目录
求字符串长度:
1. strlen(字符串长度)
长度不受限制函数:
2. strcpy(字符串拷贝)
3. strcat(字符串追加)
4. strcmp(字符串比较)
长度受限制函数:
5. strncpy(字符串拷贝)
6. strncat(字符串追加)
7. strncmp(字符串比较)
字符串查找:
8. strstr(查找字符串子串)
9. strtok(字符串分割)
错误信息报告:
10. strerror(返回错误信息)
字符操作函数:
字符转换:
1. tolower(小写->大写)
2. toupper(大写->小写)
内存操作函数:
1. memcpy(内存拷贝)
2. memmove(内存拷贝)
3. memcmp(内存比较)
4. memset(内存设置)
求字符串长度:
1. strlen(字符串长度)
size_t strlen ( const char * str );str:C 字符串。返回值:unsigned int.
#include <stdio.h>
#include <string.h>int main()
{if ((int)strlen("abc") - (int)strlen("abcdef") > 0){printf("大于\n");}else{printf("小于等于\n");}return 0;
}
长度不受限制函数:
2.strcpy(字符串拷贝)
char* strcpy(char * destination, const char * source );destinatiob:指向要在其中复制内容的目标 数组的指针。source:要复制的 C 字符串。
#include <stdio.h>
#include <string.h>int main()
{//char arr1[3] = "";//char arr2[] = "hello bit";char* arr1 = "xxxxxxxxxx";char arr2[6] = { 'a', 'b', 'c', 'd', 'e' , '\0'};strcpy(arr1, arr2);printf("%s\n", arr1);return 0;
}
3.strcat(字符串追加)
char * strcat ( char * destination, const char * source );destination:指向目标数组的指针,该数组应包含 C 字符串,并且足够大以包含串联的结果字符串。source: 要追加的 C 字符串。这不应与 目标 重叠。
#include <stdio.h>
#include <string.h>int main()
{char arr1[20] = "hello ";char arr2[] = "world";strcat(arr1, arr2);printf("%s\n", arr1);return 0;
}
4. strcmp(字符串比较)
int strcmp ( const char * str1, const char * str2 );str1:要比较的 C1 字符串。str2:要比较的 C2 字符串。
#include <stdio.h>
#include <string.h>int main()
{int ret = strcmp("bbq", "bcq");if (ret>0)printf(">\n");printf("%d\n", ret);return 0;
}
长度受限制函数:
5. strncpy(字符串拷贝)
char * strncpy ( char * destination, const char * source, size_t num );destination:指向要在其中复制内容的目标数组的指针。source:要复制的 C 字符串。num :要从 源复制的最大字符数;size_t 是无符号整数类型。
#include <stdio.h>
#include <string.h>int main()
{char arr1[20] = "abcdef";char arr2[] = "xxx";strncpy(arr1, arr2, 5);return 0;
}
6. strncat(字符串追加)
char * strncat ( char * destination, const char * source, size_t num );destination:指向目标数组的指针,该数组应包含一个 C 字符串,并且足够大以包含串联的结果字符串,包括其他 null 字符。source:要追加的 C 字符串。num: 要追加的最大字符数。size_t是无符号整数类型。
#include <stdio.h>
#include <string.h>int main()
{char arr1[20] = "abcdef\0yyyyyyyy";char arr2[] = "xxxxxxxxx";strncat(arr1, arr2, 3);return 0;
}
7. strncmp(字符串比较)
int strncmp ( const char * str1, const char * str2, size_t num );str1:要比较的 C1 字符串。str2:要比较的 C2 字符串。num:要比较的最大字符数。
size_t是无符号整数类型。
#include <stdio.h>
#include <string.h>int main()
{char arr1[] = "abcqwertyuiop";char arr2[] = "abcdef";printf("%d\n", strncmp(arr1, arr2, 4));return 0;
}
字符串查找:
8. strstr(查找字符串子串)
char * strstr ( const char *str1, const char * str2);str1:要扫描的 C 字符串。str2:包含要匹配的字符序列的 C 字符串。
#include <stdio.h>
#include <string.h>int main()
{char arr1[] = "abbbcdef";char arr2[] = "bbc";char* ret = strstr(arr1, arr2);if (ret != NULL)printf("%s\n", ret);elseprintf("找不到\n");return 0;
}
9. strtok(字符串分割)
char * strtok ( char * str, const char * sep );str:要截断的 C 字符串。请注意,此字符串是通过分解为较小的字符串(标记)来修改的。或者,可以指定空指针,在这种情况下,函数将继续扫描以前成功调用函数的位置。sep :包含分隔符字符的 C 字符串。这些可能因调用而异。
#include <stdio.h>
