1. abs()
abs() 返回数字的绝对值。
>>> abs(-7)
**输出:**7
>>> abs(7)
输出:
7
2. all()
all() 将容器作为参数。如果 python 可迭代对象中的所有值都是 True ,则此函数返回 True。空值为 False。
>>> all({'*','',''})
输出:
False
>>> all([' ',' ',' '])
输出:
True
3. any()
如果可迭代对象中的任意一个值为 True,则此函数返回 True。。
>>> any((1,0,0))
输出:
True
>>> any((0,0,0))
输出:
False
4. ascii()
返回一个表示对象的字符串。
>>> ascii('ș')
输出:
“‘\u0219′”
>>> ascii('ușor')
输出:
“‘u\u0219or’”
>>> ascii(['s','ș'])
输出:
“[‘s’, ‘\u0219’]”
5. bin()
将整数转换为二进制字符串。不能将其应用于浮点数。
>>> bin(7)
输出:
‘0b111’
>>> bin(7.0)
输出:
Traceback (most recent call last):File “<pyshell#20>”, line 1, in
bin(7.0)
TypeError: ‘float’ object cannot be interpreted as an integer
6. bool()
bool() 将值转换为布尔值。
>>> bool(0.5)
输出:
True
>>> bool('')
输出:
False
>>> bool(True)
输出:
True
7. bytearray()
bytearray() 返回给定大小的 python 新字节数组。
>>> a=bytearray(4)
>>> a
输出:
bytearray(b’\x00\x00\x00\x00′)
>>> a.append(1)
>>> a
输出:
bytearray(b’\x00\x00\x00\x00\x01′)
>>> a[0]=1
>>> a
输出:
bytearray(b’\x01\x00\x00\x00\x01′)
>>> a[0]
输出:
1
可以处理列表。
>>> bytearray([1,2,3,4])
输出:
bytearray(b’\x01\x02\x03\x04′)
8. bytes()
bytes()返回一个不可变的字节对象。
>>> bytes(5)
输出:
b’\x00\x00\x00\x00\x00′
>>> bytes([1,2,3,4,5])
输出:
b’\x01\x02\x03\x04\x05′
>>> bytes('hello','utf-8')
输出:
b’hello’Here, utf-8 is the encoding.
bytearray() 是可变的,而 bytes() 是不可变的。
>>> a=bytes([1,2,3,4,5])
>>> a
输出:
b’\x01\x02\x03\x04\x05′
>>> a[4]= 3
输出:
3Traceback (most recent call last):
File “<pyshell#46>”, line 1, in
a[4]=3
TypeError: ‘bytes’ object does not support item assignment
Let’s try this on bytearray().
>>> a=bytearray([1,2,3,4,5])
>>> a
输出:
bytearray(b’\x01\x02\x03\x04\x05′)
>>> a[4]=3
>>> a
输出:
bytearray(b’\x01\x02\x03\x04\x03′)
9. callable()
callable() 用于检查一个对象是否是可调用的。
>>> callable([1,2,3])
输出:
False
>>> callable(callable)
输出:
True
>>> callable(False)
输出:
False
>>> callable(list)
输出:
True
10. chr()
chr() 用一个范围在(0~255)整数作参数(ASCII),返回一个对应的字符。
>>> chr(65)
输出:
‘A’
>>> chr(97)
输出:
‘a’
>>> chr(9)
输出:
‘\t’
>>> chr(48)
输出:
‘0’
11. classmethod()
classmethod() 返回函数的类方法。
\>>> class hello: def sayhi(self): print("Hello World!") \>>> hello.sayhi\=classmethod(hello.sayhi)
\>>> hello.sayhi()
输出:
Hello World!
