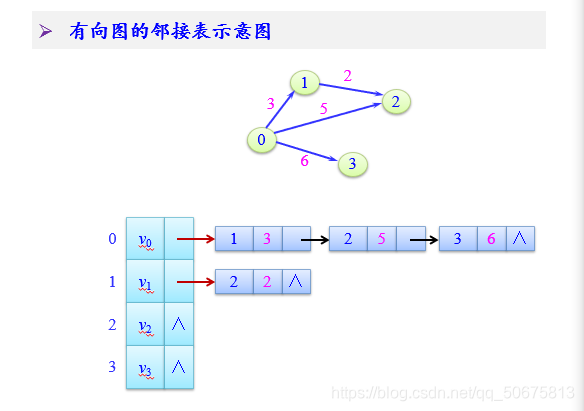
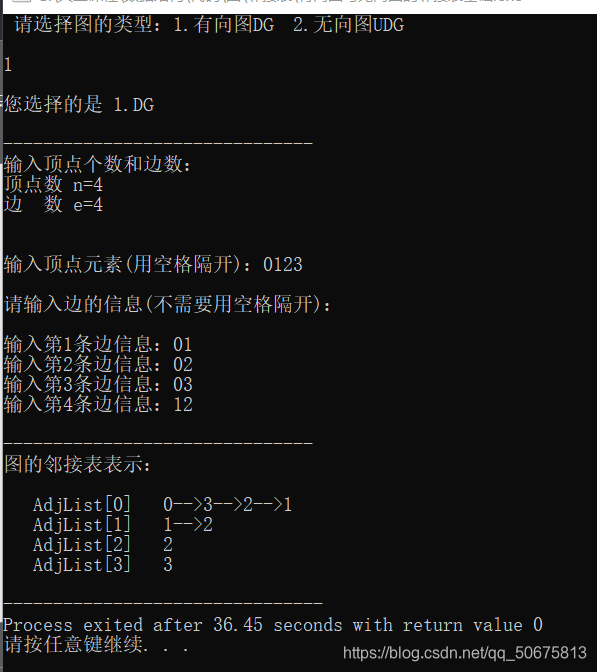
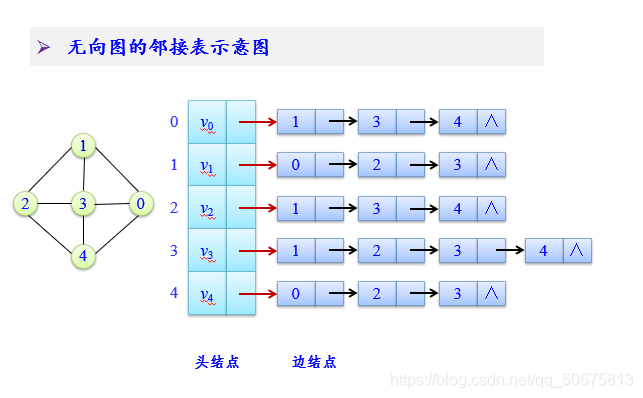
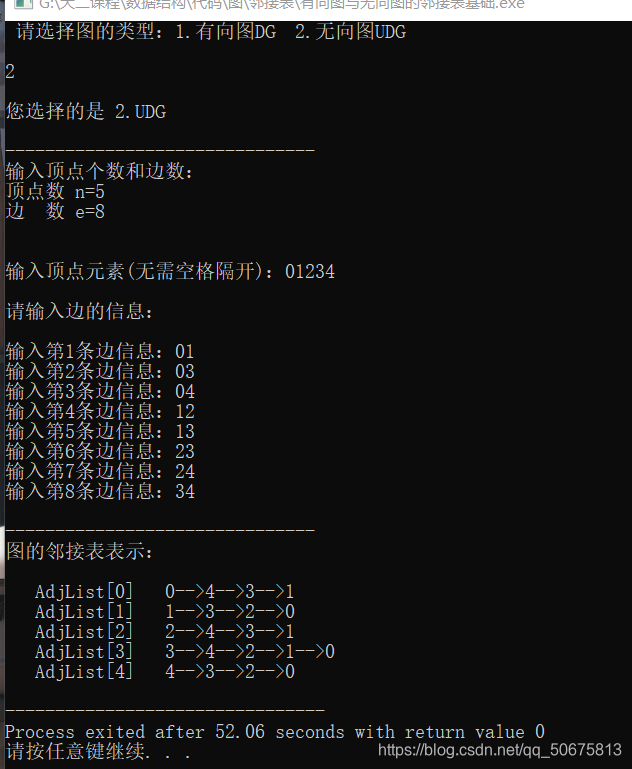
#include <stdio.h>
#include <stdlib.h>
#define VertexType char
#define VertexMax 20 typedef struct ArcNode
{int adjvex;struct ArcNode *next;
}ArcNode;typedef struct VNode
{VertexType vertex;struct ArcNode *firstarc;
}VNode;typedef struct
{VNode AdjList[VertexMax];int vexnum,arcnum; int kind;
}ALGraph;int LocateVex(ALGraph *G,VertexType v)
{ int i;for(i=0;i<G->vexnum;i++){if(v==G->AdjList[i].vertex){return i;}}printf("No Such Vertex!\n");return -1;
}
void CreateDG(ALGraph *G)
{int i,j;printf("输入顶点个数和边数:\n");printf("顶点数 n="); scanf("%d",&G->vexnum);printf("边 数 e="); scanf("%d",&G->arcnum);printf("\n"); printf("\n");printf("输入顶点元素(用空格隔开):");for(i=0;i<G->vexnum;i++){scanf(" %c",&G->AdjList[i].vertex);G->AdjList[i].firstarc=NULL;} printf("\n");int n,m;VertexType v1,v2;ArcNode *p1,*p2; printf("请输入边的信息(不需要用空格隔开):\n\n"); for(i=0;i<G->arcnum;i++){ printf("输入第%d条边信息:",i+1); scanf(" %c%c",&v1,&v2);n=LocateVex(G,v1);m=LocateVex(G,v2);if(n==-1||m==-1){printf("NO This Vertex!\n");return;} p1=(ArcNode *)malloc(sizeof(ArcNode));p1->adjvex=m;p1->next=G->AdjList[n].firstarc;G->AdjList[n].firstarc=p1;}G->kind=1;
}
void CreateUDG(ALGraph *G)
