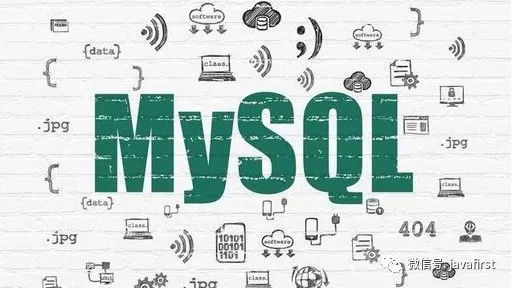
在刷了上百道sql题后,发现所有的题目都是基于某一个或几个知识点来做考察的,所以理清基础的知识细节,才能在题目考察到任意知识点时,找到解决线索。
温故而知新,学习在于总结,于是我再次对已经学习过的mysql的知识进行梳理,得到基础和进阶两篇知识框架的总结。如果对你有帮助,建议点赞收藏!
本文是MySQL基础知识的总结,主要涵盖增、删、改、查四个方面
内容大纲:
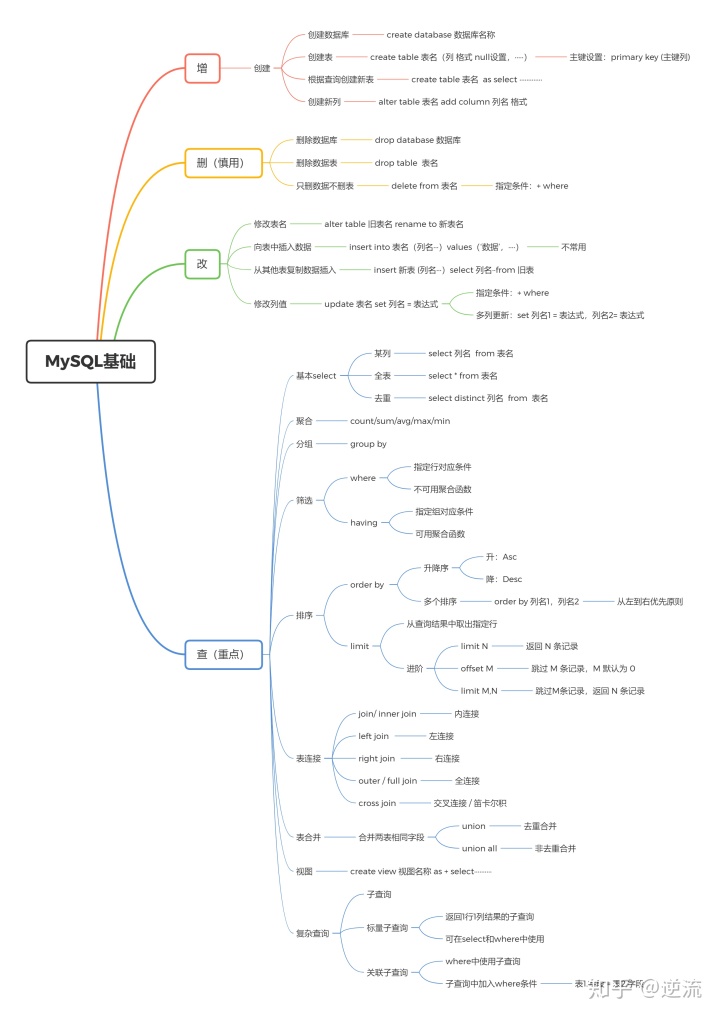
一、增
- 创建数据库:create database 数据库名称
create database taobao;
- 创建表:create table 表名(列 格式 null设置,·····),主键设置:primary key (主键列)
CREATE TABLE ProductIns(
product_id char(4) NOT NULL,
product_name VARCHAR(100) NOT NULL,
product_type VARCHAR(32) NOT NULL,
sale_price INTEGER DEFAULT 0,
purchase_price INTEGER ,
regist_date DATE,
PRIMARY KEY (product_id));
- 根据查询创建新表:create table 表名 as select ·············
create table user_lc AS select count(DISTINCT user_id) as "第一天新增用户数"
from userbehavior
where dates = '2017-11-25';
- 创建新列:alter table 表名 add column 列名 格式
alter table lc_copy add column ListingId varchar(20);
二、删(慎用)
- 删除数据库:drop database 数据库名称
drop database school;
- 删除数据表:drop table 表名
drop table product;
- 只删数据不删表:delete from 表名(指定条件:+ where)
delete from product
where sale_price >=400
三、改
- 修改表名:alter table 旧表名 rename to 新表名
alter table score rename to score2;
- 向表中插入数据:insert into 表名(列名···)values(‘数据’,····)
INSERT INTO productins(id,name,type)
VALUES('001','T恤衫','衣服');
- 从其他表复制数据插入:insert 新表 (列名···)select 列名··from 旧表
insert into producttype(pr_type,sum_sale,sum_purchase)
select pr_type,sum(sale),sum(purchase)
from product
group by type;
- 修改列值:update 表名 set 列名 = 表达式
指定条件:+ where
多列更新:set 列名1 = 表达式,列名2= 表达式
update product set regist_date ='2009-10-10'
where product_id ='008';
四、查(重点)
基本select
- 某列:select 列名 from 表名
select id from score;
- 全表:select * from 表名
select * from score;
- 去重:select distinct 列名 from 表名
--查询不同ID
select distinct id from score;--统计不同ID个数
select count(distinct id) from score;--优化写法
select count(*) from
(select distinct id from score) as a
聚合
- count/sum/avg/max/min
-- 统计最大/最小/平均薪水
select max(salary), min(salary),avg(salary)
from employee
分组
- group by
--查询每个班平均成绩
select avg(score) from student
group by class;
筛选
- where:指定行对应条件,不可用聚合函数
select score from student
where id ='001';
- having:指定组对应条件,可用聚合函数
select avg(score) from student
group by class
having avg(score)>80;
排序
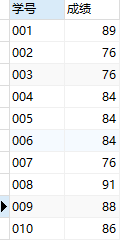
- order by
升序:Asc 降序:Desc
多个排序:order by 列名1,列名2(从左到右优先原则)
SELECT 学号,成绩 from score2
ORDER BY 成绩 Desc;
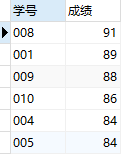
- limit:从查询结果中取出指定行
