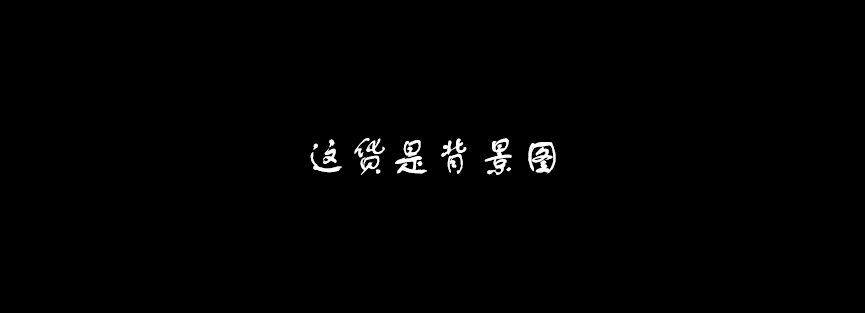
POI是一个可以对excel文件进行操作的jar包,使用它可以帮助我们对excel进行操作,也就可以帮助我们实现在jsp页面添加导入数据的功能。只要我们在控制层servlet中加入处理的方法就可以了;首先使用到POI都会与JXL进行对比:查阅之后大致是这样的
一、jxl优点:Jxl对中文支持非常好,操作简单,方法看名知意。
Jxl是纯javaAPI,在跨平台上表现的非常完美。 支持字体、数字、日期操作,能够修饰单元格属性, 支持图像和图表,但是这套API对图形和图表的支持很有限,而且仅仅识别PNG格式。缺点:效率低,图片支持不完善,对格式的支持不如POI强大
二、POI优点:效率高。支持公式,宏,一些企业应用上会非常实用 ,能够修饰单元格属性。 支持字体、数字、日期操作。缺点:不成熟,代码不能跨平台。
是具体使用那个包,看你使用的具体情况来定。下面是POI工具类的使用
POI工具类为:
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFCellStyle;
import org.apache.poi.hssf.usermodel.HSSFFont;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.hssf.util.HSSFColor;
import org.apache.poi.ss.util.CellRangeAddress;
/*OutputStream out = response.getOutputStream();//response.reset();response.setContentType("application/vnd.ms-excel");response.setHeader("Content-disposition", "attachment; fileName=" + new String(("duty.xls").getBytes(), "iso8859-1"));// OutputStream outputStream = new FileOutputStream("D:/students.xls");workbook.write(out);out.close();*/
public class ExcelOperate {public static void main(String[] args) {// 创建Excel表格createExcel(getStudent());// 读取Excel表格// List<Student> list = readExcel();// System.out.println(list.toString());}/*** 初始化数据* * @return 数据*/private static List<Student> getStudent() {List<Student> list = new ArrayList<Student>();Student student1 = new Student("小明", 8, "二年级");Student student2 = new Student("小光", 9, "三年级");Student student3 = new Student("小花", 10, "四年级");list.add(student1);list.add(student2);list.add(student3);return list;}/*** 创建Excel* * @param list* 数据*/private static void createExcel(List<Student> list) {// 创建一个Excel文件HSSFWorkbook workbook = new HSSFWorkbook();// 创建一个工作表HSSFSheet sheet = workbook.createSheet("学生表一");CellRangeAddress region = new CellRangeAddress(0, // first row0, // last row0, // first column2 // last column);sheet.addMergedRegion(region);HSSFRow hssfRow = sheet.createRow(0);HSSFCell headCell = hssfRow.createCell(0);headCell.setCellValue("学生列表");// 设置单元格格式居中HSSFCellStyle cellStyle = workbook.createCellStyle();cellStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER);/*cellStyle.setFillPattern(HSSFCellStyle.SOLID_FOREGROUND);// cellStyle.setFillBackgroundColor(HSSFColor.GREEN.index);cellStyle.setFillForegroundColor(HSSFColor.GREEN.index);HSSFFont font = workbook.createFont();font.setFontName("楷体"); //字体font.setFontHeightInPoints((short)30); //字体大小font.setColor(HSSFColor.RED.index);//颜色font.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD);//加粗font.setItalic(true); //倾斜cellStyle.setFont(font);*/headCell.setCellStyle(cellStyle);// 添加表头行hssfRow = sheet.createRow(1);// 添加表头内容headCell = hssfRow.createCell(0);headCell.setCellValue("姓名");headCell.setCellStyle(cellStyle);headCell = hssfRow.createCell(1);headCell.setCellValue("年龄");headCell.setCellStyle(cellStyle);headCell = hssfRow.createCell(2);headCell.setCellValue("年级");headCell.setCellStyle(cellStyle);// 