{int i,j;printf("输入顶点个数和边数:\n");printf("顶点数 n="); scanf("%d",&G->vexnum);printf("边 数 e="); scanf("%d",&G->arcnum);printf("\n"); printf("\n");printf("输入顶点元素(无需空格隔开):");for(i=0;i<G->vexnum;i++){scanf(" %c",&G->AdjList[i].vertex);G->AdjList[i].firstarc=NULL;} printf("\n");int n,m;VertexType v1,v2;ArcNode *p1,*p2; printf("请输入边的信息:\n\n"); for(i=0;i<G->arcnum;i++){ printf("输入第%d条边信息:",i+1); scanf(" %c%c",&v1,&v2);n=LocateVex(G,v1);m=LocateVex(G,v2);if(n==-1||m==-1){printf("NO This Vertex!\n");return;} p1=(ArcNode *)malloc(sizeof(ArcNode));p1->adjvex=m;p1->next=G->AdjList[n].firstarc;G->AdjList[n].firstarc=p1;p2=(ArcNode *)malloc(sizeof(ArcNode));p2->adjvex=n;p2->next=G->AdjList[m].firstarc;G->AdjList[m].firstarc=p2;}G->kind=2;
} void print(ALGraph G)
{int i;ArcNode *p;printf("\n-------------------------------");printf("\n图的邻接表表示:\n");for(i=0;i<G.vexnum;i++){printf("\n AdjList[%d]%4c",i,G.AdjList[i].vertex);p=G.AdjList[i].firstarc;while(p!=NULL){printf("-->%d",p->adjvex);p=p->next;}} printf("\n");
} void GraphChoice(ALGraph *G)
{int target;printf(" 请选择图的类型:1.有向图DG 2.无向图UDG\n\n");scanf("%d",&target);printf("\n");switch (target) {case 1:printf("您选择的是 1.DG\n\n"); printf("-------------------------------\n");CreateDG(G);break;case 2:printf("您选择的是 2.UDG\n\n"); printf("-------------------------------\n");CreateUDG(G);break;default:printf("无效选择!!!请重新选择!\n\n"); printf("-------------------------------\n");GraphChoice(G); break;}
}int main()
{ALGraph G;GraphChoice(&G);print(G);return 0;
}