进阶:
limit N:返回 N 条记录
offset M:跳过 M 条记录,M 默认为 0
limit M,N:跳过M条记录,返回 N 条记录
SELECT 学号,成绩 from score2
ORDER BY 成绩 Desc
LIMIT 1,2;
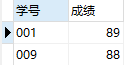
表连接(重点)
两张学生表:
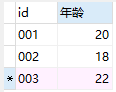
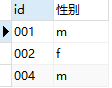
join/ inner join:内连接,左表右表都匹配的记录
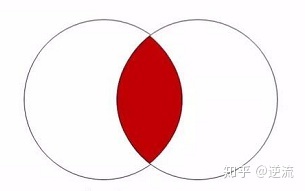
SELECT a.id,年龄,性别
from student1 a join student2 b
on a.id=b.id;
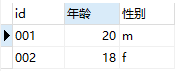
left join :左连接,以左表位置准,向右表匹配对应字段,有多条则逐次列出,无匹配,则显示NULL。
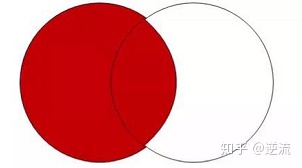
SELECT a.id,年龄,性别
from student1 a LEFT JOIN student2 b
on a.id=b.id;
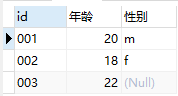
right join :右连接,以右表位置准,向左表匹配对应字段,有多条则逐次列出,无匹配,则显示NULL。
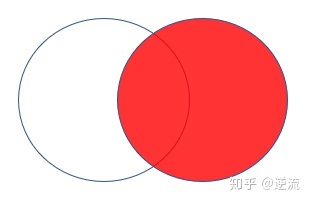
SELECT b.id,年龄,性别
from student1 a RIGHT JOIN student2 b
on a.id=b.id;
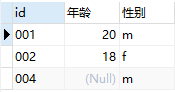
outer / full join:全连接,包含两个表全部连接结果,左、右表缺失的数据会显示为NULL。
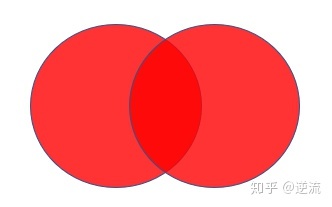
cross join:交叉连接 / 笛卡尔积,生成3X3结果的表格
SELECT a.id,年龄,性别
from student1 a CROSS JOIN student2 b;
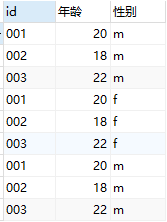
表合并
合并两表相同字段:
- union:去重合并
- union all:非去重合并
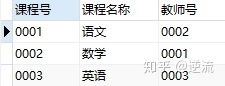
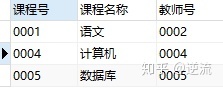
-- 去重合并
select * from course
UNION
SELECT * from course2;
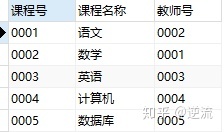
-- 非去重合并
select * from course
UNION ALL
SELECT * from course2;
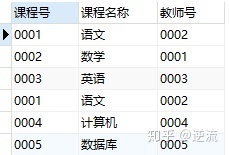
视图
视图是不保存实际数据的临时表,相当于直接调用由select语句查询结果,在实际工作中,视图可以减少写sql语句时的嵌套。
create view 视图名称 as + select··········
示例:如下图,创建一个名为“用户行为”的临时表
create view 用户行为 as
select user_id,count(behavior)as 用户行为总数,
sum(if(behavior = 'pv',1,0))as 点击量,
sum(if(behavior = 'fav',1,0))as 收藏数,
sum(if(behavior = 'cart',1,0))as 加购数,
sum(if(behavior = 'buy',1,0))as 购买量
from userbehavior
group by user_id;
后续可以直接写sql语句调用该表
select
sum(点击量) as "总点击量",
sum(收藏数) as "总收藏数",
sum(加购数) as "总加购数",
sum(购买量) as "总购买量"
from 用户行为;
复杂查询
- 子查询:也就是常说的嵌套,即将定义视图的select语句直接用于from子句中。子查询层数无上限。
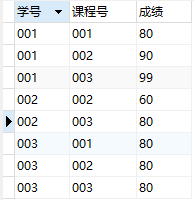
select 学号
from (select * from score GROUP BY 学号)as a

- 标量子查询:返回1行1列结果的子查询(可在select和where中使用)
-- 查询高于平均成绩的学号及对应信息
select *
from score
where 成绩>(select avg(成绩) from score);
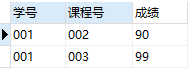
- 关联子查询:where中使用子查询
SELECT 学号,课程号,成绩
from score as s1
WHERE 成绩>(
SELECT avg(成绩)
FROM score as s2
-- 关联条件,按课程号来对表分组,同一组的数据,与这一组的平均成绩进行比较
WHERE s1.课程号 = s2.课程号
GROUP BY 课程号);