- <script></script>的三种用法:
- 放在<body>中
- 放在<head>中
- 放在外部JS文件中
- 三种输出数据的方式:
- 使用 document.write() 方法将内容写到 HTML 文档中。
- 使用 window.alert() 弹出警告框。
- 使用 innerHTML 写入到 HTML 元素。
- 使用 "id" 属性来标识 HTML 元素。
- 使用 document.getElementById(id) 方法访问 HTML 元素。
- 用innerHTML 来获取或插入元素内容。
- 登录页面准备:
- 增加错误提示框。
- 写好HTML+CSS文件。
- 设置每个输入元素的id
- 定义JavaScript 函数。
- 验证用户名6-20位
- 验证密码6-20位
- onclick调用这个函数。
1 .box {
2 border: 1px solid #cccccc;
3 position: absolute;
4 top: 25px;
5 left: 40px;
6 float: left;
7 height: 300px;
8 width: 400px;
9 }
10
11 h2 {
12 font-family: 华文楷体;
13 font-size: 28px;
14 text-align: center;
15 background: #cccccc;
16 margin-top: auto;
17 height: 40px;
18 width: 400px;
19 }
20 .input_box {
21 height: 60px;
22 width: 80px;
23 margin-left: 10%;
24 }
25
26 input {
27 align-self: center;
28 height: 30px;
29 width: 280px;
30
31 }
32
33 button {
34 align-content: center;
35 font-family: 华文楷体;
36 font-size: 28px;
37 text-align: center;
38 background: #cccccc;
39 height: 40px;
40 width: 300px;
41 }
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <title>登录</title>
6 <link href="../static/css/1.css" rel="stylesheet" type="text/css">
7
8 <script>
9 function fnLogin() {
10 var oUname = document.getElementById("uname")
11 var oError = document.getElementById("error_box")
12 var oPassward = document.getElementById("upass")
13 if (oUname.value.length<6){
14 oError.innerHTML = "用户名至少6位"
15 }
16 if(oUname.value.length>6&oPassward.value.length<6){
17 oError.innerHTML="密码至少为6位"
18 }
19 }
20 </script>
21 </head>
22 <body>
23 <div class="box">
24 <h2>登录</h2>
25
26 <div class="input_box">
27 <input type="text" id="uname" placeholder="请输入用户名">
28 </div>
29 <div class="input_box">
30 <input type="password" id="upass" placeholder="请输入密码">
31 </div>
32 <div id="error_box"><br></div>
33 <div class="input_box">
34 <button onclick="fnLogin()">登录</button>
35 </div>
36
37 </div>
38 </body>
39 </html>
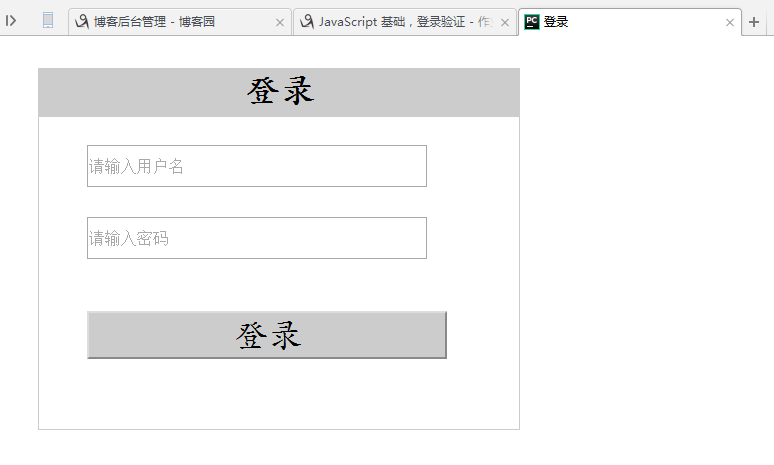