#include <string.h>int main()
{char arr[] = "zpengwei@yeah.net@666#777";char copy[30];strcpy(copy, arr);char sep[] = "@.#";char* ret = NULL;for (ret = strtok(copy, sep); ret != NULL; ret=strtok(NULL, sep)){printf("%s\n", ret);}return 0;
}
错误信息报告:
10. strerror(返回错误信息)
char * strerror ( int errnum );errnum :错误号。库函数在执行的时候,发生了错位会将一个错误码存放errno这个变量中errno是C语言提供的一个全局的变量。
#include <stdio.h>
#include <string.h>int main()
{int i = 0;for (i = 0; i < 10; i++){printf("%d: %s\n", i, strerror(i));//}return 0;
}
字符操作函数:
函数 | 如果他的参数符合下列条件就返回真 |
---|---|
iscntrl | 任何控制字符 |
isspace | 空白字符:空格 ‘ ’ ,换页 ‘\f’ ,换行 '\n' ,回车 ‘\r’ ,制表符 '\t' 或者垂直制表符 '\v' |
isdigit | 十进制数字 0~9 |
isxdigit | 十六进制数字,包括所有十进制数字,小写字母 a~f ,大写字母 A~F |
islower | 小写字母 a~z |
isupper | 大写字母 A~Z |
isalpha | 字母 a~z 或 A~Z |
isalnum | 字母或者数字, a~z,A~Z,0~9 |
ispunct | 标点符号,任何不属于数字或者字母的图形字符(可打印) |
isgraph | 任何图形字符 |
isprint | 任何可打印字符,包括图形字符和空白字 |
字符转换:
1.towlower(小写->大写)
int tolower ( int c );
c:要转换、转换为 int 或 EOF 的字符。
#include <stdio.h>
#include <ctype.h>int main()
{printf("%c\n", tolower('A'));printf("%c\n", tolower('s'));return 0;
}
2. toupper(大写->小写)
int toupper ( int c );
c:要转换、转换为 int 或 EOF 的字符。
#include <stdio.h>
#include <ctype.h>int main()
{char arr[20] = { 0 };gets(arr);//遇到空格继续读char* p = arr;while (*p){if (isupper(*p))// *p>='A' && *p<='Z'{*p = tolower(*p);//*p = *p+32;}p++;}printf("%s\n", arr);return 0;
}
内存操作函数:
1. memcpy(内存拷贝)
void * memcpy ( void * destination, const void * source, size_t num );destination:指向要在其中复制内容的目标数组的指针,类型转换为 void* 类型的指针。source:指向要复制的数据源的指针,类型转换为 const void* 类型的指针。num :要复制的字节数。
size_t 是无符号整数类型。
#include <stdio.h>
#include <string.h>int main()
{int arr1[] = { 1,2,3,4,5,6,7,8,9,10 };int arr2[20] = { 0 };//将arr1中的内容,拷贝到arr2中memcpy(arr2, arr1, 40);int* int*int i = 0;for (i = 0; i < 20; i++){printf("%d ", arr2[i]);}return 0;
}
2. mommove(内存拷贝)
void * memmove ( void * destination, const void * source, size_t num );destination:指向要在其中复制内容的目标数组的指针,类型转换为 void* 类型的指针。source:指向要复制的数据源的指针,类型转换为 const void* 类型的指针。num : 要复制的字节数。size_t 是无符号整数类型。
#include <stdio.h>
#include <string.h>int main()
{int arr1[] = { 1,2,3,4,5,6,7,8,9,10 };// 1 2 1 2 3 4 5 8 9 10memmove(arr1, arr1+2, 20);int i = 0;for (i = 0; i < 10; i++){printf("%d ", arr1[i]);}return 0;
}
3. memcmp(内存比较)
int memcmp ( const void * ptr1, const void * ptr2, size_t num );ptr1:指向内存块的指针。ptr2:指向内存块的指针。num :要比较的字节数。
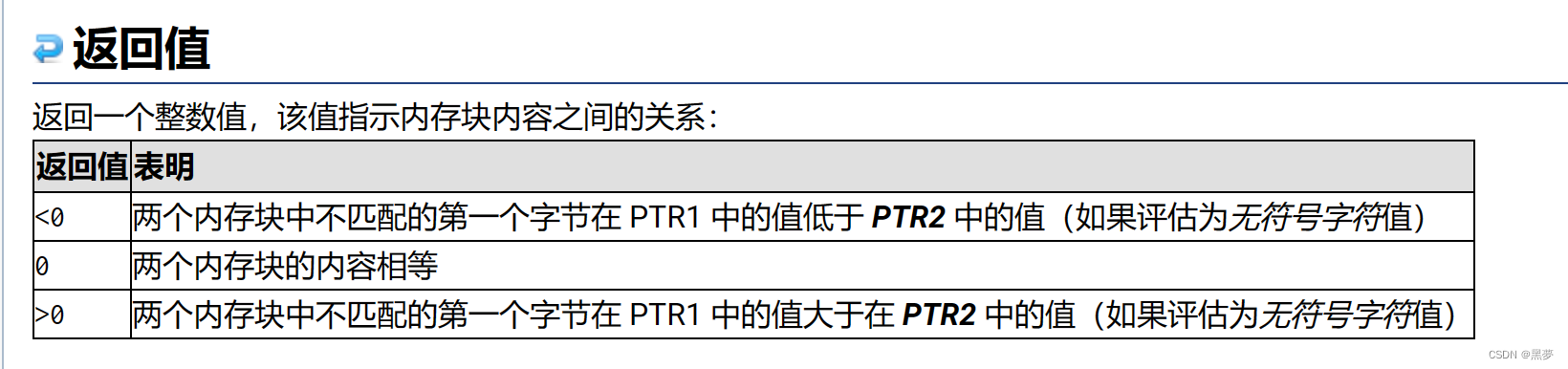
#include <stdio.h>
#include <string.h>int main()
{int arr1[] = { 1,2,1,4,5,6 };int arr2[] = { 1,2,257 };int ret = memcmp(arr1, arr2, 10);printf("%d\n", ret);return 0;
}
4. memset(内存设置)
void * memset ( void * ptr1, int value, size_t num );
ptr1:指向要填充的内存块的指针。
value:要设置的值。该值作为 int 传递,但该函数使用此值的无符号 char 转换填充内存块。
num :要设置为该值的字节数。
size_t 是无符号整数类型。
#include <stdio.h>
#include <string.h>int main()
{char arr[] = "hello bit";memset(arr+1,'x',4);//以字节为单位设置的printf("%s\n", arr);return 0;
}
以上就是个人学习见解和学习的解析,欢迎各位大佬在评论区探讨!
感谢大佬们的一键三连! 感谢大佬们的一键三连! 感谢大佬们的一键三连!