首先一个简单的折线图:
直接上代码:
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<script type="text/javascript" src="js/jquery.min.js"></script><!-- 这是必要的js库,最好顺序不要错,不然要报错-->
<script type="text/javascript" src="js/jquery.jqplot.min.js"></script>
<script type="text/javascript" src="js/jqplot.canvasTextRenderer.min.js"></script>
<script type="text/javascript" src="js/jqplot.canvasAxisLabelRenderer.min.js"></script>
<script type="text/javascript" src="js/jqplot.canvasAxisTickRenderer.min.js"></script>
<script type="text/javascript" src="js/demo.js"></script>
<link href="css/jquery.jqplot.css" rel="stylesheet">
<title>chart</title>
</head>
<body>
<div class="container" align="center">
<div id="chart1" style="height: 270px; width: 830px;"></div>
</div>
</body>
</html>
demo.js:
$(document).ready(function(){
var data=[[3,7,9,1,4,6,8,2,5]];//这里是折线点的数据,格式[x,y],这里只写了一组数据,默认为y轴上的数据,而x上的数据默认为:1,2,3,4;
var plot1 = $.jqplot ('chart1',data);//第一个参数为显示图表容器ID
});
就这样一个简单的图表就完成了。
我们还可以给它加些东西,比如这样:
代码如下:
$(document).ready(
function
(){
var
plot2 = $.jqplot (
'chart1'
, [[3,7,9,1,4,6,8,2,5]], {
// 给图表设置标题
title:
'Plot With Options'
,
// 设置坐标系一些属性
axesDefaults: {
//给坐标系的一些标签添加渲染效果
labelRenderer: $.jqplot.CanvasAxisLabelRenderer
},
// 设置坐标系样式
axes: {
xaxis: {
label:
"X Axis"
,//给X轴添加标题
},
yaxis: {
label:
"Y Axis"
}
}
});
});
还可以变成这样:
代码如下:
$(document).ready(
function
(){
// 初始化数据
var
cosPoints = [];
for
(
var
i=0; i<2*Math.PI; i+=0.4){
cosPoints.push([i, Math.cos(i)]);
}
var
sinPoints = [];
for
(
var
i=0; i<2*Math.PI; i+=0.4){
sinPoints.push([i, 2*Math.sin(i-.8)]);
}
var
powPoints1 = [];
for
(
var
i=0; i<2*Math.PI; i+=0.4) {
powPoints1.push([i, 2.5 + Math.pow(i/4, 2)]);
}
var
powPoints2 = [];
for
(
var
i=0; i<2*Math.PI; i+=0.4) {
powPoints2.push([i, -2.5 - Math.pow(i/4, 2)]);
}
var
plot3 = $.jqplot(
'chart1'
, [cosPoints, sinPoints, powPoints1, powPoints2],
{
title:
'Line Style Options'
,
// 设置线条的样式
series:[
{
// 设置线条1的宽度和点的样式
lineWidth:2,
markerOptions: { style:
'dimaond'
}//点的样式为砖石
},
{
// 设置线条2的不显示两点之间的线条,设置点的大小和样式
showLine:
false
,
markerOptions: { size: 7, style:
"x"
}
},
{
markerOptions: { style:
"circle"
}//设置点的样式为圆点
},
{
//设置点的样式为正方形
lineWidth:5,
markerOptions: { style:
"filledSquare"
, size:10 }
}
]
}
);
});
还可以做成这样:
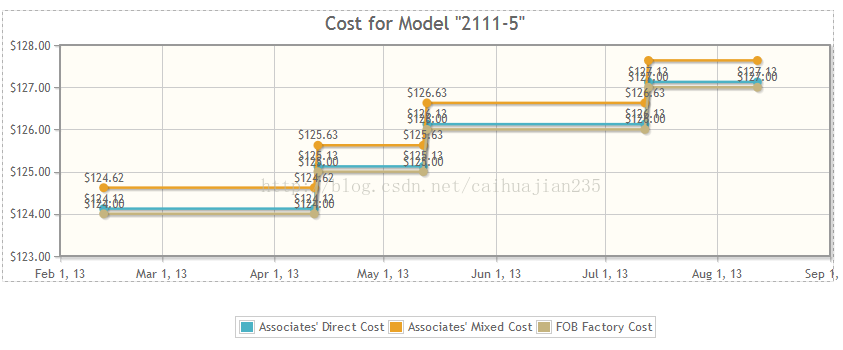
代码如下:
//
var labels=["Associates' Direct Cost"
];
var lineData=[{"model":"2111-5","cpName":"Associates' Direct Cost","value":"124.12400000","begin":"2013-02-13 00:00:00","end":"2013-04-12 00:00:00","Warehouse":"Homelegance, Inc"}]//这是数据格式
plot = $.jqplot("", lineData, {
title: "Cost for Model \"" + data[0].model + "\"",
animate: !$.jqplot.use_excanvas,//禁用扩展插件中的动画效果
animateReplot: true,//动态画线条
seriesDefaults: {
pointLabels:{ //设置坐标点标签
show:true,
location:'n',//设置标签显示在坐标点的位置,用英文方向缩写表示的。
ypadding:3//在垂直方向的偏移量
}
},
cursor:{ //设置光标样式
show: true,
zoom:true, //让光标在图表区显示
showTooltip:false//当光标在图表区时是否显示提示框,提示的是坐标信息
},
axes:{
xaxis:{
renderer:$.jqplot.DateAxisRenderer,//设置x轴日期渲染插件
tickOptions:{
formatString:'%b %#d, %y',//设置日期显示样式
angle: -30
}
},
yaxis:{
labelRenderer: $.jqplot.CanvasAxisLabelRenderer,//一种标签渲染插件
tickOptions : {
angle: -30,
formatString : '$%.2f'
}
}
},
legend:{//显示下面的示意图
renderer: $.jqplot.EnhancedLegendRenderer,//渲染插件
show:true,
location: 's',
labels : labels,//labels指的是每种颜色所表示的东西,这里放的是cp_Name;
placement:'outside',//设置显示在图表中还是在图表外
yoffset: 60,//垂直偏移量
rendererOptions:{
numberRows: 1//显示多少行
}
}
});
需要的js文件有:
<script type="text/javascript" src="js/jquery.min.js"></script>
<script type="text/javascript" src="js/jquery.jqplot.min.js"></script>
<script type="text/javascript" src="js/jqplot.canvasTextRenderer.min.js"></script>
<script type="text/javascript" src="js/jqplot.canvasAxisLabelRenderer.min.js"></script>
jqplot.enhancedLegendRenderer.min.js,jqplot.dateAxisRenderer.min.js,jqplot.highlighter.min.js,jqplot.pointLabels.min.js,jqplot.cursor.min.js
excanvas.compiled.js,jquery.jqplot.css
jqplot功能很强大的,还可以画很多很多的图形。今天只说到这里了。