两个链接合并
了解问题 (Understand the Problem)
We are given two singly linked lists and we have to find the point at which they merge.
我们给了两个单链表,我们必须找到它们合并的点。
[SLL 1] 1--->3--->5
\
9--->12--->17--->None
/
[SLL 2] 7--->8
The diagram above illustrates that the merge occurs at node 9.
上图说明了合并发生在节点9上。
We can rest assured that the parameters we are given, head1 and head2, which are the heads of both lists will never be equal and will never be none. The two lists are also guaranteed to merge at some point.
我们可以放心,我们给定的参数head1和head2都是两个列表的头部,它们永远不会相等,也永远不会相等。 这两个列表也一定会合并。
We need a plan to find and return the integer data value of the node where the two lists merge.
我们需要一个计划来查找并返回两个列表合并的节点的整数数据值。
计划 (Plan)
In order to traverse through the lists to find the point at which they merge, we need to set two different pointers. One for the first singly linked list, another for the second. Remember that we are given the heads of both as parameters, so we will set our pointers to them in order to start from the beginning of each.
为了遍历列表以查找它们合并的点,我们需要设置两个不同的指针。 一个用于第一个单链表,另一个用于第二个链表。 请记住,我们将两个的头都作为参数,因此我们将设置指向它们的指针,以便从每个头开始。
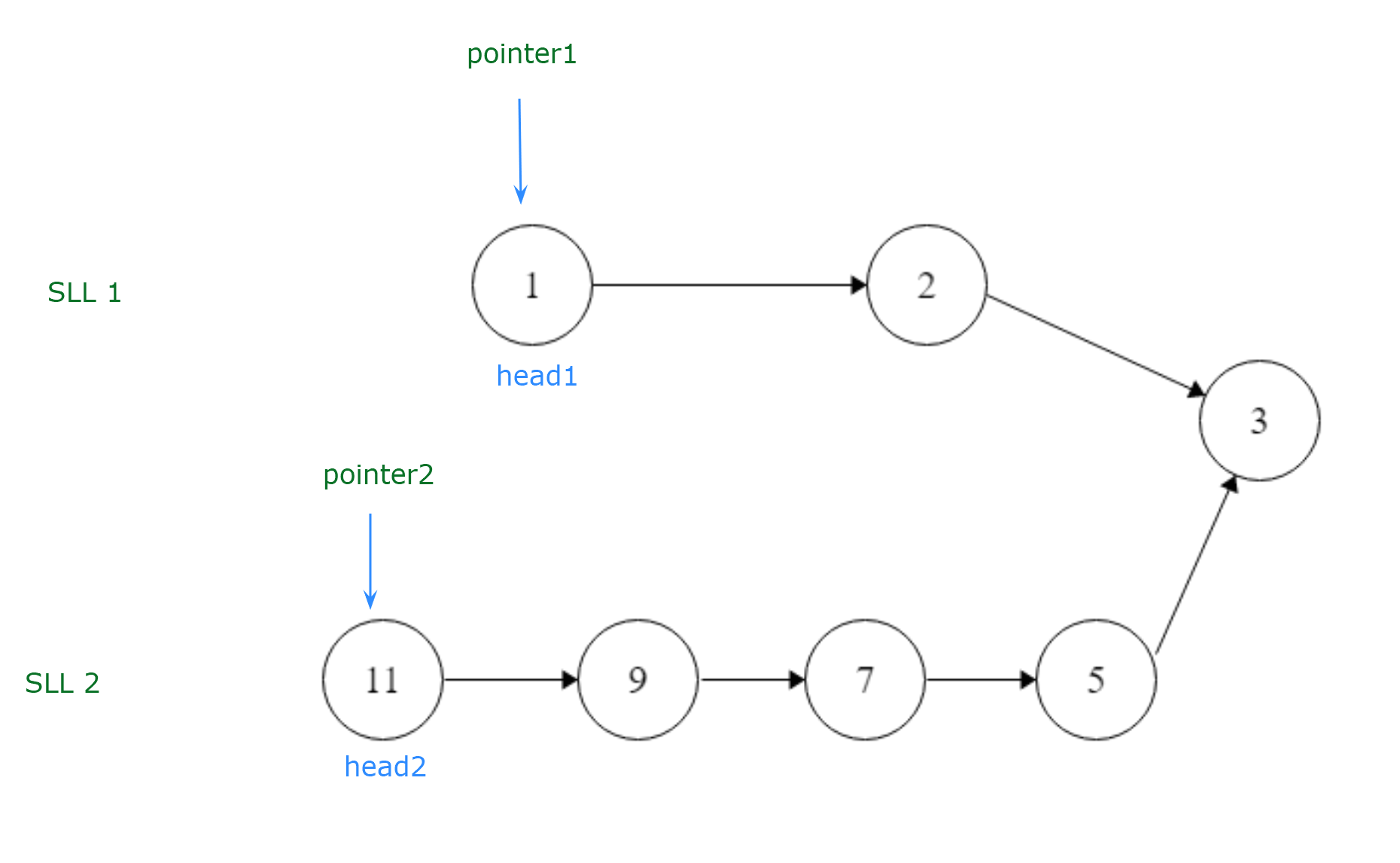
pointer1 = head1
pointer2 = head2
