目录
- 206反转链表【链表结构基础】
- 21合并两个有序链表【递归】
- 我的答案【错误】
- 自己修改【超出时间限制】
- 在官方那里学到的【然后自己复写,错误】
- 对照官方【自己修改】
- 160相交链表【未理解题目目的】
- 在b站up那里学到的【然后自己复写,错误】
- 【超出时间限制】
- 对照官方【自己修改】
- 19删除链表的倒数第 N 个结点
- 看完官方解题思路【自己复写,错误】
- 报错信息
- 第一遍修改
- 第二遍修改
- 148排序链表
- 我的答案【冒泡排序:最小的负数输出时变为0】
-
206反转链表【链表结构基础】
class Solution(object):def reverseList(self, head):""":type head: ListNode:rtype: ListNode"""pre=Nonecur=headwhile cur!=None:temp=cur.nextcur.next=prepre=curcur=tempreturn pre
21合并两个有序链表【递归】
我的答案【错误】
class Solution(object):def mergeTwoLists(self, list1, list2):""":type list1: Optional[ListNode]:type list2: Optional[ListNode]:rtype: Optional[ListNode]"""resol=ListNode()while list1!=None and list2!=None:if list1.val<=list2.val:resol.next=ListNode(list1.val)else:resol.next=ListNode(list2.val)list1=list1.nextlist2=list2.nextwhile list1!=None:resol.next=ListNode(list1.val)while list2!=None:resol.next=ListNode(list2.val)return resol.next
自己修改【超出时间限制】
class Solution(object):def mergeTwoLists(self, list1, list2):""":type list1: Optional[ListNode]:type list2: Optional[ListNode]:rtype: Optional[ListNode]"""resol=ListNode(0)temp=resolwhile list1 and list2:if list1.val<=list2.val:resol.next=list1list1=list1.nextelse:resol.next=list2list2=list2.nextresol=resol.nextwhile list1:resol.next=list1while list2:resol.next=list2return temp.next
在官方那里学到的【然后自己复写,错误】
class Solution(object):def mergeTwoLists(self, list1, list2):""":type list1: Optional[ListNode]:type list2: Optional[ListNode]:rtype: Optional[ListNode]"""if list1 is None:return list2elif list2 is None:return list1else:if list1.val<list2.val:list1.next=mergeTwoLists(self,list1.next,list2)return list1else:list2.next=mergeTwoLists(self,list2.next,list1)return list2
对照官方【自己修改】
class Solution(object):def mergeTwoLists(self, list1, list2):""":type list1: Optional[ListNode]:type list2: Optional[ListNode]:rtype: Optional[ListNode]"""if list1 is None:return list2elif list2 is None:return list1elif list1.val<list2.val:list1.next=self.mergeTwoLists(list1.next,list2)return list1else:list2.next=self.mergeTwoLists(list2.next,list1)return list2
160相交链表【未理解题目目的】
- 我看输入部分都已经给了要输出的结果了,就不明白这题目的是要做什么。
在b站up那里学到的【然后自己复写,错误】
class Solution(object):def getIntersectionNode(self, headA, headB):""":type head1, head1: ListNode:rtype: ListNode"""p=headA,q=headBwhile(p!=q):p ? p.next : headBq ? q.next : headAprint("p.val")
【超出时间限制】
class Solution(object):def getIntersectionNode(self, headA, headB):""":type head1, head1: ListNode:rtype: ListNode"""p=headAq=headBwhile(p!=q):if p.next !=None:p=p.nextelse:p=headBif q.next!=None:q=q.nextelse:q=headAreturn p
对照官方【自己修改】
class Solution(object):def getIntersectionNode(self, headA, headB):""":type head1, head1: ListNode:rtype: ListNode"""p=headA q=headB while(p!=q):p = p.next if p else headBq = q.next if q else headAreturn p
19删除链表的倒数第 N 个结点
看完官方解题思路【自己复写,错误】
class Solution(object):def removeNthFromEnd(self, head, n):""":type head: ListNode:type n: int:rtype: ListNode"""p=headfor i in range(n+1):p=p.nextq=headwhile p!=None:q=q.nextp=p.nextq.next=q.next.nextreturn head
报错信息
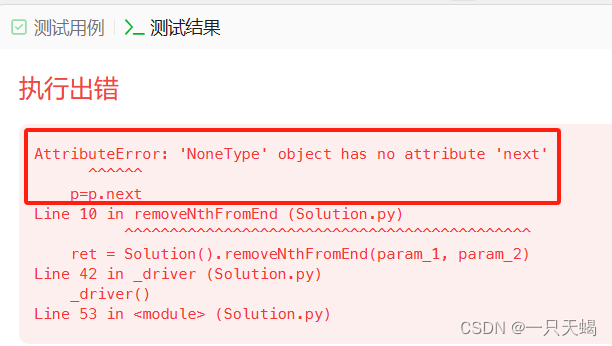
- 查阅资料后发现:【self.head是指向第一个元素的,此时为None,所以没有next属性,自然就会报错。因此第一个结点需要特殊处理,我们一般通过增加头结点的方式来避免这种特殊处理。】
第一遍修改
- 发现当链表有n个元素时,无法删除倒数第n个元素。
- 【没有考虑到第一个结点的特殊性。】
class Solution(object):def removeNthFromEnd(self, head, n):""":type head: ListNode:type n: int:rtype: ListNode"""p=ListNode(0, head)for i in range(n+1):p=p.nextq=ListNode(0, head)while p!=None:q=q.nextp=p.nextq.next=q.next.nextreturn head
第二遍修改
class Solution(object):def removeNthFromEnd(self, head, n):""":type head: ListNode:type n: int:rtype: ListNode"""dummy=ListNode(0, head)p=dummyfor i in range(n+1):p=p.nextq=dummywhile p!=None:q=q.nextp=p.nextq.next=q.next.nextreturn dummy.next
148排序链表
我的答案【冒泡排序:最小的负数输出时变为0】
class Solution(object):def sortList(self, head):""":type head: ListNode:rtype: ListNode"""dummy=ListNode(0,head)q=dummywhile q.next!=None:p=dummywhile p.next!=None:if p.val>p.next.val:temp=p.valp.val=p.next.valp.next.val=tempp=p.nextq=q.nextreturn dummy.next
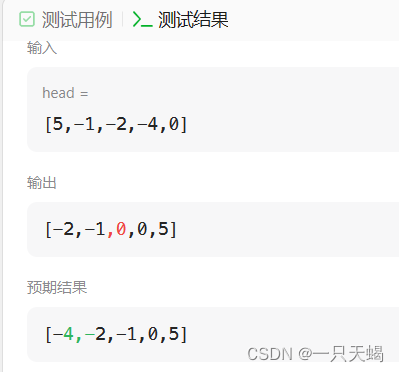
官方答案【归并排序】
class Solution(object):def sortList(self, head):def sortFunc(head, tail):if not head:return headif head.next == tail:head.next = Nonereturn headslow = fast = headwhile fast != tail:slow = slow.nextfast = fast.nextif fast != tail:fast = fast.nextmid = slowreturn merge(sortFunc(head, mid), sortFunc(mid, tail))def merge(head1, head2):dummyHead = ListNode(0)temp, temp1, temp2 = dummyHead, head1, head2while temp1 and temp2:if temp1.val <= temp2.val:temp.next = temp1temp1 = temp1.nextelse:temp.next = temp2temp2 = temp2.nexttemp = temp.nextif temp1:temp.next = temp1elif temp2:temp.next = temp2return dummyHead.nextreturn sortFunc(head, None)