI正是这样做的。
The library provides support for the following:
该库提供以下支持:
- HTTP Basic HTTP基本
- OAuth2 (JWT tokens) OAuth2(JWT令牌)
- API keys in headers, query params, or cookies. 标头,查询参数或cookie中的API密钥。
文献资料 (Documentation)
This is perhaps not exactly a feature of the framework, but it is worth mentioning. The documentation of the project is simply amazing. It’s very clear and covers topics with examples and explanations.
这也许不是框架的确切功能,但是值得一提。 该项目的文档简直太神奇了。 这非常清楚,并通过示例和解释涵盖了主题。
性能 (Performance)
FastAPI is fast! It is not only fast to code, but it also processes requests super fast! You can check the benchmarks across multiple frameworks using the TechEmpower benchmark tool. Here are the results I got for Python frameworks. Flask and Django are way behind on the list, with FastAPI being first and thus the most performant:
FastAPI很快! 它不仅可以快速编码,而且还可以超快地处理请求! 您可以使用TechEmpower基准测试工具来检查多个框架中的基准 。 这是我为Python框架获得的结果。 Flask和Django排在后面,FastAPI排名第一,因此也是性能最高的:
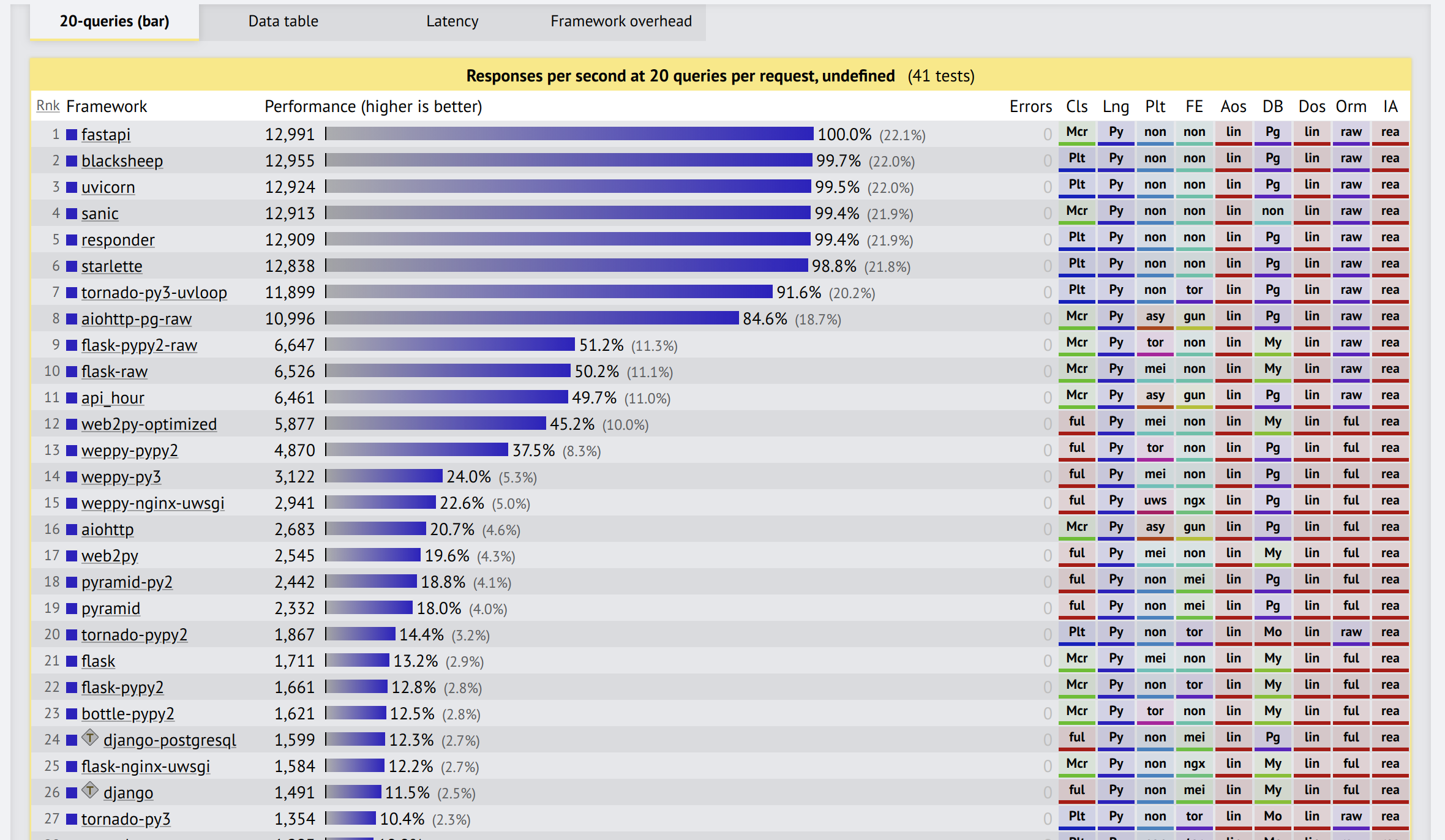
自然异步 (Asynchronous by Nature)
Let’s take a look at the following code:
让我们看下面的代码:
@app.post("/item/", response_model=Item)
async def create_item(item: Item):
result = await some_code_running_in_background(item)
return result
Is that JavaScript? I promise you it is not. Looks familiar, though, right? That snippet is actually Python using async methods.
那是JavaScript吗? 我向你保证不是。 看起来很熟悉,对吧? 该片段实际上是使用异步方法的Python。
FastAPI supports asynchronous endpoints by default, which can simplify and make your code more efficient. This is a huge advantage over Flask. Django already made some async support work, but it is not as integrated as it is in FastAPI.
FastAPI默认情况下支持异步端点,这可以简化并提高代码效率。 与Flask相比,这是一个巨大的优势。 Django已经进行了一些异步支持工作,但是集成程度不如FastAPI。
结论 (Conclusion)
FastAPI is a relatively new framework that follows the minimalist approach of Flask but adds crucial features that make it easier to work with and stunningly performant. It is a great choice for your next API project, and I will be writing more about it as I use it more and more on my APIs.
FastAPI是一个相对较新的框架,它遵循Flask的极简主义方法,但增加了一些关键功能,使其更易于使用且性能惊人。 对于您的下一个API项目来说,这是一个不错的选择,随着我在API上越来越多地使用它,我将对此进行更多的写作。
Thanks for reading!
谢谢阅读!
翻译自: https://medium.com/better-programming/quickly-develop-highly-performant-apis-with-fastapi-and-python-4ac1f252c935
python 开发api
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如若转载,请注明出处:http://www.mzph.cn/news/388087.shtml
如若内容造成侵权/违法违规/事实不符,请联系多彩编程网进行投诉反馈email:809451989@qq.com,一经查实,立即删除!