Question JavaScript实现继承的方式?
包含原型链继承、构造函数继承、组合继承、原型式继承、寄生式继承、寄生组合式继承和ES6 类继承
JavaScript实现继承的方式
在JavaScript中,实现继承的方式多种多样,每种方式都有其优势和适用场景。以下是一些常见的继承方式:
1. 原型链继承
原型链继承是通过将子类的原型设置为父类的实例来实现继承。这样,子类就可以访问父类原型上的属性和方法。
function Parent() {
this.name = 'Parent';
}
function Child() {}
Child.prototype = new Parent();
const childInstance = new Child();
console.log(childInstance.name); // 输出 'Parent'
优势: 简单易懂。
缺点: 引用类型的属性会被所有实例共享,无法传递参数给父类构造函数。
2. 构造函数继承
构造函数继承通过在子类构造函数中调用父类构造函数来实现继承。
function Parent(name) {
this.name = name || 'Parent';
}
function Child(name) {
Parent.call(this, name);
}
const childInstance = new Child('Child');
console.log(childInstance.name); // 输出 'Child'
优势: 解决了原型链继承中引用类型属性共享的问题。
缺点: 方法都在构造函数中定义,无法实现函数复用。
3. 组合继承
组合继承结合了原型链继承和构造函数继承,通过调用父类构造函数设置实例属性,再通过将父类实例作为子类原型来实现。
function Parent(name) {
this.name = name || 'Parent';
}
function Child(name) {
Parent.call(this, name);
}
Child.prototype = new Parent();
const childInstance = new Child('Child');
console.log(childInstance.name); // 输出 'Child'
优势: 同时继承实例属性和方法。
缺点: 调用了两次父类构造函数,存在一定的性能问题。
4. 原型式继承
原型式继承通过创建一个空对象,然后将该对象作为参数传递给一个函数,该函数的原型被赋值为这个对象,从而实现继承。
function createObject(obj) {
function F() {}
F.prototype = obj;
return new F();
}
const parent = {
name: 'Parent'
};
const child = createObject(parent);
console.log(child.name); // 输出 'Parent'
优势: 简单灵活。
