JDBC技术
通过JDBC连接MySQL数据库
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8" import = "java.sql.*"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<%try {// 加载数据库驱动,注册到驱动管理器Class.forName("com.mysql.cj.jdbc.Driver");// 数据库连接字符串String url = "jdbc:mysql://localhost:3306";// 数据库用户名String username = "root";// 数据库密码String password = "98";// 创建Connection连接Connection conn = DriverManager.getConnection(url,username,password);// 判断 数据库连接是否为空if(conn != null){// 输出连接信息out.println("数据库连接成功!");// 关闭数据库连接conn.close();}else{// 输出连接信息out.println("数据库连接失败!");}} catch (ClassNotFoundException e) {e.printStackTrace();} catch (SQLException e) {e.printStackTrace();}
%></body>
</html>
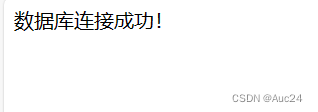
JDBC操作数据库
创建Web项目,通过JDBC实现图书信息添加功能
package com.lyq.bean;
public class Book {// 编号private int id;// 图书名称private String name;// 价格private double price;// 数量private int bookCount;// 作者private String author;public int getId() {return id;}public void setId(int id) {this.id = id;}public String getName() {return name;}public void setName(String name) {this.name = name;}public double getPrice() {return price;}public void setPrice(double price) {this.price = price;}public int getBookCount() {return bookCount;}public void setBookCount(int bookCount) {this.bookCount = bookCount;}public String getAuthor() {return author;}public void setAuthor(String author) {this.author = author;}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>添加图书信息</title>
<script type="text/javascript">function check(form){with(form){if(name.value == ""){alert("图书名称不能为空");return false;}if(price.value == ""){alert("价格不能为空");return false;}if(author.value == ""){alert("作者不能为空");return false;}return true;}}
</script>
</head>
<body><form action="AddBook.jsp" method="post" onsubmit="return check(this);"><table align="center" width="450"><tr><td align="center" colspan="2"><h2>添加图书信息</h2><hr></td></tr><tr><td align="right">图书名称:</td><td><input type="text" name="name" /></td></tr><tr><td align="right">价 格:</td><td><input type="text" name="price" /></td></tr><tr><td align="right">数 量:</td><td><input type="text" name="bookCount" /></td></tr><tr><td align="right">作 者:</td><td><input type="text" name="author" /></td></tr><tr><td align="center" colspan="2"><input type="submit" value="添 加"></td></tr></table></form>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"><%@page import="java.sql.Connection"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.PreparedStatement"%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>添加结果</title>
</head>
<body><jsp:useBean id="book" class="com.lyq.bean.Book"></jsp:useBean><jsp:setProperty property="*" name="book"/><%try {// 加载数据库驱动,注册到驱动管理器Class.forName("com.mysql.jdbc.Driver");// 数据库连接字符串String url = "jdbc:mysql://localhost:3306/db_database10";// 数据库用户名String username = "root";// 数据库密码String password = "98661376";// 创建Connection连接Connection conn = DriverManager.getConnection(url,username,password);// 添加图书信息的SQL语句String sql = "insert into tb_books(name,price,bookCount,author) values(?,?,?,?)";// 获取PreparedStatementPreparedStatement ps = conn.prepareStatement(sql);// 对SQL语句中的第1个参数赋值ps.setString(1, book.getName());System.out.println("name:"+book.getName());// 对SQL语句中的第2个参数赋值ps.setDouble(2, book.getPrice());// 对SQL语句中的第3个参数赋值ps.setInt(3,book.getBookCount());// 对SQL语句中的第4个参数赋值ps.setString(4, book.getAuthor());// 执行更新操作,返回所影响的行数int row = ps.executeUpdate();// 判断是否更新成功if(row > 0){// 更新成输出信息out.print("成功添加了 " + row + "条数据!");}// 关闭PreparedStatement,释放资源ps.close();// 关闭Connection,释放资源conn.close();} catch (Exception e) {out.print("图书信息添加失败!");e.printStackTrace();}%><br>返回
</body>
</html>
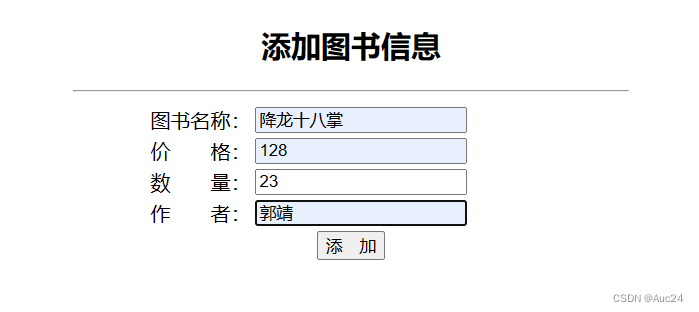
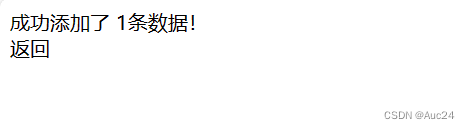
通过JDBC查询图书信息表中的图书信息数据,并显示在页面中
