python分句
Python中的循环 (Loops in Python)
for
loopfor
循环while
loopwhile
循环
Let’s learn how to use control statements like break
, continue
, and else
clauses in the for
loop and the while
loop.
让我们学习如何在for
循环和while
循环中使用诸如break
, continue
和else
子句之类的控制语句。
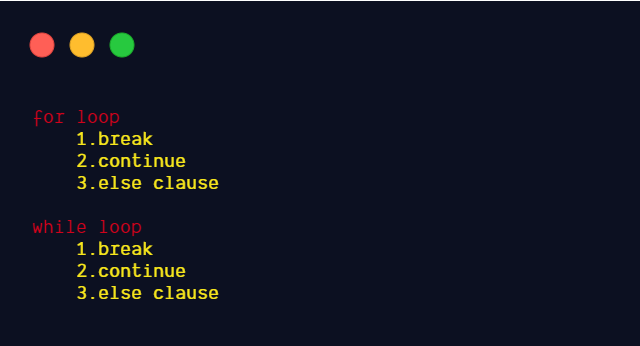
声明 (‘for’ Statement)
The for
statement is used to iterate over the elements of a sequence (such as a string, tuple, or list) or any other iterable object.
for
语句用于遍历序列的元素(例如字符串,元组或列表)或任何其他可迭代对象。
for item in iterable:
suite
- The iterable is evaluated only once. An iterator is created for the result of that iterable. 可迭代仅被评估一次。 为该可迭代的结果创建一个迭代器。
- The suite is then executed once for each item provided by the iterator, in the order returned by the iterator. 然后按迭代器返回的顺序对迭代器提供的每个项目执行一次套件。
- When the items are exhausted, the loop terminates. 物品用完后,循环终止。
例子1.'for'循环 (Example 1. ‘for’ loop)
for i in range(1,6):
print (i)'''
Output:
1
2
3
4
5
'''
The for
loop may have control statements like break
andcontinue
, or the else
clause.
for
循环可能具有控制语句,例如break
和continue
或else
子句。
There may be a situation in which you may need to exit a loop completely for a particular condition or you want to skip a part of the loop and start the next execution. The for
loop and while
loop have control statements break
and continue
to handle these situations.
在某些情况下,您可能需要针对特定条件完全退出循环,或者想要跳过循环的一部分并开始下一次执行。 for
循环和while
循环的控制语句break
并continue
处理这些情况。
“中断”声明 (‘break’ Statement)
The break
statement breaks out of the innermost enclosing for
loop.
break
语句脱离了最里面的for
循环。
A break
statement executed in the first suite terminates the loop without executing the else
clause’s suite.
在第一个套件中执行的break
语句将终止循环,而不执行else
子句的套件。
The break
statement is used to terminate the execution of the for
loop or while
loop, and the control goes to the statement after the body of the for
loop.
