点击上方"蓝字",关注了解更多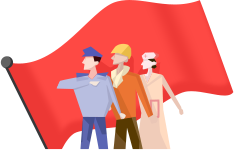
精彩推荐
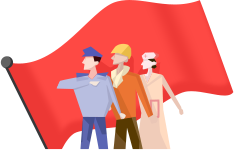
二维数组中的查找
1. 题目描述
在一个二维数组中(每个一维数组的长度相同),每一行都按照从左到右递增的顺序排序,每一列都按照从上到下递增的顺序排序。请完成一个函数,输入这样的一个二维数组和一个整数,判断数组中是否含有该整数。
2. 示例
现有矩阵 matrix 如下:
[ [1, 4, 7, 11, 15],
[2, 5, 8, 12, 19],
[3, 6, 9, 16, 22],
[10, 13, 14, 17, 24],
[18, 21, 23, 26, 30] ]
给定 target = 5,返回 true。
给定 target = 20,返回 false。
3. 解题思路
使用以下方法的前提是:该矩阵是有序的。
从矩阵的左下角开始搜索,即从18 开始查找,如果该数大于目标数,则 行数 减去 1,向上搜索小的数值;
如果小于目标数,则 列数 + 1 ,向右边搜索,搜索更大的数值
4. Java实现
// Java语言实现
public class Solution {
public boolean Find(int target, int [][] array) {
int row = array.length-1;
int col = 0;
// 从左下角开始搜索,array.length 表示行的大小,array[0].length表示列的大小
while (row >= 0 && col <= array[0].length-1){
if (array[row][col] == target){
return true;
}else if(array[row][col] > target){
row--;
}else{
col++;
}
}
return false;
}
}
5. Python实现
class Solution(object):
def searchMatrix(self, matrix, target):
"""
:type matrix: List[List[int]]
:type target: int
:rtype: bool
"""
if not matrix:
return False
rows, cols = len(matrix), len(matrix[0])
#从左下角开始搜索
row, col = rows - 1, 0
while row >= 0 and col <= cols-1:
if matrix[row][col] == target:
return True
elif matrix[row][col] col += 1
else:
row -= 1 # row一直减少
return False
如果您觉得本文有用,请点个“在看”

Java开发微服务畅购商城实战-全357集【附代码课件】
Java微服务实战谷粒商城-296集【附代码课件】
Spring Boot开发小而美的个人博客【附课件和源码】
基于Python flask框架的租房项目实战【附资料和源码】
PyQt5开发与实战视频【附课件和源码】
最全最详细数据结构与算法视频-【附课件和源码】
2020年微信小程序全栈项目之喵喵交友【附课件和源码】
你点的每一个在看,我都认真当成了喜欢