一、 什么是make&Makefile ?
①make 是一条命令,makefile是一个文件,配合使用,通过依赖关系和依赖方法达到我们形成可执行程序的目的
②makefile好处就是可以进行 自动化编译 ” ,极大的提高软件开发的效率,一旦写好,只需要一个 make 命令,整个工程即可以完全自动编译③make 是一个命令工具,是一个解释 makefile 中指令的命令工具
二、 实例代码
0x01 Makefile的编辑
[wh@localhost lesson7]$ touch test.c
[wh@localhost lesson7]$ touch Makefile //Makefile建议开头大写
[wh@localhost lesson7]$ vim test.c
[wh@localhost lesson7]$ vim Makefile
[wh@localhost lesson7]$ make
gcc test.c -o test -std=c99
[wh@localhost lesson7]$ ll
total 20
-rw-rw-r--. 1 wh wh 41 Oct 5 05:54 Makefile
-rwxrwxr-x. 1 wh wh 8512 Oct 5 05:54 test
-rw-rw-r--. 1 wh wh 73 Oct 5 05:54 test.c
[wh@localhost lesson7]$ ./test
hello world
[wh@localhost lesson7]$ cat Makefile
test:test.c //test的形成依赖于test.c,也可以说目标文件依赖于依赖文件gcc test.c -o test -std=c99 //依赖方法
上述代码是对一个项目的形成,当然我们也要对其进行清理:
[wh@localhost lesson7]$ cat Makefile
test:test.cgcc test.c -o test -std=c99.PHONY:clean
clean:rm -f test
.PHONY是对clean的一个修饰,可以说clean是一个伪目标,而clean只有依赖方法,没有依赖关系,并且目标可执行程序如果没有修改,不需要重复执行,而伪目标可以多次执行
0x02 上述代码中为什么只输入make就形成了可执行程序test,而必须输入make clean才能进行清理呢?
make扫描makefile文件的时候,默认只会形成一个目标依赖关系,一般就是第一个
当然也可以用$@代替目标文件,$^代替源文件
1 test:test.c2 //gcc test.c -o test -std=c99 3 gcc -o $@ $^ 4 .PHONY:clean5 clean:6 rm -f test
上述的test:test.c依赖关系也可以细分为:
1 test:test.o2 gcc test.o -o test 3 test.o:test.s4 gcc -c test.s -o test.o5 test.s:test.i6 gcc -S test.i -o test.s7 test.i:test.c8 gcc -E test.c -o test.i9 .PHONY:clean10 clean:11 rm -f test test.i test.s test.o
0x03 printf在sleep之前,为什么先进行6s之后,printf才输出呢?难道是sleep先于printf运行吗?
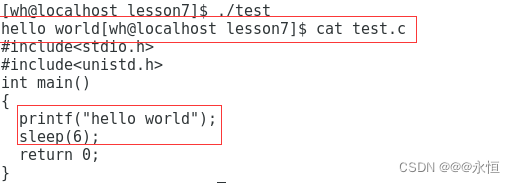
当然不是,是因为printf已经执行,但是数据没有被立即刷新到显示器当中
①没有\n,字符串会暂时保存起来,保存在用户C语言级别的缓冲区中
②显示器设备刷新策略就是行刷新,遇到\n即进行刷新
③如果想要立马刷新,则可以使用fflush(stdout);因为printf打印向stdout打印,则刷新即是向stdout刷新
C程序,默认会打开三个流:
stdin对应键盘,stdout对应显示器,stderr对应显示器
因为程序的运行需要数据的输入,结果的输出以及错误的输出
三、Linux 第一个小程序 --进度条
0x01 初步学习
1 #include<stdio.h>2 #include<unistd.h>3 4 int main()5 {6 int count = 10; 7 while(count)8 {9 printf("%d\r",count);10 fflush(stdout);11 count--;12 sleep(1);13 }14 return 0;15 }
① 此时,显示器中显示的是10,90,80,70,...,10,这是为什么呢?
凡是从键盘读取的内容都是字符和显示到显示器上面的内容都是字符,比如pirntf("%d\n",234);每次显示的是字符2,字符3,字符4,每次%r,都是只重置到首字母,但是不换行,fflush刷新的都是一个字符
②那如何解决这个问题呢?
可以预留一部分空间出来,printf("%2d\r");留出来俩个空间
0x02 进一步编写
1 #include<stdio.h>2 #include<string.h>3 #include<unistd.h>4 5 int main()6 {7 #define NUM 1008 char bar[NUM+1];//预留出'\0'的位置9 memset(bar,'\0',sizeof(bar));10 11 int i = 0;12 while(i <= 100)13 {14 printf("%s\r",bar);15 fflush(stdout);16 bar[i] = '#';17 i++;18 sleep(1);19 }20 }
0x03 增加[ ]和百分比和运行光标
1 #include<stdio.h>2 #include<string.h>3 #include<unistd.h>4 5 int main()6 {7 #define NUM 1008 char bar[NUM+1];9 memset(bar,'\0',sizeof(bar));10 const char* label = '|/-\\'11 int i = 0;12 while(i <= 100)13 {14 printf("[%-100s][%d%%] %c\r",bar,i,label[i%4]);//预留100个空间,%是转义字符,%%表示一个%//因为默认格式控制是右对齐,所以是从右往左打印,加'-'则是左对齐,从左往右打印15 fflush(stdout);16 bar[i] = '#';17 i++;18 usleep(50000);19 }20 printf("\n"); 21 }