break
语句用于终止for
循环或while
的执行 循环,然后控制转到for
循环主体之后的语句。
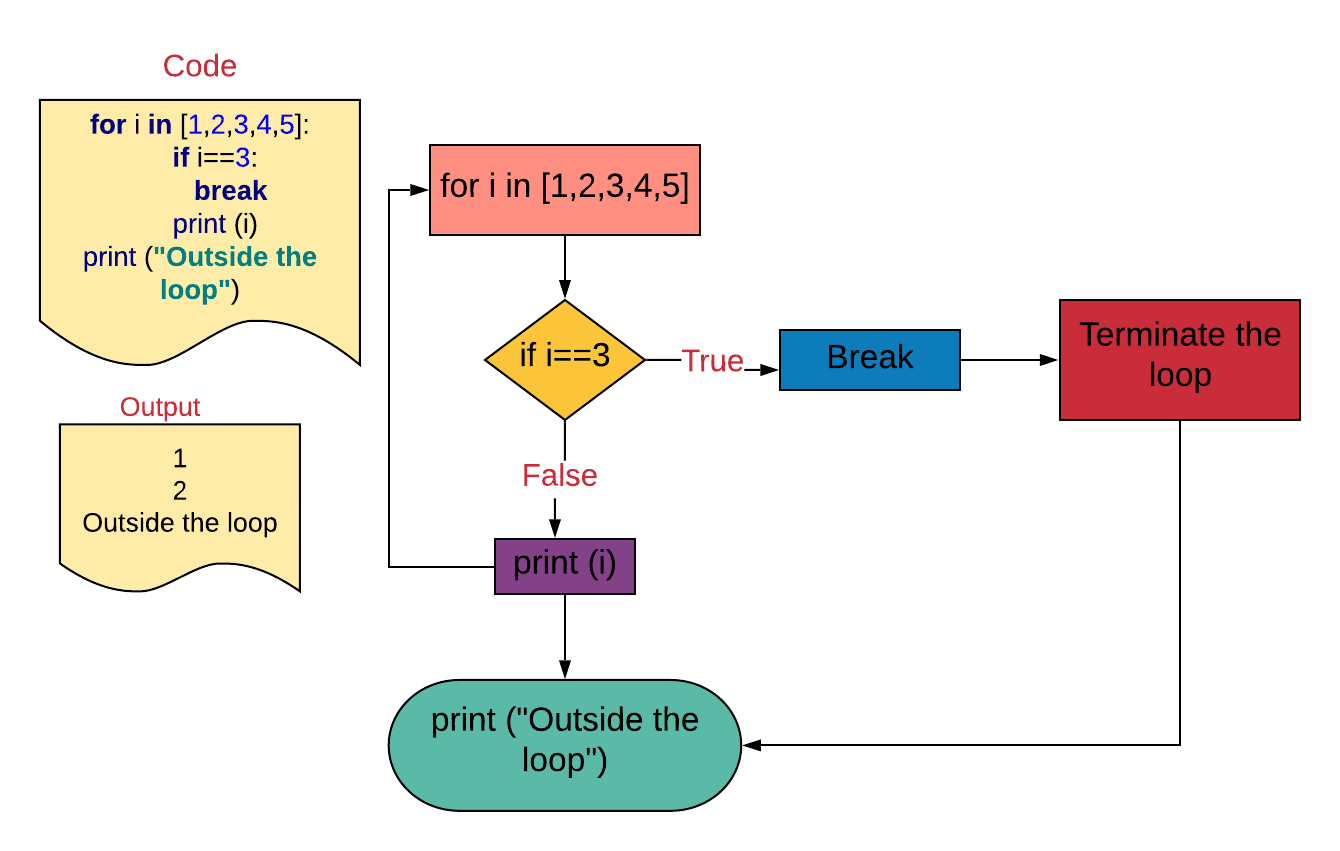
示例2.在“ for”循环中使用“ break”语句 (Example 2. Using the ‘break’ statement in a ‘for’ loop)
The
for
loop will iterate through the iterable.for
循环将迭代可迭代对象。If the item in the iterable is
3
, it will break the loop and the control will go to the statement after thefor
loop, i.e.,print (“Outside the loop”)
.如果iterable中的项目为
3
,它将中断循环,并且控件将在for
循环后转到语句,即print (“Outside the loop”)
。If the item is not equal to
3
, it will print the value, and thefor
loop will continue until all the items are exhausted.如果该项不等于
3
,它将打印该值,并且for
循环将继续进行,直到用尽所有项。
for i in [1,2,3,4,5]:
if i==3:
break
print (i)
print ("Outside the loop")'''
Output:
1
2
Outside the loop
'''
例子3.在带有“ else”子句的“ for”循环中使用“ break”语句 (Example 3. Using the ‘break’ statement in a ‘for’ loop having an ‘else’ clause)
A break
statement executed in the first suite terminates the loop without executing the else
clause’s suite.
