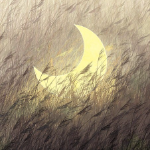
🌈个人主页:人不走空
💖系列专栏:算法专题
⏰诗词歌赋:斯是陋室,惟吾德馨
在Android开发中,控件(也称为视图或控件组件)是构建用户界面的基本元素。它们用于显示信息和与用户进行交互。控件可以是简单的按钮或文本框,也可以是复杂的列表或图表。《第一行代码——Android》这本书涵盖了大量的控件知识,帮助开发者创建丰富和响应式的用户界面。下面,我们将详细讲解一些常用的Android控件及其用法。
目录
🌈个人主页:人不走空
💖系列专栏:算法专题
⏰诗词歌赋:斯是陋室,惟吾德馨
常用控件
1. TextView
属性
示例
2. EditText
属性
示例
3. Button
属性
示例
4. ImageView
属性
示例
5. CheckBox
属性
示例
6. RadioButton 和 RadioGroup
属性
示例
7. Switch
属性
示例
8. ProgressBar
属性
示例
9. SeekBar
属性
示例
10. ListView
使用步骤
示例
11. RecyclerView
使用步骤
示例
12. WebView
属性
示例
自定义控件
创建自定义控件的基本步骤
示例
总结
作者其他作品:
常用控件
1. TextView
TextView
是一个用于显示文本的控件。它可以用来显示短句、段落或者标题。
属性
android:text
: 设置显示的文本。android:textSize
: 设置文本的大小。android:textColor
: 设置文本的颜色。android:gravity
: 设置文本的对齐方式(如居中、左对齐、右对齐等)。
示例
xml
复制代码
<TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello, Android!" android:textSize="18sp" android:textColor="#000000" android:gravity="center"/>
2. EditText
EditText
是一个可编辑的文本控件,用于接收用户输入。它通常用于表单、搜索框等需要用户输入文本的地方。
属性
android:hint
: 设置提示文本,在用户输入前显示。android:inputType
: 设置输入类型(如文本、数字、密码等)。android:text
: 设置初始的输入文本。
示例
xml
复制代码
<EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Enter your name" android:inputType="text"/>
3. Button
Button
是一个点击按钮控件,用于触发特定的操作或事件。
属性
android:text
: 设置按钮上的文本。android:onClick
: 设置按钮点击时触发的事件处理方法。
示例
xml
复制代码
<Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Click Me" android:onClick="onButtonClick"/>
在Activity中实现点击事件:
kotlin
复制代码
fun onButtonClick(view: View) { // 处理按钮点击事件 }
4. ImageView
ImageView
用于显示图片。它支持加载和显示各种格式的图片资源。
属性
android:src
: 设置显示的图片资源。android:scaleType
: 设置图片的缩放类型(如适应、裁剪、填充等)。
示例
xml
复制代码
<ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/sample_image" android:scaleType="centerCrop"/>
5. CheckBox
CheckBox
是一个复选框控件,允许用户在多项选择中进行选择。
属性
android:text
: 设置复选框旁边的文本。android:checked
: 设置复选框的初始状态(是否选中)。
示例
xml
复制代码
<CheckBox android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="I agree" android:checked="false"/>
6. RadioButton 和 RadioGroup
RadioButton
是单选按钮,通常与 RadioGroup
一起使用,形成一组选项,用户只能选择其中一个。
属性
android:text
: 设置单选按钮旁边的文本。android:checked
: 设置单选按钮的初始状态。
示例
xml
复制代码
<RadioGroup android:layout_width="wrap_content" android:layout_height="wrap_content"> <RadioButton android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Option 1"/> <RadioButton android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Option 2"/> </RadioGroup>
7. Switch
Switch
是一个切换开关控件,用于在开和关之间切换状态。
属性
android:textOn
: 设置开状态时的文本。android:textOff
: 设置关状态时的文本。android:checked
: 设置开关的初始状态。
示例
xml
复制代码
<Switch android:layout_width="wrap_content" android:layout_height="wrap_content" android:textOn="On" android:textOff="Off" android:checked="true"/>
8. ProgressBar
ProgressBar
是一个进度条控件,用于显示任务的进度。
属性
android:indeterminate
: 设置进度条是否为不确定模式(即加载中,不显示具体进度)。android:max
: 设置进度条的最大值。android:progress
: 设置当前进度。
示例
xml
复制代码
<ProgressBar android:layout_width="wrap_content" android:layout_height="wrap_content" android:indeterminate="true"/>