package com.lyq.bean;import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;public class FindServlet extends HttpServlet {private static final long serialVersionUID = 1L;protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {try {// 加载数据库驱动,注册到驱动管理器Class.forName("com.mysql.cj.jdbc.Driver");// 数据库连接字符串String url = "jdbc:mysql://localhost:3306";// 数据库用户名String username = "root";// 数据库密码String password = "986";// 创建Connection连接Connection conn = DriverManager.getConnection(url,username,password);// 获取StatementStatement stmt = conn.createStatement();// 添加图书信息的SQL语句String sql = "select * from db_database10.tb_books";// 执行查询ResultSet rs = stmt.executeQuery(sql);// 实例化List对象List<Book> list = new ArrayList<Book>();// 判断光标向后移动,并判断是否有效while(rs.next()){// 实例化Book对象Book book = new Book();// 对id属性赋值book.setId(rs.getInt("id"));// 对name属性赋值book.setName(rs.getString("name"));// 对price属性赋值book.setPrice(rs.getDouble("price"));// 对bookCount属性赋值book.setBookCount(rs.getInt("bookCount"));// 对author属性赋值book.setAuthor(rs.getString("author"));// 将图书对象添加到集合中list.add(book);}// 将图书集合放置到request之中request.setAttribute("list", list);rs.close(); // 关闭ResultSetstmt.close(); // 关闭Statementconn.close(); // 关闭Connection} catch (ClassNotFoundException e) {e.printStackTrace();} catch (SQLException e) {e.printStackTrace();}// 请求转发到book_list.jsprequest.getRequestDispatcher("book_list.jsp").forward(request, response);}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"><%@page import="java.util.List"%>
<%@page import="com.lyq.bean.Book"%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>所有图书信息</title>
<style type="text/css">td{font-size: 12px;}h2{margin: 0px}
</style>
</head>
<body><table align="center" width="450" border="1" height="180" bordercolor="white" bgcolor="black" cellpadding="1" cellspacing="1"><tr bgcolor="white"><td align="center" colspan="5"><h2>所有图书信息</h2></td></tr><tr align="center" bgcolor="#e1ffc1" ><td><b>ID</b></td><td><b>图书名称</b></td><td><b>价格</b></td><td><b>数量</b></td><td><b>作者</b></td></tr><%// 获取图书信息集合List<Book> list = (List<Book>)request.getAttribute("list");// 判断集合是否有效if(list == null || list.size() < 1){out.print("没有数据!");}else{// 遍历图书集合中的数据for(Book book : list){%><tr align="center" bgcolor="white"><td><%=book.getId()%></td><td><%=book.getName()%></td><td><%=book.getPrice()%></td><td><%=book.getBookCount()%></td><td><%=book.getAuthor()%></td></tr><%}}%></table>
</body>
</html>
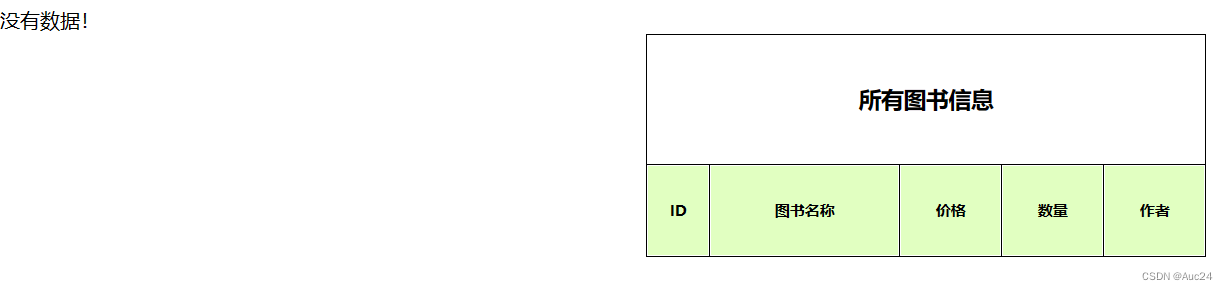
在查询所有图书信息的页面中,添加修改图书数量表单
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"><%@page import="java.util.List"%>
<%@page import="com.lyq.Book"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>所有图书信息</title><style type="text/css">form{margin: 0px;}td{font-size: 12px;}h2{margin: 2px}</style><script type="text/javascript">function check(form){with(form){if(bookCount.value == ""){alert("请输入更新数量!");return false;}if(isNaN(bookCount.value)){alert("格式错误!");return false;}return true;;}}</script>
</head>
<body>
<table align="center" width="500" border="1" height="170" bordercolor="white" bgcolor="black" cellpadding="1" cellspacing="1"><tr bgcolor="white"><td align="center" colspan="6"><h2>所有图书信息</h2></td></tr><tr align="center" bgcolor="#e1ffc1" ><td><b>ID</b></td><td><b>图书名称</b></td><td><b>价格</b></td><td><b>数量</b></td><td><b>作者</b></td><td><b>修改数量</b></td></tr><%// 获取图书信息集合List<Book> list = (List<Book>)request.getAttribute("list");// 判断集合是否有效if(list == null || list.size() < 1){out.print("没有数据!");}else{// 遍历图书集合中的数据for(Book book : list){%><tr align="center" bgcolor="white"><td><%=book.getId()%></td><td><%=book.getName()%></td><td><%=book.getPrice()%></td><td><%=book.getBookCount()%></td><td><%=book.getAuthor()%></td><td><form action="UpdateServlet" method="post" onsubmit="return check(this);"><input type="hidden" name="id" value="<%=book.getId()%>"><input type="text" name="bookCount" size="3"><input type="submit" value="修 改"></form></td></tr><%}}%>
</table>
</body>
</html>
package com.lyq;import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;/*** Servlet implementation class UpdateServlet*/
public class UpdateServlet extends HttpServlet {private static final long serialVersionUID = 1L;protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {int id = Integer.valueOf(request.getParameter("id"));int bookCount = Integer.valueOf(request.getParameter("bookCount"));try {// 加载数据库驱动,注册到驱动管理器Class.forName("com.mysql.jdbc.Driver");// 数据库连接字符串String url = "jdbc:mysql://localhost:3306/db_database10";// 数据库用户名String username = "root";// 数据库密码String password = "986";// 创建Connection连接Connection conn = DriverManager.getConnection(url,username,password);// 更新SQL语句String sql = "update tb_books set bookcount=? where id=?";// 获取PreparedStatementPreparedStatement ps = conn.prepareStatement(sql);// 对SQL语句中的第一个参数赋值ps.setInt(1, bookCount);// 对SQL语句中的第二个参数赋值ps.setInt(2, id);// 执行更新操作ps.executeUpdate();// 关闭PreparedStatementps.close();// 关闭Connectionconn.close();} catch (Exception e) {e.printStackTrace();}// 重定向到FindServletresponse.sendRedirect("FindServlet");}}