在第一个套件中执行的break
语句将终止循环,而不执行else
子句的套件。
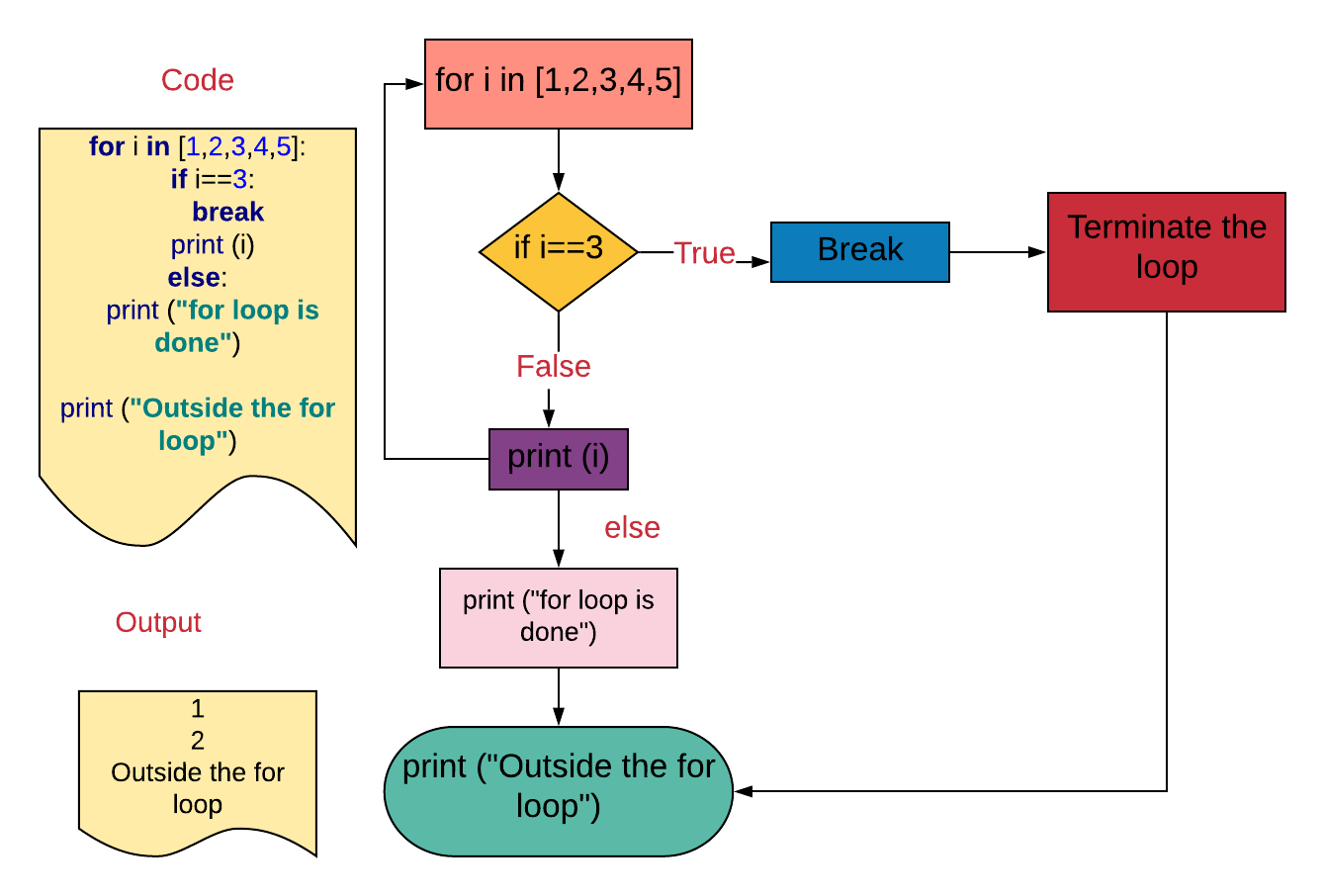
Example:
例:
In the for
loop, when the condition i==3
is satisfied, it will break the for
loop, and the control will go to the statement after the body of the for
loop, i.e., print (“Outside the for loop”)
.
