206. 反转链表
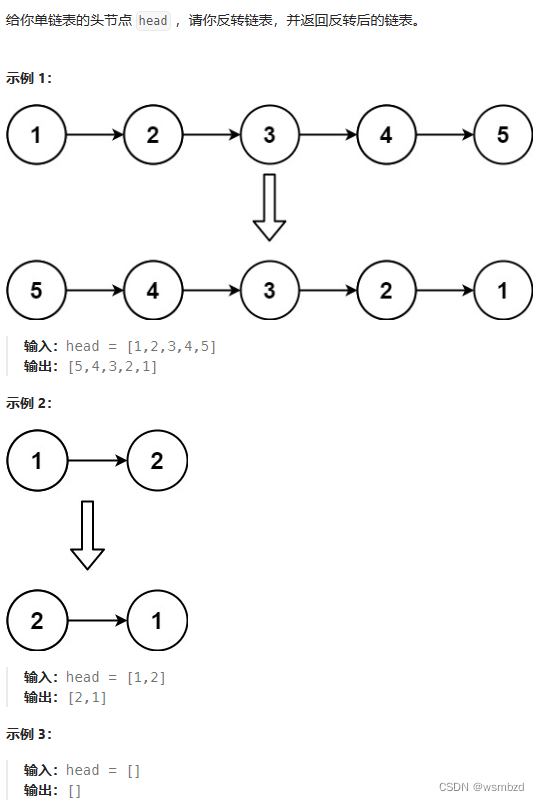
class Solution {
public:ListNode* reverseList(ListNode* head) {if(!head || !head -> next){return head;}ListNode *newhead = reverseList(head -> next);head -> next -> next = head;head -> next = nullptr;return newhead;}
};
234. 回文链表
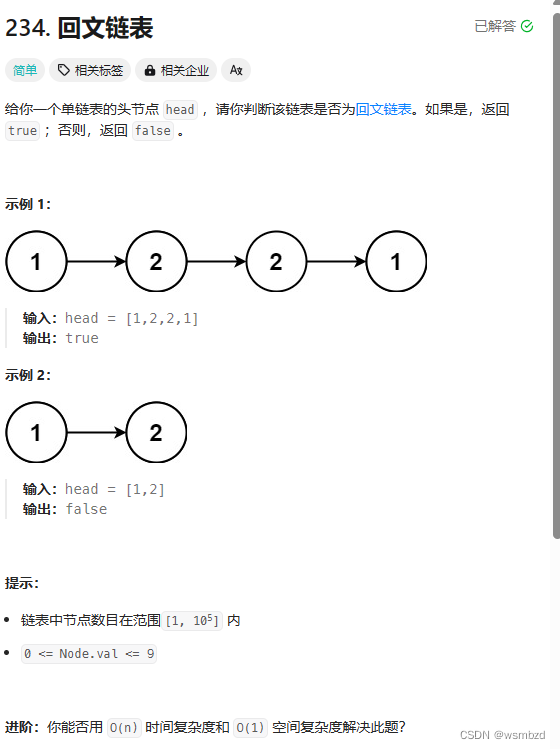
1、快慢指针+翻转(可以实现进阶的要求,也就是说O(1)空间复杂度)
class Solution {
public:bool isPalindrome(ListNode* head) {if(!head || !head->next) return 1;ListNode *fast = head, *slow = head;ListNode *p,*pre = NULL;while(fast && fast->next){p = slow;slow = slow -> next;fast = fast -> next ->next;p -> next = pre;pre = p;}if(fast)slow = slow -> next;while(p){if(p->val != slow->val)return 0;p = p -> next;slow = slow -> next;}return 1;}
};
2、栈
stack<int> s; ListNode *p = head;while(p){s.push(p -> val);p = p -> next;}p = head;while(p){if(p -> val != s.top()){return 0;}s.pop();p = p -> next;}return 1;