在查询所有图书信息页面中,添加删除图书信息的超链接,通过Servlet实现对数据的删除操作
package com.lyq;import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;/*** Servlet implementation class DeleteServlet*/
public class DeleteServlet extends HttpServlet {private static final long serialVersionUID = 1L;protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {// 获取图书idint id = Integer.valueOf(request.getParameter("id"));try {// 加载数据库驱动,注册到驱动管理器Class.forName("com.mysql.jdbc.Driver");// 数据库连接字符串String url = "jdbc:mysql://localhost:3306/db_database10";// 数据库用户名String username = "root";// 数据库密码String password = "986";// 创建Connection连接Connection conn = DriverManager.getConnection(url,username,password);// 删除图书信息的SQL语句String sql = "delete from tb_books where id=?";// 获取PreparedStatementPreparedStatement ps = conn.prepareStatement(sql);// 对SQL语句中的第一个占位符赋值ps.setInt(1, id);// 执行更新操作ps.executeUpdate();// 关闭PreparedStatementps.close();// 关闭Connectionconn.close();} catch (Exception e) {e.printStackTrace();}// 重定向到FindServletresponse.sendRedirect("FindServlet");}
}
通过JDBC的批处理操作,一次性将多个学生写入数据库中
<%@ page language="java" contentType="text/html; charset=GB18030"pageEncoding="GB18030"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=GB18030"><title>首页</title>
</head>
<body>
<jsp:useBean id="batch" class="com.lyq.Batch"></jsp:useBean>
<%// 执行批量插入操作int row = batch.saveBatch();out.print("批量插入了【" + row + "】条数据!");
%>
</body>
</html>
package com.lyq;import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Random;public class Batch {/*** 获取数据库连接* @return Connection对象*/public Connection getConnection(){// 数据库连接Connection conn = null;try {// 加载数据库驱动,注册到驱动管理器Class.forName("com.mysql.jdbc.Driver");// 数据库连接字符串String url = "jdbc:mysql://localhost:3306";// 数据库用户名String username = "root";// 数据库密码String password = "986";// 创建Connection连接conn = DriverManager.getConnection(url,username,password);} catch (ClassNotFoundException e) {e.printStackTrace();} catch (SQLException e) {e.printStackTrace();}// 返回数据库连接return conn;}/*** 批量添加数据* @return 所影响的行数*/public int saveBatch(){// 行数int row = 0 ;// 获取数据库连接Connection conn = getConnection();try {// 插入数据的SQL语句String sql = "insert into db_database10.tb_students_batch(name,sex,age) values(?,?,?)";// 创建PreparedStatementPreparedStatement ps = conn.prepareStatement(sql);// 实例化RandomRandom random = new Random();// 循环添加数据for (int i = 0; i < 10; i++) {// 对SQL语句中的第1个参数赋值ps.setString(1, "学生" + i);// 对SQL语句中的第2个参数赋值ps.setBoolean(2, i % 2 == 0 ? true : false);// 对SQL语句中的第3个参数赋值ps.setInt(3, random.nextInt(5) + 10);// 添加批处理命令ps.addBatch();}// 执行批处理操作并返回计数组成的数组int[] rows = ps.executeBatch();// 对行数赋值row = rows.length;// 关闭PreparedStatementps.close();// 关闭Connectionconn.close();} catch (Exception e) {e.printStackTrace();}// 返回添加的行数return row;}
}
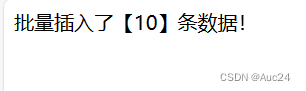
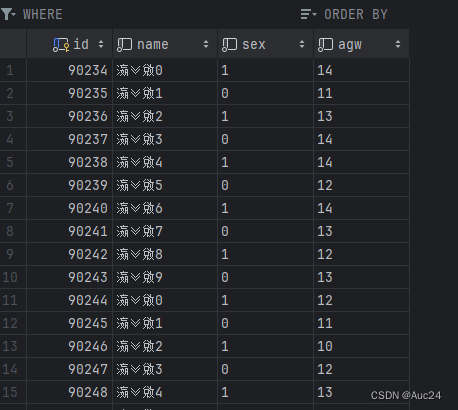
创建查询所有图书信息的存储过程,通过JDBC API对其调用获取所有图书信息,并将其输出到JSP页面中
<%@ page language="java" contentType="text/html; charset=GB18030"pageEncoding="GB18030"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"><%@page import="java.util.List"%>
<%@page import="com.lyq.Book"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=GB18030"><title>所有图书信息</title><style type="text/css">td{font-size: 12px;}h2{margin: 0px}</style>
</head>
<body>
<jsp:useBean id="findBook" class="com.lyq.FindBook"></jsp:useBean>
<table align="center" width="450" border="1" height="180" bordercolor="white" bgcolor="black" cellpadding="1" cellspacing="1"><tr bgcolor="white"><td align="center" colspan="5"><h2>所有图书信息</h2></td></tr><tr align="center" bgcolor="#e1ffc1" ><td><b>ID</b></td><td><b>图书名称</b></td><td><b>价格</b></td><td><b>数量</b></td><td><b>作者</b></td></tr><%// 获取图书信息集合List<Book> list = findBook.findAll();// 判断集合是否有效if(list == null || list.size() < 1){out.print("没有数据!");}else{// 遍历图书集合中的数据for(Book book : list){%><tr align="center" bgcolor="white"><td><%=book.getId()%></td><td><%=book.getName()%></td><td><%=book.getPrice()%></td><td><%=book.getBookCount()%></td><td><%=book.getAuthor()%></td></tr><%}}%>