在for
循环中,当满足条件i==3
,它将中断for
循环,并且控制将进入for
循环主体之后的语句,即print (“Outside the for loop”)
。
The else
clause is also skipped.
else
子句也被跳过。
for i in [1,2,3,4,5]:
if i==3:
break
print (i)else:
print ("for loop is done")
print ("Outside the for loop")'''
Output:
1
2
Outside the for loop
'''
“继续”声明 (‘continue’ Statement)
The continue
statement continues with the next iteration of the loop.
continue
语句继续执行循环的下一个迭代。
A continue
statement executed in the first suite skips the rest of the suite and continues with the next item or with the else
clause, if there is no next item.
在第一个套件中执行的continue
语句将跳过套件的其余部分,并继续执行下一项或else
子句(如果没有下一项)。
例子4.在“ for”循环中使用“ continue”语句 (Example 4. Using the ‘continue’ statement in a ‘for’ loop)
The
for
loop will iterate through the iterable.for
循环将迭代可迭代对象。If the item in the iterable is
3
, it will continue thefor
loop and won’t execute the rest of the suite, i.e.,print (i)
.如果iterable中的项目为
3
,它将继续for
循环,并且将不执行套件的其余部分,即print (i)
。So element
3
will be skipped.因此元素
3
将被跳过。The
for
loop will continue execution from the next element.for
循环将从下一个元素继续执行。The
else
clause is also executed.else
子句也将执行。
for i in [1,2,3,4,5]:
if i==3:
continue
print (i)else:
print ("for loop is done")
print ("Outside the for loop")'''
1
2
4
5
for loop is done
Outside the for loop
'''
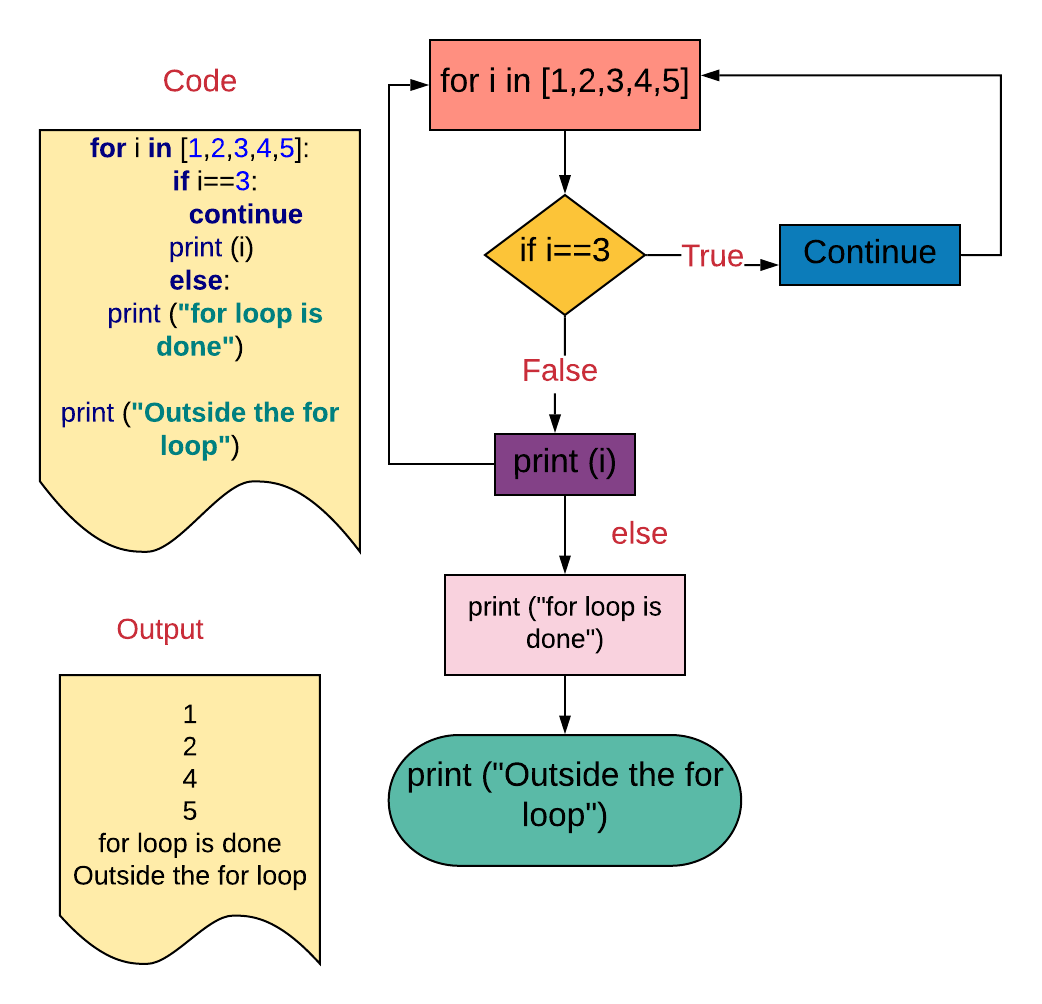
for循环中的else子句 (‘else’ Clause in ‘for’ Loop)
Loop statements may have an else
clause. It is executed when the for
loop terminates through exhaustion of the iterable — but not when the loop is terminated by a break
statement.
循环语句可能包含else
子句。 当for
循环通过迭代器的穷尽而终止时,将执行该语句,但当该循环由break
语句终止时,则不会执行该语句。
例子5.在“ for”循环中使用“ else”子句 (Example 5. Using the ‘else’ clause in a ‘for’ loop)
The else
clause is executed when the for
loop terminates after the exhaustion of the iterable.
当for
循环在迭代器用尽后终止时,将执行else
子句。
for i in [1,2,3,4,5]:
print (i)else:
print ("for loop is done")
