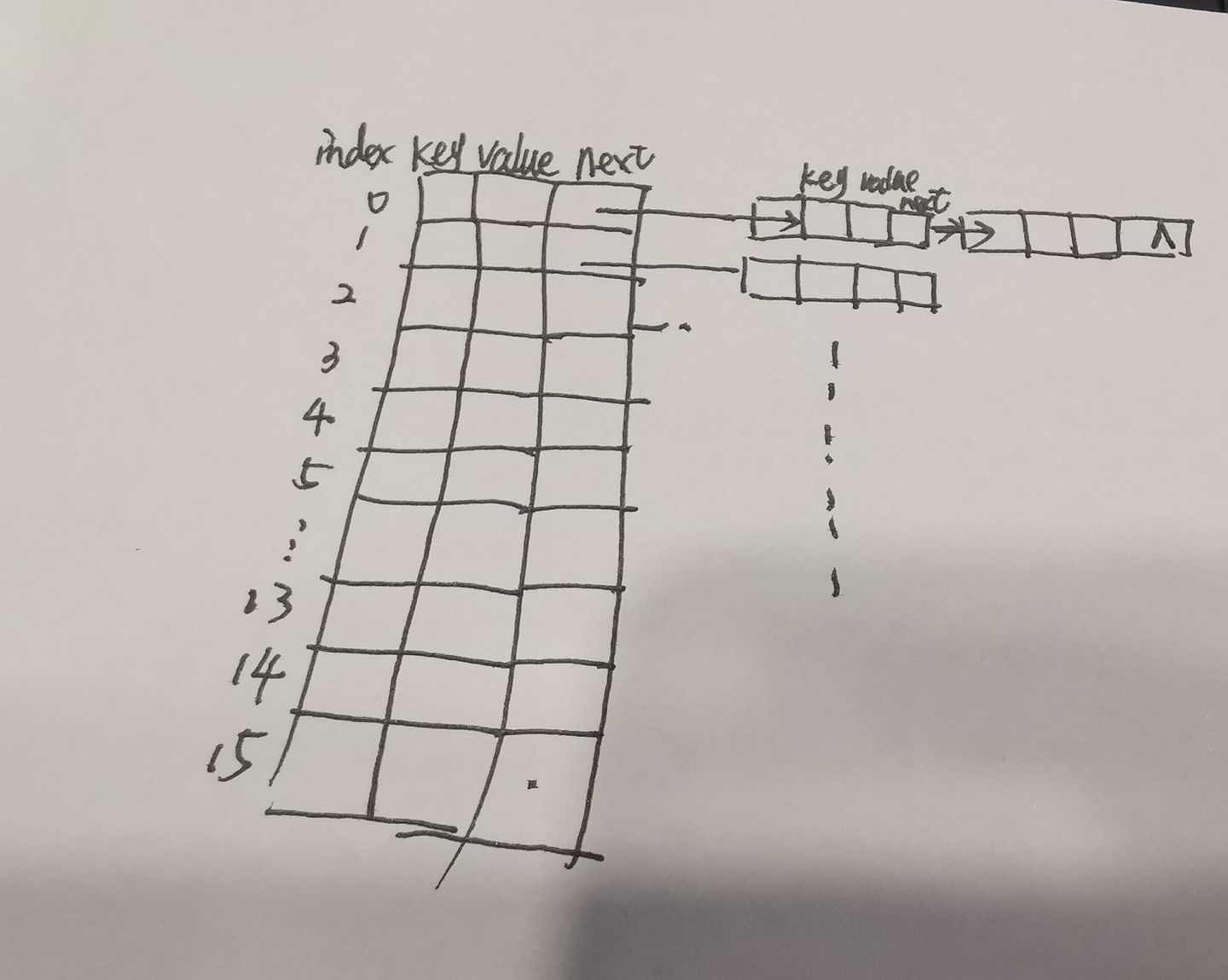
哈希表的主要用处:快速的数据存储和查找。例如,在我们日常上网过程中搜索某条信息的时候,信息的存储方式决定了查找该信息的速度,哈希表结合了数组的便捷访问和链表的便捷查找和删除的特点。
实现方式:通过哈希函数获取哈希表的地址,遇到冲突的情况下采用拉链法解决冲突。
时间复杂度O(1)
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
/*定义哈希表数组的长度*/
#define BUCKETCOUNT 16
/*哈希表结点数据结构定义*/
struct hashEntry
{
char *key;
char *value;
struct hashEntry *next;
};
typedef struct hashEntry entry;
struct hashTable
{
entry bucket[BUCKETCOUNT];/*先默认定义16个桶*/
};
typedef struct hashTable table;
/*初始化哈希表*/
void initHashTable(table *t)
{
int i;
if(!t)
return;
for(i = 0; i < BUCKETCOUNT; ++i)
{
t->bucket[i].key = NULL;
t->bucket[i].next = NULL;
t->bucket[i].value = NULL;
}
}
/*散列函数*/
int keyToIndex(const char *key)
{
int index, len, i;
if(key == NULL)
return -1;
len = strlen(key);
index = (int)key[0];
for(i = 1; i < len; ++i)
{
index *= 1103515245 + (int)key[i];
}
index >>= 27;
index &= (BUCKETCOUNT - 1);
return index;
}
/*在堆上分配足以保存str的内存,并拷贝str的内容到新分配的位置*/
char *strDup(const char *str)
{
int len;
char *ret;
if(!str)
return NULL;
len = strlen(str);
ret = (char *)malloc(sizeof(len + 1));
if(!ret)
return NULL;
memcpy(ret, str, len);
ret[len] = '0';
return ret;
}
/*插入数据到hash表中*/
int insertEntry(table *t, const char *key, const char *value)
{
int index, vlen1, vlen2;
entry *e, *ep;
if(t == NULL || key == NULL || value == NULL)
return -1;
index = keyToIndex(key);
if(!t->bucket[index].key)
{
t->bucket[index].key = strDup(key);
