文章目录
- 引言
- 准备工作
- 前置条件
- 代码实现与解析
- 导入必要的库
- 初始化Pygame
- 定义星系类
- 主循环
- 完整代码
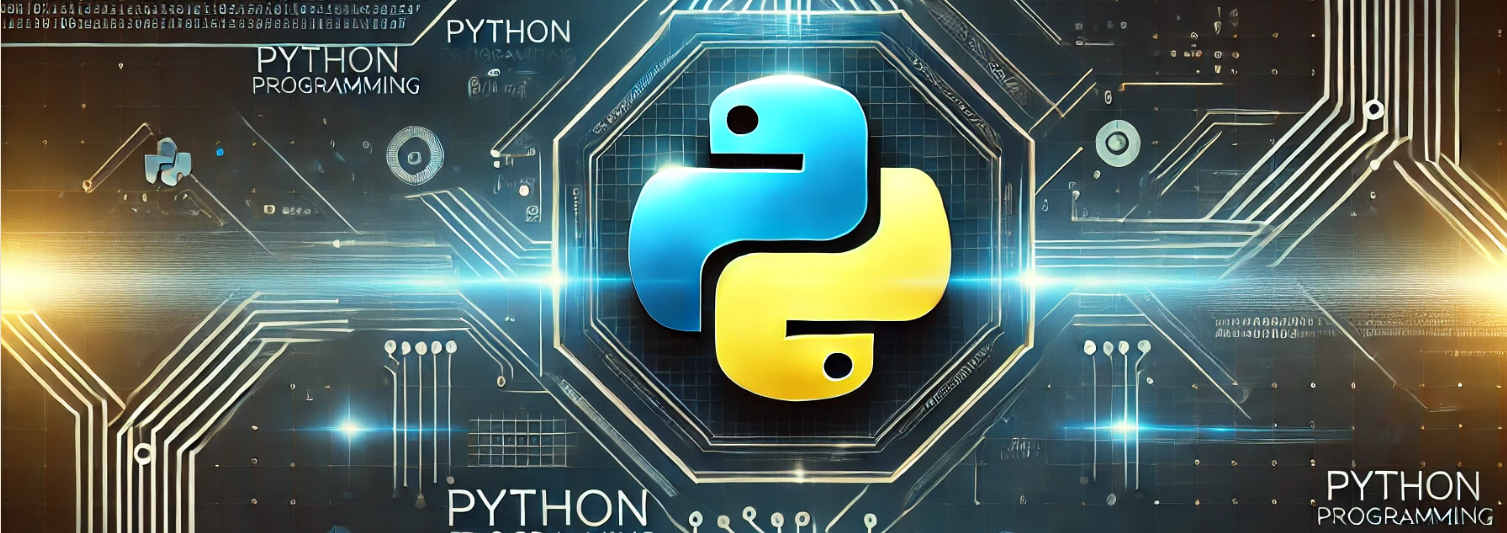
引言
银河系的旋转动画是一个迷人且富有挑战性的项目。通过模拟星系的旋转,我们可以更好地理解天文学现象,并创造出视觉上令人惊叹的效果。在这篇博客中,我们将使用Python创建一个动态旋转的银河系动画。通过利用Pygame库,我们可以实现这个美丽的视觉效果。
准备工作
前置条件
在开始之前,你需要确保你的系统已经安装了Pygame库。如果你还没有安装它,可以使用以下命令进行安装:
pip install pygame
Pygame是一个跨平台的Python模块,用于编写视频游戏。它包括计算机图形和声音库,使得游戏开发更加简单。
代码实现与解析
导入必要的库
我们首先需要导入Pygame库和其他必要的模块:
import pygame
import random
import math
初始化Pygame
我们需要初始化Pygame并设置屏幕的基本参数:
pygame.init()
screen = pygame.display.set_mode((800, 800))
pygame.display.set_caption("旋转的银河动画")
clock = pygame.time.Clock()
定义星系类
我们创建一个Galaxy
类来定义星系的属性和行为:
class Galaxy:def __init__(self, num_stars):self.num_stars = num_starsself.stars = []self.center = (400, 400)self.generate_stars()def generate_stars(self):for _ in range(self.num_stars):angle = random.uniform(0, 2 * math.pi)distance = random.uniform(50, 350)x = self.center[0] + distance * math.cos(angle)y = self.center[1] + distance * math.sin(angle)speed = random.uniform(0.001, 0.01)self.stars.append([x, y, angle, distance, speed])def update(self):for star in self.stars:star[2] += star[4]star[0] = self.center[0] + star[3] * math.cos(star[2])star[1] = self.center[1] + star[3] * math.sin(star[2])def draw(self, screen):for star in self.stars:pygame.draw.circle(screen, (255, 255, 255), (int(star[0]), int(star[1])), 2)
主循环
我们在主循环中更新星系的旋转状态并绘制:
galaxy = Galaxy(500)running = True
while running:for event in pygame.event.get():if event.type == pygame.QUIT:running = Falsescreen.fill((0, 0, 0))galaxy.update()galaxy.draw(screen)pygame.display.flip()clock.tick(60)pygame.quit()
完整代码
import pygame
import random
import math# 初始化Pygame
pygame.init()
screen = pygame.display.set_mode((800, 800))
pygame.display.set_caption("旋转的银河动画")
clock = pygame.time.Clock()# 星系类定义
class Galaxy:def __init__(self, num_stars):self.num_stars = num_starsself.stars = []self.center = (400, 400)self.generate_stars()def generate_stars(self):for _ in range(self.num_stars):angle = random.uniform(0, 2 * math.pi)distance = random.uniform(50, 350)x = self.center[0] + distance * math.cos(angle)y = self.center[1] + distance * math.sin(angle)speed = random.uniform(0.001, 0.01)self.stars.append([x, y, angle, distance, speed])def update(self):for star in self.stars:star[2] += star[4]star[0] = self.center[0] + star[3] * math.cos(star[2])star[1] = self.center[1] + star[3] * math.sin(star[2])def draw(self, screen):for star in self.stars:pygame.draw.circle(screen, (255, 255, 255), (int(star[0]), int(star[1])), 2)# 主循环
galaxy = Galaxy(500)running = True
while running:for event in pygame.event.get():if event.type == pygame.QUIT:running = Falsescreen.fill((0, 0, 0))galaxy.update()galaxy.draw(screen)pygame.display.flip()clock.tick(60)pygame.quit()