print ("Outside the for loop")'''
1
2
3
4
5
for loop is done
Outside the for loop
'''
例6.在“ for”循环中使用“ break”语句使用“ else”子句 (Example 6. Using the ‘else’ clause in a ‘for’ loop with the ‘break’ statement)
The else
clause is not executed when the for
loop is terminated by a break
statement.
当for
循环由break
语句终止时, else
子句不会执行。
for i in [1,2,3,4,5]:
if i==3:
break
print (i)else:
print ("for loop is done")
print ("Outside the for loop")'''
1
2
Outside the for loop
'''
示例7.在“ for”循环中使用“ continue”语句使用“ else”子句 (Example 7. Using the ‘else’ clause in a ‘for’ loop with the ‘continue’ statement)
The else
clause is also executed.
else
子句也将执行。
for i in [1,2,3,4,5]:
if i==3:
continue
print (i)else:
print ("for loop is done")
print ("Outside the for loop")'''
1
2
4
5
for loop is done
Outside the for loop
'''
例子8.在“ for”循环中使用“ break”语句和“ else”子句 (Example 8. Using the ‘break’ statement and ‘else’ clause in a ‘for’ loop)
Search the particular element in the list. If it exists, break the loop and return the index of the element; else return “Not found.”
搜索列表中的特定元素。 如果存在,则中断循环并返回该元素的索引;否则,返回0。 否则返回“未找到”。
l1=[1,3,5,7,9]def findindex(x,l1):
for index,item in enumerate(l1):
if item==x:
return index
break
else:
return "Not found"print (findindex(5,l1))#Output:2print (findindex(10,l1))#Output:Not found
“ while”循环 (‘while’ Loop)
The while
statement is used for repeated execution as long as an expression is true.
只要表达式为真, while
语句将用于重复执行。
while expression:
suiteelse:
suite
This repeatedly tests the expression and, if it is true, executes the first suite. If the expression is false (which it may be the first time it is tested) the suite of the else
clause, if present, is executed and the loop terminates.
这将反复测试表达式,如果为true,则执行第一个套件。 如果表达式为假(可能是第一次测试),则执行else
子句套件(如果存在)的套件,并终止循环。
例子9.在“ while”循环中使用“ else”子句 (Example 9. Using the ‘else’ clause in a ‘while’ loop)
The while
loop is executed until the condition i<5
is False.
