控制结构
- 程序流程控制介绍
顺序控制
分支控制if-else
单分支
案例演示
01:
import java.util.Scanner;
public class IfWorkDemo
{public static void main(String[] args){Scanner myScanner = new Scanner(System.in);System.out.println("input your age");int age = myScanner.nextInt();if (age > 18){System.out.println("Your age beyond 18 ,you shoule be responsible to your action ");}}
}
流程图
双分支
案例演示
01:
import java.util.Scanner;
public class IfWorkDemo01
{public static void main(String [] args){Scanner myScanner = new Scanner(System.in);int age = myScanner.nextInt();System.out.println("input your age");if (age > 18){System.out.println("Your age beyond 18 ,you shoule be responsible to your action ")}else{System.out.println("You are not old no enough to let you off this time");}}
}
流程图
单分支和双分支练习题
01:
public class PracticeWorkDemo
{public static void main(String [] args){int x = 7;int y = 4;if (x > 5){if (y > 5){System.out.println(x+y);}System.out.println("okokok");}else{System.out.println("x is "+x);}}
}
OUTPUT:
okokok
02:
public class IfWorkDemo
{public static void main(String[] args){double d1 = 34.5;double d2 = 2.6;if (d1 > 10.0 && d2 < 20.0){System.out.println("两个数之和 = "+(d1+d2);}}
}
03:
public class SumWorkDemo
{public static void main(String[] args){int num1 = 10;int num2 = 5;int sum = num1+num2;if (sum % 3==0 && sum % 5==0){System.out.println("ok");}else{System.out.println("no");}}
}
04:
import java.util.Scanner;
public class YearWorkDemo
{public static void main(String[] args){Scaneer myYear = new Scanner(System.in);int year = myYear.nextInt();if ((year %4==0 && year %100 !=0 )|| year %400 ==0){System.out.println("ok");}else{System.out.println("no");}}
}
多分支
流程图
案例演示
import java.util.Scanner;
public class MaWorkDemo
{public static void main(String[] args){Scanner myScanner = new Scanner(System.in);int score = myScanner.nextInt();if (score >= 1 && score <= 100){ if (score == 100){System.out.println("信用极好");}else if (score > 80 && score <= 99){System.out.println("信用优秀");}else if (score >=60 && score <= 80){System.out.println("信用一般");}else{System.out.println("信用不及格");}}else{System.out.println("Input is Error");}}
}
小练习
01:
boolean b = true;
if (b==false)
{System.out.println("a");
}
else if (b)
{System.out.println("b");
}
else if (!b)
{System.out.println("c");
}
else
{System.out.println("d");
}//output = b
02:
boolean b = true;
if (b=false)
{System.out.println("a");
}
else if (b)
{System.out.println("b");
}
else if (!b)
{System.out.println("c");
}
else
{System.out.println("d");
}//output = c
嵌套分支
应用案例
01:
import java.util.Scanner;
public class MatchWorkDemo
{public static void main(String [] args){Scanner myScanner = new Scanner(System.in);System.out.println("Please input score");double score = myScanner.nextDouble();if (score > 8.0){System.out.println("Please input gender");char gender = myScanner.next().charAt(0);if (gender == '男'){System.out.println("Enter the men's division");}else if (gender== '女'){System.out.println("Enter the women's division");}else {System.out.println("Input Error");}}else{System.out.println("cup tie");}}
}
代码略。
switch分支结构
流程图
案例演示
01:
import java.util.Scanner;
public class SwitchWorkDemo