当我们将方法 sayhi() 作为参数传递给 classmethod() 时,它会将其转换为属于该类的 python 类方法。
然后,我们调用它,就像我们在 python 中调用静态方法一样。
12. compile()
compile() 将一个字符串编译为字节代码。
>>> exec(compile('a=5\nb=7\nprint(a+b)','','exec'))
输出:
12
13. complex()
complex() 创建转换为复数。
>>> complex(3)
输出:
(3+0j)
>>> complex(3.5)
输出:
(3.5+0j)
>>> complex(3+5j)
输出:
(3+5j)
14. delattr()
delattr() 用于删除类的属性。
\>>> class fruit: size\=7
\>>> orange\=fruit() \>>> orange.size
输出:
7
\>>> delattr(fruit,'size') \>>> orange.size
输出:
Traceback (most recent call last):File “<pyshell#95>”, line 1, in
orange.size
AttributeError: ‘fruit’ object has no attribute ‘size’
15. dict()
dict() 用于创建一个字典。
>>> dict()
输出:
{}
>>> dict([(1,2),(3,4)])
输出:
{1: 2, 3: 4}
16. dir()
dir() 返回对象的属性。
\>>> class fruit: size\=7 shape\='round'
\>>> orange\=fruit()
\>>> dir(orange)
输出:
\[‘\_\_class\_\_’, ‘\_\_delattr\_\_’, ‘\_\_dict\_\_’, ‘\_\_dir\_\_’, ‘\_\_doc\_\_’, ‘\_\_eq\_\_’, ‘\_\_format\_\_’, ‘\_\_ge\_\_’, ‘\_\_getattribute\_\_’, ‘\_\_gt\_\_’, ‘\_\_hash\_\_’, ‘\_\_init\_\_’, ‘\_\_init\_subclass\_\_’, ‘\_\_le\_\_’, ‘\_\_lt\_\_’, ‘\_\_module\_\_’, ‘\_\_ne\_\_’, ‘\_\_new\_\_’, ‘\_\_reduce\_\_’, ‘\_\_reduce\_ex\_\_’, ‘\_\_repr\_\_’, ‘\_\_setattr\_\_’, ‘\_\_sizeof\_\_’, ‘\_\_str\_\_’, ‘\_\_subclasshook\_\_’, ‘\_\_weakref\_\_’, ‘shape’, ‘size’\]
17. divmod()
divmod() 把除数和余数运算结果结合起来,返回一个包含商和余数的元组。
>>> divmod(3,7)
输出:
(0, 3)
>>> divmod(7,3)
输出:
(2, 1)
18. enumerate()
用于将一个可遍历的数据对象(如列表、元组或字符串)组合为一个索引序列,同时列出数据和数据下标,一般用在 for 循环当中。
\>>> for i in enumerate(\['a','b','c'\]): print(i)
输出:
(0, ‘a’)(1, ‘b’)(2, ‘c’)
19. eval()
用来执行一个字符串表达式,并返回表达式的值。
字符串表达式可以包含变量、函数调用、运算符和其他 Python 语法元素。
>>> x=7
>>> eval('x+7')
输出:
14
>>> eval('x+(x%2)')
输出:
8
20. exec()
exec() 动态运行 Python 代码。
>>> exec('a=2;b=3;print(a+b)')
输出:
5
>>> exec(input("Enter your program"))
输出:
Enter your programprint
21. filter()
过滤掉条件为True的项目。
>>> list(filter(lambda x:x%2==0,[1,2,0,False]))
输出:
[2, 0, False]
22. float()
转换为浮点数。
>>> float(2)
输出:
2.0
>>> float('3')
输出:
3.0
>>> float('3s')
输出:
Traceback (most recent call last):File “<pyshell#136>”, line 1, in
float(‘3s’)
ValueError: could not convert string to float: ‘3s’
>>> float(False)
输出:
0.0
>>> float(4.7)
输出:
4.7
23. format()
格式化输出字符串。
>>> a,b=2,3
>>> print("a={0} and b={1}".format(a,b))
输出:
a=2 and b=3
>>> print("a={a} and b={b}".format(a=3,b=4))
输出:
a=3 and b=4
24. frozenset()
frozenset() 返回一个冻结的集合,冻结后集合不能再添加或删除任何元素。
>>> frozenset((3,2,4))
输出:
frozenset({2, 3, 4})
25. getattr()
getattr() 返回对象属性的值。
>>> getattr(orange,'size')
输出:
7
26. globals()
以字典类型返回当前位置的全部全局变量。
>>> globals()
输出:
{‘\_\_name\_\_’: ‘\_\_main\_\_’, ‘\_\_doc\_\_’: None, ‘\_\_package\_\_’: None, ‘\_\_loader\_\_’: <class ‘\_frozen\_importlib.BuiltinImporter’>, ‘\_\_spec\_\_’: None, ‘\_\_annotations\_\_’: {}, ‘\_\_builtins\_\_’: <module ‘builtins’ (built-in)>, ‘fruit’: <class ‘\_\_main\_\_.fruit’>, ‘orange’: <\_\_main\_\_.fruit object at 0x05F937D0>, ‘a’: 2, ‘numbers’: \[1, 2, 3\], ‘i’: (2, 3), ‘x’: 7, ‘b’: 3}
27. hasattr()
用于判断对象是否包含对应的属性。
>>> hasattr(orange,'size')
输出:
True
>>> hasattr(orange,'shape')
输出:
True
>>> hasattr(orange,'color')
输出:
False
28. hash()
hash() 返回对象的哈希值。
>>> hash(orange)
输出:
6263677
>>> hash(orange)
输出:
6263677
>>> hash(True)
输出:
1
>>> hash(0)
输出:
0
>>> hash(3.7)
输出:
644245917
>>> hash(hash)
输出:
25553952
This was all about hash() Python In Built function
29. help()
获取有关任何模块、关键字、符号或主题的详细信息。
>>> help()
Welcome to Python 3.7’s help utility!