t->bucket[index].value = strDup(value);
}
else
{
e = ep = &(t->bucket[index]);
/*先从已经存在的找*/
while(e)
{
/*找到key所在的位置,替换相应的值*/
if(strcmp(e->key, key) == 0)
{
vlen1 = strlen(value);
vlen2 = strlen(e->value);
if(vlen1 > vlen2)
{
free(e->value);
e->value = (char *)malloc(sizeof(vlen1 + 1));
}
memcpy(e->value, value, vlen1 + 1);
return index;/*插入完成*/
}
ep = e;
e = e->next;
}
/*没有在当前桶中找到,创建条目加入*/
e = (entry *)malloc(sizeof(entry));
e->key = strDup(key);
e->value = strDup(value);
e->next = NULL;
ep->next = NULL;
}
return index;
}
/*找到哈希表中key对应的entry,找到之后返回对应的entry,并将其删除*/
/*没找到返回NULL*/
entry *removeEntry(table *t, char *key)
{
int index;
entry *e, *ep;
if(!t || !key)
return NULL;
index = keyToIndex(key);
e = &(t->bucket[index]);
while(e)
{
if(strcmp(e->key, key) == 0)/*如果是桶的第一个*/
{
if(e == &(t->bucket[index]))
{
ep = e->next;
if(ep)
{
entry *tmp = e;
e = ep;
ep = tmp;
ep->next = NULL;
}
else/*这个桶只有一个元素*/
{
//ep = (entry*)malloc(sizeof(entry));
ep = e;
e->key = e->value = NULL;
e->next = NULL;
}
return ep;
}
}
else/*如果不是木桶第一个元素*/
{
//找到它的前一个
ep = t->bucket[index].next;
while((ep->key != key) && ep)
{
ep = ep->next;
}
if(!ep)
return NULL;
return ep;
}
e = e->next;
}
return NULL;
}
/*打印hash表*/
void printTable(table *t)
{
int i;
entry *e;
if(!t)
return;
for(i = 0; i < BUCKETCOUNT; i++)
{
printf("nbucket[%d]:n", i);
e = &(t->bucket[i]);
while(e)
{
printf("t%st = t%sn",e->key, e->value);
e = e->next;
}
}
}
/*释放哈希表*/
void freeHashTable(table* t)
{