缺点: 属性共享问题,引用类型属性会被所有实例共享。
5. 寄生式继承
寄生式继承在原型式继承的基础上,增加了对父类构造函数的调用,从而可以传递参数给父类构造函数。
function createObject(obj) {
const clone = Object.create(obj);
clone.sayHello = function() {
console.log('Hello!');
};
return clone;
}
const parent = {
name: 'Parent'
};
const child = createObject(parent);
console.log(child.name); // 输出 'Parent'
child.sayHello(); // 输出 'Hello!'
优势: 可以在对象上添加新的方法。
缺点: 仍然存在属性共享问题。
6. 寄生组合式继承
寄生组合式继承是为了解决组合继承中调用两次父类构造函数的性能问题,通过使用 Object.create
创建父类原型的副本,然后将该副本赋值给子类原型。
function inheritPrototype(child, parent) {
const prototype = Object.create(parent.prototype);
prototype.constructor = child;
child.prototype = prototype;
}
function Parent(name) {
this.name = name || 'Parent';
}
function Child(name) {
Parent.call(this, name);
}
inheritPrototype(Child, Parent);
const childInstance = new Child('Child');
console.log(childInstance.name); // 输出 'Child'
优势: 解决了组合继承的性能问题,同时保持了原型链继承的优点。
缺点: 略显繁琐。
7. ES6 类继承
ES6 引入了 class
关键字,使得面向对象编程更加直观。通过 extends
关键字可以实现类的继承。
class Parent {
constructor(name) {
this.name = name || 'Parent';
}
}
class Child extends Parent {
constructor(name) {
super(name);
}
}
const childInstance = new Child('Child');
console.log(childInstance.name); // 输出 'Child'
优势: 语法更加简洁,易读易写。
缺点: 底层仍然是基于原型链的继承。
100+小程序源码关注公众号回复 5 获取(不想看激励视频的可私信)
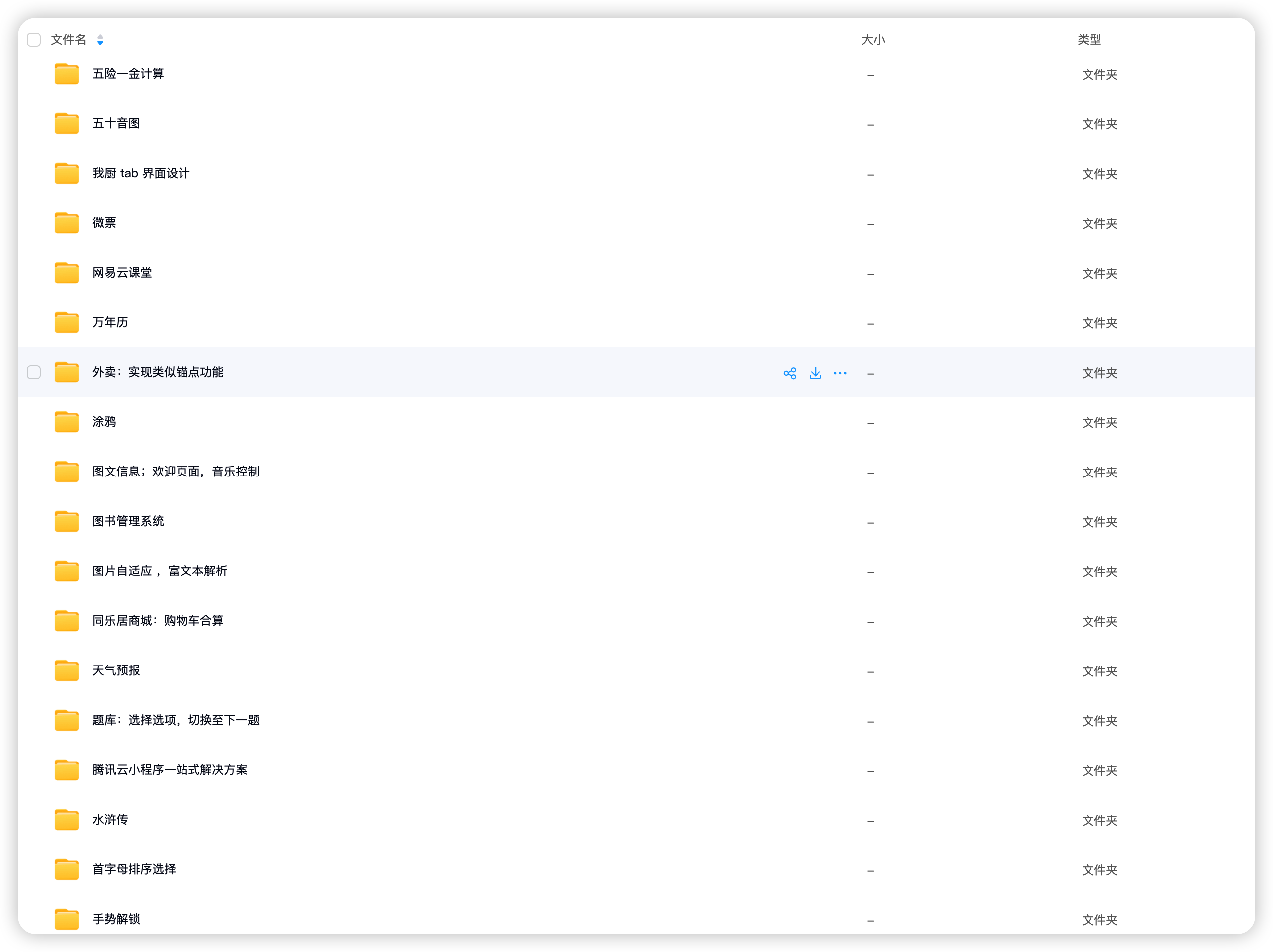
本文由 mdnice 多平台发布