#include<iostream>
#include<vector>
#include<stack>
using namespace std;
// Definition for binary tree 先序遍历 根左右
struct TreeNode {int val;TreeNode *left;TreeNode *right;TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
#if 0
class Solution { //me ok
public:vector<int> v;vector<int> postorderTraversal(TreeNode *root) {if (root == NULL)return v;if ((*root).left != NULL)postorderTraversal((*root).left);if ((*root).right != NULL)postorderTraversal((*root).right);v.push_back((*root).val);return v;}
};
#endif#if 0
class Solution { //因为这是栈结构,先进后出,所以根左右进,根右左出。
public:vector<int> postorderTraversal(TreeNode *root){vector<int> res;if (!root)return res;stack<TreeNode *> st;st.push(root);while (st.size()){TreeNode *temp = st.top();st.pop();res.push_back(temp->val);if (temp->left)st.push(temp->left);if (temp->right)st.push(temp->right);}reverse(res.begin(), res.end());return res;}
};
#endif#if 1 //非迭代
class Solution {
public:vector<int> v;stack<TreeNode*> s;vector<int> postorderTraversal(TreeNode *root) {if (root == NULL) {return v;}s.push(root);TreeNode *cur = NULL;while (!s.empty()) {cur = s.top();if (cur->left == NULL && cur->right == NULL){s.pop();v.push_back(cur->val);}else {if (cur->right != NULL){s.push(cur->right);cur->right = NULL;}if (cur->left != NULL) {s.push(cur->left);cur->left = NULL;}}}return v;}
};
#endif
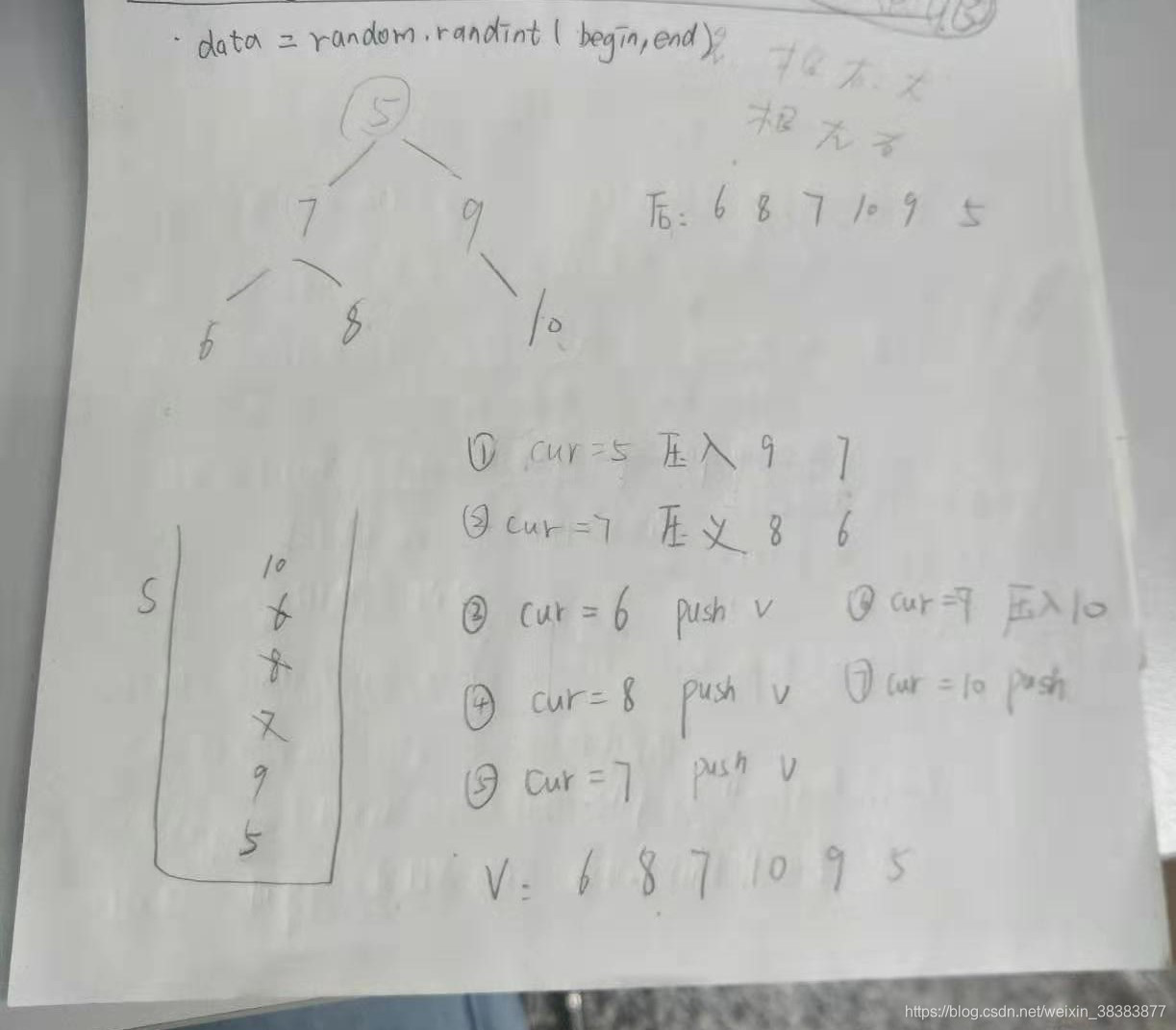