int i;
entry* e,*ep;
if (t == NULL)return;
for (i = 0; i<BUCKETCOUNT; ++i) {
e = &(t->bucket[i]);
while (e->next != NULL) {
ep = e->next;
e->next = ep->next;
free(ep->key);
free(ep->value);
free(ep);
}
}
}
int main(void)
{
table t;
initHashTable(&t);
insertEntry(&t , "电脑型号" , "华硕 X550JK 笔记本电脑");
insertEntry(&t , "操作系统" , "Windows 8.1 64位 (DirectX 11)");
insertEntry(&t , "处理器" , "英特尔 Core i7 - 4710HQ @ 2.50GHz 四核");
insertEntry(&t , "主板" , "华硕 X550JK(英特尔 Haswell)");
insertEntry(&t , "内存" , "4 GB(Hynix / Hyundai)");
insertEntry(&t , "主硬盘" , "日立 HGST HTS541010A9E680(1 TB / 5400 转 / 分)");
insertEntry(&t , "显卡" , "NVIDIA GeForce GTX 850M (2 GB / 华硕)");
insertEntry(&t , "显示器" , "奇美 CMN15C4(15.3 英寸)");
insertEntry(&t , "光驱" , "松下 DVD - RAM UJ8E2 S DVD刻录机");
insertEntry(&t , "声卡" , "Conexant SmartAudio HD @ 英特尔 Lynx Point 高保真音频");
insertEntry(&t , "网卡" , "瑞昱 RTL8168 / 8111 / 8112 Gigabit Ethernet Controller / 华硕");
insertEntry(&t , "主板型号" , "华硕 X550JK");
insertEntry(&t , "芯片组" , "英特尔 Haswell");
insertEntry(&t , "BIOS" , "X550JK.301");
insertEntry(&t , "制造日期" , "06 / 26 / 2014");
insertEntry(&t , "主人" , "就是我");
insertEntry(&t , "价格" , "六十张红色毛主席");
insertEntry(&t , "主硬盘" , "换了个120G的固态");
entry *e = removeEntry(&t, "主板型号");
if(!e)
{
printf("不存在相关记录.n");
}
else
{
puts("释放记录结点的内存.");
free(e->key);
free(e->value);
free(e);
e = NULL;
}
printTable(&t);
freeHashTable(&t);
getchar();
return 0;
}
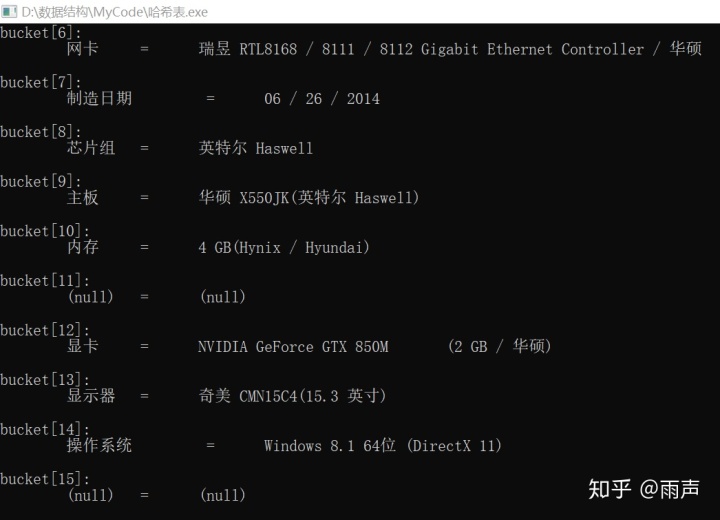