</table>
</body>
</html>
package com.lyq;public class Book {// 编号private int id;// 图书名称private String name;// 价格private double price;// 数量private int bookCount;// 作者private String author;public int getId() {return id;}public void setId(int id) {this.id = id;}public String getName() {return name;}public void setName(String name) {this.name = name;}public double getPrice() {return price;}public void setPrice(double price) {this.price = price;}public int getBookCount() {return bookCount;}public void setBookCount(int bookCount) {this.bookCount = bookCount;}public String getAuthor() {return author;}public void setAuthor(String author) {this.author = author;}
}
package com.lyq;import java.io.IOException;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.annotation.WebFilter;/*** Servlet Filter implementation class Char*/
@WebFilter("/*")
public class Char implements Filter {public Char() {}public void destroy() {}public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {request.setCharacterEncoding("UTF-8");response.setCharacterEncoding("UTF-8");chain.doFilter(request, response);}public void init(FilterConfig fConfig) throws ServletException {}
}
package com.lyq;import java.sql.CallableStatement;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;public class FindBook {/*** 获取数据库连接* @return Connection对象*/public Connection getConnection(){// 数据库连接Connection conn = null;try {// 加载数据库驱动,注册到驱动管理器Class.forName("com.mysql.jdbc.Driver");// 数据库连接字符串String url = "jdbc:mysql://localhost:3306/db_database10";// 数据库用户名String username = "root";// 数据库密码String password = "986";// 创建Connection连接conn = DriverManager.getConnection(url,username,password);} catch (ClassNotFoundException e) {e.printStackTrace();} catch (SQLException e) {e.printStackTrace();}// 返回数据库连接return conn;}/*** 通过存储过程查询数据* @return List<Book>*/public List<Book> findAll(){// 实例化List对象List<Book> list = new ArrayList<Book>();// 创建数据库连接Connection conn = getConnection();//调用存储过程try {CallableStatement cs = conn.prepareCall("{call findAllBook()}");// 执行查询操作,并获取结果集ResultSet rs = cs.executeQuery();System.out.println("sssssssssssss:"+(rs == null));// 判断光标向后移动,并判断是否有效while(rs.next()){// 实例化Book对象Book book = new Book();// 对id属性赋值book.setId(rs.getInt("id"));// 对name属性赋值book.setName(rs.getString("name"));// 对price属性赋值book.setPrice(rs.getDouble("price"));// 对bookCount属性赋值book.setBookCount(rs.getInt("bookCount"));// 对author属性赋值book.setAuthor(rs.getString("author"));System.out.println("ssssssss");// 将图书对象添加到集合中list.add(book);}conn.close();} catch (Exception e) {e.printStackTrace();}// 返回listreturn list;}
}
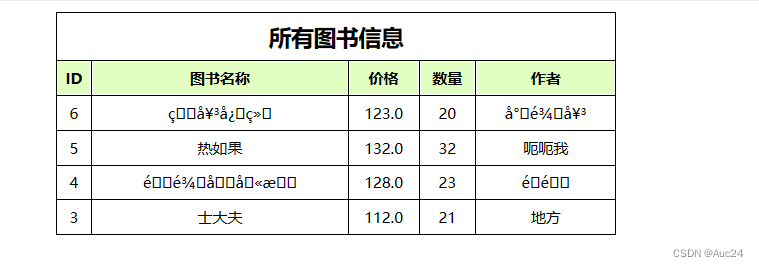
通过MySQL数据库提供的分页机制,实现商品信息的分页查询功能,将分页数据显示在JSP页面中
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>主页</title>
</head>
<body>
<a href="FindServlet">查看所有商品信息</a>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"><%@page import="java.util.List"%>
<%@page import="com.lyq.Product"%><html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>所有商品信息</title><style type="text/css">td{font-size: 12px;}h2{margin: 0px}</style>
</head>
<body>
<table align="center" width="450" border="1" height="180" bordercolor="white" bgcolor="black" cellpadding="1" cellspacing="1"><tr bgcolor="white"><td align="center" colspan="5"><h2>所有商品信息</h2></td></tr><tr align="center" bgcolor="#e1ffc1" ><td><b>ID</b></td><td><b>商品名称</b></td><td><b>价格</b></td><td><b>数量</b></td><td><b>单位</b></td></tr><%List<Product> list = (List<Product>)request.getAttribute("list");for(Product p : list){%><tr align="center" bgcolor="white"><td><%=p.getId()%></td><td><%=p.getName()%></td><td><%=p.getPrice()%></td><td><%=p.getNum()%></td><td><%=p.getUnit()%></td></tr><%}%><tr><td align="center" colspan="5" bgcolor="white"><%=request.getAttribute("bar")%></td></tr>
</table>
</body>
</html>