To begin traversing the lists, we’ll need create a while loop to loop through the lists while the lists are not None.
要开始遍历列表,我们需要创建一个while循环来遍历列表,而列表不是None。
while not None:
If at any point, pointer1 and pointer2 are equal, we must break out of the while loop, as we have found the node where the two lists merge.
如果在任何时候,pointer1和pointer2相等,则必须打破while循环,因为我们找到了两个列表合并的节点。
if pointer1 == pointer2:
break
However, if it is not equal, we will move forward by utilizing .next.
但是,如果不相等,我们将利用.next向前移动。
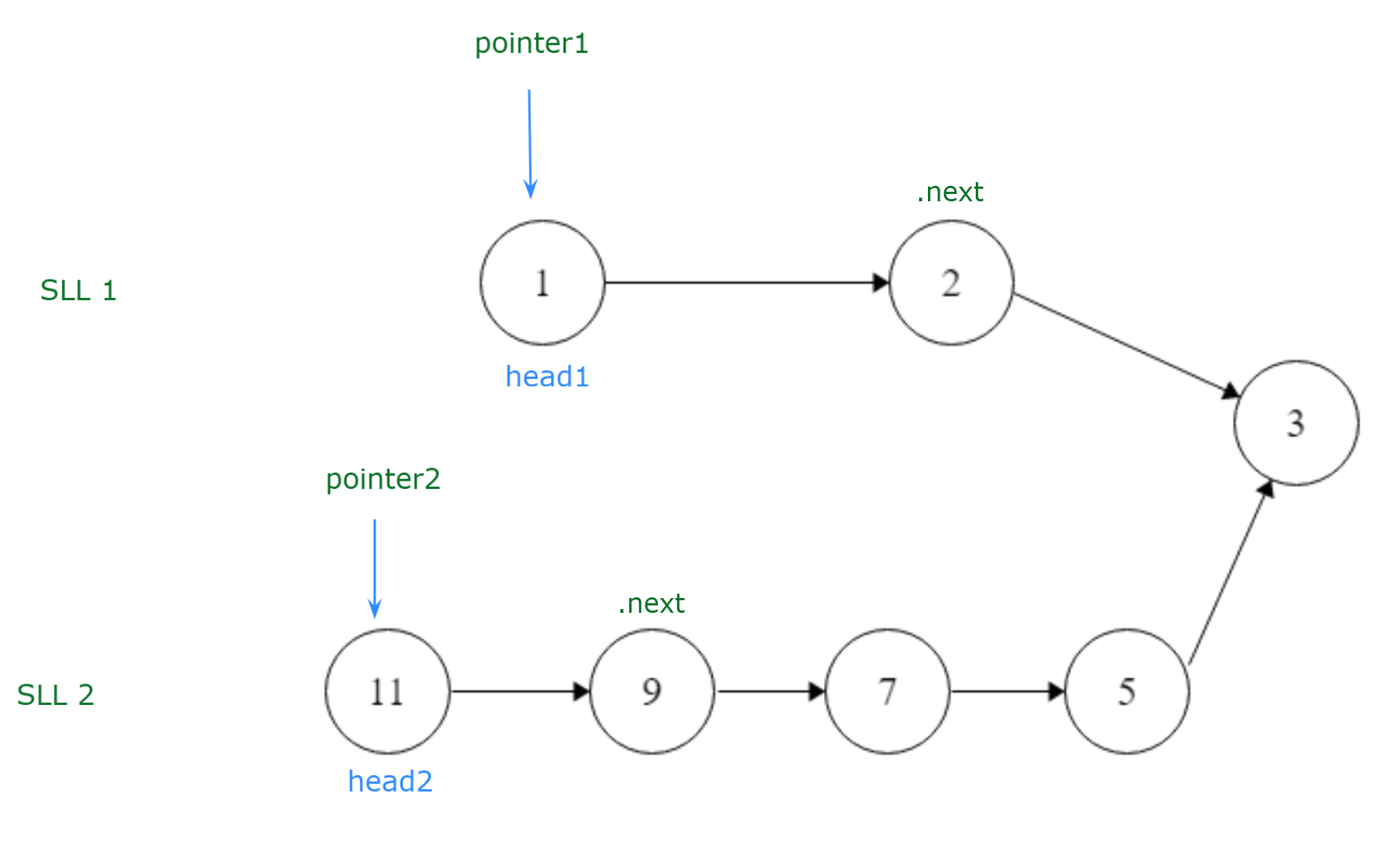
pointer1 = pointer1.next
pointer2 = pointer2.next
As we can see from the example diagram, our first singly linked list, SLL 1, is shorter than SLL 2. So let’s suppose SLL 1 hits None before SLL 2 finds the merge node. In this case we will simply begin again, setting the same if statement for SLL 2, in case we have a test case in our program where the second SLL is the shorter one.
从示例图中可以看到,我们的第一个单链列表SLL 1比SLL 2短。因此,假设SLL 1在SLL 2找到合并节点之前命中None。 在这种情况下,我们将再次开始,为SLL 2设置相同的if语句,以防程序中有一个测试用例,其中第二个SLL是较短的。
if pointer1 == None:
pointer1 == head1
if pointer2 == None:
pointer2 == head1