If this is your first time using Python, you should definitely check outthe tutorial on the Internet at https://docs.python.org/3.7/tutorial/.
Enter the name of any module, keyword, or topic to get help on writingPython programs and using Python modules. To quit this help utility andreturn to the interpreter, just type “quit”.
To get a list of available modules, keywords, symbols, or topics, type"modules", “keywords”, “symbols”, or “topics”. Each module also comeswith a one-line summary of what it does; to list the modules whose nameor summary contain a given string such as “spam”, type “modules spam”.
help> mapHelp on class map in module builtins:
class map(object) | map(func, _iterables) --> map object | | Make an iterator that computes the function using arguments from | each of the iterables. Stops when the shortest iterable is exhausted. | | Methods defined here: | | getattribute(self, name, /) | Return getattr(self, name). | | iter(self, /) | Implement iter(self). | | next(self, /) | Implement next(self). | | reduce(…) | Return state information for pickling. | | ---------------------------------------------------------------------- | Static methods defined here: | | new(_args, **kwargs) from builtins.type | Create and return a new object. See help(type) for accurate signature.
help>
30. hex()
Hex() 将整数转换为十六进制。
>>> hex(16)
输出:
‘0x10’
>>> hex(False)
输出:
‘0x0’
31. id()
id() 返回对象的标识。
>>> id(orange)
输出:
100218832
>>> id({1,2,3})==id({1,3,2})
输出:
True
32. input()
Input() 接受一个标准输入数据,返回为 string 类型。
>>> input("Enter a number")
输出:
Enter a number7‘7’
请注意,输入作为字符串返回。如果我们想把 7 作为整数,我们需要对它应用 int() 函数。
>>> int(input("Enter a number"))
输出:
Enter a number7
7
33. int()
int() 将值转换为整数。
>>> int('7')
输出:
7
34. isinstance()
如果对象的类型与参数二的类型相同则返回 True,否则返回 False。
>>> isinstance(0,str)
输出:
False
>>> isinstance(orange,fruit)
输出:
True
35. issubclass()
有两个参数 ,如果第一个类是第二个类的子类,则返回 True。否则,它将返回 False。
>>> issubclass(fruit,fruit)
输出:
True
\>>> class fruit:\` pass
\>>> class citrus(fruit): pass
\>>> issubclass(fruit,citrus)
输出:
False
36. iter()
Iter() 返回对象的 python 迭代器。
\>>> for i in iter(\[1,2,3\]): print(i)
输出:
123
37. len()
返回对象的长度。
>>> len({1,2,2,3})
输出:
3
38. list()
list() 从一系列值创建一个列表。
>>> list({1,3,2,2})
输出:
[1, 2, 3]
39. locals()
以字典类型返回当前位置的全部局部变量。
>>> locals()
输出:
{‘\_\_name\_\_’: ‘\_\_main\_\_’, ‘\_\_doc\_\_’: None, ‘\_\_package\_\_’: None, ‘\_\_loader\_\_’: <class ‘\_frozen\_importlib.BuiltinImporter’>, ‘\_\_spec\_\_’: None, ‘\_\_annotations\_\_’: {}, ‘\_\_builtins\_\_’: <module ‘builtins’ (built-in)>, ‘fruit’: <class ‘\_\_main\_\_.fruit’>, ‘orange’: <\_\_main\_\_.fruit object at 0x05F937D0>, ‘a’: 2, ‘numbers’: \[1, 2, 3\], ‘i’: 3, ‘x’: 7, ‘b’: 3, ‘citrus’: <class ‘\_\_main\_\_.citrus’>}
