1.计算单位阶跃函数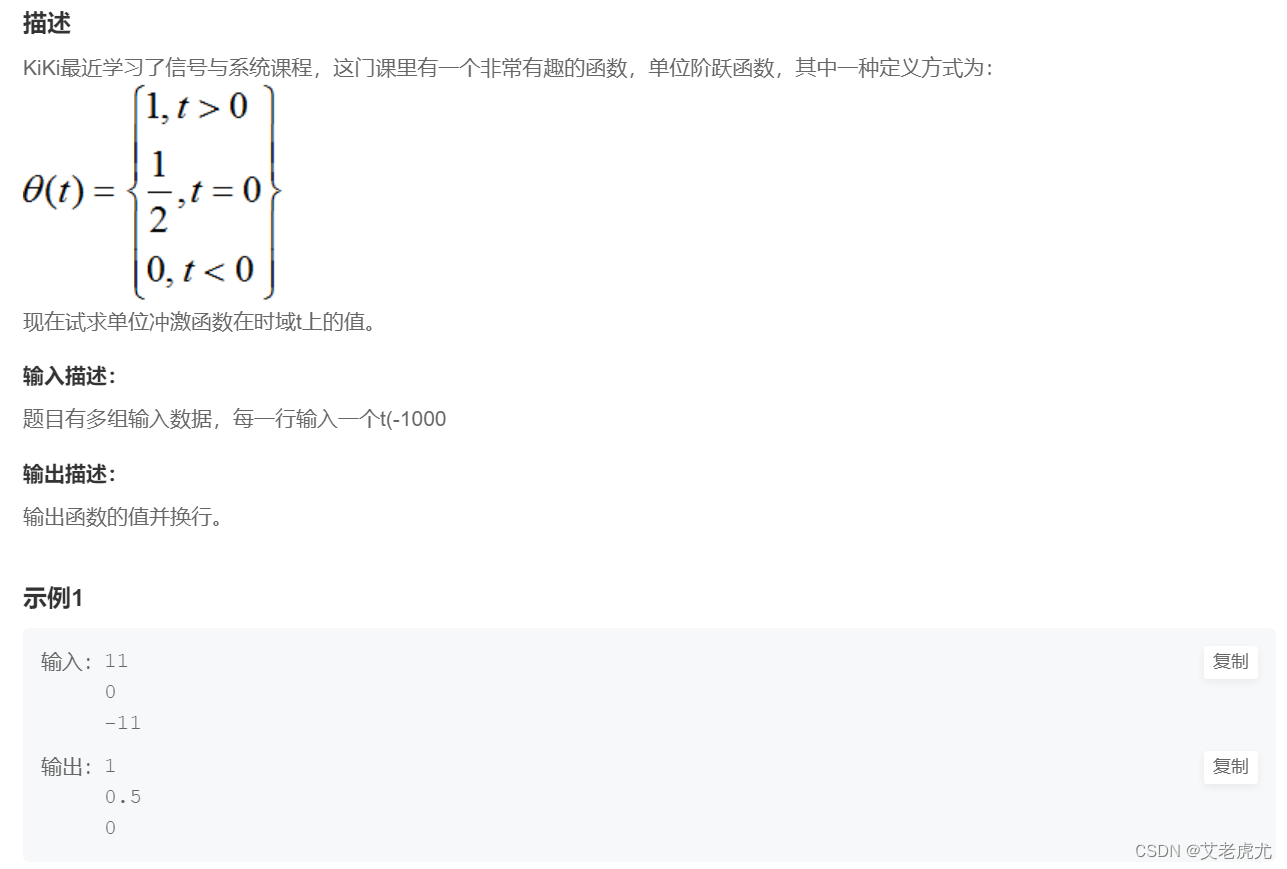
解题思路:
这个非常简单,只需要if else语句即可完成
解题代码:
#include <stdio.h>int main()
{int t = 0;while(scanf("%d",&t)!=EOF){if (t > 0)printf("1\n");else if (t < 0)printf("0\n");elseprintf("0.5\n");}return 0;
}
2.三角形判断
解题思路:
首先我们要判断他是不是三角形,三角形的判断公式是任意两条边之和要大于第三条边。
解题代码:
#include<stdio.h>
int main()
{int a = 0;int b = 0;int c = 0;while ((scanf("%d %d %d", &a, &b, &c) != EOF)){ //判断是不是三角形if (a + b > c && a + c > b && b + c > a){ //判断是不是等腰三角形if (a == b && b == c)printf("Equilateral triangle!\n");//判断是不是等边三角形else if (a == b || a == c||b==c)printf("Isosceles triangle!\n");//是其他三角形else printf("Ordinary triangle!\n");}//不是三角形elseprintf("Not a triangle!");}return 0;
}
3.衡量人体胖瘦程度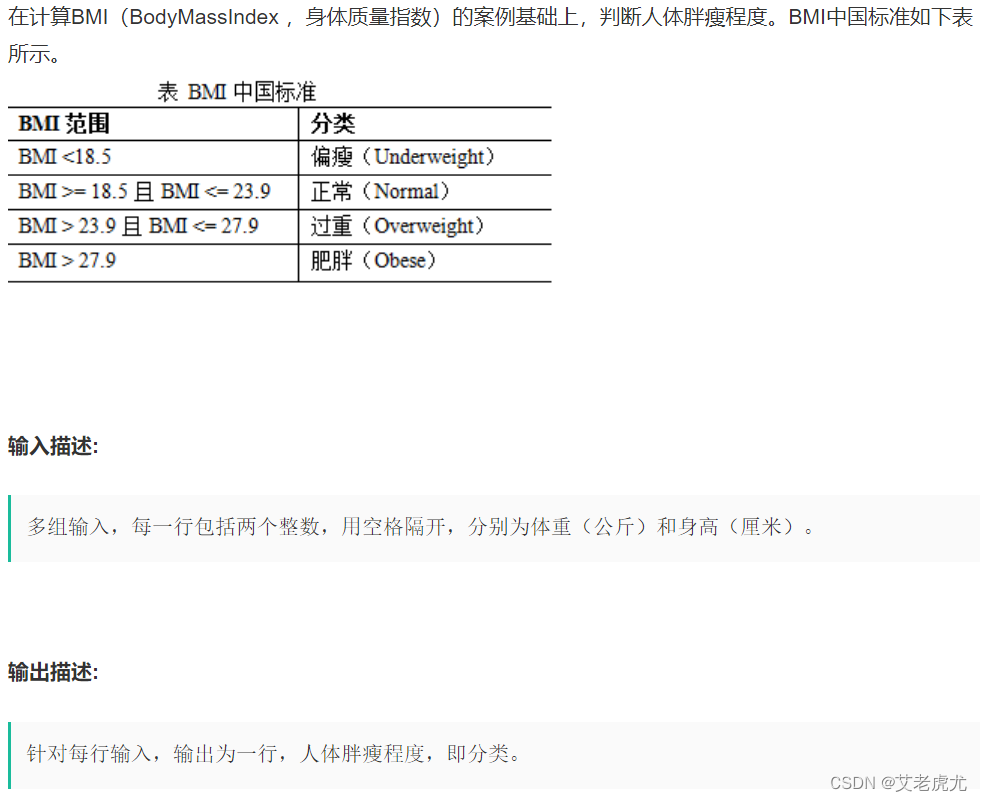
解题思路:
首先我们要知道BIM的公式:体质指数(BMI)=体重(千克)/身高(米)×身高(米)
再利用if else语句判断即可。
解题代码:
#include<stdio.h>
int main()
