Redux快速上手、三个核心概念、React组件使用、修改store的数据、提交action传参、异步操作、Redux调试
- 一、Redux快速上手
- 1.概念
- 2.快速体验(纯redux计数案例)
- 二、Redux与React-环境准备
- 1.配套工具
- 2.配置基础环境
- 3.store目录结构设计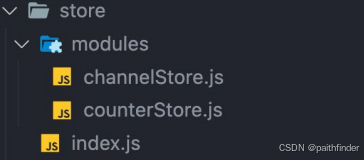
- 三、Reduc与React-实现counter
- 1.使用React Toolkit 创建 counterStore
- 2.为React注入store
- 3.React组件使用store中的数据
- 3.React组件修改store中的数据
- 四、提交action传参实现需求
- 五、异步状态操作
- 1. 创建store的写法保持不变,配置好同步修改状态的方法
- 2. 单独封装一个函数,在函数内部return一个新函数,在新函数中
- 3. 组件中dispatch的写法保持不变
- 六、Redux调试 - devtools
- 七、美团外卖案例
- 1.分类和商品列表渲染
- 2.点击分类激活交互实现
- 3.商品列表切换显示
- 4.添加购物车实现
- 5.统计区域功能实现
- 6.购物车列表功能实现
- 7.控制购物车显示和隐藏
一、Redux快速上手
1.概念
Redux 是React最常用的集中状态管理工具,类似于Vue中的Pinia(Vuex),可以独立于框架运行
作用:通过集中管理的方式管理应用的状态
案例:使用纯Redux实现计数器
2.快速体验(纯redux计数案例)
<button id="decrement">-<button/>
<span id="count">0<span/>
<button id="increment">+<button/>
//1. 定义一个 reducer 函数 (根据当前想要做的修改返回一个新的状态)
function reducer(state={count:0},action){//数据不可变:基于原始状态生成一个新的状态if(action.type === 'INCREMENT'){return {count:state.count+1}}if(action.type==='DECREMENT'){return {count:state.count-1}}return state
//2. 使用createStore方法传入 reducer函数 生成一个store实例对象const store=Redux.createStore(reducer)
//3. 使用store实例的 subscribe方法 订阅数据的变化(数据一旦变化,可以得到通知)
//回调函数可以在每次state发生变化时自动执行store.subscribe(()=>{console.log("State变化了“,state.getState())document.getElementById('count').innerText=store.getState().count})
//4. 使用store实例的 dispatch方法提交action对象 触发数据变化(告诉reducer你想怎么改数据)
const inBtn = document.getElementById('increment')
inBtn.addEventListener('click',()=>{//增store.dispatch({type:"INCREMENT"})
})
const inBtn = document.getElementById('dncrement')
inBtn.addEventListener('click',()=>{//减store.dispatch({type:"DECREMENT"})
})
//5. 使用store实例的 getState方法 获取最新的状态数据更新到视图中
3.三个核心概念
为了职责清晰,数据流向明确,Redux把整个数据修改的流程分成了三个核心概念,分别是:state、action和reducer
state-一个对象 存放着我们管理的数据状态
action - 一个对象 用来描述你想怎么改数据
reducer - 一个函数 根据action的描述生成一个新的state
二、Redux与React-环境准备
1.配套工具
Redux Toolkit(RTK)- 官方推荐编写Redux逻辑的方式,是一套工具的集合集,简化书写方式
react-redux - 用来 链接 Redux 和 React组件 的中间件
2.配置基础环境
(1). 使用 CRA 快速创建 React 项目
npx create-react-app react-redux
(2). 安装配套工具
npm i @reduxjs/toolkit react-redux
(3). 启动项目
npm run start
3.store目录结构设计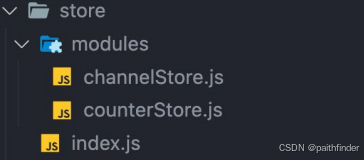
通常集中状态管理的部分都会单独创建一个单独的 store
目录
应用通常会有很多个子store模块,所以创建一个 modules
目录,在内部编写业务分类的子store
store中的入口文件 index.js 的作用是组合modules中所有的子模块,并导出store
三、Reduc与React-实现counter
1.使用React Toolkit 创建 counterStore
子模块的创建:
// store/modules/counterStore.js
import {createSlice} from "@reduxjs/toolkit"
