将搜索二叉树转换为链表
Given a Binary tree and we have to convert it to a Doubly Linked List (DLL).
给定二叉树,我们必须将其转换为双链表(DLL)。
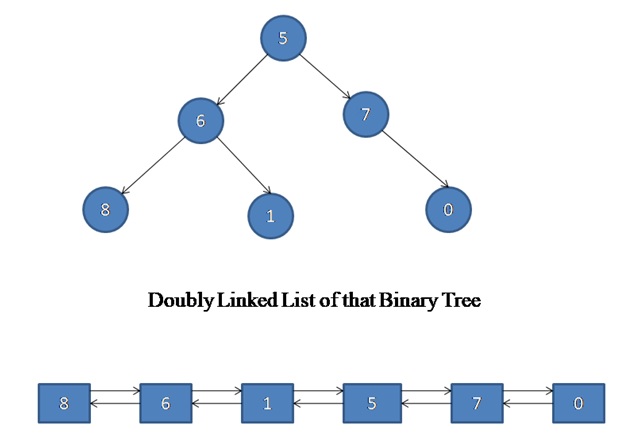
Algorithm:
算法:
To solve the problem we can follow this algorithm:
为了解决这个问题,我们可以遵循以下算法:
We start to convert the tree node to DLL from the rightmost tree node to the leftmost tree node.
我们开始从最右边的树节点到最左边的树节点将树节点转换为DLL。
Every time we connect the right pointer of a node to the head of the DLL.
每次我们将节点的右指针连接到DLL的头时。
Connect the left pointer of the DLL to that node.
将DLL的左指针连接到该节点。
Make that node to the head of the linked list.
使该节点位于链接列表的开头。
Repeat the process from right to left most node of the tree.
从树的最右端到最左端重复该过程。
C++ implementation:
C ++实现:
#include <bits/stdc++.h>
using namespace std;
struct node {
int data;
node* left;
node* right;
};
//Create a new node
struct node* create_node(int x)
{
struct node* temp = new node;
temp->data = x;
temp->left = NULL;
temp->right = NULL;
return temp;
}
//convert a BST to a DLL
void BinarytoDll(node* root, node** head)
{
if (root == NULL)
return;
BinarytoDll(root->right, head);
root->right = *head;
if (*head != NULL) {
(*head)->left = root;
}
*head = root;
BinarytoDll(root->left, head);
}
//Print the list
void print(node* head)
{
struct node* temp = head;
while (temp) {
cout << temp->data << " ";
temp = temp->right;
}
}
//print the tree in inorder traversal
void print_tree(node* root)
{
if (root == NULL) {
return;
}
print_tree(root->left);
cout << root->data << " ";
print_tree(root->right);
}
int main()
{
struct node* root = create_node(5);
root->left = create_node(6);
root->right = create_node(7);
root->left->left = create_node(8);
root->left->right = create_node(1);
root->right->right = create_node(0);
cout << "Print Tree" << endl;
print_tree(root);
struct node* head = NULL;
BinarytoDll(root, &head);
cout << "\nDoubly Linked List" << endl;
print(head);
return 0;
}
Output
输出量
Print Tree
8 6 1 5 7 0
Doubly Linked List
8 6 1 5 7 0
翻译自: https://www.includehelp.com/cpp-programs/convert-a-given-binary-tree-to-doubly-linked-list-dll.aspx
将搜索二叉树转换为链表