40. map()
会根据提供的函数对指定序列做映射。
>>> list(map(lambda x:x%2==0,[1,2,3,4,5]))
输出:
[False, True, False, True, False]
41. max()
返回最大值。
>>> max(2,3,4)
输出:
4
>>> max([3,5,4])
输出:
5
>>> max('hello','Hello')
输出:
‘hello’
42. memoryview()
memoryview() 返回给定参数的内存查看对象(memory view)。
>>> a=bytes(4)
>>> memoryview(a)
输出:
<memory at 0x05F9A988>
\>>> for i in memoryview(a): print(i)
43. min()
min() 返回序列中的最小值。
>>> min(3,5,1)
输出:
1
>>> min(True,False)
输出:
False
44. next()
从迭代器返回下一个元素。
>>> myIterator=iter([1,2,3,4,5])
>>> next(myIterator)
输出:
1
>>> next(myIterator)
输出:
2
>>> next(myIterator)
输出:
3
>>> next(myIterator)
输出:
4
>>> next(myIterator)
输出:
5
遍历了所有项目后,再次调用 next() 时,会引发 StopIteration。
>>> next(myIterator)
输出:
Traceback (most recent call last):File “<pyshell#392>”, line 1, in
next(myIterator)
StopIteration
45. object()
Object() 创建一个无特征的对象。
>>> o=object()
>>> type(o)
输出:
<class ‘object’>
>>> dir(o)
输出:
\[‘\_\_class\_\_’, ‘\_\_delattr\_\_’, ‘\_\_dir\_\_’, ‘\_\_doc\_\_’, ‘\_\_eq\_\_’, ‘\_\_format\_\_’, ‘\_\_ge\_\_’, ‘\_\_getattribute\_\_’, ‘\_\_gt\_\_’, ‘\_\_hash\_\_’, ‘\_\_init\_\_’, ‘\_\_init\_subclass\_\_’, ‘\_\_le\_\_’, ‘\_\_lt\_\_’, ‘\_\_ne\_\_’, ‘\_\_new\_\_’, ‘\_\_reduce\_\_’, ‘\_\_reduce\_ex\_\_’, ‘\_\_repr\_\_’, ‘\_\_setattr\_\_’, ‘\_\_sizeof\_\_’, ‘\_\_str\_\_’, ‘\_\_subclasshook\_\_’\]
46. oct()
oct() 将整数转换为其八进制表示形式。
>>> oct(7)
输出:
‘0o7’
>>> oct(8)
输出:
‘0o10’
>>> oct(True)
输出:
‘0o1’
47. open()
open() 打开一个文件。
\>>> import os \>>> os.chdir('C:\\\\Users\\\\lifei\\\\Desktop') \# Now, we open the file ‘topics.txt’. \>>> f=open('topics.txt') \>>> f
输出:
<_io.TextIOWrapper name=’topics.txt’ mode=’r’ encoding=’cp1252′>
>>> type(f)
输出:
<class ‘_io.TextIOWrapper’>
48. ord()
是 chr() 函数或 unichr() 函数的配对函数,它以一个字符(长度为1的字符串)作为参数,返回对应的 ASCII 数值,或者 Unicode 数值
>>> ord('A')
输出:
65
>>> ord('9')
输出:
57
>>> chr(65)
输出:
‘A’
49. pow()
pow() 有两个参数。比如 x 和 y。它将值返回 x 的 y 次方。
>>> pow(3,4)
输出:
81
>>> pow(7,0)
输出:
1
>>> pow(7,-1)
输出:
0.14285714285714285
>>> pow(7,-2)
输出:
0.02040816326530612
50. print()
用于打印输出,最常见的一个函数。
>>> print("Hello world!")
输出:
Hello world!
51. property()
作用是在新式类中返回属性值。
class C(object): def \_\_init\_\_(self): self.\_x = None def getx(self): return self.\_x def setx(self, value): self.\_x = value def delx(self): del self.\_x x = property(getx, setx, delx, "I'm the 'x' property.")