9. SeekBar
SeekBar
是一个滑动条控件,允许用户在指定范围内选择一个值。
属性
android:max
: 设置滑动条的最大值。android:progress
: 设置当前选定的值。
示例
xml
复制代码
<SeekBar android:layout_width="match_parent" android:layout_height="wrap_content" android:max="100" android:progress="50"/>
10. ListView
ListView
是一个用于显示滚动列表的控件,每个列表项可以是一个自定义的视图。
使用步骤
- 定义布局: 创建一个包含
ListView
的布局。 - 准备数据: 准备一个数据源(如数组或列表)。
- 创建适配器: 使用适配器将数据绑定到
ListView
。 - 设置适配器: 将适配器设置到
ListView
上。
示例
xml
复制代码
<ListView android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/listView"/>
在 Activity
中使用 ListView
:
kotlin
复制代码
val listView: ListView = findViewById(R.id.listView) val items = arrayOf("Item 1", "Item 2", "Item 3") val adapter = ArrayAdapter(this, android.R.layout.simple_list_item_1, items) listView.adapter = adapter
11. RecyclerView
RecyclerView
是一个更灵活和高效的列表控件,可以替代 ListView
。它支持布局管理器和视图持有者(ViewHolder)模式。
使用步骤
- 定义布局: 创建一个包含
RecyclerView
的布局。 - 创建适配器: 实现
RecyclerView.Adapter
,定义数据和视图的绑定逻辑。 - 设置布局管理器: 选择适当的布局管理器(如
LinearLayoutManager
或GridLayoutManager
)。 - 设置适配器: 将适配器设置到
RecyclerView
上。
示例
xml
复制代码
<androidx.recyclerview.widget.RecyclerView android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/recyclerView"/>
在 Activity
中使用 RecyclerView
:
kotlin
复制代码
val recyclerView: RecyclerView = findViewById(R.id.recyclerView) recyclerView.layoutManager = LinearLayoutManager(this) recyclerView.adapter = MyAdapter(myItemList)
12. WebView
WebView
是一个可以加载和显示网页内容的控件。它支持显示HTML内容、执行JavaScript代码,并与网页进行交互。
属性
android:layout_width
: 设置WebView的宽度。android:layout_height
: 设置WebView的高度。android:id
: 设置WebView的唯一标识符。
示例
xml
复制代码
<WebView android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/webView"/>
在 Activity
中加载网页内容:
kotlin
复制代码
val webView: WebView = findViewById(R.id.webView) webView.loadUrl("https://www.example.com")
自定义控件
除了使用内置控件,Android还允许开发者创建自定义控件,以满足特定的需求。自定义控件可以继承已有的控件类(如 View
或 ViewGroup
),然后重写绘制方法和事件处理方法。
创建自定义控件的基本步骤
- 继承已有控件: 创建一个类,继承自
View
或ViewGroup
。 - 重写绘制方法: 在
onDraw
方法中定义控件的绘制逻辑。 - 处理事件: 在
onTouchEvent
方法中处理用户的交互事件。 - 添加自定义属性: 在
res/values
文件夹中定义自定义属性,并在控件中解析这些属性。
示例
创建一个简单的自定义圆形控件:
kotlin
复制代码
class CircleView(context: Context, attrs: AttributeSet?) : View(context, attrs) { private val paint: Paint = Paint() override fun onDraw(canvas: Canvas?) { super.onDraw(canvas) canvas?.let { paint.color = Color.BLUE val radius = min(width, height) / 2.0f it.drawCircle(width / 2.0f, height / 2.0f, radius, paint) } } }
在布局文件中使用自定义控件:
xml
复制代码
<com.example.myapp.CircleView android:layout_width="100dp" android:layout_height="100dp"/>
总结
掌握Android中的各种控件及其用法是创建丰富用户界面的基础。通过合理地使用这些控件,可以开发出功能强大且用户体验良好的应用程序。希望这篇博客能够帮助你更好地理解和使用Android的控件,如果有任何问题或想法,欢迎在评论区讨论!
作者其他作品:
【Java】Spring循环依赖:原因与解决方法
OpenAI Sora来了,视频生成领域的GPT-4时代来了
[Java·算法·简单] LeetCode 14. 最长公共前缀 详细解读
【Java】深入理解Java中的static关键字
[Java·算法·简单] LeetCode 28. 找出字a符串中第一个匹配项的下标 详细解读
了解 Java 中的 AtomicInteger 类
算法题 — 整数转二进制,查找其中1的数量
深入理解MySQL事务特性:保证数据完整性与一致性
Java企业应用软件系统架构演变史