问题描述
小M面对一组从 1 到 9 的数字,这些数字被分成多个小组,并从每个小组中选择一个数字组成一个新的数。目标是使得这个新数的各位数字之和为偶数。任务是计算出有多少种不同的分组和选择方法可以达到这一目标。
numbers
: 一个由多个整数字符串组成的列表,每个字符串可以视为一个数字组。小M需要从每个数字组中选择一个数字。
例如对于[123, 456, 789]
,14个符合条件的数为:147 149 158 167 169 248 257 259 268 347 349 358 367 369
。
#include <iostream>
#include <vector>
#include<string>
int solution(std::vector<int> numbers) {// Please write your code herelong long evenCount = 0; // 记录偶数选择的组合数 long long oddCount = 0; // 记录奇数选择的组合数 // 初始情况下,没有任何选择,所以组合数为0evenCount = 1; // 初始化为1,因为至少有一种选择(即不选择任何数字)for (int num : numbers) {int even = 0, odd = 0;std::string strNum = std::to_string(num);// 统计当前数字组中的偶数和奇数 for (char ch : strNum) {if ((ch - '0') % 2 == 0) {even++;}else {odd++;}}// 更新组合数 long long newEvenCount = evenCount * even + oddCount * odd; // 偶数和偶数的组合 + 奇数和奇数的组合long long newOddCount = evenCount * odd + oddCount * even; // 偶数和奇数的组合evenCount = newEvenCount;oddCount = newOddCount;}// 返回符合条件的组合数(偶数和的组合) return evenCount; // 返回偶数和的组合数//return -1;
}int main() {// You can add more test cases herestd::cout << (solution({123, 456, 789}) == 14) << std::endl;std::cout << (solution({123456789}) == 4) << std::endl;std::cout << (solution({14329, 7568}) == 10) << std::endl;return 0;
}
后记:才反应过来,字节青训入营题目前只能交python和Java,难怪上面 显示我一题也没刷,要细心,学习别拖延,截至日期快到了——
def solution(numbers):even_count = 0 # 记录偶数选择的组合数 odd_count = 0 # 记录奇数选择的组合数 # 初始情况下,没有任何选择,所以组合数为0even_count = 1 # 初始化为1,因为至少有一种选择(即不选择任何数字)for num in numbers:even = 0odd = 0str_num = str(num)# 统计当前数字组中的偶数和奇数 for ch in str_num:if int(ch) % 2 == 0:even += 1else:odd += 1# 更新组合数 new_even_count = even_count * even + odd_count * odd # 偶数和偶数的组合 + 奇数和奇数的组合new_odd_count = even_count * odd + odd_count * even # 偶数和奇数的组合even_count = new_even_countodd_count = new_odd_count# 返回符合条件的组合数(偶数和的组合) return even_count # 返回偶数和的组合数# 测试用例
if __name__ == "__main__":print(solution([123, 456, 789]) == 14) # 应输出 Trueprint(solution([123456789]) == 4) # 应输出 Trueprint(solution([14329, 7568]) == 10) # 应输出 True
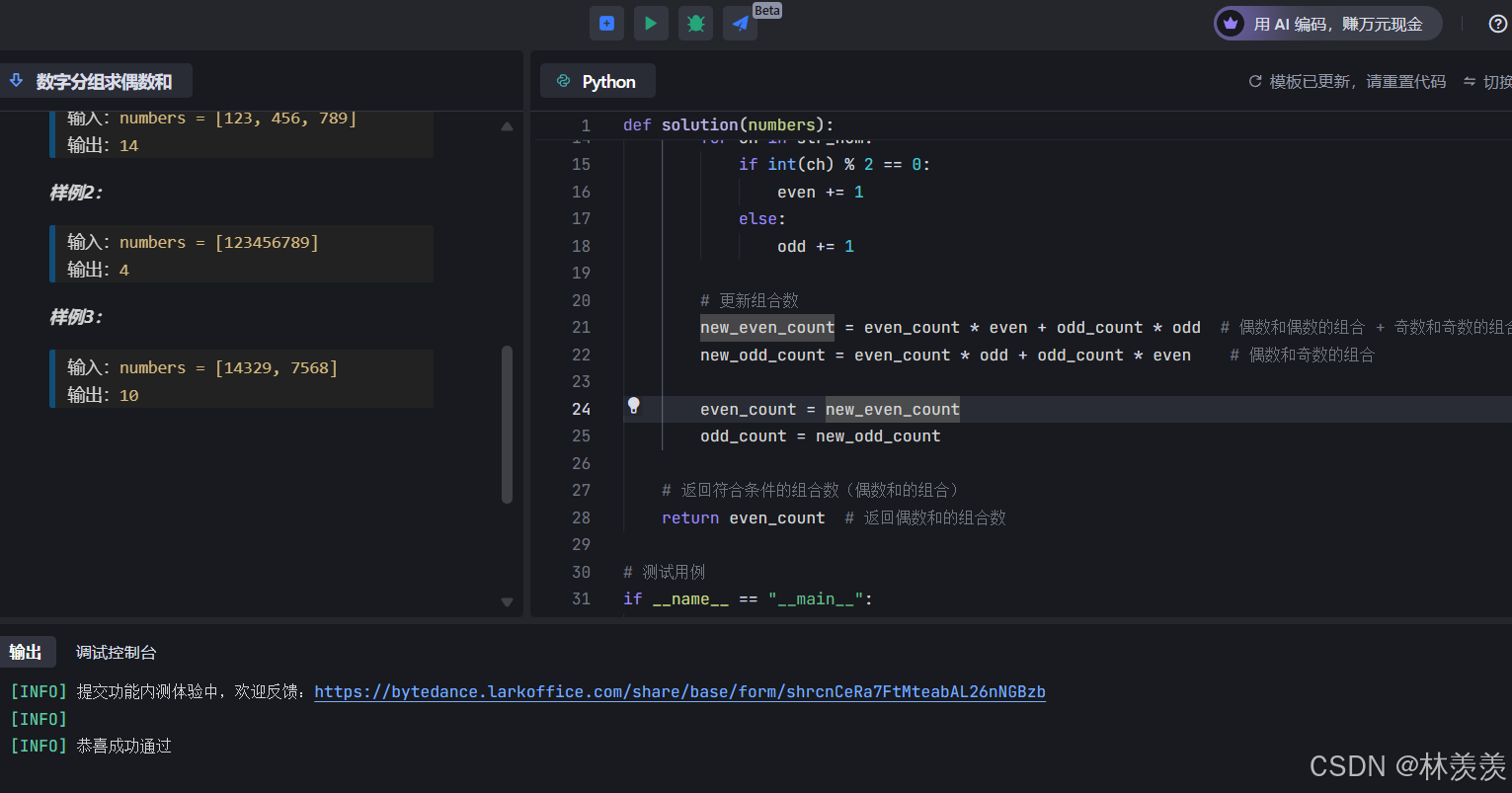