52. range()
可创建一个整数列表,一般用在 for 循环中。
\>>> for i in range(7,2,-2): print(i)
输出:
753
53. repr()
将对象转化为供解释器读取的形式。
>>> repr("Hello")
输出:
“‘Hello’”
>>> repr(7)
输出:
‘7’
>>> repr(False)
输出:
‘False’
54. reversed()
返回一个反转的迭代器。
>>> a=reversed([3,2,1])
>>> a
输出:
<list_reverseiterator object at 0x02E1A230>
\>>> for i in a: print(i)
输出:
123
>>> type(a)
输出:
<class ‘list_reverseiterator’>
55. round()
将浮点数舍入到给定的位数。
>>> round(3.777,2)
输出:
3.78
>>> round(3.7,3)
输出:
3.7
>>> round(3.7,-1)
输出:
0.0
>>> round(377.77,-1)
输出:
380.0
56. set()
创建一个无序不重复元素集合。
>>> set([2,2,3,1])
输出:
{1, 2, 3}
57. setattr()
对应函数 getattr(),用于设置属性值,该属性不一定是存在的。
>>> orange.size
输出:
7
>>> orange.size=8
>>> orange.size
输出:
8
58. slice()
slice() 实现切片对象,主要用在切片操作函数里的参数传递。
>>> slice(2,7,2)
输出:
slice(2, 7, 2)
>>> 'Python'[slice(1,5,2)]
输出:
‘yh’
59. sorted()
对所有可迭代的对象进行排序操作。
>>> sorted('Python')
输出:
[‘P’, ‘h’, ‘n’, ‘o’, ‘t’, ‘y’]
>>> sorted([1,3,2])
输出:
[1, 2, 3]
60. staticmethod()
返回函数的静态方法。
\>>> class fruit: def sayhi(): print("Hi")
\>>> fruit.sayhi=staticmethod(fruit.sayhi)
\>>> fruit.sayhi()
输出:
Hi
\>>> class fruit: @staticmethod def sayhi(): print("Hi")
\>>> fruit.sayhi()
输出:
Hi
61. str()
str() 返回一个对象的string格式。
>>> str('Hello')
输出:
‘Hello’
>>> str(7)
输出:
‘7’
>>> str(8.7)
输出:
‘8.7’
>>> str(False)
输出:
‘False’
>>> str([1,2,3])
输出:
‘[1, 2, 3]’
62. sum()
将可迭代对象作为参数,并返回所有值的总和。
>>> sum([3,4,5],3)
输出:
15
63. super()
super() 用于调用父类(超类)的一个方法。
\>>> class person: def \_\_init\_\_(self): print("A person")
\>>> class student(person): def \_\_init\_\_(self): super().\_\_init\_\_() print("A student")
\>>> Avery=student()
输出:
A personA student
64. tuple()
创建一个元组。
输出:
(1, 3, 2)
>>> tuple({1:'a',2:'b'})
输出:
(1, 2)
65. type()
返回对象的类型。
>>> type({})
输出:
<class ‘dict’>
>>> type(set())
输出:
<class ‘set’>
>>> type(())
输出:
<class ‘tuple’>
>>> type((1))
输出:
<class ‘int’>
>>> type((1,))
输出:
<class ‘tuple’>
66. vars()
vars() 返回对象object的属性和属性值的字典对象。
>>> vars(fruit)
输出:
mappingproxy({‘\_\_module\_\_’: ‘\_\_main\_\_’, ‘size’: 7, ‘shape’: ’round’, ‘\_\_dict\_\_’: <attribute ‘\_\_dict\_\_’ of ‘fruit’ objects>, ‘\_\_weakref\_\_’: <attribute ‘\_\_weakref\_\_’ of ‘fruit’ objects>, ‘\_\_doc\_\_’: None})
67. zip()
zip() 用于将可迭代的对象作为参数,将对象中对应的元素打包成一个个元组,然后返回由这些元组组成的列表。
>>> set(zip([1,2,3],['a','b','c']))
输出:
{(1, ‘a’), (3, ‘c’), (2, ‘b’)}
>>> set(zip([1,2],[3,4,5]))
输出:
{(1, 3), (2, 4)}
>>> a=zip([1,2,3],['a','b','c'])
>>> x,y,z=a
>>> x
输出:
(1, ‘a’)
>>> y
输出:
(2, ‘b’)
>>> z
输出:
(3, ‘c’)
点亮在看,你最好看!
本文转自 https://mp.weixin.qq.com/s/KwRpAHT-Df1KVplUJCU63w,如有侵权,请联系删除。
题外话
感兴趣的小伙伴,赠送全套Python学习资料,包含面试题、简历资料等具体看下方。
👉CSDN大礼包🎁:全网最全《Python学习资料》免费赠送🆓!(安全链接,放心点击)
一、Python所有方向的学习路线
Python所有方向的技术点做的整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照下面的知识点去找对应的学习资源,保证自己学得较为全面。
二、Python必备开发工具
工具都帮大家整理好了,安装就可直接上手!