执行while
循环,直到条件i<5
为False。
The else
clause is executed after the condition is False.
条件为False后执行else
子句。
i=0while i<5:
print (i)
i+=1else:
print ("Element is not less than 5")'''
Output:
0
1
2
3
4
Element is not less than 5
'''
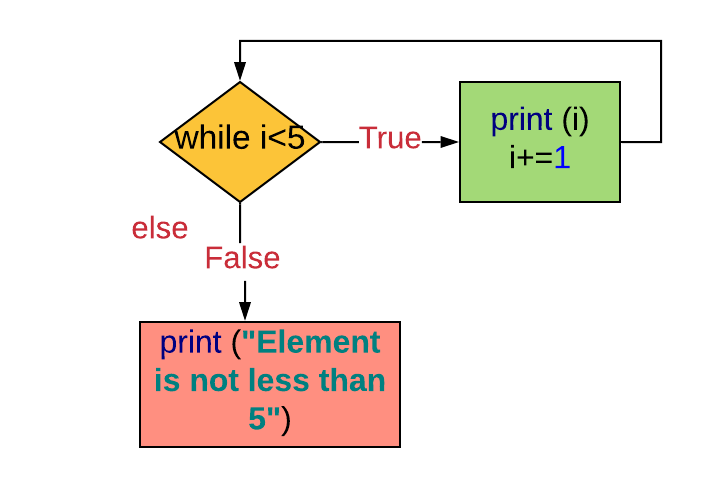
“中断”声明 (‘break’ Statement)
A break
statement executed in the first suite terminates the loop without executing the else
clause’s suite.
在第一个套件中执行的break
语句将终止循环,而不执行else
子句的套件。
例子10.在“ while”循环中使用“ break”语句和“ else”子句 (Example 10. Using the ‘break’ statement and ‘else’ clause in a ‘while’ loop)
The break
statement terminates the loop and the else
clause is not executed.
break
语句终止循环,而else
子句未执行。
i=0while i<5:
print (i)
i+=1
if i==3:
break
else:
print ("Element is not less than 5")'''
Output:
0
1
2
'''
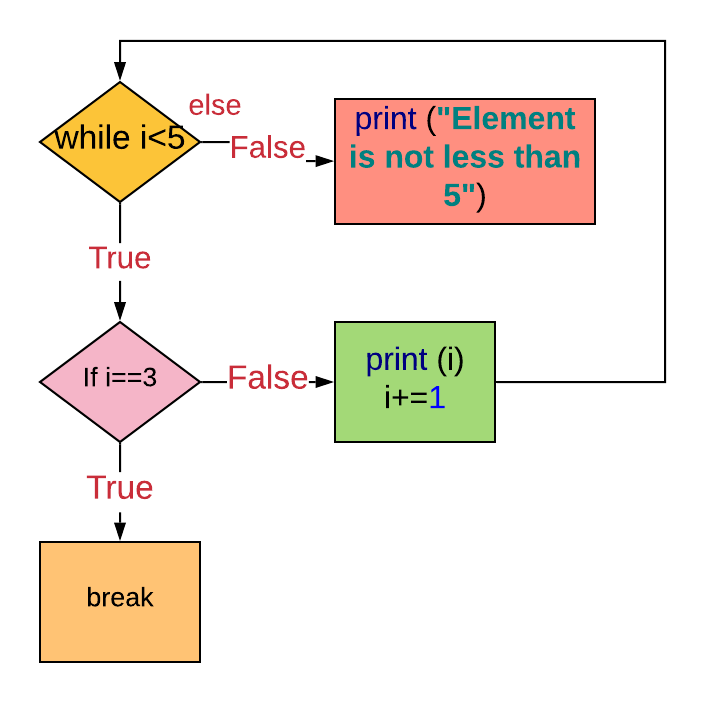
“继续”声明 (‘continue’ Statement)
A continue
statement executed in the first suite skips the rest of the suite and goes back to testing the expression.
