题目链接:https://vjudge.net/problem/POJ-1459
Power Network
Time Limit: 2000MS | Memory Limit: 32768K | |
Total Submissions: 29270 | Accepted: 15191 |
Description
A power network consists of nodes (power stations, consumers and dispatchers) connected by power transport lines. A node u may be supplied with an amount s(u) >= 0 of power, may produce an amount 0 <= p(u) <= pmax(u) of power, may consume an amount 0 <= c(u) <= min(s(u),cmax(u)) of power, and may deliver an amount d(u)=s(u)+p(u)-c(u) of power. The following restrictions apply: c(u)=0 for any power station, p(u)=0 for any consumer, and p(u)=c(u)=0 for any dispatcher. There is at most one power transport line (u,v) from a node u to a node v in the net; it transports an amount 0 <= l(u,v) <= lmax(u,v) of power delivered by u to v. Let Con=Σuc(u) be the power consumed in the net. The problem is to compute the maximum value of Con.
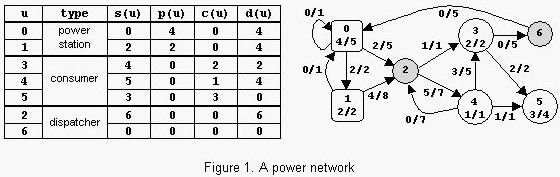
An example is in figure 1. The label x/y of power station u shows that p(u)=x and pmax(u)=y. The label x/y of consumer u shows that c(u)=x and cmax(u)=y. The label x/y of power transport line (u,v) shows that l(u,v)=x and lmax(u,v)=y. The power consumed is Con=6. Notice that there are other possible states of the network but the value of Con cannot exceed 6.
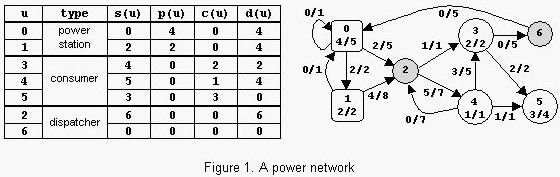
An example is in figure 1. The label x/y of power station u shows that p(u)=x and pmax(u)=y. The label x/y of consumer u shows that c(u)=x and cmax(u)=y. The label x/y of power transport line (u,v) shows that l(u,v)=x and lmax(u,v)=y. The power consumed is Con=6. Notice that there are other possible states of the network but the value of Con cannot exceed 6.
Input
There are several data sets in the input. Each data set encodes a power network. It starts with four integers: 0 <= n <= 100 (nodes), 0 <= np <= n (power stations), 0 <= nc <= n (consumers), and 0 <= m <= n^2 (power transport lines). Follow m data triplets (u,v)z, where u and v are node identifiers (starting from 0) and 0 <= z <= 1000 is the value of lmax(u,v). Follow np doublets (u)z, where u is the identifier of a power station and 0 <= z <= 10000 is the value of pmax(u). The data set ends with nc doublets (u)z, where u is the identifier of a consumer and 0 <= z <= 10000 is the value of cmax(u). All input numbers are integers. Except the (u,v)z triplets and the (u)z doublets, which do not contain white spaces, white spaces can occur freely in input. Input data terminate with an end of file and are correct.
Output
For each data set from the input, the program prints on the standard output the maximum amount of power that can be consumed in the corresponding network. Each result has an integral value and is printed from the beginning of a separate line.
Sample Input
2 1 1 2 (0,1)20 (1,0)10 (0)15 (1)20 7 2 3 13 (0,0)1 (0,1)2 (0,2)5 (1,0)1 (1,2)8 (2,3)1 (2,4)7(3,5)2 (3,6)5 (4,2)7 (4,3)5 (4,5)1 (6,0)5(0)5 (1)2 (3)2 (4)1 (5)4
Sample Output
15 6
Hint
The sample input contains two data sets. The first data set encodes a network with 2 nodes, power station 0 with pmax(0)=15 and consumer 1 with cmax(1)=20, and 2 power transport lines with lmax(0,1)=20 and lmax(1,0)=10. The maximum value of Con is 15. The second data set encodes the network from figure 1.