{public static void main(String[] args){Scanner myScanner = new Scanner(System.in);System.out.println("Please input a-g");char c1 = myScanner.next().charAt(0);switch(c1){case 'a':System.out.println("Today is monday");break;case 'b':System.out.println("Today is Tuesday");break;case 'c':System.out.println("Today is Wednesday");break;//......default :System.out.println("Input Error");}}
}
细节讨论
- 表达式数据类型,应和case后的常量类型一致,或者是可以自动转成相互比较的类型,比如输入的是字符,而常量是int
01:
public class SwitchWorkDemo
{public static void main(String[] args){char c = 'a';switch(c){case 'a':System.out.println("ok1");break;//correct char -> int case 20:System.out.println("ok2");break;//error String 无法转成 charcase 'hello':System.out.println("ok3");break;default:System.out.println("ok4");}}
}
02:
public class SwitchWorkDemo
{public static void main(String[] args){String c = "a";switch(c){case "b":System.out.println("ok1");break;case "20":System.out.println("ok2");break;//error String 无法转成 intcase 20:System.out.println("ok3");break;default:System.out.println("ok4");}}
}
- switch(表达式)中表达式的返回值必须是(byte,short,int,char,enum[枚举],String)
01:
public class SwitchWorkDemo
{public static void main(String[] args){double c = 1.1;switch(c){//error switch(表达式)中表达式的返回值必须是(byte,short,int,char,enum[枚举],String)case 1.1:System.out.println("ok1");break;default:System.out.println("ok2");}}
}
- case子句中的值必须是常量(1,‘a’)或者是常量表达式,而不能是变量
class SwitchWork
{public static void main(String[] args){char c = 'a';char c2 = 'c';switch(c){//error case子句中的值必须是常量(1,'a')或者是常量表达式,而不能是变量case c2:System.out.println("ok");break;default:System.out.println("ok2");}}
}
-
default是可选的,就是说,可以没有default。如果没有default子句,有没有匹配任何常量,则没有输出
-
break语句用来在执行完一个case分支后使程序跳出switch语句块,如果没有写break,程序会顺序执行到switch结尾
public class SwitchDemo {public static void main(String[] args){char c = 'b';char c2 = 'c';switch(c){case 'b'://没有break,穿透了System.out.println("ok1");case 'c':System.out.println("ok2");default:System.out.println("ok3");}}}
结果如下:
小练习
01:
import java.util.Scanner;
public class ConvertWork
{public static void main(String[] args){Scanner myScanner = new Scanner(System.in);System.out.println("Please input a-e");char c1 = myScanner.next().charAt(0);switch(c1){case 'a':System.out.println("A");break;case 'b':System.out.println("B");break;case 'c':System.out.println("C");break;case 'd':System.out.println("D");break;case 'e':System.out.println("E");break;default :System.out.println("Input Error");}}
}
02:
public class SwitchDemo
{public static void main(String[] args){double score = 88.5;if (score >=0 && score <=100){switch((int)(score/60)){case 0:System.out.println("不合格");break;case 1:System.out.println("合格");break;// default:// System.out.println("Input Error");}}else{System.out.println("Input Error");}}
}
03:
import java.util.Scanner;
public class SwitchDemo
{public static void main(String [] args){Scanner myScanner = new Scanner(System.in);System.out.println("Input month");int month = myScanner.nextInt();switch(month){case 3:case 4:case 5:System.out.println("springtime");break;case 6:case 7:case 8:System.out.println("summer");break;case 9:case 10:case 11:System.out.println("autumn");case 1:case 2:case 12:System.out.println("winter");default :System.out.println("Input Error");}}
}
switch和if的比较
先死后活,化繁为简
for循环控制
流程图
使用细节
小练习
01:
public class ForExercise