package com.lyq;import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;/*** 商品数据库操作* @author Li YongQiang**/public class BookDao {/*** 获取数据库连接* @return Connection对象*/public Connection getConnection(){// 数据库连接Connection conn = null;try {// 加载数据库驱动,注册到驱动管理器Class.forName("com.mysql.jdbc.Driver");// 数据库连接字符串String url = "jdbc:mysql://localhost:3306/db_database10";// 数据库用户名String username = "root";// 数据库密码String password = "98";// 创建Connection连接conn = DriverManager.getConnection(url,username,password);} catch (ClassNotFoundException e) {e.printStackTrace();} catch (SQLException e) {e.printStackTrace();}// 返回数据库连接return conn;}/*** 分页查询所有商品信息* @param page 页数* @return List<Product>*/public List<Product> find(int page){// 创建ListList<Product> list = new ArrayList<Product>();// 获取数据库连接Connection conn = getConnection();// 分页查询的SQL语句String sql = "select * from tb_product order by id desc limit ?,?";try {// 获取PreparedStatementPreparedStatement ps = conn.prepareStatement(sql);// 对SQL语句中的第1个参数赋值ps.setInt(1, (page - 1) * Product.PAGE_SIZE);// 对SQL语句中的第2个参数赋值ps.setInt(2, Product.PAGE_SIZE);// 执行查询操作ResultSet rs = ps.executeQuery();// 光标向后移动,并判断是否有效while(rs.next()){// 实例化ProductProduct p = new Product();// 对id属性赋值p.setId(rs.getInt("id"));// 对name属性赋值p.setName(rs.getString("name"));// 对num属性赋值p.setNum(rs.getInt("num"));// 对price属性赋值p.setPrice(rs.getDouble("price"));// 对unit属性赋值p.setUnit(rs.getString("unit"));// 将Product添加到List集合中list.add(p);}// 关闭ResultSetrs.close();// 关闭PreparedStatementps.close();// 关闭Connectionconn.close();} catch (SQLException e) {e.printStackTrace();}return list;}/*** 查询总记录数* @return 总记录数*/public int findCount(){// 总记录数int count = 0;// 获取数据库连接Connection conn = getConnection();// 查询总记录数SQL语句String sql = "select count(*) from tb_product";try {// 创建StatementStatement stmt = conn.createStatement();// 查询并获取ResultSetResultSet rs = stmt.executeQuery(sql);// 光标向后移动,并判断是否有效if(rs.next()){// 对总记录数赋值count = rs.getInt(1);}// 关闭ResultSetrs.close();// 关闭Connectionconn.close();} catch (SQLException e) {e.printStackTrace();}// 返回总记录数return count;}
}
package com.lyq;public class Product {public static final int PAGE_SIZE = 2;// 编号private int id;// 名称private String name;// 价格private double price;// 数量private int num;// 单位private String unit;public int getId() {return id;}public void setId(int id) {this.id = id;}public String getName() {return name;}public void setName(String name) {this.name = name;}public double getPrice() {return price;}public void setPrice(double price) {this.price = price;}public int getNum() {return num;}public void setNum(int num) {this.num = num;}public String getUnit() {return unit;}public void setUnit(String unit) {this.unit = unit;}
}
package com.lyq;import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.List;/*** Servlet implementation class FindServlet*/
public class FindServlet extends HttpServlet {private static final long serialVersionUID = 1L;protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {// 当前页码int currPage = 1;// 判断传递页码是否有效if(request.getParameter("page") != null){// 对当前页码赋值currPage = Integer.parseInt(request.getParameter("page"));}// 实例化ProductDaoBookDao dao = new BookDao();// 查询所有商品信息List<Product> list = dao.find(currPage);// 将list放置到request之中request.setAttribute("list", list);// 总页数int pages ;// 查询总记录数int count = dao.findCount();// 计算总页数if(count % Product.PAGE_SIZE == 0){// 对总页数赋值pages = count / Product.PAGE_SIZE;}else{// 对总页数赋值pages = count / Product.PAGE_SIZE + 1;}// 实例化StringBufferStringBuffer sb = new StringBuffer();// 通过循环构建分页条for(int i=1; i <= pages; i++){// 判断是否为当前页if(i == currPage){// 构建分页条sb.append("『" + i + "』");}else{// 构建分页条sb.append("<a href='FindServlet?page=" + i + "'>" + i + "</a>");}// 构建分页条sb.append(" ");}// 将分页条的字符串放置到request之中request.setAttribute("bar", sb.toString());// 转发到product_list.jsp页面request.getRequestDispatcher("product_list.jsp").forward(request, response);}}
表达式语言
通过EL输出数组的全部元素
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>Insert title here</title>
</head>
<body>
<%String[] arr={"Java Web开发典型模块大全","Java范例完全自学手册","JSP项目开发全程实录"}; //定义一维数组request.setAttribute("book",arr); //将数组保存到request对象中
%>
<%String[] arr1=(String[])request.getAttribute("book"); //获取保存到request范围内的变量
//通过循环和EL输出一维数组的内容for(int i=0;i<arr1.length;i++){request.setAttribute("requestI",i);
%>${requestI}:${book[requestI]}<br> <!-- 输出数组中第i个元素 -->
<%} %></body>
</html>
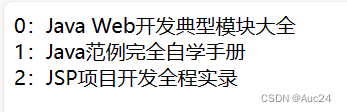
向session域中保存一个包含3个元素的List集合对象,并应用EL输出该集合的全部元素的代码
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8" import ="java.util.*"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>Insert title here</title>
</head>
<body>