{//体重int s = 0;//身高 厘米int t = 0;while ((scanf("%d %d", &s, &t)) != EOF){//身高 米double m = (t / 100.0);double BIM = (s / (m * m));if (BIM < 18.5){printf("Underweight\n");}else if (BIM >= 18.5 && BIM <= 23.9){printf("Normal\n");}else if (BIM > 23.9 && BIM <= 27.9){printf("Overweight\n");}else{printf("Obese\n");} }return 0;
}
4.计算一元二次方程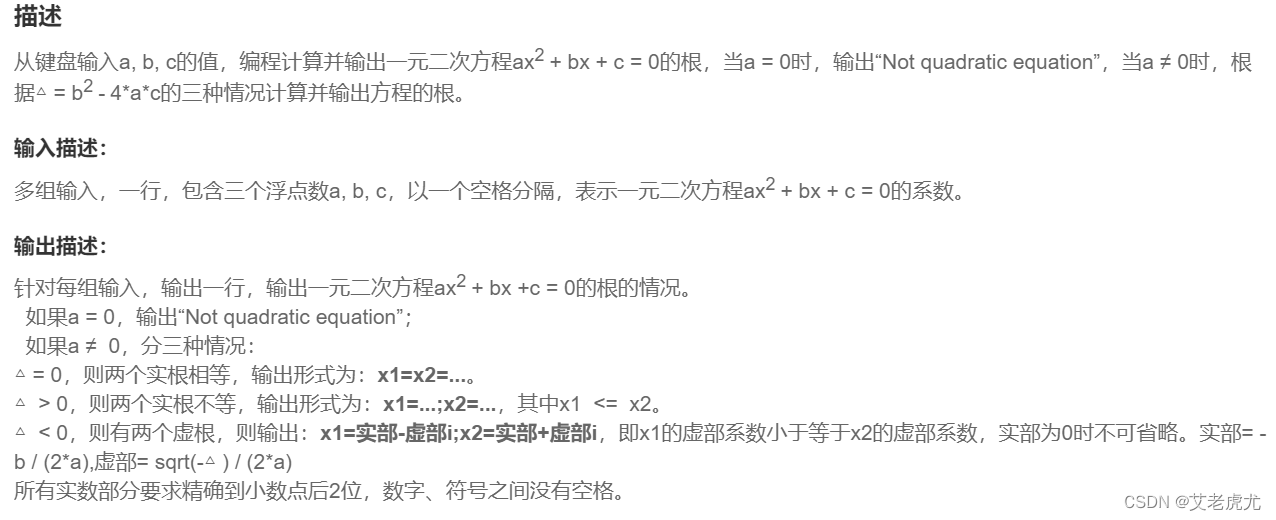
解题思路:
这里稍微要用到点数学知识,我们再用if else判断即可。
解题代码:
#include <math.h>
int main()
{float a = 0.0;float b = 0.0;float c = 0.0;while (scanf("%f %f %f", &a, &b, &c) == 3){if (a == 0){printf("Not quadratic equation\n");}else{float deta = b * b - 4 * a * c;if (deta >= 0){float result1 = (-b + sqrt(deta)) / (2 * a);float result2 = (-b - sqrt(deta)) / (2 * a);if (deta > 0){printf("x1=%.2f;x2=%.2f\n", result2, result1);}else{if (result1 == 0){printf("x1=x2=0.00\n");}else{printf("x1=x2=%.2f\n", result1);}}}else{float shibu = (-b) / (2.0 * a);float xubu = (sqrt(-deta)) / (2.0 * a);if(xubu < 0){xubu = -xubu;printf("x1=%.2f-%.2fi;x2=%.2f+%.2fi\n", shibu, xubu, shibu, xubu);}else{printf("x1=%.2f-%.2fi;x2=%.2f+%.2fi\n", shibu, xubu, shibu, xubu);}}}}return 0;