{public static void main(String[] args){int cnt = 0;int sum = 0;int start = 1;int end = 100;int num = 9;for (int i = start;i<=end;i++){if (i % num==0){System.out.println("i = "+i);cnt++;sum+=i;}}System.out.println("cnt = "+cnt);System.out.println("sum = "+sum);}
}
02:
public class ForWorkDemo
{public static void main(String [] args){int n = 5;for (int i = 0;i<=n;i++){System.out.println(i+"+"+(n-i)+"="+n);}}
}
while循环控制
01:
public class WhileWorkDemo
{public static void main(String[] args){int i = 1;while(i <= 10){System.out.println("i = "+i);i++;}}
}
流程图
注意事项和细节说明
小练习
01:
public class WhileWork
{public static void main(String[] args){int i = 1;while(i <= 100){if (i%3==0){System.out.println(i);//i++;//easy error}i++;}}
}
02:
public class WhileWork
{public static void main(String[] args){int i = 40;while(i<=200){if (i %2==0){System.out.println(i);}i++;}}
}
do…while循环控制
流程图
01:
public class DoWhileWork
{public static void main(String[] args){int i = 1;do{System.out.println(i);i++;}while(i <= 10);System.out.println("continue");}
}
注意事项和细节说明
小练习
01:
public class DoWhileWork
{public static void main(String[] args){int cnt = 0;int i = 1;do{if (i % 5==0 && i%3!=0){System.out.println(i);cnt++;}i++;}while(i<=200);System.out.println(cnt);}
}
02:
import java.util.Scanner;
public class DoWhileWork
{public static void main(String[] args){Scanner myScanner = new Scanner(System.in);char answer = ' ';do{System.out.println("老韩使用五连鞭");System.out.println("老韩问:还钱吗?y/n");answer = myScanner.next().charAt(0);System.out.println("His ansmer = "+answer);}while(answer!='y');System.out.println("李三还钱了");}
}
多重循环控制
01:
public class ForWorkDemo
{public static void main(String[] args){for (int i = 0;i<2;i++) for (int j = 0;j<3;j++){System.out.println("i = "+i+" j = "+j);}}
}
结果如下:
小练习
01:
import java.util.Scanner;
public class ForWorkDemo
{public static void main(String[] args){Scanner myScanner = new Scanner(System.in);double sumbig = 0;for (int i = 1;i<=3;i++){double sum = 0;int cnt = 0;for (int j = 1;j<=5;j++){System.out.println("Please input 第"+i+"个班级的第"+j+"个学生");double score = myScanner.nextDouble();sum+=score;if (score >= 60){cnt++;}}System.out.println("第"+i+"个班级及格的学生有"+cnt+"个");System.out.println("第"+i+"个班级的平均分为:" + sum/5);sumbig+=(sum/5);}System.out.println("bigsum = "+(sumbig/3));}
}
02:
代码略。
大练习
化繁为简
01:
public class PrintWorkDemo {public static void main(String[] args){for (int i = 1;i<=5;i++){System.out.println("*****");
// *****
// *****
// *****
// *****
// *****}}
}
02:
public class PrintWorkDemo {public static void main(String[] args){for (int i = 1;i<=5;i++){for (int j = 1;j<=i;j++){System.out.print("*");}System.out.println();}}
}//*
//**
//***
//****
//*****
03:
public class PrintWorkDemo {public static void main(String[] args){for (int i = 1;i<=5;i++){for (int k = 1;k<=5-i;k++){System.out.print(" ");}for (int j = 1;j<=2*i-1;j++){System.out.print("*");}System.out.println();}}
}// *
// ***
// *****
// *******
//*********
04:
public class PrintWorkDemo {public static void main(String[] args){for (int i = 1;i<=5;i++){for (int k = 1;k<=5-i;k++){System.out.print(" ");}for (int j = 1;j<=2*i-1;j++){if (i!=5 && j==1 || j==2*i-1)System.out.print("*");else if (i!=5)System.out.print(" ");elseSystem.out.print("*");}System.out.println();}}
}// *
// * *
// * *
// * *
//*********
跳转控制语句break
- Math.random()