<%List<String> list = new ArrayList<String>(); //声明一个List集合的对象list.add("饼干"); //添加第1个元素list.add("牛奶"); //添加第2个元素list.add("果冻"); //添加第3个元素session.setAttribute("goodsList",list); //将List集合保存到session对象中
%>
<%List<String> list1=(List<String>)session.getAttribute("goodsList"); //获取保存到session范围内的变量
//通过循环和EL输出List集合的内容for(int i=0;i<list1.size();i++){request.setAttribute("requestI",i); //将循环增量保存到request范围内
%>${requestI}:${goodsList[requestI]}<br> <!-- 输出集合中的第i个元素 -->
<%} %></body>
</html>
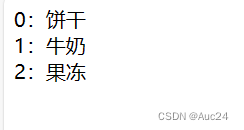
通过EL输出数组的全部元素
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>Insert title here</title>
</head>
<body>
<%request.setAttribute("userName","mr"); //定义request范围内的变量userNamerequest.setAttribute("pwd","mrsoft"); //定义pwd范围内的变量pwd
%>
userName=${userName}<br> <!-- 输入变量userName -->
pwd=${pwd}<br> <!-- 输入变量pwd -->
\${userName!="" and (userName=="明日") }: <!-- 将EL原样输出 -->
${userName!="" and userName=="明日" }<br> <!-- 输出由关系和逻辑运算符组成的表达式的值 -->
\${userName=="mr" and pwd=="mrsoft" }: <!-- 将EL原样输出 -->
${userName=="mr" and pwd=="mrsoft" } <!-- 输出由关系和逻辑运算符组成的表达式的值 --></body>
</html>
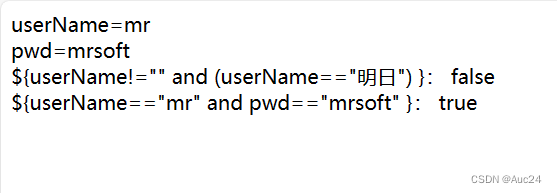
通过pageScope隐含对象读取page范围内的javaBean的属性值
package com.wgh;public class UserInfo {private String name = "";// 用户名// name属性对应的set方法public void setName(String name) {this.name = name;}// name属性对应的get方法public String getName() {return name;}
}
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8" %>
<jsp:useBean id="user" scope="page" class="com.wgh.UserInfo" type="com.wgh.UserInfo"><jsp:setProperty name="user" property="name" value="明日科技"/>
</jsp:useBean><html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>通过pageScope隐含对象读取page范围内的JavaBean的属性值</title>
</head>
<body>
${pageScope.user.name}
</body>
</html>
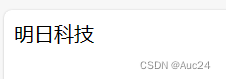
定义EL函数处理字符串中的回车换行符和空格符
package com.wgh;public class StringDeal {public static String shiftEnter(String str) { // 定义公用的静态方法String newStr = str.replaceAll("\r\n", "<br>"); // 替换回车换行符newStr = newStr.replaceAll(" ", " ");// 替换空格符return newStr;}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8" %>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>定义EL函数处理字符串中的回车换行符和空格符</title>
</head>
<body>
<form name="form1" method="post" action="deal.jsp"><textarea name="content" cols="30" rows="5"></textarea><br><input type="submit" name="Button" value="提交" >
</form></body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib uri="/stringDeal" prefix="wghfn"%>
<%request.setCharacterEncoding("UTF-8"); %>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>显示结果</title>
</head>
<body>
内容为:<br>
${wghfn:shiftEnter(param.content)}
</body>
</html>
<?xml version="1.0" encoding="UTF-8"?>
<taglib xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://java.sun.com/xml/ns/j2eeweb-jsptaglibrary_2_0.xsd"version="2.0"><tlib-version>1.0</tlib-version><uri>/stringDeal</uri><function><name>shiftEnter</name><function-class>com.wgh.StringDeal</function-class><function-signature>java.lang.String shiftEnter(java.lang.String)</function-signature></function>
</taglib>
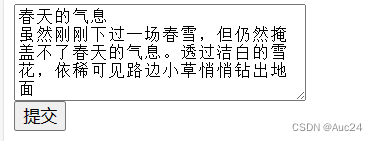
JSTL标签
应用<c:out>标签输出字符串
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"><html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>应用<c:out>标签输出字符串“水平线标记<hr>”</title>
</head>
<body>
escapeXml属性为true时:
<c:out value="水平线标记<hr>" escapeXml="true"></c:out>
<br>
escapeXml属性为false时:
<c:out value="水平线标记<hr>" escapeXml="false"></c:out>
</body>
</html>

应用<c:set>标签定义变量并为JavaBean属性赋值
public class UserInfo {private String name=""; //名称属性public void setName(String name) {this.name = name;}public String getName() {return name;}}
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>应用<c:set>标签的应用</title>
</head>
<body>
<ul><li>定义request范围内的变量username</li><br><c:set var="username" value="明日科技" scope="request"/><c:out value="username的值为:${username}"/><li>设置UserInfo对象的name属性</li><jsp:useBean class="com.wgh.UserInfo" id="userInfo"/><c:set target="${userInfo}" property="name">cdd</c:set><br><c:out value="UserInfo的name属性值为:${userInfo.name}"></c:out>
</ul>
</body>
</html>
应用<c:remove>标签移除变量
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@ page isELIgnored="false" %>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>应用<c:remove>标签移除变量</title>
</head>
<body>