在第一个套件中执行的continue
语句将跳过套件的其余部分,然后返回测试表达式。
例子11.在“ while”循环中使用“ continue”语句和“ else”子句 (Example 11. Using the ‘continue’ statement and ‘else’ clause in a ‘while’ loop)
The continue
statement skips the part of the suite when the condition i==3
is True. The control goes back to the while
loop again.
当条件i==3
为True时, continue
语句将跳过套件的一部分。 控件再次返回while
循环。
The else
clause is also executed.
else
子句也将执行。
i=0while i<5:
print (i)
i+=1
if i==3:
continue
else:
print ("Element is not less than 5")'''
Output:
0
1
2
3
4
Element is not less than 5
'''
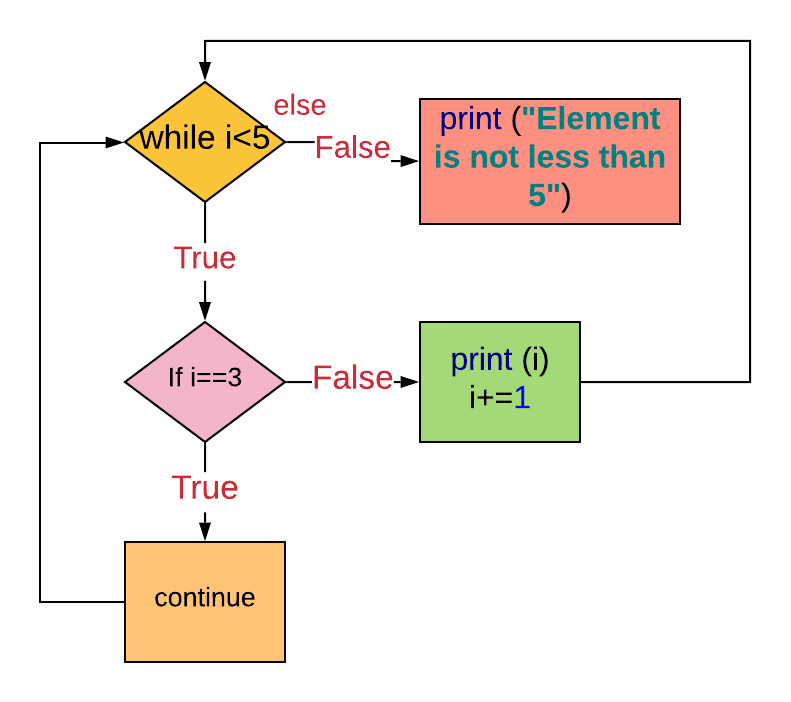
结论 (Conclusion)
Python version used is 3.8.1.
使用的Python版本是 3.8.1。
The
break
statement will terminate the loop (bothfor
andwhile
). Theelse
clause is not executed.break
语句将终止循环(for
和while
)。else
子句不执行。The
continue
statement will skip the rest of the suite and continue with the next item or with theelse
clause, if there is no next item.如果没有下一个项目,则
continue
语句将跳过套件的其余部分,并继续下一个项目或else
子句。The
else
clause is executed when thefor
loop terminates after the exhaustion of the iterable.当
for
循环在迭代器用尽后终止时,将执行else
子句。
资源(Python文档) (Resources (Python Documentation))
break
and continue
Statements, and else
Clauses on Loops
break
并 continue
语句, else
循环中的子句
break
statement in Python
Python中的 break
语句
continue
statement in python
在python中的 continue
语句
for
statement in python
for
python中的语句
翻译自: https://medium.com/better-programming/break-continue-and-else-clauses-on-loops-in-python-b4cdb57d12aa
python分句
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如若转载,请注明出处:http://www.mzph.cn/news/388127.shtml
如若内容造成侵权/违法违规/事实不符,请联系多彩编程网进行投诉反馈email:809451989@qq.com,一经查实,立即删除!