返回带正号的double值,该值大于等于0.0,且小于1.0
01:
public class RandomBreakWork {public static void main(String[] agrs){int cnt = 0;while(true){cnt++;int flag = (int)(Math.random()*100)+1;if (flag==97) break;}System.out.println(cnt);}
}
基本介绍和流程图
注意事项和细节说明
01:
public class RandomBreakWork {public static void main(String[] agrs){label1:for (int j = 0;j<4;j++){label2:for (int i = 0;i<10;i++){if (i == 2) break;System.out.println("i = "+i);}}}
}
结果如下:
02:
public class RandomBreakWork {public static void main(String[] agrs){label1:for (int j = 0;j<4;j++){label2:for (int i = 0;i<10;i++){if (i == 2) break label1;System.out.println("i = "+i);}}}
}
结果如下:
小练习
01:
public class RandomBreakWork {public static void main(String[] agrs){int sum = 0;for (int i = 1;i<=100;i++){sum+=i;if (sum > 20){System.out.println(i);break;}}}
}//------------------------------------------public class RandomBreakWork {public static void main(String[] agrs){int flag = 0;int sum = 0;for (int i = 1;i<=100;i++){sum+=i;if (sum > 20){flag = i;break;}}System.out.println(flag);}
}
02:
tip:
- 字符串的内容比较使用的方法equals
public class StringTestDemo
{public static void main(String[] args){String name = "Tom";System.out.println(name.equals("Jack"));System.out.println("jack".equals(name));//[推荐,可以避免空指针]}
}
import java.util.Scanner;public class RandomBreakWork {public static void main(String[] agrs){Scanner myScanner = new Scanner(System.in);String name = "";String passwd = "";int chance = 3;for (int i = 1;i<=3;i++){System.out.println("Please input name");name = myScanner.next();System.out.println("Please input passwd");passwd = myScanner.next();//compareif("丁真".equals(name) && "666".equals(passwd)){System.out.println("ok");break;}chance--;System.out.println("你还有"+chance+"次机会");}}
}
跳转控制语句continue
流程图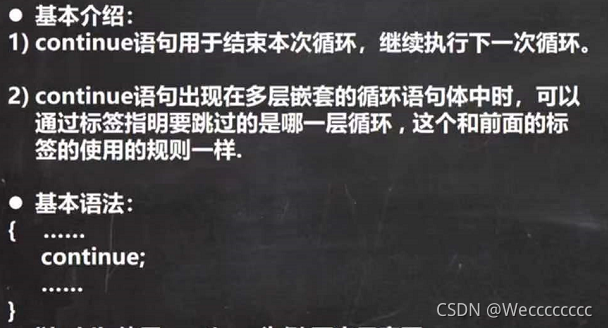
01:
public class RandomBreakWork {public static void main(String[] agrs){int i = 1;while(i <= 4){i++;if (i==2){continue;}System.out.println("i = "+i);
// i = 3
// i = 4
// i = 5}}
}
案例说明
01:
public class RandomBreakWork {public static void main(String[] agrs){label1:for (int j = 0;j<2;j++){label2:for (int i = 0;i<10;i++){if (i==2){continue;}System.out.println("i = "+i);}}}
}
结果如下:
i = 0
i = 1
i = 3
i = 4
i = 5
i = 6
i = 7
i = 8
i = 9
i = 0
i = 1
i = 3
i = 4
i = 5
i = 6
i = 7
i = 8
i = 9
02:
public class RandomBreakWork {public static void main(String[] agrs){label1:for (int j = 0;j<2;j++){label2:for (int i = 0;i<10;i++){if (i==2){continue label1;}System.out.println("i = "+i);}}}
}
结果如下:
i = 0
i = 1
i = 0
i = 1
跳转控制语句return
01:
public class ReturnDemo {public static void main(String[] args){for (int i = 1;i<=5;i++){if (i==3){System.out.println(i);break ;}System.out.println("hello world");}System.out.println("go on...");}
}
结果如下:
hello world
hello world
3
go on…
02:
public class ReturnDemo {public static void main(String[] args){for (int i = 1;i<=5;i++){if (i==3){System.out.println(i);continue;}System.out.println("hello world");}System.out.println("go on...");}
}
结果如下:
hello world
hello world
3
hello world
hello world
go on…
03:
public class ReturnDemo {public static void main(String[] args){for (int i = 1;i<=5;i++){if (i==3){System.out.println(i);return;}System.out.println("hello world");}System.out.println("go on...");}
}
结果如下:
hello world
hello world
3