const counterStore=createSlice({name:"counter",//初始化stateinitialState:{count:0},//修改状态的方法,同步方法,支持直接修改reducer:{increment(state){state.count++},decrement(state){state.count--}}
})
//解构出来actionCreater函数
const {increment,decrement}=counterStore.actions
//获取reducer
const reducer =counterStore.reducer
//以按需导出的方式导出actionCreater
export {increment,decrement}
//z以默认导出的方式导出reducer
export default reducer
子模块的整合:
// store/index.js
import {configureStore} from "@reduxjs/toolkit"
//导入子模块reducer
import counterReducer from "./modules/counterStore"
const store = configureStore({reducer:{counter:counterReducer}
})
export default store
2.为React注入store
react-redux负责把Redux和React 链接 起来,内置 Provider组件 通过 store 参数把创建好的store实例注入到应用中,链接正式建立
index.js:
3.React组件使用store中的数据
在React组件中使用store中的数据,需要用到一个 钩子函数 - useSelector,它的作用是把store中的数据映射到组件
在app.js组件中使用数据:
//App.js
import {useSelector}from 'react-redux'
function App(){const {count}=useSelector(state=>state.counter)return (<div className="App">{count}<div/>)
}
3.React组件修改store中的数据
React组件中修改store中的数据需要借助另外一个hook函数 - useDispatch,它的作用是生成提交action对象的dispatch函数
//App.js
import {useDispatch,useSelector}from 'react-redux'
//导入actionCreater
import {increment,decrement}from './store/moduls/counterStore'
function App(){const {count}=useSelector(state=>state.counter)const dispatch=useDispatch()return (<div className="App"><button onClick={()=>dispatch(decrement())}>-</button>{count}<button onClick={()=>dispatch(decrement())}>+</button><div/>)
}
四、提交action传参实现需求
在reducers的同步修改方法中添加action对象参数,在调用actionCreater的时候传递参数,参数会被传递到action对象payload属性上
在reducers的同步修改方法中添加action对象参数,在调用actionCreater的时候传递参数,参数会被传递到action对象payload属性上
五、异步状态操作
1. 创建store的写法保持不变,配置好同步修改状态的方法
2. 单独封装一个函数,在函数内部return一个新函数,在新函数中
封装异步请求获取数据
调用同步actionCreater传入异步数据生成一个action对象,并使用dispatch提交
3. 组件中dispatch的写法保持不变
六、Redux调试 - devtools
Redux官方提供了针对于Redux的调试工具,支持实时state信息展示,action提交信息查看等
七、美团外卖案例
1.分类和商品列表渲染
启动项目(mock服务 + 前端服务 ):npm run serve && npm run start
使用RTK编写store(异步action)
组件触发action并且渲染数据
App.js:
2.点击分类激活交互实现
使用RTK编写管理activeIndex
组件中点击时触发action更改activeIndex
动态控制激活类名显示
3.商品列表切换显示
条件渲染:控制对应项显示
activeIndex === index && 视图
4.添加购物车实现
使用RTK管理新状态cartList
思路:如果添加过,只更新数量count,没有添加过,直接push进去
组件中点击时收集数据提交action添加购物车
5.统计区域功能实现
基于store中的cartList的length渲染数量
基于store中的cartList累加price * count
购物车cartList的length不为零则高亮
6.购物车列表功能实现
使用cartList遍历渲染列表
RTK中增加增减reducer,组件中提交action
RTK中增加清除购物车reducer,组件中提交action
……
7.控制购物车显示和隐藏
使用useState声明控制显隐的状态
点击统计区域设置状态为true
……
点击蒙层区域设置状态为false
……