<ul><li>定义request范围内的变量username</li><br><c:set var="username" value="明日科技" scope="request"/>username的值为:<c:out value="${username}"/><li>移除request范围内的变量username</li><br><c:remove var="username" scope="request"/>username的值为:<c:out value="${username}" default="空"/>
</ul>
</body>
</html>
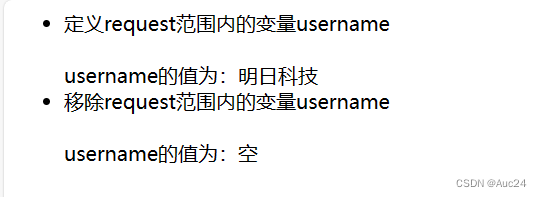
应用<c:catch>标签捕获异常信息
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>应用<c:catch>标签捕获异常信息</title>
</head>
<body>
<c:catch var="error"><jsp:useBean class="com.wgh.UserInfo" id="userInfo"/><c:set target="${userInfo}" property="pwd">111</c:set>
</c:catch>
<c:out value="${error}"/>
</body>
</html>

应用<c:import>标签导入网站Banner
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>应用<c:import>标签导入网站Banner</title>
</head>
<body style=" margin:0px;">
<c:set var="typeName" value="流行金曲 | 经典老歌 | 热舞DJ | 欧美金曲 | 少儿歌曲 | 轻音乐 | 最新上榜"/>
<!-- 导入网站的Banner -->
<c:import url="navigation.jsp" charEncoding="UTF-8"><c:param name="typeList" value="${typeName}"/>
</c:import></body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%request.setCharacterEncoding("UTF-8"); %>
<table width="901" height="128" border="0" align="center" cellpadding="0" cellspacing="0" background="images/bg.jpg"><tr><td width="16" height="91"> </td><td width="885"> </td></tr><tr><td> </td><td style=" font-size:11pt; color:#FFFFFF"><b>${param.typeList}</b></td></tr>
</table>

应用<c:url>标签生成带参数的URL地址
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>应用<c:url>标签生成带参数的URL地址</title>
</head>
<body>
<c:url var="path" value="register.jsp" scope="page"><c:param name="user" value="mr"/><c:param name="email" value="wgh717@sohu.com"/>
</c:url>
<a href="${pageScope.path }">提交注册</a>
</body>
</html>
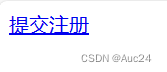
应用<c:redirect>和<c:param>标签实现重定向页面并传递参数
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>重定向页面并传递参数</title>
</head>
<body>
<c:redirect url="main.jsp"><c:param name="user" value="wgh/"></c:param>
</c:redirect>
</body>
</html>
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>显示结果</title>
</head>
<body>
[${param.user}]您好,欢迎访问我公司网站!
</body>
</html>

应用<c:if>标签根据是否登录显示不同的内容
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>根据是否登录显示不同的内容</title>
</head>
<body>
<c:if var="result" test="${empty param.username}"><form name="form1" method="post" action="">用户名:<input name="username" type="text" id="username"><br><br><input type="submit" name="Submit" value="登录"></form>
</c:if>
<c:if test="${!result}">[${param.username}] 欢迎访问我公司网站
</c:if>
</body>
</html>
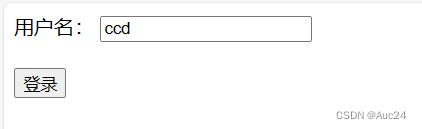

应用<c:choose>标签根据是否登录显示不同的内容
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>根据是否登录显示不同的内容</title>
</head>
<body>
<c:choose><c:when test="${empty param.username}"><form name="form1" method="post" action="">用户名:<input name="username" type="text" id="username"><br><br><input type="submit" name="Submit" value="登录"></form></c:when><c:otherwise>[${param.username }] 欢迎访问我公司网站!</c:otherwise>
</c:choose>
</body>
</html>
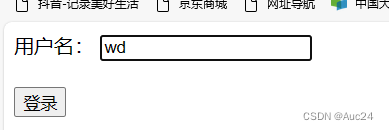

实现分时问候
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>实现分时问候</title>
</head>
<body>
<!-- 获取小时并保存到变量中 -->
<c:set var="hours"><%=new java.util.Date().getHours()%>
</c:set>
<!-- 获取分钟并保存到变量中-->
<c:set var="second"><%=new java.util.Date().getMinutes()%>
</c:set>
<c:choose><c:when test="${hours>1 && hours<6}">早上好!</c:when><c:when test="${hours>6 && hours<11}" >上午好!</c:when><c:when test="${hours>11 && hours<17}">下午好!</c:when><c:when test="${hours>17 && hours<24}">晚上好!</c:when>
</c:choose>
现在时间是:${hours}:${second}
</body>
</html>

幸运大抽奖
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%@ page import="java.util.*" %>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>幸运大抽奖</title>
</head>
<body>
<%Random rnd=new Random();%>
<!-- 将抽取的幸运数字保存到变量中 -->
<c:set var="luck"><%=rnd.nextInt(10)%>
</c:set>
<c:choose><c:when test="${luck==6}">恭喜你,中了一等奖!</c:when><c:when test="${luck==7}" >恭喜你,中了二等奖!</c:when><c:when test="${luck==8}">恭喜你,中了三等奖!</c:when><c:otherwise>谢谢您的参与!</c:otherwise>
</c:choose>
</body>
</html>
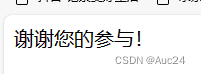
遍历List集合
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%@ page import="java.util.*" %>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>遍历List集合</title>
</head>
<body>