This logic of starting over will repeat in the while loop until both pointers find the merging node at the same time, or in other words, until pointer1 and pointer2 are equal. When that happens, we must remember to do what was asked of us and return the integer data value of that node.
重新开始的逻辑将在while循环中重复,直到两个指针同时找到合并节点,或者换句话说,直到指针1和指针2相等为止。 发生这种情况时,我们必须记住执行要求我们执行的操作,并返回该节点的整数数据值。
return pointer1.data
Because this will also be pointer2’s data, we can return this in lieu of pointer1. It has the same value.
因为这也将是指针2的数据,所以我们可以代替指针1返回它。 它具有相同的值。
return pointer2.data
执行 (Execute)
# For our reference:
#
# SinglyLinkedListNode:
# int data
# SinglyLinkedListNode next
#
#def findMergeNode(head1, head2): # Set the pointers
pointer1 = head1
pointer2 = head2 while not None: # if we found the merging node, then break out of the loop
if pointer1 == pointer2:
break #traverse through lists
pointer1 = pointer1.next
pointer2= pointer2.next # Begin again if one was shorter than the other
if pointer1 == None:
pointer1 = head1
if pointer2 == None:
pointer2 = head2 return pointer1.data
翻译自: https://levelup.gitconnected.com/how-to-find-the-merge-point-of-two-linked-lists-ba55a129caa2
两个链接合并
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如若转载,请注明出处:http://www.mzph.cn/news/392398.shtml
如若内容造成侵权/违法违规/事实不符,请联系多彩编程网进行投诉反馈email:809451989@qq.com,一经查实,立即删除!