1、编写模板
<el-form ref="form" label-width="100px"><el-form-item label="商品id:"><el-input v-model="id" disabled></el-input></el-form-item><el-form-item label="商品名称:"><el-input v-model="name" placeholder="请输入内容"></el-input></el-form-item><el-form-item label="商品价格:"><el-input-number v-model="price" :step="10" :min="10" :max="100"></el-input-number> </el-form-item><el-form-item><el-button size="small" type="success" @click="editGoods">保存</el-button> </el-form-item>
</el-form>
2、前端发请求
editGoods() {if (this.id == '' && this.id == null) {this.$message({message: 'id不能为空',type: 'error'})return;} else if (this.name == '' && this.name == null) {this.$message({message: '名称不能为空',type: 'error'})return;}this.$axios({method: 'post',url: 'http://localhost:8080/api/upload/editGoods',data: {id: this.id,name: this.name,price: this.price}}).then((res) => {this.$message({message: '修改成功',type: 'success'})})}
3、后端返回数据
1.编写实体类
package com.example.goods_admin.entity;public class Goods extends Page {private String id;private String name;private int price;private String imageUrl;private String status;public Goods() {super();}public Goods(int pageNum, int pageSize, String keyWord) {super(pageNum, pageSize, keyWord);}public Goods(int pageNum, int pageSize, String keyWord, String id, String name, int price, String imageUrl, String status) {super(pageNum, pageSize, keyWord);this.id = id;this.name = name;this.price = price;this.imageUrl = imageUrl;this.status = status;}public String getId() {return id;}public void setId(String id) {this.id = id;}public String getName() {return name;}public void setName(String name) {this.name = name;}public int getPrice() {return price;}public void setPrice(int price) {this.price = price;}public String getImageUrl() {return imageUrl;}public void setImageUrl(String imageUrl) {this.imageUrl = imageUrl;}public String getStatus() {return status;}public void setStatus(String status) {this.status = status;}
}
2.Controller类