三、最新Python学习笔记
当我学到一定基础,有自己的理解能力的时候,会去阅读一些前辈整理的书籍或者手写的笔记资料,这些笔记详细记载了他们对一些技术点的理解,这些理解是比较独到,可以学到不一样的思路。
四、Python视频合集
观看全面零基础学习视频,看视频学习是最快捷也是最有效果的方式,跟着视频中老师的思路,从基础到深入,还是很容易入门的。
五、实战案例
纸上得来终觉浅,要学会跟着视频一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。
六、面试宝典
简历模板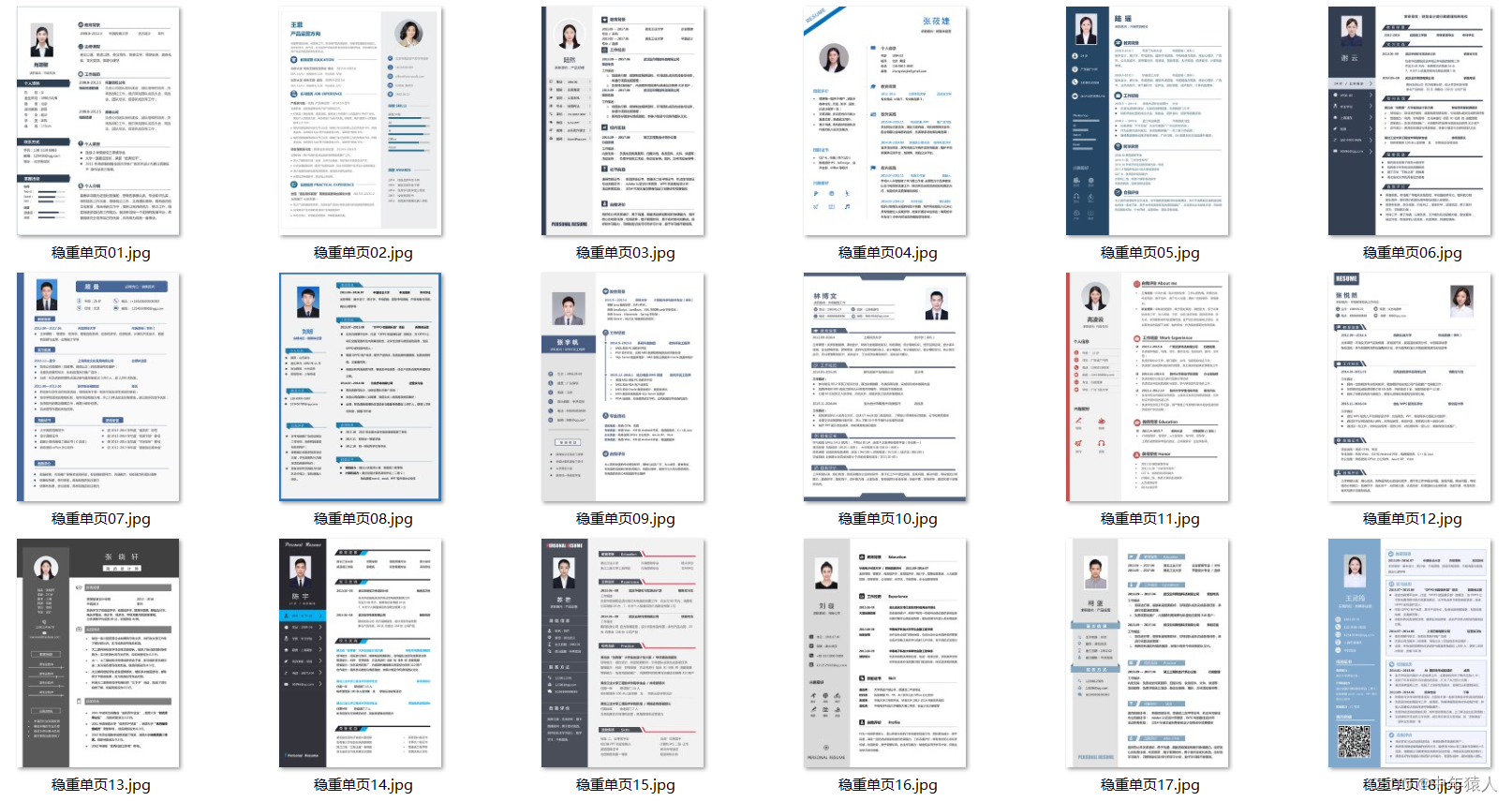
👉CSDN大礼包🎁:全网最全《Python学习资料》免费赠送🆓!(安全链接,放心点击)
若有侵权,请联系删除