#include <stdio.h>
#include <string.h>
#include <malloc.h>typedef struct Queue {int * pBase;int front;int rear;} QUEUE;void init(QUEUE *);
bool en_queue(QUEUE *pQ, int val); //入队
void traverse_queue(QUEUE * pQ);
bool full_queue(QUEUE * pQ);bool out_queue(QUEUE *pQ, int *pVal); //出队 *pVal用来保存出队的元素
bool empty_queue(QUEUE *pQ); //判空int main(void) {QUEUE Q;int val; //保存出队元素init(&Q);en_queue(&Q, 1);en_queue(&Q, 2);en_queue(&Q, 3);en_queue(&Q, 4);en_queue(&Q, 5);en_queue(&Q, 6);en_queue(&Q, 7);en_queue(&Q, 8);traverse_queue(&Q); //遍历输出if (out_queue(&Q, &val)) { //出队printf("出队成功 出队元素 %d\n",val);//成功}else {printf("出队失败 出队元素 %d\n", val);//失败}traverse_queue(&Q); //遍历输出while (true){}return 0;
}void init(QUEUE *pQ) {pQ->pBase = (int *)malloc(sizeof(int) * 6);pQ->front = 0;pQ->rear = 0;
}//入队
bool en_queue(QUEUE *pQ, int val) {if (full_queue(pQ)) {return false;}else {pQ->pBase[pQ->rear] = val;pQ->rear=(pQ->rear + 1) % 6;return true;}}//判断队列是不是满了
bool full_queue(QUEUE * pQ) {if ( (pQ->rear + 1)%6==pQ->front) {return true;}else {return false;}}//遍历输出
void traverse_queue(QUEUE *pQ) {int i=pQ->front;while (i != pQ->rear) {printf("%d ", pQ->pBase[i]);i = (i + 1) % 6;}printf("\n");return;
}//出队
bool out_queue(QUEUE *pQ, int *pVal) {if (empty_queue(pQ)) {return false; }else {*pVal = pQ->pBase[pQ->front]; //队列从头部出队,保存头部出队的元素pQ->front = (pQ->front + 1) % 6;}
}//队列判空
bool empty_queue(QUEUE *pQ) {if (pQ->front == pQ->rear) {return true;}else {return false;}}
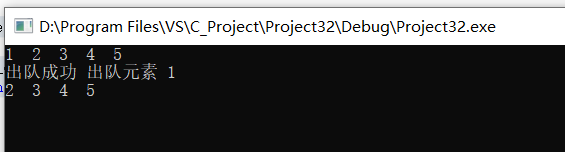