Source
Southeastern Europe 2003
题意:
有np个供电站(只供电不用电)、nc个用电站(只用电不供电),以及n-np-nc个中转站(既不供电也不用电),且已经知道这些站的连接关系,问单位时间最多能消耗多少的电?
题解:
最大流问题。
1.建立超级源点,超级源点与每个供电站相连,且边的容量为供电站的最大供电量,表明流经此供电站的电量最多只能为自身的供电量。
2.建立超级汇点,每个用电站与超级汇点相连,且边的容量为用电站的最大用电量,表明流经此用电站的电量最多只能为自身的消耗量。
代码如下:


1 #include <iostream> 2 #include <cstdio> 3 #include <cstring> 4 #include <algorithm> 5 #include <vector> 6 #include <cmath> 7 #include <queue> 8 #include <stack> 9 #include <map> 10 #include <string> 11 #include <set> 12 using namespace std; 13 typedef long long LL; 14 const int INF = 2e9; 15 const LL LNF = 9e18; 16 const int mod = 1e9+7; 17 const int MAXN = 1e2+10; 18 19 int maze[MAXN][MAXN]; 20 int gap[MAXN], dis[MAXN], pre[MAXN], cur[MAXN]; 21 int flow[MAXN][MAXN]; 22 23 int sap(int start, int end, int nodenum) 24 { 25 memset(cur, 0, sizeof(cur)); 26 memset(dis, 0, sizeof(dis)); 27 memset(gap, 0, sizeof(gap)); 28 memset(flow, 0, sizeof(flow)); 29 int u = pre[start] = start, maxflow = 0, aug = INF; 30 gap[0] = nodenum; 31 32 while(dis[start]<nodenum) 33 { 34 loop: 35 for(int v = cur[u]; v<nodenum; v++) 36 if(maze[u][v]-flow[u][v]>0 && dis[u] == dis[v]+1) 37 { 38 aug = min(aug, maze[u][v]-flow[u][v]); 39 pre[v] = u; 40 u = cur[u] = v; 41 if(v==end) 42 { 43 maxflow += aug; 44 for(u = pre[u]; v!=start; v = u, u = pre[u]) 45 { 46 flow[u][v] += aug; 47 flow[v][u] -= aug; 48 } 49 aug = INF; 50 } 51 goto loop; 52 } 53 54 int mindis = nodenum-1; 55 for(int v = 0; v<nodenum; v++) 56 if(maze[u][v]-flow[u][v]>0 && mindis>dis[v]) 57 { 58 cur[u] = v; 59 mindis = dis[v]; 60 } 61 if((--gap[dis[u]])==0) break; 62 gap[dis[u]=mindis+1]++; 63 u = pre[u]; 64 } 65 return maxflow; 66 } 67 68 int main() 69 { 70 int n, np, nc, m; 71 while(scanf("%d%d%d%d", &n,&np,&nc,&m)!=EOF) 72 { 73 int start = n, end = n+1; 74 memset(maze, 0, sizeof(maze)); 75 for(int i = 1; i<=m; i++) 76 { 77 int u, v, w; 78 while(getchar()!='('); 79 scanf("%d,%d)%d",&u,&v,&w); 80 maze[u][v] = w; 81 } 82 83 for(int i = 1; i<=np; i++) 84 { 85 int id, p; 86 while(getchar()!='('); 87 scanf("%d)%d", &id,&p); 88 maze[start][id] = p; 89 } 90 91 for(int i = 1; i<=nc; i++) 92 { 93 int id, p; 94 while(getchar()!='('); 95 scanf("%d)%d", &id,&p); 96 maze[id][end] = p; 97 } 98 99 cout<< sap(start, end, n+2) <<endl; 100 } 101 }