<%List<String> list=new ArrayList<String>(); //创建List集合的对象list.add("简单是可靠的先决条件"); //向List集合中添加元素list.add("兴趣是最好的老师");list.add("知识上的投资总能得到最好的回报");request.setAttribute("list",list); //将List集合保存到request对象中
%>
<b>遍历List集合的全部元素:</b><br>
<c:forEach items="${requestScope.list}" var="keyword" varStatus="id">${id.index } ${keyword}<br>
</c:forEach>
<b>遍历List集合中第1个元素以后的元素(不包括第1个元素):</b><br>
<c:forEach items="${requestScope.list}" var="keyword" varStatus="id" begin="1">${id.index } ${keyword}<br>
</c:forEach>
</body>
</html>
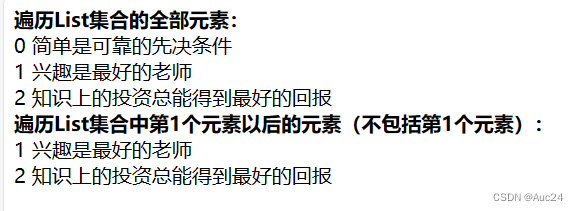
应用<c:forEach>列举0以内的全部奇数
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>应用<c:forEach>列举10以内全部奇数</title>
</head>
<body>
<b>10以内的全部奇数为:</b>
<c:forEach var="i" begin="1" end="10" step="2">${i}
</c:forEach>
</body>
</html>

应用<c:forTokens>标签分割字符串
<%@ page isELIgnored="false" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<html>
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"><title>应用<c:forTokens>分隔字符串</title>
</head>
<body>
<c:set var="sourceStr" value="Java Web:程序开发范例宝典、典型模块大全;Java:实例完全自学手册、典型模块大全"/>
<b>原字符串:</b><c:out value="${sourceStr}"/>
<br><b>分割后的字符串:</b><br>
<c:forTokens items="${sourceStr}" delims=":、;" var="item">${item}<br>
</c:forTokens>
</body>
</html>
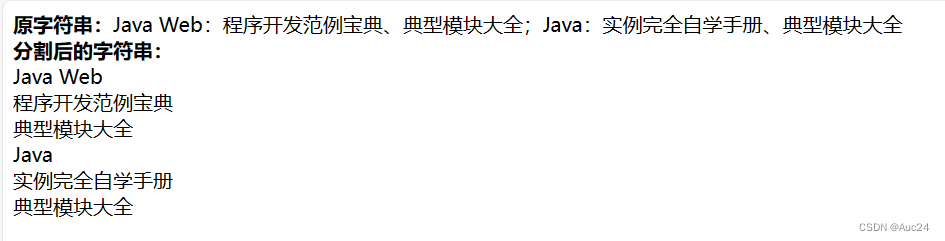
Ajax技术
检测用户名是否唯一
<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"><html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>检测用户是否唯一</title>
<script language="JavaScript">
function createRequest(url){http_request=false;if(window.XMLHttpRequest){http_request=new XMLHttpRequest();}else if(window.ActiveXObject){try{http_request=new ActiveXObject("Msxml2.XMLHTTP");}catch (e){try {http_request=new ActiveXObject("Microsoft.XMLHTTP");}catch (e){}}}if(!http_request){alert("不能创建实例对象");return false;}http_request.onreadystatechange=getResult;http_request.open('GET',url,true);http_request.send(null);}function getResult(){if(http_request.readyState==4){if(http_request.stattus==200){document.getElementById("toolTip").innerHTML=http_request.respoonseText;document.getElementById("toolTip").style.display="block";}else {alert("您所请求的页面有错误")}}}function checkUser(userName){if(userName.value==""){alert("请输入用户名!");userName.focus();return;}else {createRequest('checkUser.jsp?user='+userName.value);}}
</script>
<style type="text/css">
<!--
#toolTip{position:absolute;left: 331px;top: 39px;width: 98px;height: 48px;padding-top: 45px;padding-left: 25px;padding-right: 25px;z-index: 1;display: none;color: red;}
-->
</style>
</head>
<body style="margin: 0px">
<form method="post" action="" name="form1">
<b>用户名:</b><input name="username" type="text" id="usermame" size="32"><b></b>
<img src="images/checkBt.jpg" width="104" height="23" style="cursor:hand;" onclick="checkUser(form .username);">
<b>密码:</b><input name="pwd1" type="password" id="pwd1" size="35">
<b>确认密码:</b><input name="pwd2" type="password" id="pwd2" size="35">
E-mail: <input name="email" type="text" id="email" size="45">
<input type="image" name="imageField" src="images/registerBt.jpg">
</form>

应用Ajax重构实现
var net = new Object(); // 定义一个全局变量net
// 编写构造函数
net.AjaxRequest = function(url, onload, onerror, method, params) {this.req = null;this.onload = onload;this.onerror = (onerror) ? onerror : this.defaultError;this.loadDate(url, method, params);
}
// 编写用于初始化XMLHttpRequest对象并指定处理函数,最后发送HTTP请求的方法
net.AjaxRequest.prototype.loadDate = function(url, method, params) {if (!method) {method = "GET";}if (window.XMLHttpRequest) {this.req = new XMLHttpRequest();} else if (window.ActiveXObject) {this.req = new ActiveXObject("Microsoft.XMLHTTP");}if (this.req) {try {var loader = this;this.req.onreadystatechange = function() {net.AjaxRequest.onReadyState.call(loader);}this.req.open(method, url, true);// 建立对服务器的调用if (method == "POST") {// 如果提交方式为POSTthis.req.setRequestHeader("Content-Type","application/x-www-form-urlencoded"); // 设置请求头}this.req.send(params); // 发送请求} catch (err) {this.onerror.call(this);}}
}// 重构回调函数
net.AjaxRequest.onReadyState = function() {var req = this.req;var ready = req.readyState;if (ready == 4) {// 请求完成if (req.status == 200) {// 请求成功this.onload.call(this);} else {this.onerror.call(this);}}
}
// 重构默认的错误处理函数
net.AjaxRequest.prototype.defaultError = function() {alert("错误数据\n\n回调状态:" + this.req.readyState + "\n状态: " + this.req.status);
}