}
5.获得月份天数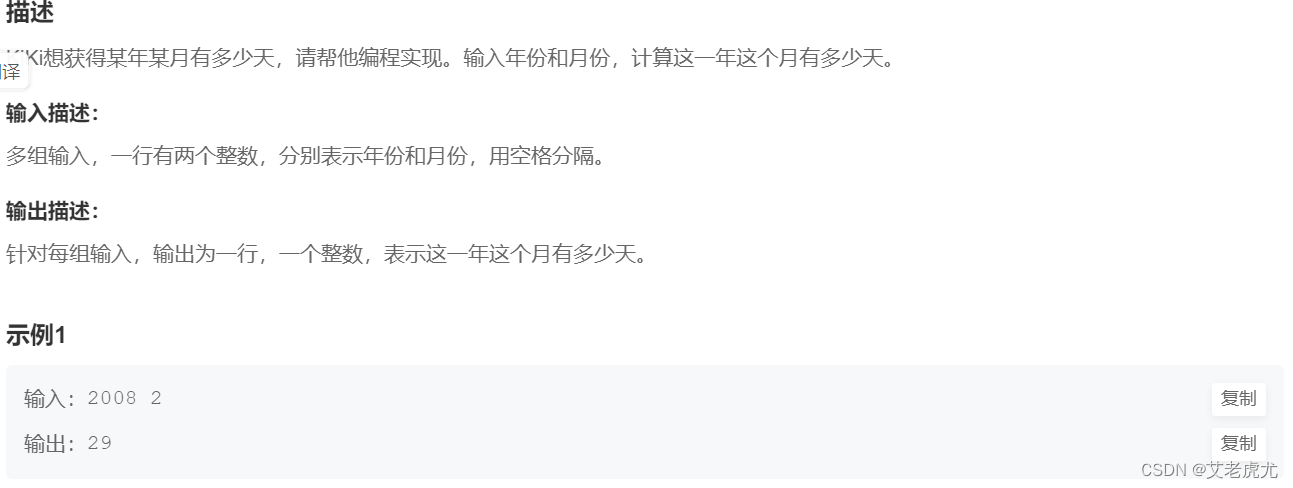
解题思路:
闰年的判断:
1.能被4整除,并且不能被100整除
2.能被400整除是闰年
解题代码:
#include<stdio.h>
int main()
{int y = 0;int m = 0;int days[12] = { 31,28,31,30,31,30,31,31,30,31,30,31 };while ((scanf("%d %d", &y, &m)) != EOF){int day = days[m - 1];if ((y % 4 == 0 && y % 100 != 0) || (y % 400 == 0)){if (m == 2){day++;}}printf("%d\n", day);}return 0;
}
6.简单计算器
解题思路:
这里我们要确定好输入格式和打印格式,其他的按照题目要求来即可。
解题代码:
#include<stdio.h> int main() {double a = 0;//第一个操作数double b = 0;//第二个操作数char ch = 0;//运算符while ((scanf("%lf %c %lf", &a, &ch, &b)) != EOF)//输入{if (ch == '+' || ch == '-' || ch == '*' || ch == '/')//判断是不是+—*/{if (ch == '+')printf("%.4lf%c%.4lf=%.4lf", a, ch, b, a + b);else if (ch == '-')printf("%.4lf%c%.4lf=%.4lf", a, ch, b, a - b);else if (ch == '*')printf("%.4lf%c%.4lf=%.4lf", a, ch, b, a * b);else{if (b == 0.0)printf("Wrong!Division by zero!\n");elseprintf("%.4lf%c%.4lf=%.4lf", a, ch, b, a / b);}}elseprintf("Invalid operation!\n");}return 0; }