一、单链表
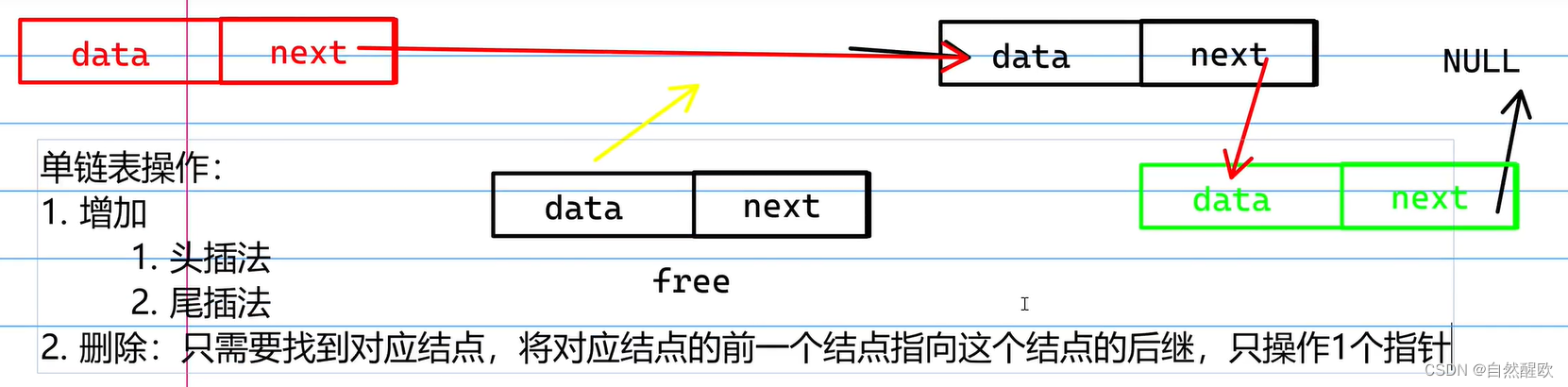
#include <stdio.h>
#include <stdlib.h>typedef struct Node {int data;Node *next;
}Node;Node* initList()
{Node *list = (Node*)malloc(sizeof(Node));list->data = 0;list->next = NULL;return list;
}void headInsert(Node* L, int data)
{Node *node = (Node*)malloc(sizeof(Node));node->data = data;node->next = L->next;L->next = node;L->data++;
}void tailInsert(Node* L, int data)
{Node *List = L;Node *node = (Node*)malloc(sizeof(Node));node->data = data;while (List->next){List = List->next;}node->next = List->next;List->next = node;L->data++;
}int delete_node(Node* L, int data)
{Node *pre = L;Node *current = L->next;while(current){if (current->data==data){pre->next = current->next;free(current);L->data--;return true;}else{pre = current;current = current->next;}}return false;
}void printList(Node* L)
{while (L->next){L = L->next;printf("node = %d\n", L->data);}
}int main()
{Node* L = initList();headInsert(L, 1);headInsert(L, 2);headInsert(L, 3);headInsert(L, 4);headInsert(L, 5);tailInsert(L, 6);tailInsert(L, 7);printList(L);if (delete_node(L, 3)) {printf("success delete\n");}else {printf("fail delete\n");}printList(L);system("pause");return 0;
}
二、单循环链表
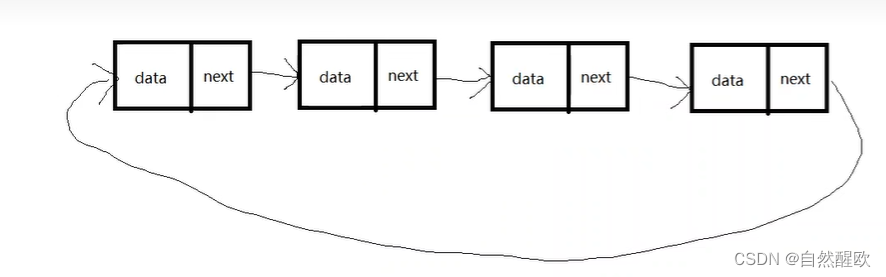
#include <stdio.h>
#include <stdlib.h>typedef struct Node {int data;Node *next;
}Node;Node* initList()
{Node *L = (Node*)malloc(sizeof(Node));L->next = NULL;L->data = 0;L->next = L;return L;
}void headInsert(Node* L, int data)
{Node *node = (Node*)malloc(sizeof(Node));node->data = data;node->next = L->next;L->next = node;L->data++;
}void tailInsert(Node* L, int data)
{Node *List = L;Node *node = (Node*)malloc(sizeof(Node));while (List->next!=L){List = List->next;}node->data = data;node->next = L;List->next = node;L->data++;
}int delete_node(Node* L, int data)
{Node *pre = L;Node *current = L->next;while (current!=L){if (current->data == data){pre->next=current->next;free(current);L->data--;return true;}else{pre = current;current = current->next;} }return false;
}void printList(Node* L)
{Node *node = L->next;while (node !=L){printf("%d->", node->data);node=node->next;}printf("NULL\n");
}
int main()
{Node* L = initList();headInsert(L, 1);headInsert(L, 2);headInsert(L, 3);headInsert(L, 4);headInsert(L, 5);tailInsert(L, 6);tailInsert(L, 7);printList(L);delete_node(L, 4);delete_node(L, 7);printList(L);system("pause");return 0;
}