添加数据内容for (int i = 0; i < list.size(); i++) {hssfRow = sheet.createRow((int) i + 2);Student student = list.get(i);// 创建单元格,并设置值HSSFCell cell = hssfRow.createCell(0);cell.setCellValue(student.getName());cell.setCellStyle(cellStyle);cell = hssfRow.createCell(1);cell.setCellValue(student.getAge());cell.setCellStyle(cellStyle);cell = hssfRow.createCell(2);cell.setCellValue(student.getGrade());cell.setCellStyle(cellStyle);}// 保存Excel文件try {OutputStream outputStream = new FileOutputStream("D:/students.xls");workbook.write(outputStream);outputStream.close();} catch (Exception e) {e.printStackTrace();}}/*** 读取Excel* * @return 数据集合*/private static List<Student> readExcel() {List<Student> list = new ArrayList<Student>();HSSFWorkbook workbook = null;try {// 读取Excel文件InputStream inputStream = new FileInputStream("D:/students.xls");workbook = new HSSFWorkbook(inputStream);inputStream.close();} catch (Exception e) {e.printStackTrace();}// 循环工作表for (int numSheet = 0; numSheet < workbook.getNumberOfSheets(); numSheet++) {HSSFSheet hssfSheet = workbook.getSheetAt(numSheet);if (hssfSheet == null) {continue;}// 循环行for (int rowNum = 2; rowNum <= hssfSheet.getLastRowNum(); rowNum++) {HSSFRow hssfRow = hssfSheet.getRow(rowNum);if (hssfRow == null) {continue;}// 将单元格中的内容存入集合Student student = new Student();HSSFCell cell = hssfRow.getCell(0);if (cell == null) {continue;}student.setName(cell.getStringCellValue());cell = hssfRow.getCell(1);if (cell == null) {continue;}student.setAge((int) cell.getNumericCellValue());cell = hssfRow.getCell(2);if (cell == null) {continue;}student.setGrade(cell.getStringCellValue());list.add(student);}}return list;}
}class Student {private String name;private int age;private String grade;public Student() {}public Student(String name, int age, String grade) {super();this.name = name;this.age = age;this.grade = grade;}public String getName() {return name;}public void setName(String name) {this.name = name;}public int getAge() {return age;}public void setAge(int age) {this.age = age;}public String getGrade() {return grade;}public void setGrade(String grade) {this.grade = grade;}@Overridepublic String toString() {return "Student [name=" + name + ", age=" + age + ", grade=" + grade+ "]";}
}
这个里面是具体的用法,与对一个学生列表实现的案例;使用该工具我们只要在servelt中调用工具类的创建excel与实现excel的方法即可;就像:
DutyService ds=new DutyServiceImpl();List<Duty> dutyList=ds.findBy(empId,deptno,dtDate);//返回输出流createExcel(dutyList,resp);
我将查询返回到的列表传输到创建excel的方法,并且对excel的方法进行了重写,在与前台页面进行交互的时候,因为要对客户端进行写入操作,而不是对服务器端进行操作,所以我将response也传入进去,因为我们不能简单对返回的数据进行重定向或者请求转发操作,而是以流的方式写回去
try {resp.setContentType("application/vnd.ms-excel");//声明导出excelresp.setHeader("Content-disposition", "attachment;filename=duty.xls");//附件形式下载包括名字//OutputStream outputStream = new FileOutputStream("D:/kaoqin.xls");ServletOutputStream outputStream = resp.getOutputStream();workbook.write(outputStream);outputStream.close();} catch (Exception e) {e.printStackTrace();}