@RestController
@RequestMapping("/upload")
public class UploadFileController {@AutowiredUploadFileService uploadFileService;//json传参@GetMapping("/editGoods")public Result editGoods(@RequestBody Goods goods) {return uploadFileService.editGoods(goods);}
}
3、interface接口(Service层接口)
public interface UploadFileService {Result editGoods(Goods goods);
}
4.Service(接口实现)
@Service
public class UploadFileServiceImpl implements UploadFileService {@AutowiredUploadFileMapper uploadFileMapper;@Overridepublic Result editGoods(Goods goods) {int count=uploadFileMapper.updateGoods(goods);if (count==1){return Result.succeed("删除成功");}else{return Result.failed("删除失败");}}}
5、interface接口(Mapper层接口)
public interface UploadFileMapper {int saveGoods(Goods goods);}
6、xml(动态sql)
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.goods_admin.mapper.UploadFileMapper"><resultMap id="BaseResultMap" type="com.example.goods_admin.entity.Goods"><id column="id" jdbcType="VARCHAR" property="id" /><result column="name" jdbcType="VARCHAR" property="name" /><result column="price" jdbcType="INTEGER" property="price" /><result column="imageUrl" jdbcType="VARCHAR" property="imageUrl" /><result column="status" jdbcType="VARCHAR" property="status" /></resultMap><insert id="saveGoods">INSERT INTO goods (<if test="id != null and id != ''">id</if><if test="name != null and name != ''">,name</if><if test="price != null and price != ''">,price</if><if test="imageUrl != null and imageUrl != ''">,imageUrl</if><if test="status != null and status != ''">,status</if>) VALUES (<if test="id != null and id != ''">#{id}</if><if test="name != null and name != ''">,#{name}</if><if test="price != null and price != ''">,#{price}</if><if test="imageUrl != null and imageUrl != ''">,#{imageUrl}</if><if test="status != null and status != ''">,#{status}</if>)</insert>
</mapper>
7、效果
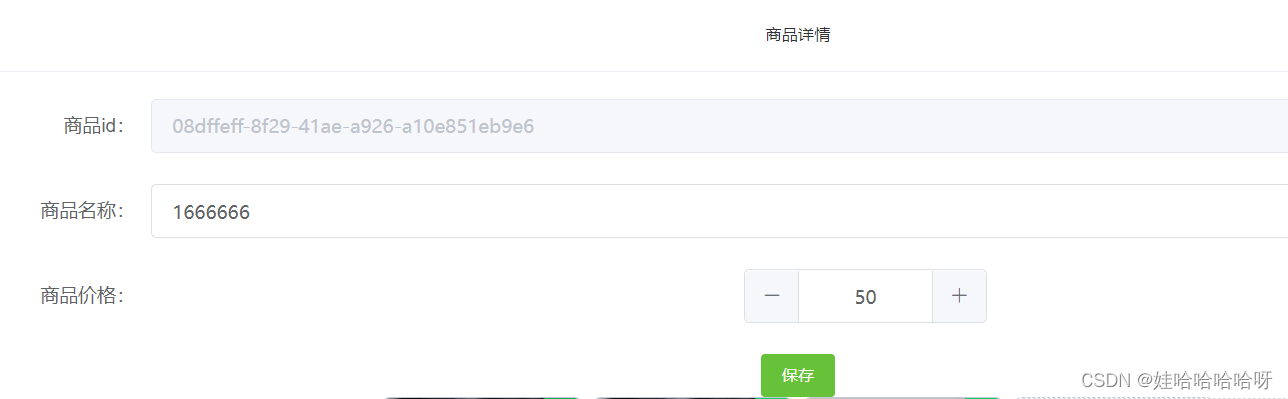
4、el-input-number相关参数和方法
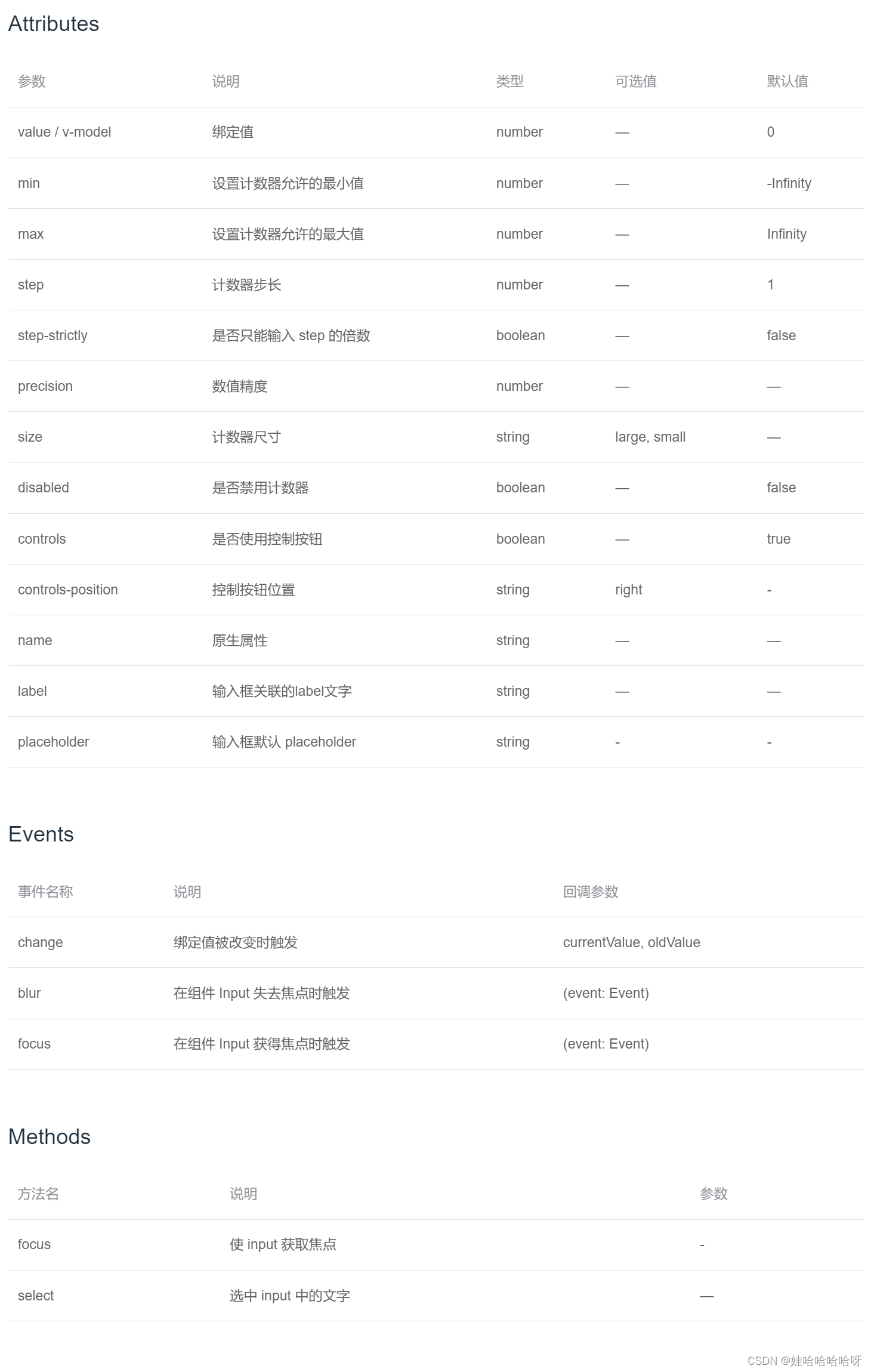