Modules Used:
使用的模块:
python-opencv(cv2)
python-opencv(cv2)
python-opencv(cv2)
Opencv(Open source computer vision) is a python library that will help us to solve computer vision problems.
Opencv(开源计算机视觉)是一个Python库,可帮助我们解决计算机视觉问题。
Download python-opencv(cv2)
下载python-opencv(cv2)
General Way: pip install python-opencv
通用方式: pip install python-opencv
Pycharm users: Pycharm users can download this module from the project interpreter.
Pycharm用户:Pycharm用户可以从项目解释器下载此模块。
Here, we will detect the face and eyes of the individual. For this, we use the webcam of our system and the XML files to detect the face and eyes. We will detect the face in the frame and after that eyes are in the face so we will enter into the coordinates of the face and will detect the eyes and draw the rectangle on the face and eye detected.
在这里,我们将检测个人的脸和眼睛 。 为此,我们使用系统的网络摄像头和XML文件来检测面部和眼睛。 我们将在帧中检测到面部,然后将眼睛插入面部,因此我们将进入面部坐标并检测眼睛,并在检测到的面部和眼睛上绘制矩形。
Functions related the face and eye detection
与面部和眼睛检测相关的功能
cv2.CascadeClassifier("<xml file for detection>"): This function is for getting the face and eyes extracts, how we will detect them.
cv2.CascadeClassifier(“ <用于检测的xml文件>”):此函数用于获取面部和眼睛的提取物,以及我们将如何检测它们。
cv2.Videocapture(): This is for the Videocapturing through the webcam of our system.
cv2.Videocapture():用于通过我们系统的网络摄像头进行视频捕获。
<face or eye extract>.detectmultiscale(<Gray_scale image>,1.3,5): To detect the the face or eyes in the frame.
<面部或眼睛提取物> .detectmultiscale(<灰度图像>,1.3,5):检测帧中的面部或眼睛。
cv2.rectangle(<frame on which we want the rectangle>,(<starting position>,<ending position>,(<color>),thickness=<thickness of the border>)
cv2.rectangle(<我们要在其上放置矩形的框架>,(<开始位置>,<结束位置>,(<颜色>),厚度= <边框的厚度>)
Note: The detection of the face and eyes will be in grayscale mode.
注意:面部和眼睛的检测将处于灰度模式。
The link to the XML files for the face and eye detection is :
用于面部和眼睛检测的XML文件的链接是:
Face : https://github.com/abhinav0606/Face-and-Eyes-Tracker/blob/master/face.xml
人脸: https : //github.com/abhinav0606/Face-and-Eyes-Tracker/blob/master/face.xml
Eye: https://github.com/abhinav0606/Face-and-Eyes-Tracker/blob/master/eye.xml
眼睛: https : //github.com/abhinav0606/Face-and-Eyes-Tracker/blob/master/eye.xml
用于检测人脸和眼睛的Python代码 (Python code to detect face and eyes)
# import the modules
import cv2
# now we have the haarcascades files
# to detect the face and eyes to detect the face
faces=cv2.CascadeClassifier("face.xml")
# to detect the eyes
eyes=cv2.CascadeClassifier("eye.xml")
# capture the frame through webcam
capture=cv2.VideoCapture(0)
# now running the loop for the webcam
while True:
# reading the webcam
ret,frame=capture.read()
# now the face is in the frame
# the detection is done with the gray scale frame
gray_frame=cv2.cvtColor(frame,cv2.COLOR_BGR2GRAY)
face=faces.detectMultiScale(gray_frame,1.3,5)
# now getting into the face and its position
for (x,y,w,h) in face:
# drawing the rectangle on the face
cv2.rectangle(frame,(x,y),(x+w,y+h),(0,0,255),thickness=4)
# now the eyes are on the face
# so we have to make the face frame gray
gray_face=gray_frame[y:y+h,x:x+w]
# make the color face also
color_face=frame[y:y+h,x:x+w]
# check the eyes on this face
eye=eyes.detectMultiScale(gray_face,1.3,5)
# get into the eyes with its position
for (a,b,c,d) in eye:
# we have to draw the rectangle on the
# coloured face
cv2.rectangle(color_face,(a,b),(a+c,b+d),(0,255,0),thickness=4)
# show the frame
cv2.imshow("Abhinav's Frame",frame)
if cv2.waitKey(1)==13:
break
# after ending the loop release the frame
capture.release()
cv2.destroyAllWindows()
Output:
输出:
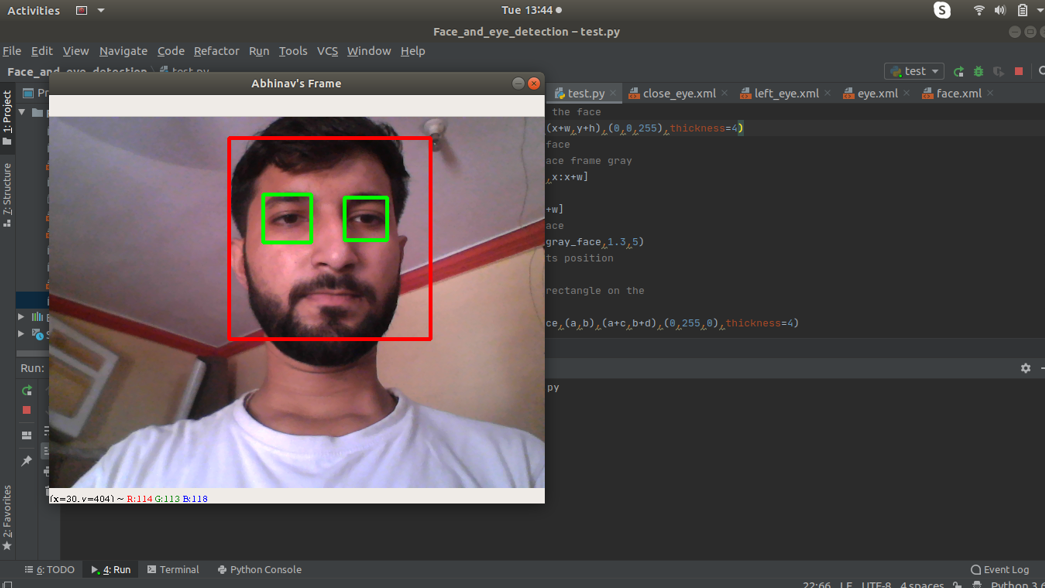
翻译自: https://www.includehelp.com/python/face-and-eye-detection-in-python-using-opencv.aspx